Before I get into the heart of the Web application (the content management itself), I need to create two more pages. One will be index.php and will act as the home page for the site. The second will be mysql_connect.php, a script that connects to MySQL and selects the database to be used (see the "Database Scheme" sidebar in the next section for more information about the database). To make the home page 1. | Create a new PHP script in your text editor (Script 12.3).
<?php # Script 12.3 - index.php Script 12.3. The application's home page (add meaningful content as needed). 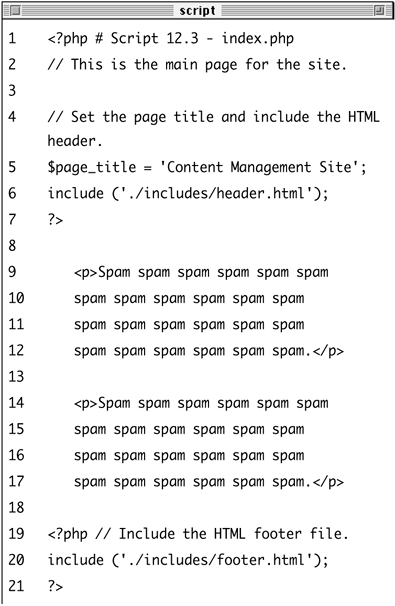
| 2. | Set the page title and include the HTML header.
$page_title = 'Content Management Site'; include ('./includes/header.html'); ?>
| 3. | Create the content.
<p>Spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam.</p> <p>Spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam spam.</p> Obviously, you'll want to put more useful content on your home page, but I'm using spam as filler (it's surprisingly filling).
| 4. | Require the HTML footer.
<?php include ('./includes/footer.html'); ?>
| 5. | Save the file as index.php, upload to your Web server (in the main html directory, see Figure 12.3), and test in a Web browser (Figure 12.4).
Figure 12.4. The home page. 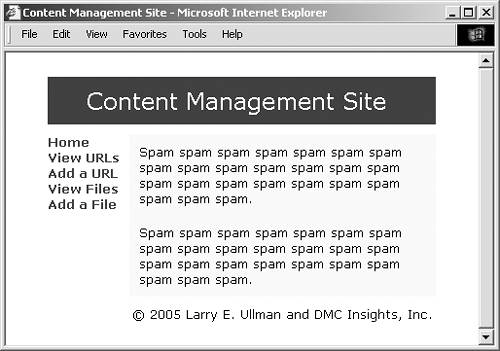
| More Content Management The content management example in this chapter deals with two specific kinds of content: files and lists of URLs. But content management systems (CMS) normally handle a third type of information: general Web content. Many CMS tools provide an interface to dynamically generate the text and/or images presented on specific pages. I have not included such an example in this chapter because it's really not that complicated and there's only so much space. Here's the quick lowdown: Having a Web page's content be dynamically driven is just a matter of storing text in a database table, somehow indicating what text belongs to what page on the public side. To place the text there in the first place, create a simple form with a large text area. Validate the text area upon submission and address HTML code and special characters, as warranted. The dynamic page can then pull the data out of the database and plop it into the template where appropriate. If you have multiple pages based upon the same template but using different valuessay several pages of book reviewspass the templated page the ID for the specific record in the database. This notionpassing an ID to a pagewill be demonstrated with the edit_url.php page. If you want to dynamically add images to a page, follow the same process as the files examples at the end of the chapter. Store the image's name in the database, store the file itself on the server, and dynamically create the HTML for the image on the public page. |
To make mysql_connect.php 1. | Create a new PHP script in your text editor (Script 12.4).
<?php # Script 12.4 - mysql_connect. php Script 12.4. This script connects to MySQL, selects the content database, and defines the escape_data() function, used for sanctifying submitted text. 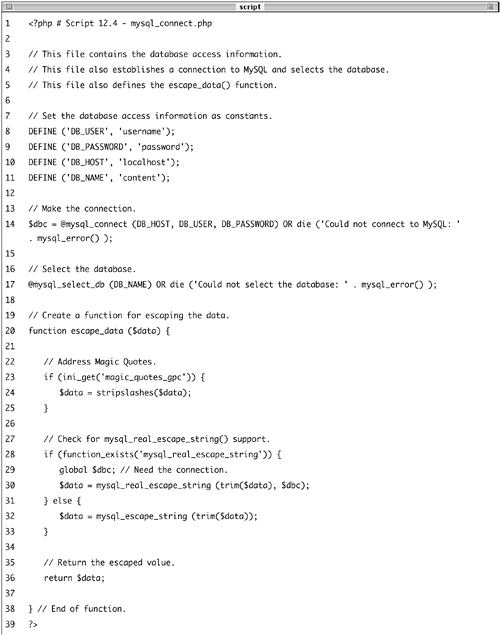 This script will be identical to previous versions of it from other chapters (except perhaps for the specific username, password, and database settings). You can either create this script from scratch or modify an existing version accordingly.
| 2. | Set the database information as constants.
DEFINE ('DB_USER', 'username'); DEFINE ('DB_PASSWORD', 'password'); DEFINE ('DB_HOST', 'localhost'); DEFINE ('DB_NAME', 'content'); For security's sake, the MySQL user for this application should have INSERT, SELECT, UPDATE, and DELETE privileges only, and just for the content database.
| 3. | Connect to MySQL and select the database.
$dbc = @mysql_connect (DB_HOST, DB_USER, DB_PASSWORD) OR die ('Could not connect to MySQL: ' . mysql_error() ); @mysql_select_db (DB_NAME) OR die ('Could not select the database: ' . mysql_error() ); This application will have basic error management, using the mysql_error() function (as discussed in Chapter 7, "Using PHP with MySQL"). Remember that you don't want to display MySQL errors to the general public, so you may want to use the more elaborate error handling techniques discussed in Chapter 6, "Error Handling and Debugging."
| 4. | Define the escape_data() function.
function escape_data ($data) { if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } if (function_exists ('mysql_real_escape_string)) { global $dbc; $data = mysql_real_escape_string (trim($data), $dbc); } else { $data = mysql_escape_string (trim($data)); } return $data; } This function will be used to handle submitted form values, so that inserting those values into the database will not break the queries. The function is defined as it was in Chapter 8, "Web Application Development," using several savvy techniques.
The function expects to receive one argument, assigned to $data. If Magic Quotes are onas determined using the ini_get() functionthen the stripslashes() function is applied to avoid over-escaping the data. Finally, a check is made to see if the mysql_real_escape_string() is available (it was added in PHP 4.3). If so, this function is used (and it requires a database connection, $dbc). If that function is unavailable, the older mysql_escape_string() function is called (it does not use a database connection). Finally, the well-groomed data is returned by the function.
| 5. | Complete the PHP page.
?>
| 6. | Save the file as mysql_connect.php and upload to your Web server (outside of the Web document root, see Figure 12.3).
| Tips The example in the next chapter will use more advanced error-management techniques and a modified version of this MySQL connection script. For more information on MySQL privileges and creating users, see Appendix A, "Installation."
|