In the preceding example, a new version of the view_users.php script was written. This one now includes links to the edit_user.php and delete_user.php pages, passing each a user's ID through the URL. This next example, delete_user.php, will take the passed user ID and allow the administrator to delete that user. Although you could have this page simply execute a DELETE query on the database, for security purposes (and to prevent an inadvertent deletion), there will be multiple steps: 1. | The page must check that it received a numeric user ID.
| 2. | A message will confirm that this user should be deleted.
| 3. | The user ID will be stored in a hidden form input.
| 4. | Upon submission of this form, the user will actually be deleted.
| To use hidden form inputs 1. | Create a new PHP document in your text editor or IDE (Script 8.3).
<?php # Script 8.3 - delete_user.php Script 8.3. This script expects a user ID to be passed to it through the URL. It then presents a confirmation form and deletes the user upon submission. 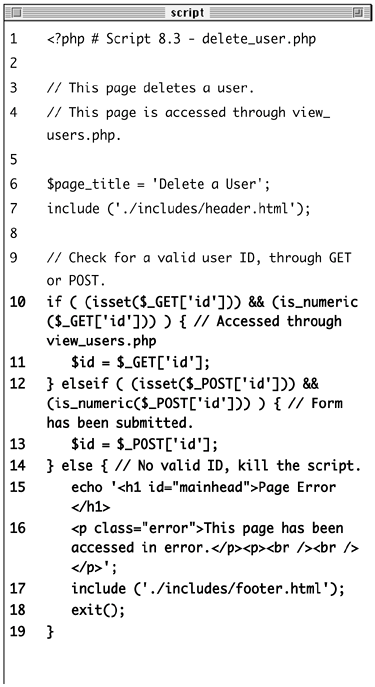 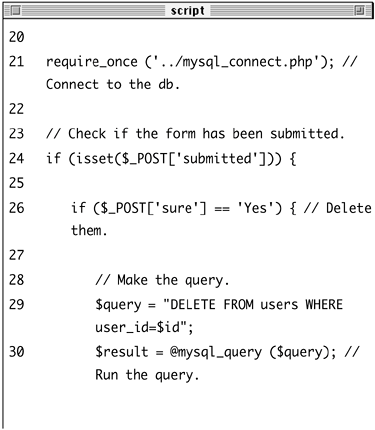 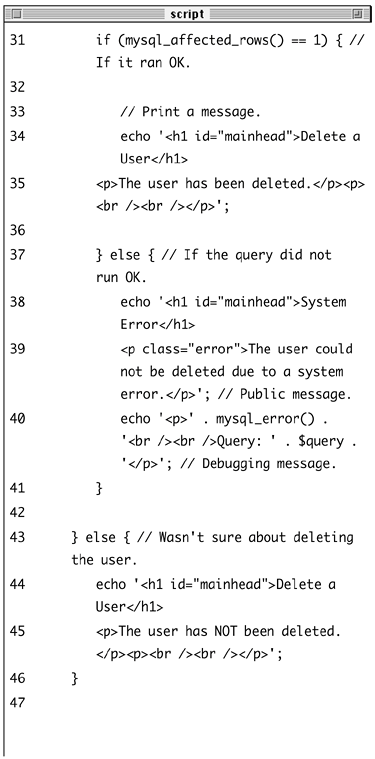 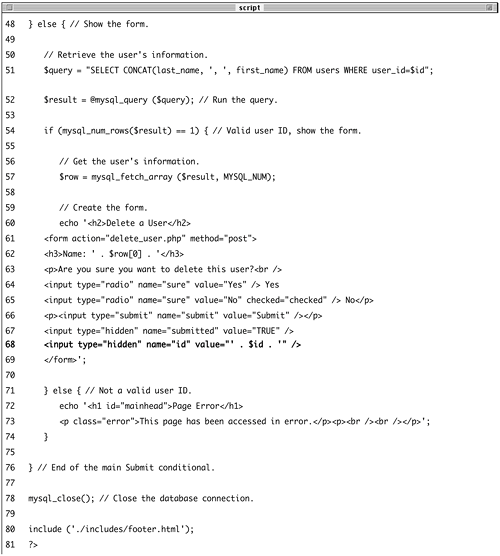
| 2. | Include the page header.
$page_title = 'Delete a User'; include ('./includes/header.html'); This document will use the same template system as the other pages in the application.
| 3. | Check for a valid user ID value.
if ( (isset($_GET['id'])) && (is_numeric($_GET['id])) ) { $id = $_GET['id']; } elseif ( (isset($_POST['id'])) && (is_numeric($_POST['id])) ) { $id = $_POST['id']; } else { echo '<h1 > Page Error</h1> <p >This page has been accessed in error.</p><p> <br /><br /></p>'; include ('./includes/footer. html); exit(); } This script relies upon having a valid user ID, which will be used in a DELETE query's WHERE clause. The first time this page is accessed, the user ID should be passed in the URL (the page's URL will end with delete_user.php?id=X), after clicking the Delete link in the view_users.php page. The first if condition checks for such a value and that the value is numeric.
As you will see, the script will then store the user ID value in a hidden form input. When the form is submitted (back to this same page), the page will receive the ID through $_POST. The second condition checks this and, again, that the ID value is numeric.
If neither of these conditions are trUE, then the page cannot proceed, so an error message is displayed and the script's execution is terminated.
| 4. | Include the MySQL connection script.
require_once ('../mysql_connect. php); Both of this script's processesshowing the form and handling the formrequire a database connection, so this line is outside of the main submit conditional (Step 5).
| 5. | Begin the main submit conditional.
if (isset($_POST['submitted'])) {
| 6. | Delete the user, if appropriate.
if ($_POST['sure'] == 'Yes') { $query = "DELETE FROM users WHERE user_id=$id; $result = @mysql_query ($query); The form (Figure 8.3) will make the user click a radio button to confirm the deletion. This little step prevents any accidents. Thus, the handling process first checks that the right radio button was selected. If so, a basic DELETE query is defined, using the user's ID in the WHERE clause.
Figure 8.3. The page confirms the user deletion using this simple form. 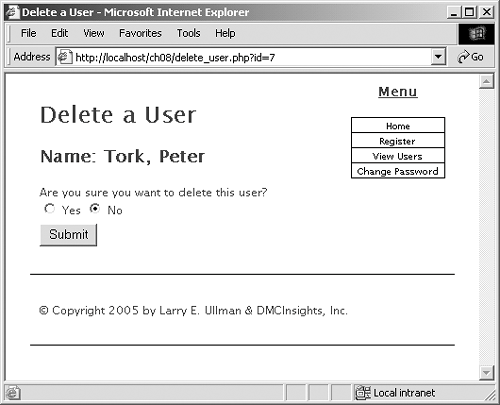
| 7. | Check if the deletion worked and respond accordingly.
if (mysql_affected_rows() == 1) { echo '<h1 >Delete a User</h1> <p>The user has been deleted. </p><p><br /><br /></p>'; } else { echo '<h1 >System Error</h1> <p >The user could not be deleted due to a system error.</p>'; echo '<p>' . mysql_error() . '<br /><br />Query: ' . $query . '</p>'; } The mysql_affected_rows() function checks that exactly one row was affected by the DELETE query. If so, a happy message is displayed. If not, an error message is sent out.
Keep in mind that it's possible that no rows were affected without a MySQL error occurring. For example, if the query tries to delete the record where the user ID is equal to 42000 (which presumably doesn't exist), no rows will be deleted but no MySQL error will occur.
| 8. | Complete the $_POST['sure'] conditional.
} else { echo '<h1 >Delete a User</h1> <p>The user has NOT been deleted. </p><p><br /><br /></p>'; } If the page user did not explicitly check the Yes box, the user will not be deleted and this message is displayed.
| 9. | Begin the else clause of the main submit conditional.
} else { The page will either handle the form or display it. Most of the code prior to this takes effect if the form has been submitted (if $_POST['submitted'] is set). The code from here on takes effect if the form has not yet been submitted, in which case the form should be displayed.
| 10. | Retrieve the information for the user being deleted.
$query = "SELECT CONCAT(last_name, ', ', first_name) FROM users WHERE user_id=$id; $result = @mysql_query ($query); if (mysql_num_rows($result) == 1) { To confirm that the script received a valid user ID and to state exactly who is being deleted (refer back to Figure 8.3), the to-be-deleted user's name is retrieved from the database.
The conditionalchecking that a single row was returnedensures that a valid user ID was provided.
| 11. | Display the form.
$row = mysql_fetch_array ($result, MYSQL_NUM); echo '<h2>Delete a User</h2> <form action="delete_user.php" method="post> <h3>Name: ' . $row[0] . '</h3> <p>Are you sure you want to delete this user?<br /> <input type="radio" name="sure" value="Yes /> Yes <input type="radio" name="sure" value="No checked="checked" /> No</p> <p><input type="submit" name= "submit value="Submit" /></p> <input type="hidden" name= "submitted value="TRUE" /> <input type="hidden" name="id" value="' . $id . '" /> </form>'; First, the database information is retrieved using the mysql_fetch_array() function. Then the form is printed, showing the name value retrieved from the database at the top. An important step here is that the user ID ($id) is stored as a hidden form input so that the handling process can also access this value.
| 12. | Complete the mysql_num_rows() conditional.
} else { echo '<h1 >Page Error</h1> <p >This page has been accessed in error.</p> <p><br /><br /></p>'; } If no record was returned from the database (because an invalid user ID was submitted), this message is displayed.
If you are unsure of your MySQL query, you could call the mysql_error() function here and print out the query to check for errors. Again though, an invalid user ID can cause no records to be returned without a MySQL error having occurred.
| 13. | Complete the PHP page.
} mysql_close(); include ('./includes/footer.html'); ?> The closing brace finishes the main submit conditional. Then the MySQL connection is closed and the footer is included.
| 14. | Save the file as delete_user.php and upload it to your Web server (it should be placed in the same directory as view_users.php).
| 15. | Run the page by first clicking a Delete link in the view_users.php page (Figures 8.4 and 8.5).
Figure 8.4. If you select Yes in the form (see Figure 8.3) and click Submit, this should be the result. 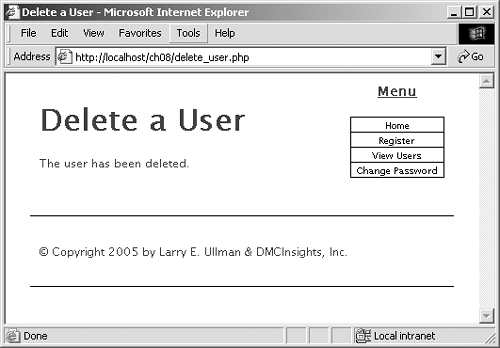 Figure 8.5. If you do not select Yes in the form, no database changes are made. 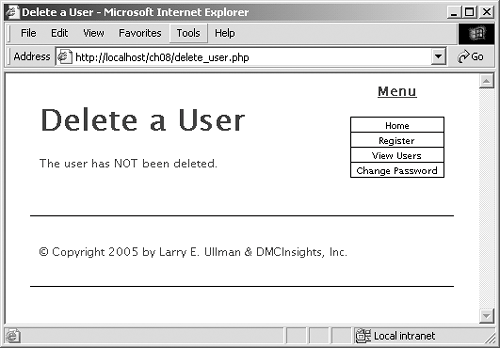
| Tips Another way of writing this script would be to have the form use the GET method. Then the validation conditional (lines 1019) would only have to validate $_GET['id'], as the ID would be passed in the URL whether the page was first being accessed or the form had been submitted. Hidden form elements don't display in the Web browser but are still present in the HTML source code (Figure 8.6). For this reason, never store anything there that must be kept truly secure. Figure 8.6. The user ID is stored as a hidden input so that it's available when the form is handled. 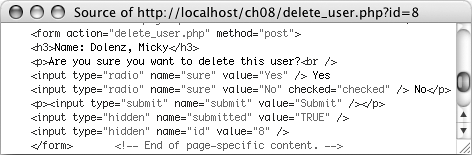
Using hidden form inputs and appending values to a URL are just two ways to make data available to other PHP pages. Two more methodscookies and sessionsare thoroughly covered in the next chapter. PHP's parse_url() function can be used to break a URL down into its subparts. See the PHP manual for more information.
|