If you're like most other beginning XML developers, you might have asked yourself "How can anyone communicate using XML? Since every set of XML data uses different tags, how can anyone know what's supposed to be in the data?" You won't have been alone in asking yourself these questions. The answer is that without more information, XML is just data, and there's no way to determine whether it's the correct data. Sure, you can tell whether the XML is well-formed (you saw how you can verify that in the previous section). But you need more information to determine that the contents of the data are valid. The XML Schema specification provides XML elements that describe the contents of other XML data. XML Schema is a rich specification, allowing for simple and complex data types, including all the standard types you're used to working with, and many more. An XML Schema corresponding to the XML data you've been working with so far might look like the XML shown in Listing 27.2. As you can see, the XML Schema document shown in Listing 27.2 contains specifications for data types, the minimum number of occurrences, element and attributes names and types, and the XML root element. TIP Although it's not required, most XML Schema documents are saved in files with the .xsd extension. For a file named EmployeeData.xml, you will most likely see a corresponding schema named EmployeeData.xsd. This convention isn't required but is very common. Listing 27.2 XML Schema Completely Defines the Contents of XML Data, in XML Itself <?xml version="1.0" ?> <xs:schema targetNamespace="http://tempuri.org/EmployeeData.xsd" xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="qualified" elementFormDefault="qualified"> <xs:element name="Employees"> <xs:complexType> <xs:choice maxOccurs="unbounded"> <xs:element name="Employee"> <xs:complexType> <xs:sequence> <xs:element name="FirstName" type="xs:string" minOccurs="0" /> <xs:element name="LastName" type="xs:string" minOccurs="0" /> </xs:sequence> <xs:attribute name="ID" form="unqualified" type="xs:string" /> </xs:complexType> </xs:element> </xs:choice> </xs:complexType> </xs:element> </xs:schema> Although you can create XML Schema content yourself, doing so is tedious and error prone. You're more likely to create the data (or simply receive the XML data from another source) and have some tool or another generate a starting point schema for you. Visual Studio .NET does a good job at inferring schema, and in the next section you'll work through the details of creating a schema based on the XML data you've been working with in this chapter and then modifying the schema to add more information. TIP For more information about XML Schema, and about XML in general, drop by Microsoft's XML site: http://msdn.microsoft.com/xml. As is the case with http://www.w3.org, you'll find the Microsoft site full of information about all aspects of XML, but from a slightly Microsoftian perspective.  | Follow these steps to create, modify, and test an XML Schema corresponding to the XML data you've been using so far in this chapter: | -
In the Solution Explorer window, double-click EmployeeData.xml, loading the data into the XML editor window. -
Select the XML, Create Schema menu item. You should immediately see EmployeeData.xsd in the Solution Explorer window. TIP Your XML data should now include a reference to the newly generated schema, in the Employees root element. This reference links the two files together. -
In the Solution Explorer window, double-click EmployeeData.xsd, displaying the designer shown in Figure 27.5. Figure 27.5. Visual Studio includes a full-featured schema editor.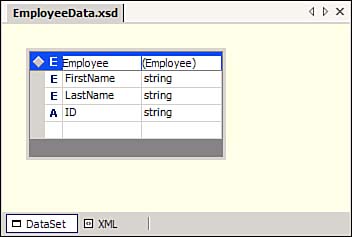 TIP If you click the XML tab at the bottom of the schema designer window, you'll see the same XML shown in Listing 27.2-the XML providing the schema for the EmployeeData.xml data. Over the next few steps, your goal will be to make the ID attribute required (that is, any Employee element that doesn't contain an ID attribute will cause the document to be invalid) and to add an optional element named Phone. Follow these steps to modify the schema and then test it: -
Click the DataSet tab at the bottom of the window to make sure you're viewing the XSD designer. In the final, empty row in the schema designer grid, click the first column. -
Click the arrow next to the cell and select Element from the drop-down list. (Note that the designer moves your new row up, above the ID attribute.) -
Enter Phone for the name of the element and select string for the data type. -
Select the ID attribute in the grid and then press F4 to display the Properties window (you can also use the View, Properties menu item). -
Set the use property to required. -
Save the changes to your schema. -
In the Solution Explorer window, double-click EmployeeData.xml to load the file back into the XML editor window. -
Click the XML tab at the bottom of the window to ensure that you're working with the XML data directly. -
Below the final </Employee> tag (the end of the last employee in the data), create a new employee named Stephen Buchanan. Enter only the first and last names. When you're done, the new element should look like this: <Employee> <FirstName>Stephen</FirstName> <LastName>Buchanan</LastName> </Employee> TIP Amazed to see IntelliSense support in the XML editor? Why is it available now when it wasn't available earlier? The answer is simple: an XML Schema. Because you've associated a schema with the XML document, the editor can now help you out as you type. This is exactly the same thing that happens when you enter HTML data directly into the HTML view of an ASP.NET Web form the designer looks up the schema for the page, including all its controls, in an XML Schema document that ships with Visual Studio .NET. -
Choose the XML, Validate XML Data menu item, which attempts to validate the data against the schema. It should fail, because you haven't supplied the required ID attribute for the new employee. -
Add the ID attribute (Stephen Buchanan is employee 5) and then validate the data again. This time, it should succeed. -
Although the schema supports an optional Phone element, it won't allow you to enter any other elements. Try adding an Address element within an Employee element, and the editor will immediately prompt you with a warning. Remove the offending element and then save the project. Using XML Schema Once your project contains both XML data and a schema, you have everything you need to take advantage of XML on an ASP.NET page. You could, for example, bind a DataGrid control to the contents of the XML file. In order to use the XML data, you'll need to generate a typed DataSet class based on the XML data/schema. Follow these steps to create the DataSet and then bind a DataGrid to the data: -
In the Solution Explorer window, double-click EmployeeData.xsd to load the schema into the Schema Designer window. -
Select the Schema, Generate DataSet menu item, creating a code-behind file for the schema. (Use the Project, Show All Files menu item to see the new EmployeeData.vb file.) -
Select the Project, Add Web Form menu item to add a new page to your project. Name the new page EmployeesXML.aspx. -
In the Toolbox window, select the Data tab. -
Drag a DataSet object onto your page. This displays the Add DataSet dialog box. In the dialog box, select the typed DataSet named Northwind.EmployeeData1 that you created in an earlier step. Click OK to dismiss the dialog box, and your page should now include a DataSet object named EmployeesData1 in its tray area. -
In the Toolbox window, select the Web Forms tab. -
Place a DataGrid control on your new page. Set the DataGrid control's DataSource property to EmployeeData1 (the DataSet object you just created). -
Double-click the page (not on the DataGrid control) and modify the Page_Load procedure so that it looks like the code in Listing 27.3. Listing 27.3 Bind a DataGrid to the Contents of an XML File Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles MyBase.Load If Not Page.IsPostBack Then EmployeeData1.ReadXml( _ Server.MapPath("EmployeeData.xml")) DataGrid1.DataBind() End If End Sub -
In the Solution Explorer window, right-click EmployeesXML.aspx and select Build and Browse from the context menu. The page should display the contents of the XML file in the DataGrid control, as shown in Figure 27.6. Figure 27.6. It's easy to display the contents of an XML file within a DataGrid control.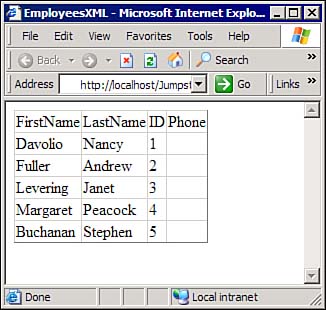 What's going on in the code? The second line (calling the DataBind method of the DataGrid control) looks familiar, but what about the call to the ReadXml method of the DataSet? When you created the DataSet object (EmployeesData1) on the page, Visual Studio .NET created a reference to the EmployeeData.vb class you created when you selected the Generate DataSet menu item. You can see this in the page's code-behind file, near the top of the code: Protected WithEvents EmployeesData1 As Northwind.Employees Normally, you fill a DataSet using the Fill method of a DataAdapter object. In this case, because you're reading data from an XML file rather than from a database, you simply call the ReadXml method of the DataSet, passing in the name of the XML file to read from. You must also indicate the full path of the XML file. The Server.MapPath method takes as its parameter the name of a file in the application's folder and returns the full path for the file within the file system. Calling the ReadXml method, given the full path of the XML file, loads the DataSet so that the DataGrid can display the data. TIP It's interesting to note that you see the Phone column in the grid, even though none of the elements within the XML file contain this information. How did the DataGrid find this column? Obviously, when you created the typed DataSet object from the XML Schema in which you added the Phone element, that information was copied into the DataSet. Although you've read the actual data from the XML file, the schema was set at the time you created the typed DataSet. |