This example adds an object to the Insert bar so users can add a line through (strike through) selected text by clicking a button. This object is useful if a user needs to make editorial comments in a document. Because this example performs text manipulation, you may want to explore some of the objects from the Text pop-up menu in the HTML category on the Insert bar as models. For example, look at the Bold, Emphasis, and Heading object files to see similar functionality, where Dreamweaver wraps a tag around selected text. You will perform the following steps to create the strike-through insert object: - Creating the HTML file
- Adding the JavaScript functions
- Creating the image
- Editing the insertbar.xml file
- Adding a dialog box
- Building an Insert bar pop-up menu
Creating the HTML file The title of the object is specified between the opening and closing title tags. You also specify that the scripting language is JavaScript. To create the HTML file: 1. | Create a new blank file.
| 2. | Add the following code:
<html> <head> <title>Strikethrough</title> <script language="javascript"> </script> </head> <body> </body> </html>
| 3. | Save the file as Strikethrough.htm in the Configuration/Objects/Text folder.
| Adding the JavaScript functions In this example, the JavaScript functions define the behavior and insert code for the Strikethrough object. You must place all the API functions in the HEAD section of the file. The existing object files, such as Configuration/Objects/Text/Em.htm, follow a similar pattern of functions and comments. The first function the object definition file uses is I sDOMRequired(), which tells whether the Design view needs to be synchronized to the existing Code view before execution continues. However, because the Superscript object might be used with many other objects in the Code view, it does not require a forced synchronization. To add the isDOMRequired() function: 1. | In the HEAD section of the Strikethrough.htm file, between the opening and closing script tags, add the following function:
<script language="javascript"> function isDOMRequired() { // Return false, indicating that this object is available in Code view. return false; } </script>
| 2. | Save the file.
| Next, decide whether to use objectTag() or insertObject() for the next function. The Strikethrough object simply wraps the s tag around the selected text, so it doesn't meet the criteria for using the insertObject() function (see "insertObject()" on page 162). Within the objectTag() function, use dw.getFocus() to determine whether the Code view is the current view. If the Code view has input focus, the function should wrap the appropriate (uppercase or lowercase) tag around the selected text. If the Design view has input focus, the function can use dom.applyCharacterMarkup() to assign the formatting to the selected text. Remember that this function works only for supported tags (see dom.applyCharacterMarkup() in the Dreamweaver API Reference). For other tags or operations, you may need to use other API functions. After Dreamweaver applies the formatting, it should return the insertion point (cursor) to the document without any messages or prompting. The following procedure shows how the objectTag() function now reads. To add the objectTag() function: 1. | In the HEAD section of the Strikethrough.htm file, after the isDOMRequired() function, add the following function:
function objectTag() { // Determine if the user is in Code view. var dom = dw.getDocumentDOM(); if (dw.getFocus() == 'textView' || dw.getFocus(true) == 'html'){ var upCaseTag = (dw.getPreferenceString("Source Format", "Tags Upper Case", "") == 'TRUE'); // Manually wrap tags around selection. if (upCaseTag){ dom.source.wrapSelection('<S>','</S>'); }else{ dom.source.wrapSelection('<s>','</s>'); } // If the user is not in Code view, apply the formatting in Design view. }else if (dw.getFocus() == 'document'){ dom.applyCharacterMarkup("s"); } // Just return--don't do anything else. return; }
| 2. | Save the file as Strikethrough.htm in the Configuration/Objects/Text folder.
| Instead of including the JavaScript functions in the HEAD section of the HTML file, you can create a separate JavaScript file. This separate organization is useful for objects that contain several functions, or functions that might be shared by other objects. To separate the HTML object definition file from the supporting JavaScript functions: 1. | Create a new blank file.
| 2. | Paste all the JavaScript functions into the file.
| 3. | Remove the functions from Strikethrough.htm, and add the JavaScript filename to the src attribute of the script tag, as shown in the following example:
<html> <head> <title>Strikethrough</title> <script language="javascript" src="/books/4/533/1/html/2/Strikethrough.js"> </script> </head> <body> </body> </html>
| 4. | Save the Strikethrough.htm file.
| 5. | Save the file that now contains the JavaScript functions as Strikethrough.js in the Configuration/Objects/Text folder.
| Creating the image To create the image for the Insert bar: 1. | Create a GIF image (18 x 18 pixels), as shown in the following figure:
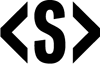
| 2. | Save the file as Strikethrough.gif in the Configuration/Objects/Text folder.
| Editing the insertbar.xml file Next, you need to edit the insertbar.xml file so Dreamweaver can associate these two items with the Insert bar interface. NOTE Before you edit the insertbar.xml file, you might want to copy the original one as insertbar.xml.bak, so you have a backup. The code within the insertbar.xml file identifies all the existing objects on the Insert bar Each category tag in the XML file creates a category in the interface. Each menubutton tag creates a pop-up menu on the Insert bar. Each button tag in the XML file places an icon on the Insert bar and connects it to the proper HTML file or function. To add the new object to the Insert bar: 1. | Find the following line near the beginning of the inserbar.xml file:
<category MMString:name="insertbar/category/common" folder="Common"> This line identifies the beginning of the Common category on the Insert bar.
| 2. | Start a new line after the category tag; then insert the button tag and assign it the id, image, and file attributes for the Strikethrough object.
The ID must be a unique name for the button (following standard naming conventions, use DW_Text_Strikethrough for this object). The image and file attributes simply tell Dreamweaver the location of the supporting files, as shown here:
<button image="Text\Strikethrough.gif" file="Text\Strikethrough.htm"/>
| 3. | Save the insertbar.xml file.
| 4. | Reload the extensions (see "Reloading extensions" on page 104).
| The new object appears at the beginning of the Common category on the Insert bar, as shown in the following figure:  Adding a dialog box You can add a form to your object to let the user enter parameters before Dreamweaver inserts the specified code (for example, the Hyperlink object requests the text, link, target, category index, title, and access key values from the user). In this example, you add a form to the Strikethrough object from the previous example. The form opens a dialog box that provides the user with the option to change the text color to red as well as add the strike-through tag. This example assumes you have already created a separate JavaScript file called Strikethrough.js. First, in Strikethrough.js, you add the function that the form calls if the user changes the text color. This function is similar to the objectTag() function for the Strikethrough object, but is an optional function. To create the function: 1. | After the objectTag() function in Strikethrough.js, create a function called fontColorRed() by entering the following code:
function fontColorRed(){ var dom = dw.getDocumentDOM(); if (dw.getFocus() == 'textView' || dw.getFocus(true) == 'html'){ var upCaseTag = (dw.getPreferenceString("Source Format", "Tags Upper Case", "") == 'TRUE'); // Manually wrap tags around selection. if (upCaseTag){ dom.source.wrapSelection('<FONT COLOR="#FF0000">','</FONT>'); }else{ dom.source.wrapSelection('<font color="#FF0000">','</font>'); } }else if (dw.getFocus() == 'document'){ dom.applyFontMarkup("color", "#FF0000"); } // Just return -- don't do anything else. return; } NOTE Because dom.applyCharacterMarkup() doesn't support font color changes, you need to find the appropriate API function for font color changes. (For more information, see dom.applyFontMarkup() in the Dreamweaver API Reference). | 2. | Save the file as Strikethrough.js.
| Next, in the Strikethrough.htm file, you add the form. The form for this example is a simple checkbox that calls the fontColorRed() function when the user clicks on it. You use the form tag to define your form, and the table tag for layout control (otherwise, the dialog box might wrap words or size awkwardly). To add the form: 1. | After the body tag, add the following code:
<form> <table border="0" height="100" width="100"> <tr valign="baseline"> <td align="left" nowrap> <input type="checkbox" name="red" onClick=fontColorRed()>Red text</input> </td> </tr> </table> </form>
| 2. | Save the file as Strikethrough.htm.
| 3. | Reload the extensions (see "Reloading extensions" on page 104).
| To test the dialog box: 1. | Click the Red Text checkbox.
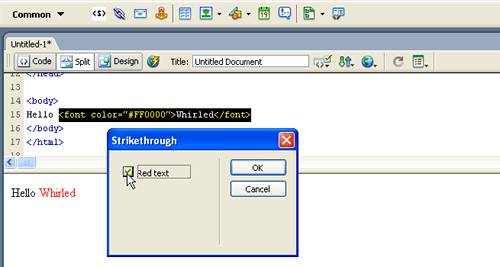 | 2. | Click OK to perform the objectTag() function, which adds the strike-through:
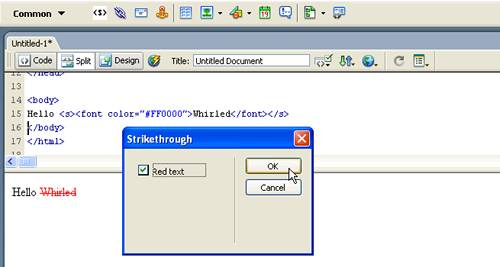 | Building an Insert bar pop-up menu The Dreamweaver Insert bar introduces a new organization for objects and now supports pop-up menus to help organize objects into smaller groups, as shown in the following figure. 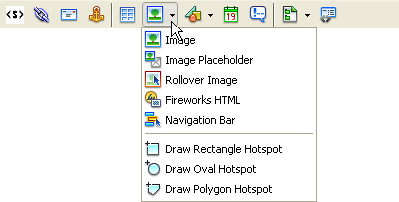 The following example builds a new category on the Insert bar called Editorial and then adds a pop-up menu to that category. The pop-up menu contains the Strikethrough object you already created and groups it with a Blue Text object you will create. The objects in the Editorial category let users make editorial comments in a file and either strike through the content they want to remove or make new content blue. To organize the files: 1. | Create a new Configuration/Objects/Editorial folder in your Dreamweaver installation folder.
| 2. | Copy the files for the Strikethrough object example you created (Strikethrough.htm, Strikethrough.js, and Strikethrough.gif) to the Editorial folder.
| To create the Blue Text object: 1. | Create a new HTML file.
| 2. | Add the following code:
<html> <head> <title>Blue Text</title> <script language="javascript"> //--------------- API FUNCTIONS --------------- function isDOMRequired() { // Return false, indicating that this object is available in Code view. return false; } function objectTag() { // Manually wrap tags around selection. var dom = dw.getDocumentDOM(); if (dw.getFocus() == 'textView' || dw.getFocus(true) == 'html'){ var upCaseTag = (dw.getPreferenceString("Source Format", "Tags Upper Case", "") == 'TRUE'); // Manually wrap tags around selection. if (upCaseTag){ dom.source.wrapSelection('<FONT COLOR="#0000FF">','</FONT>'); }else{ dom.source.wrapSelection('<font color="#0000FF">','</font>'); } }else if (dw.getFocus() == 'document'){ dom.applyFontMarkup("color", "#0000FF"); } // Just return -- don't do anything else. return; } </script> </head> <body> </body> </html>
| 3. | Save the file as AddBlue.htm in the Editorial folder.
| Now you can create an image for the Blue Text Object. To create the image: 1. | Create a GIF file that is 18 x 18 pixels, which will look like the following figure:
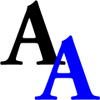
| 2. | Save the image as AddBlue.gif in the Editorial folder.
| Next, you edit the insertbar.xml file. This file defines the objects on the Insert bar and their locations. Notice the various menubutton tags and their attributes within the category tags; these menubutton tags define each pop-up menu in the HTML category. Within the menubutton tags, each button tag defines an item in the pop-up menu. To edit insertbar.xml: 1. | Find the following line of code near the beginning of the file:
<insertbar xmlns:MMString="http://www.macromedia.com/schemes/data/string/"> The insertbar tag defines the beginning of all the Insert bar contents.
| 2. | After that line, add a new category tag for the Editorial category you want to create, giving it unique ID, name, and folder attributes, and then add a closing category tag, as shown in the following example:
<category name="Editorial" folder="Editorial"> </category>
| 3. | Reload extensions. For information on reloading extensions, see "Reloading extensions" on page 104.
The Editorial category appears on the Insert bar:
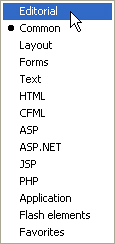
| 4. | Within the opening and closing category tags, add the pop-up menu by using the menubutton tag and the following attributes, including a unique ID.
<menubutton name="markup" image="Editorial\Strikethrough.gif" folder="Editorial"> For more information about attributes, see "Insert bar definition tag attributes" on page 144.
| 5. | Add the objects for the new pop-up menu using the button tag, as follows:
<button image="Editorial\Strikethrough.gif" file="Editorial\Strikethrough.htm"/>
| 6. | After the Strikethrough object button tag, add the hypertext object, as follows:
<button image="Editorial\AddBlue.gif name="Blue Text" file="Editorial \AddBlue.htm"/> NOTE The button tag does not have a separate closing tag; it simply ends with "/>". | 7. | End the pop-up menu with the </menubutton> closing tag.
| The following code shows your entire category with the pop-up menu and the two objects: <category name="Editorial" folder="Editorial"> <menubutton name="markup" image="Editorial\Strikethrough.gif" folder="Editorial"> <button image="Editorial\Strikethrough.gif" file="Editorial\Strikethrough.htm"/> <button image="Editorial\AddBlue.gif" name="Blue Text" file="Editorial\AddBlue.htm"/> </menubutton> </category> To test the new pop-up menu: 1. | Reload extensions. For information on reloading extensions, see "Reloading extensions" on page 104.
| 2. | Click the Editorial menu.
The following pop-up menu appears:
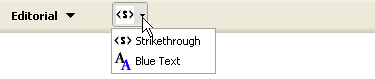
| |