When it comes to debugging, what experience teaches you most is the causes of certain types of errors. Knowing this makes fixing the error much, much faster. To shorten the chase, Table 6.3 lists the likely reasons for the most common PHP errors. Table 6.3. These are some of the most common errors you'll see in PHP, along with their most probable causes.Common PHP Errors |
---|
ERROR | LLIKELY CAUSE |
---|
Blank Page | HTML problem, or PHP error and display_errors is off. | Parse error | Missing semicolon; unbalanced curly braces, parentheses, or quotation marks; or use of an unescaped quotation mark in a string. | Empty variable value | Forgot the initial $, misspelled or miscapitalized the variable name, inappropriate variable scope (with functions), or the register_globals setting is turned off. | Undefined variable | Reference made to a variable before it is given a value or an empty variable value (see those potential causes). | Call to undefined function | Misspelled function name, PHP is not configured to use that function (like a MySQL function), or document that contains the function definition is not properly included. | Cannot redeclare function | Two definitions of your own function exist, check within included files. | Headers already sent | White space exists in the script before the PHP tags, data has already been printed, or a file has been included. |
The first, and most common, type of error that you'll run across is syntactical and will prevent your scripts from executing. An error like this will result in messages like the one in Figure 6.13, which every PHP developer has seen too many times. To avoid making this sort of mistake when you program, be sure to: End every statement (but not language constructs like loops and conditionals) with a semicolon. Balance all quotation marks, parentheses, curly braces, and square brackets (each opening character must be closed). Be consistent with your quotation marks (single quotes can be closed only with single quotes and double quotes with double quotes). Escape, using the backslash, all single- and double-quotation marks within a print() statement, as appropriate. Figure 6.13. The parse error prevents a script from running because of invalid PHP syntax. This one was caused by failing to enclose $array['key'] within curly braces when printing its value. 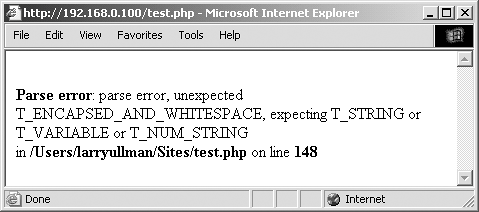
One thing you should also understand about syntactical errors is that just because the PHP error message says the error is occurring on line 12, that doesn't mean that the mistake is actually on that line. At the very least, it is not uncommon for there to be a difference between what PHP thinks is line 12 and what your text editor indicates is line 12. So while PHP's direction is useful in tracking down a problem, treat the line number referenced as more of a starting point than an absolute. If PHP reports an error on the last line of your document, this is almost always because a mismatched parenthesis, curly brace, or quotation mark was not caught until that moment. The second type of error you'll encounter results from misusing a function. This error occurs, for example, when a function is called without the proper arguments. This error is discovered by PHP when attempting to execute the code. In later chapters you'll probably see such errors when using the header() function, cookies, or sessions. To fix errors, you'll need to do a little detective work to see what mistakes were made and where. For starters, though, always thoroughly read and trust the error message PHP offers. Although the referenced line number may not always be correct, a PHP error is very descriptive. To debug your scripts Turn on display_errors. Use the earlier steps to enable display_errors for your entire server as you develop your applications. Use comments. Just as you can use comments to document your scripts, you can also use them to rule out problematic lines. If PHP is giving you an error on line 12, then commenting out that line should get rid of the error. If not, then you know the error is elsewhere. Just be careful that you don't introduce more errors by improperly commenting out only a portion of a code block: the syntax of your scripts must be maintained. Call the print() and echo() functions. In more complicated scripts, I frequently use print() statements to leave me notes as to what is happening as the script is executed (Figure 6.14). When a script has several steps, it may not be easy to know if the problem is occurring in step 2 or step 5. By using the print() statement, you can narrow the problem down to the specific juncture. Figure 6.14. More complex debugging can be accomplished by leaving yourself notes as to what the script is doing. 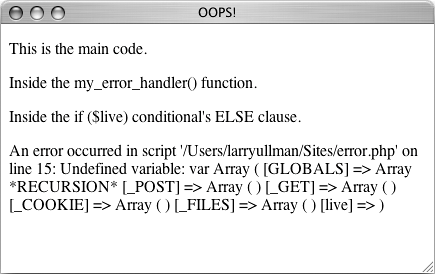
Check what quotation marks are being used for printing variables. It's not uncommon for programmers to mistakenly use single quotation marks and then wonder why their variables are not printed properly. Remember that single quotation marks treat text literally and that you must use double quotation marks to print out the values of variables. Track variables (Figure 6.15). Figure 6.15. Using the print() and echo() functions is the easiest way to track the values of variables over the course of a script. 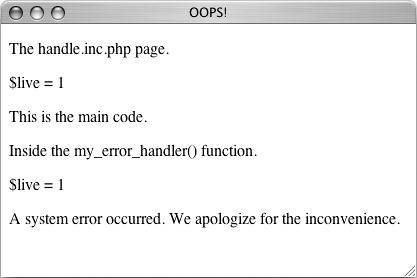
It is pretty easy for a script not to work because you referred to the wrong variable or the right variable by the wrong name or because the variable does not have the value you would expect. To check for these possibilities, use the print() or echo() statements to print out the values of variables at important points in your scripts. This is simply a matter of echo "\$var = $var <br />\n";
The first dollar sign is escaped so that the variable's name is printed. The second reference of the variable will print its value. Print array values. For more complicated variable types (arrays and objects), the print_r() and var_dump() functions will print out their values without the need for loops. Both functions accomplish the same task, although var_dump() is more detailed in its reporting than print_r(). Tips Many text editors include utilities to check for balanced parentheses, brackets, and quotation marks. If you cannot find the parse error in a complex script, begin by using the /* */ comments to render the entire PHP code inert. Then continue to uncomment sections at a time (by moving the opening or closing comment characters) and rerun the script until you deduce what lines are causing the error. Watch how you comment out control structures, though, as the curly braces must continue to be matched in order to avoid parse errors. For example: if (condition) { /* Start comment. Inert code. End comment. */ }
Some programmers advocate reverse testing to minimize the occurrence of logical errors. Reverse testing is the policy of switching your conditionals. For example, if ($variable = 5) {...
may not seem incorrect among 500 lines of code but still causes problems because that condition will always be trUE. Changing it to if (5 = $variable) {...
will immediately send up a red flag because the number 5 cannot be assigned a value. To make the results of either print_r() or var_dump() more readable in the Web browser, I will frequently wrap them within HTML <pre> (preformatted) tags: echo '<pre>'; print_r ($var); echo '</pre>';
|