The first step when dealing with MySQLconnecting to the serverrequires the appropriately named mysql_connect() function: $dbc = mysql_connect ($host, $user, $password); The arguments sent to the function (host, username, and password) are based upon the users and privileges set up within the mysql database (see Appendix A, "Installation," for more information). Commonly (but not always), the host to specify will be localhost (naming a different host will allow you to connect to MySQL running on a different server). If the connection was made, the $dbc variable will become a reference point for all of your subsequent database interactions. Most of the PHP functions for working with MySQL can take this as an optional argument, but if it is omitted, the functions will automatically use the open connection. Once you have connected to MySQL, you will need to select the database with which you want to work. This is the equivalent of saying USE databasename within the mysql client and is accomplished with the mysql_select_db() function: mysql_select_db($database_name); I'll start the demonstration of connecting to MySQL by creating a special file just for that purpose. Other PHP scripts that require a MySQL connection can include this file. I'll also make use of the mysql_error() function, which was briefly introduced at the end of the preceding chapter. To connect to and select a database 1. | Create a new PHP document in your text editor (Script 7.2).
<?php # Script 7.2 - mysql_connect.php Script 7.2. The mysql_connect.php script will be used by every other script in this application. It establishes a connection to MySQL and selects the database. 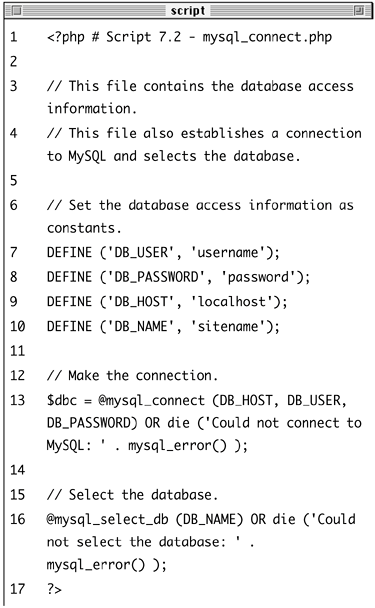
| 2. | Set the database host, username, password, and database name as constants.
DEFINE ('DB_USER', 'username'); DEFINE ('DB_PASSWORD', 'password'); DEFINE ('DB_HOST', 'localhost'); DEFINE ('DB_NAME', 'sitename'); I prefer to establish these variables as constants for security reasons (they cannot be changed this way), but that isn't required. In general, setting these values as some sort of variable makes sense so that you can separate the configuration parameters from the functions that use them, but again, this is not obligatory.
When writing your script, change these values to ones that will work with your database. Or do what I did and grant the proper permissions to a user called username with a password of password.
| 3. | Connect to MySQL.
$dbc = @mysql_connect (DB_HOST, DB_USER, DB_PASSWORD) OR die ('Could not connect to MySQL: ' . mysql_error() ); The mysql_connect() function, if it successfully connects to MySQL, will return a resource link that corresponds to the open connection. This link will be assigned to the $dbc variable (for database connection), which gives me the option of referring to the connection explicitly when using the other MySQL-specific functions.
The function call is preceded by the error suppression operator (@). This prevents the PHP error from being displayed in the Web browser (which is preferred, as the error will be handled by the OR die() clause).
If the mysql_connect() function cannot return a valid resource link, then the OR die() part of the statement is executed (because the first part of the OR will be false, so the second part must be true). As discussed in the preceding chapter, the die() function terminates the execution of the script. The function can also take as an argument a string that will be printed to the Web browser. In this case, the string is a combination of Could not connect to MySQL: and the specific MySQL error. Using this blunt error management system makes debugging much easier as you develop your sites.
| 4. | Select the database to be used and close the PHP page.
@mysql_select_db (DB_NAME) OR die ('Could not select the database: ' . mysql_error() ); ?> This final step tells MySQL on what database every query should be run. Failure to select the database will create problems in later scripts, although if an application uses multiple databases, you might not want to select one here.
Again, the OR die() construct is used to handle any MySQL problems, and the @ suppresses the original PHP error.
| 5. | Save the file as mysql_connect.php.
Since this file contains information that must be kept private (the database access data), I'll use a .php extension. With a .php extension, even if malicious users ran this script in their Web browser, they would not see the page's actual content.
| 6. | Upload the file to your server, outside of the Web document root (Figure 7.2).
Figure 7.2. A visual representation of a server's Web documents, where mysql_connect.php is not stored within the main directory (html). 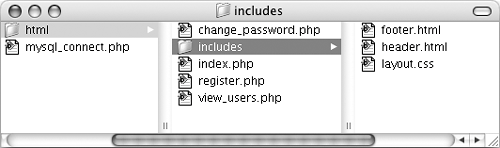
Because the file contains sensitive MySQL access information, it ought to be stored securely. If you can, place it in the directory immediately above or otherwise outside of the Web directory. This way the file will not be accessible from a Web browser.
| 7. | Temporarily place a copy of the script within the Web document root and run the script in your Web browser (Figures 7.3 and 7.4).
Figure 7.3. If the MySQL connection script works properly, the end result will be a blank page (no HTML is generated by the script). 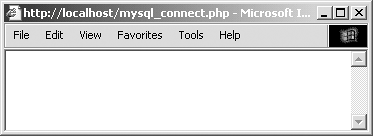
Figure 7.4. If there were problems connecting to MySQL, an informative message is displayed and the script is halted. 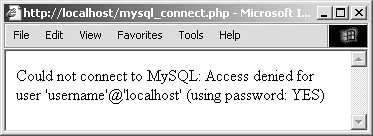
In order to test the script, you'll want to place a copy on the server so that it's accessible from the Web browser (which means it must be in the Web directory). If the script works properly, the result should be a blank page (see Figure 7.3). If you see an Access denied… or similar message (see Figure 7.4), it means that the combination of username, password, and host does not have permission to access the particular database.
| 8. | Remove the temporary copy from the Web directory.
| Tips The same values used in Chapter 4, "Introduction to SQL and MySQL," and Chapter 5, "Advanced SQL and MySQL," to log into the mysql client should work from your PHP scripts. If you receive an error that claims mysql_connect() is an undefined function, it means that PHP has not been compiled with MySQL support. See the first appendix for installation information. If you see an Access denied… error message when running the script (see Figure 7.4), use the mysql client to test your connection information. Also confirm that MySQL is running. Once you've written one mysql_connect.php file, you can easily make changes to the define() lines to use the script for other projects. In case you are curious, Figure 7.5 shows what would happen if you didn't use @ before mysql_connect() and an error occurred. Figure 7.5. If you don't use the error suppression operator (@), you'll see both the PHP error and the custom OR die() error. 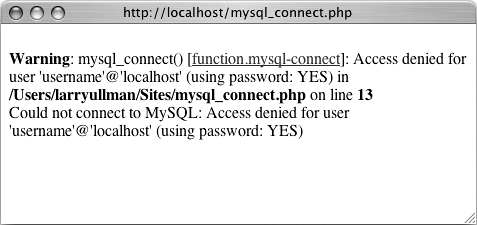
If you need to connect to multiple database servers in the same script, you can use multiple mysql_connect() calls, assigning the returned results to different PHP variables.
|