In this section, we'll introduce the major portions of the sample application you'll be building and investigating as you work through this book. To get the most out of this book, we assume you are able to perform the following tasks: Create programs in some programming language (preferably Visual Basic or VBScript) Create a virtual directory in IIS Create basic HTML Web pages Write basic SQL statements At this point, it's worth taking a few minutes to see what types of pages you'll be creating and how they display data from the Northwind sample database. We've described the functionality of each page and what you can expect to learn from each example. The Main Page The main page, shown in Figure 1.1, provides a jumping-off place for the rest of the application. On this page, you'll learn: How to create hyperlinks using HyperLink controls How to use LinkButton controls How to dynamically choose which hyperlinks to display How to navigate to other pages Figure 1.1. Use main.aspx to navigate to all your other pages.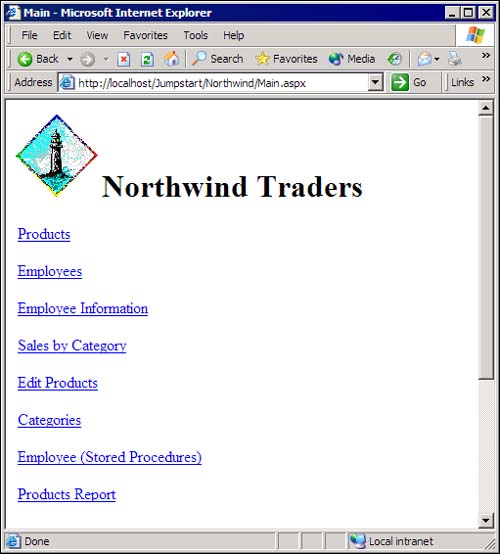 The Login Page You'll use the login page, shown in Figure 1.2, to allow users to log in to your application. In the beginning of this book, you will use this page to restrict access to certain menu items. Later, you'll learn to use this page as a required login page, disallowing access to the site until the user has successfully authenticated. (In the sample application, one of the valid login ID/password pairs is King/465.) Figure 1.2. The Login page helps you to secure your site against unwanted intruders.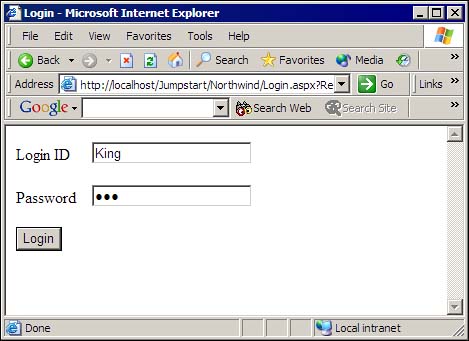 On this page, you'll learn: How to respond to Click events on Button controls How to set Session variables How to use Forms-based authentication How to redirect to a starting page if a user enters valid authentication information How to authenticate users against data in a database The Products Page The Products.aspx page, shown in Figure 1.3, uses a number of different controls and displays data from Microsoft SQL Server. You'll fill DropDownList controls, a DataGrid control, and Label controls with data from several tables in the Northwind database. Figure 1.3. The Products.aspx page shows how to respond to many different events on different controls.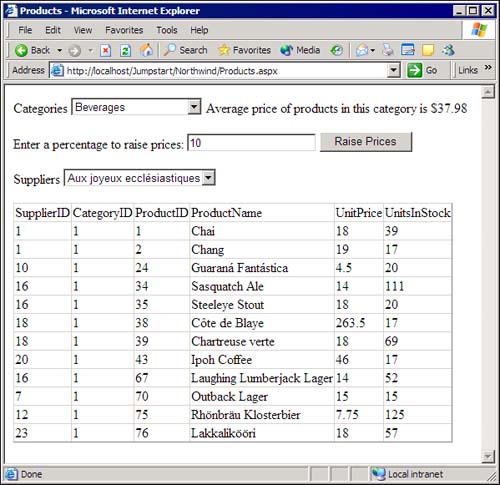 On this page, you'll learn: How to react to handle control events How to use the ADO.NET DataSet object How to configure a DataAdapter so that it can retrieve data How to fill a DropDownList control using a DataReader How to use Connection and Command objects How to execute action queries The Employees and Orders Page The Employees.aspx page, shown in Figure 1.4, displays a drop-down list containing employee names. When you select an employee, the page displays orders for the selected employee in a DataGrid control. Figure 1.4. The Employees.aspx page shows that setting relationships between tables is easy with the DataSet object.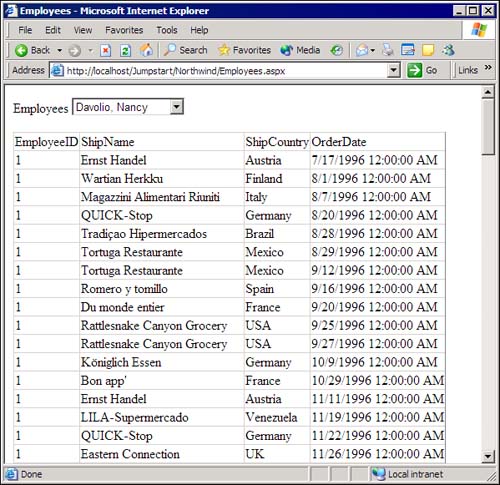 On this page, you'll learn: How to build a DataSet containing multiple DataTable objects How to add a relationship between DataTable objects How to retrieve orders based on a selected employee, using parent/child relationships How to store and retrieve a DataSet using a Session variable The Employees (Stored Procedures) Page The EmployeeSP.aspx page, shown in Figure 1.5, will show you how to work with SQL Server stored procedures. This page demonstrates calling several stored procedures that you'll add to the Northwind sample database. Figure 1.5. The EmployeeSP.aspx page shows you how to call stored procedures using both OleDb and SqlClient namespaces.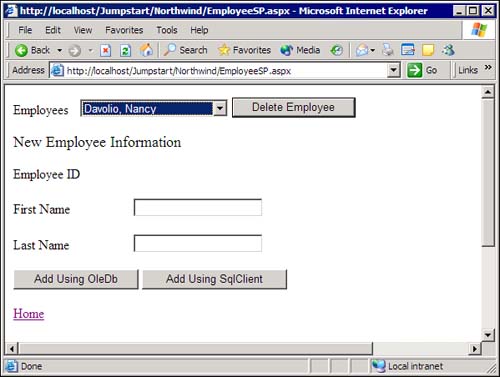 On this page, you'll learn: How to call stored procedures How to pass parameters to stored procedures How to retrieve output parameters returned from a stored procedure How to use both the OleDb and SqlClient namespaces, and why you would use one or the other Differences between the OleDb and SqlClient namespaces, with regards to stored procedures The Sales by Category Page The CategorySales.aspx page, shown in Figure 1.6, shows you techniques for formatting, sorting, and selecting items within the DataGrid control. The DataGrid control is incredibly powerful, and this page demonstrates many of the useful features of this control. Figure 1.6. The DataGrid control can be formatted in many different ways, and you'll use several useful techniques on this page.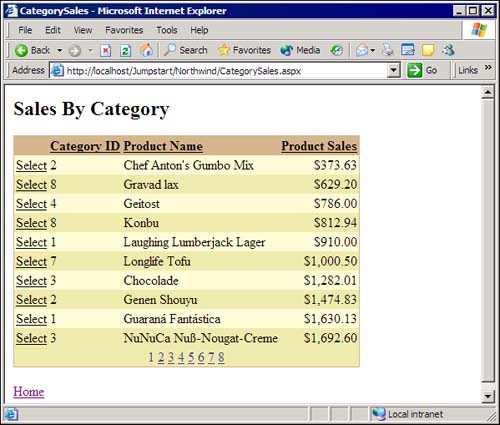 On this page, you'll learn: How to format columns in a DataGrid control How to sort a DataGrid's data by clicking the column header How to select an item and respond to the ItemCommand event procedure How to pass information to the ItemCommand event procedure How to enable paging on a DataGrid control The Edit Products Page The DataGrid control not only allows you to display data, it allows you to edit data in place, as well. The ProductsEdit.aspx page, shown in Figure 1.7, will allow you to edit product information within the grid as well as edit detail information on a separate page. Figure 1.7. The tasks of adding, editing, and deleting on a DataGrid control are relatively easy, but they do require some care. This page shows you how.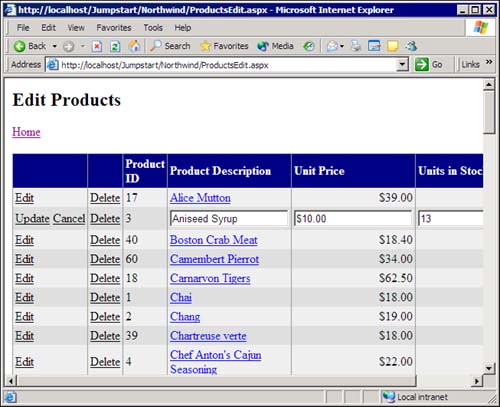 On this page, you'll learn: How to edit data "in place" in the DataGrid control How to delete a row from a DataGrid and the underlying database table How to add a row to a DataGrid control and the underlying database table How to add a hyperlink to a column of data so that selecting an item from the column navigates to a detail editing page How to use template columns in a DataGrid control The Product Details Page When you select a link on the ProductsEdit.aspx page, shown in Figure 1.7, you navigate to the ProductDetail.aspx page, shown in Figure 1.8. This page shows you how to add or edit data on a "detail page" and save that data back to the underlying database table. Figure 1.8. A detail page is somewhat easier to enter data into than editing directly on the DataGrid control. This page shows how you can link a detail page and a DataGrid control.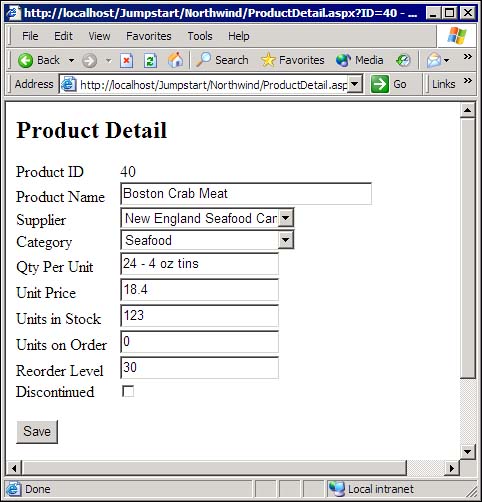 On this page, you'll learn: How to add or edit data retrieved from a DataGrid control How to submit data back to the database How to position a DropDownList control to a specified value The Categories Page The Categories.aspx page, shown in Figure 1.9, demonstrates how to display bound lists, drawing items from a data source. Figure 1.9. Using the Repeater allows you to customize the display of the data using any HTML templates. In this case, the item template displays a bulleted list.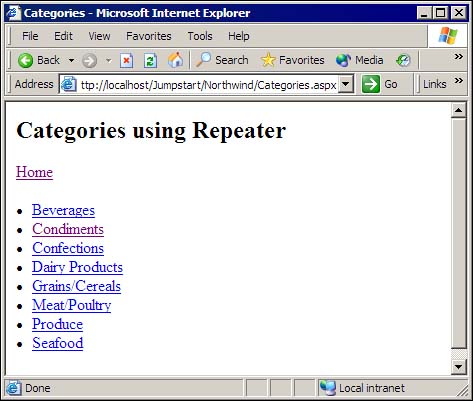 On this page, you'll learn: How to use the Repeater control How to create header, footer, and item templates How to load data into the Repeater control How to display links using the Repeater control The Category Detail Page The CategoryDetail.aspx page, shown in Figure 1.10, shows another use of the Repeater control. In this case, the item template is more complex and displays data in a nontabular format. Figure 1.10. You can create complex formatting using the Repeater control because you decide how to lay out the various data elements.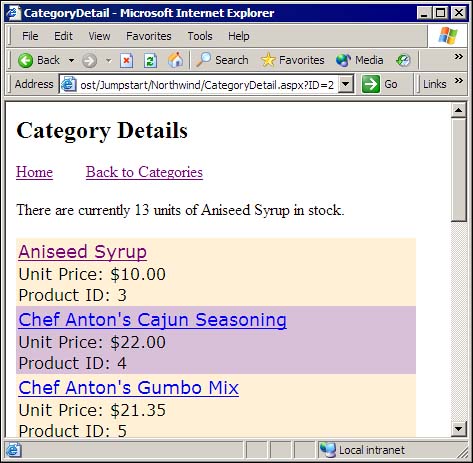 On this page, you'll learn: The Employee Information Page The EmployeeInfo.aspx page, shown in Figure 1.11, shows you how to display images and detail information and how to edit the data. This page also demonstrates how to display data in multiple rows and columns (as you might when displaying data for a phone list or items available for sale). Figure 1.11. The DataList control is the most flexible control available if you want to display multiple rows and columns of data.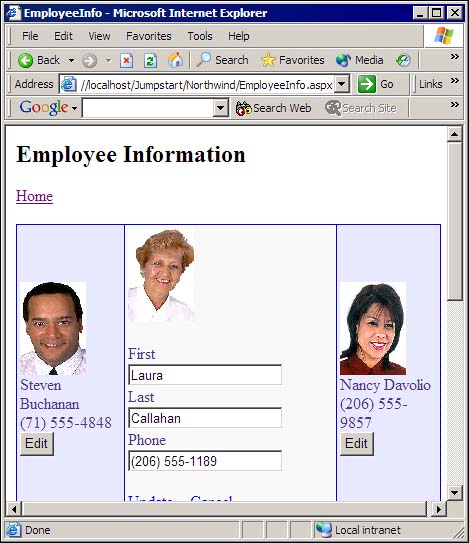 On this page, you'll learn: How to use the DataList control How to display rows of data using the DataList control and its HTML templates How to use the HTML designers for DataList templates How to add, edit, and delete data using the DataList control The Employee Maintenance Page The EmpMaint.aspx page, shown in Figure 1.12, shows how you can validate data on an ASP.NET page. This page demonstrates several different techniques for validating data, including requiring input, ensuring data is within a certain range, and running your own code in order to validate data. Figure 1.12. Validating data is simple using the ASP.NET validation controls.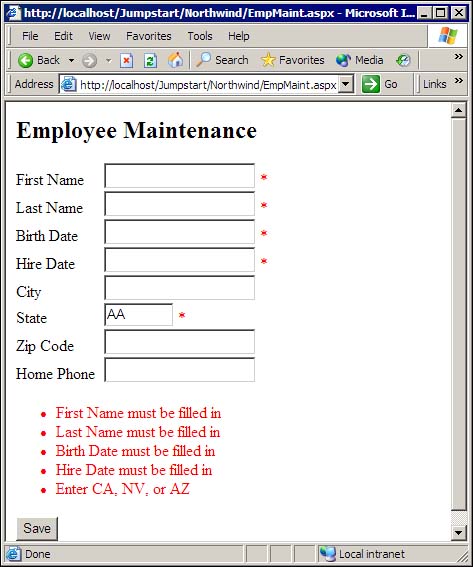 On this page, you'll learn: How to use all the validation controls, including the RequiredFieldValidator, RegularExpressionValidator, and RangeValidator controls How to display a validation summary using the ValidationSummary control How to create your own validation procedures for use with the CustomValidator control The Change Password Page The PwdChange.aspx page, shown in Figure 1.13, shows how you can compare two values and disallow posting the page until the values are the same. This is a common technique used when asking for a new user password. On this page, you'll learn how to use the CompareValidator control. Figure 1.13. Use the CompareValidator control to verify that two values match.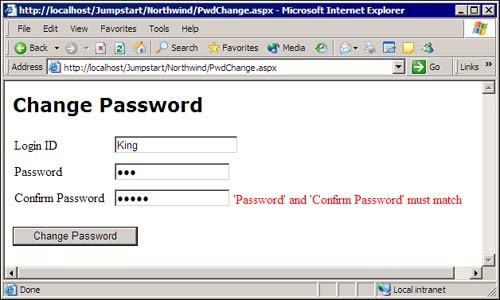 Using the Debugger The Jumpstart\Debugger\Debugger.sln solution, shown in Figure 1.14, walks you through using many of the features of the debugger provided by Visual Studio .NET. Figure 1.14. Learn to use the Visual Studio .NET debugger with this project.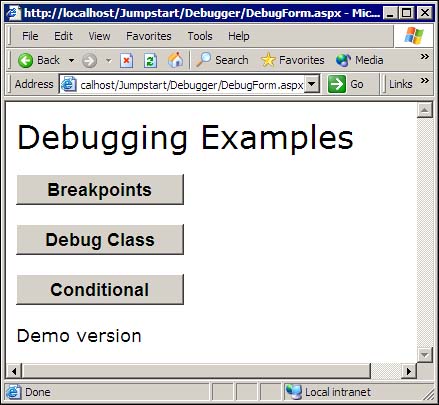 In this solution, you'll learn: How to set breakpoints How to use the Debug class How to use WriteLine and Assert How to use conditional compilation Handling Errors The Jumpstart\ErrorHandling\ErrorHandling.sln solution, shown in Figure 1.15, walks you through structured exception handling, which is new in Visual Basic .NET. Figure 1.15. Demonstrate various types of exception handling using this sample project.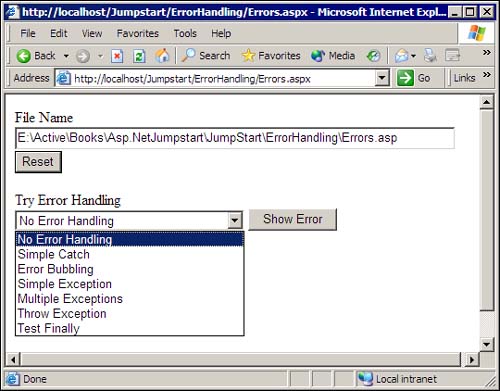 In this solution, you'll learn: How to add exception handling to your code How to use the Try and Catch keywords How to retrieve exception information How to add code that runs whether or not an exception occurs using the Finally keyword Using the Framework Classes The Jumpstart\Framework\FrameworkClasses.sln solution, shown in Figure 1.16, introduces and demonstrates several objects and namespaces (selected from the thousands of available objects and namespaces) provided by the .NET Framework. Figure 1.16. This page navigates to sample pages, testing out several of the .NET Framework classes.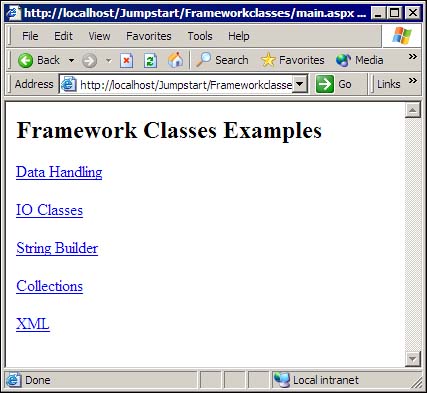 In this solution, you'll learn: How to use the OleDb and SqlClient namespaces How to write to a file using the System.IO namespace How to use the StringBuilder class How to use the Collection classes How to use XML classes Using Other ASP.NET Controls The Jumpstart\MiscWebControls\MiscWebControls.sln solution, shown in Figure 1.17, walks you through using many of the ASP.NET controls not covered elsewhere in this book. The .NET Framework provides a rich palette of server controls, and some of them just didn't fit into the Northwind project you'll create. Figure 1.17. Learn how to use the RadioButtonList, CheckBoxList, AdRotator, Calendar, Literal, and Placeholder controls in this solution.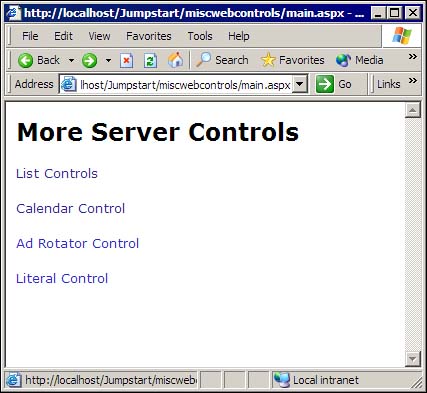 In this solution, you'll learn: How to use the CheckBoxList and RadioButtonList controls to select from a list of values How to use the Calendar control to select dates How to use the AdRotator control to display randomly selected images from an XML file containing a list of available images How to use the Literal control to inject HTML into a page How to use the Placeholder control to reserve space for dynamically created controls Using the Mobile Internet Toolkit The Jumpstart\MITSample\MITSample.sln solution, shown in Figure 1.18, walks you through creating a simple page using the Mobile Internet Toolkit, showing you how to create Web applications that can be browsed from multiple handheld devices. Figure 1.18. The Mobile Internet Toolkit allows you to use Visual Studio .NET to create mobile applications.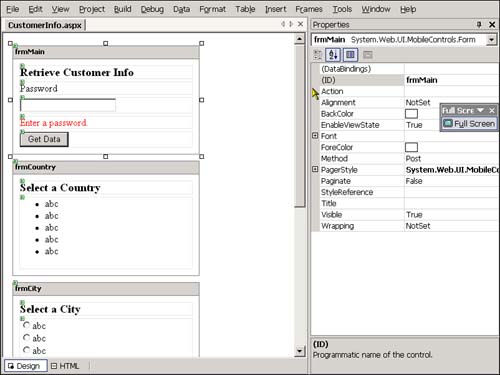 In this solution, you'll learn: How to build a Web application targeted to mobile devices How to use the server controls provided by the Microsoft Mobile Internet Toolkit How to display data on mobile Web forms Using State Management The Jumpstart\StateMgmt\StateMgmt.sln solution, shown in Figure 1.19, demonstrates using the built-in features of state management in the .NET Framework. Figure 1.19. The .NET Framework provides multiple ways in which you can manage state within your applications. This solution walks you through several of these techniques.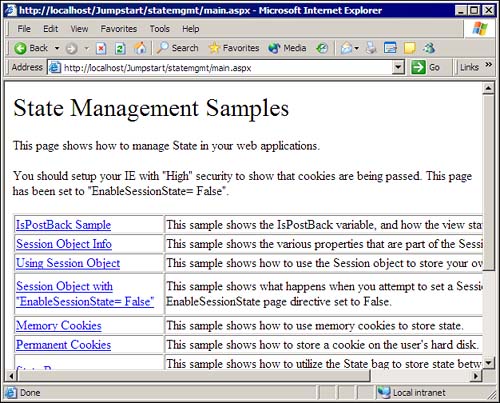 In this solution, you'll learn: How to manage state using cookies How to manage state using StateBag objects How to manage state using the ASP.NET State service How to manage state using SQL Server Introducing Visual Basic .NET Language Basics The Jumpstart\VBLanguage\VBLanguage.sln solution, shown in Figure 1.20, introduces you to many of the new features of the Visual Basic .NET language. Figure 1.20. Learn features of Visual Basic .NET using this project.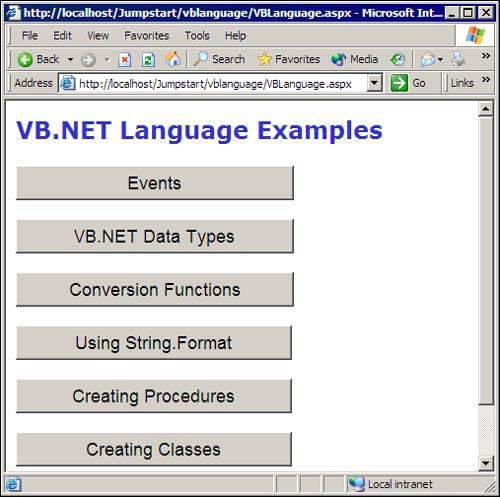 In this solution, you'll learn: How to respond to events Which data types are available in VB .NET How to convert data from one type to another How to create procedures How to create classes How to use Shared methods in classes |