To build dynamic Web sites with PHP, you must know how to send data to the Web browser. PHP has a number of built-in functions for this purpose, the most common being echo() and print(). Looking for an Escape As you might discover, one of the complications with sending data to the Web involves printing single and double quotation marks. Either of the following will cause errors (Figure 1.2): Figure 1.2. This may be the first of many parse errors you see as a PHP programmer (this one is caused by an un-escaped quotation mark). 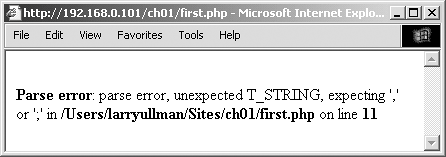
echo "She said, "How are you?""; print 'I'm just ducky.'; There are two solutions to this problem. First, use single quotation marks when printing a double quotation mark and vice versa: echo 'She said, "How are you?"'; print "I'm just ducky."; Or, you can escape the problematic character by preceding it with a backslash: echo "She said, \"How are you?\""; print 'I\'m just ducky.'; Understanding how to use the backslash to escape a character is an important concept, and one I'll cover in more depth at the end of the chapter. |
echo 'Hello, world!'; print "It's nice to see you."; As you can see from this example, either single or double quotation marks will work with either function (the distinction between the two types of quotation marks will be made clear by the chapter's end). Also note that in PHP all statements (a line of executed code, in layman's terms) must end with a semicolon. To send data to the Web browser 1. | Open first.php (refer to Script 1.2) in your text editor.
| 2. | Between the PHP tags (lines 10 and 11), add a simple message (Script 1.3).
echo 'This was generated using PHP!'; Script 1.3. Using print() or echo(), PHP can send data to the Web browser (see Figure 1.3). 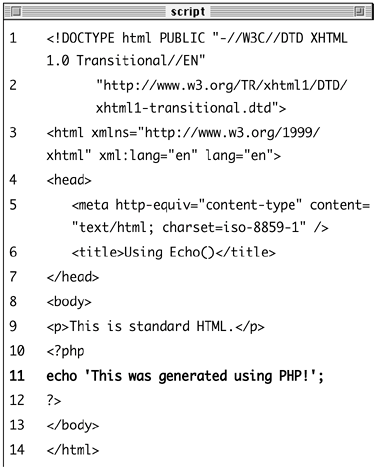
Figure 1.3. The results still aren't glamorous, but this page was in part dynamically generated by PHP. 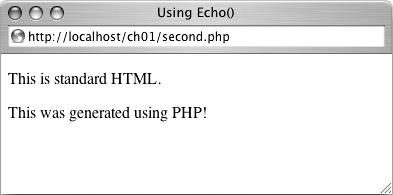
It truly doesn't matter what message you type here, which function you use (echo() or print()), or which quotation marks, for that matterjust be careful if you are printing a single or double quotation mark as part of your message (see the sidebar "Looking for an Escape").
| 3. | If you want, change the page title (line 6).
<title>Using Echo()</title> This is an optional and purely cosmetic change.
| 4. | Save the file as second.php, upload to your Web server, and test in your Web browser (Figure 1.3).
If you see a parse error instead of your message (see Figure 1.2), check that you have both opened and closed your quotation marks and escaped any problematic characters. Also be certain to conclude each statement with a semicolon.
If you see an entirely blank page, this is probably for one of two reasons:
There is a problem with your HTML. Test this by viewing the source of your page and looking for HTML problems there (Figure 1.4). If you don't know how to view the source, see the instructions at the end of the next set of steps. Figure 1.4. One common cause for seeing a blank PHP page is a simple HTML error, like the closing title tag here (it's missing the slash). 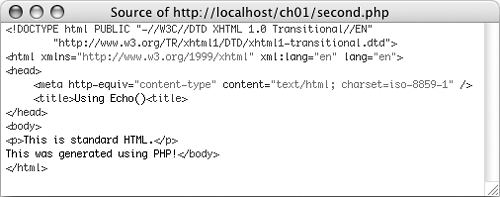
An error occurred, but display_errors is turned off in your PHP configuration, so nothing is shown. In this case, see the section in Appendix A on how to configure PHP so that you can turn display_errors back on.
| Tips Technically, echo() and print() are language constructs, not functions. That being said, don't be surprised as I continue to call them "functions" for convenience. Also, I include the parentheses when referring to functions to help distinguish them from variables and other parts of PHP. This is just my own little convention. You can also use echo() and print() to send HTML code to the Web browser, like so (Figure 1.5): echo '<b>Hello, <font size="+2"> world</font>!</b>';
Figure 1.5. PHP can send HTML code (like the formatting here) as well as simple text (see Figure 1.3) to the Web browser. 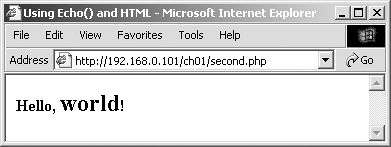
PHP is case-insensitive when it comes to function names, so ECHO(), echo(), eCHo(), and so forth will all work. With echo() but not print(), you can send multiple, separate chunks of data to the Web browser using commas: echo 'Hello, ', 'world!';
Echo() and print() can both be used to print text over multiple lines, as you'll see. I prefer to use echo() over print(), for no particular reason. If you prefer print(), you can replace any reference I make to echo() with print() without a problem.
|