PEAR, the PHP Extension and Application Repository, is a collection of established code that programmers can incorporate into their own projects. The official Web site for PEAR is http://pear.php.net (Figure 11.26). Figure 11.26. The home page for PEAR. 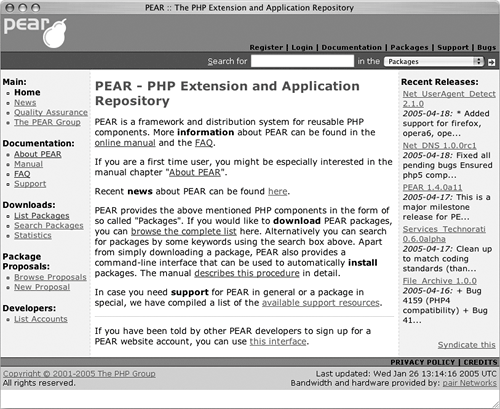 Many things I have done (or will do) in this book could be done using PEAR, including: Interacting with MySQL Controlling page caching Sending plain or HTML email Performing HTTP authentication Creating and validating HTML forms There are many advantages to using PEAR. First of all, you can more quickly develop applications. Second, your Web sites will then be relying upon tested, established code instead of your own (potentially buggy) work. There are really only two downsides to PEAR, in my opinion. For one, PEAR and the modules you'll want to use must be installed on a server (and the installation processsee the PEAR manualis not as foolproof as it could be). Second, not every PEAR module is as well documented or as smooth-running as it could be. Still, it's not difficult for the intermediate PHP programmer to use PEAR's classes, even without any formal training in object-oriented programming. As just one example of what you can do with PEAR, I'll add benchmarking to an existing script so that I can see how long various aspects of the script take to execute. In order to follow this example, you'll need to make sure that PEAR is installed, as well as the Benchmark class. To benchmark a script 1. | Open mysqli.php in your text editor or IDE.
I'm going to add benchmarking to the mysqli.php script, written earlier in the chapter. If you have not yet created this script, you can apply the concept demonstrated here to any other file.
| 2. | At the beginning of the script, create a new timer (Script 11.8).
<?php # Script 11.8 - benchmark.php require_once('Benchmark/Timer.php'); $t = new Benchmark_Timer; $t->start(); ?> Script 11.8. Using PEAR's Benchmark class, I time how long various aspects of this script take. 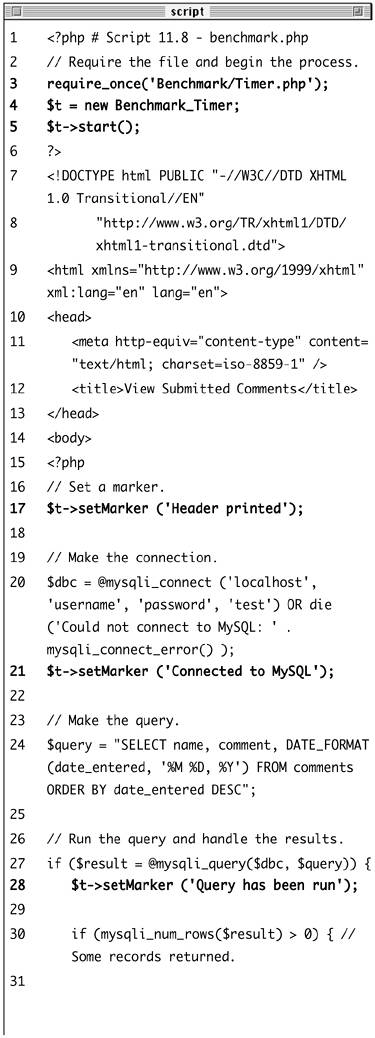 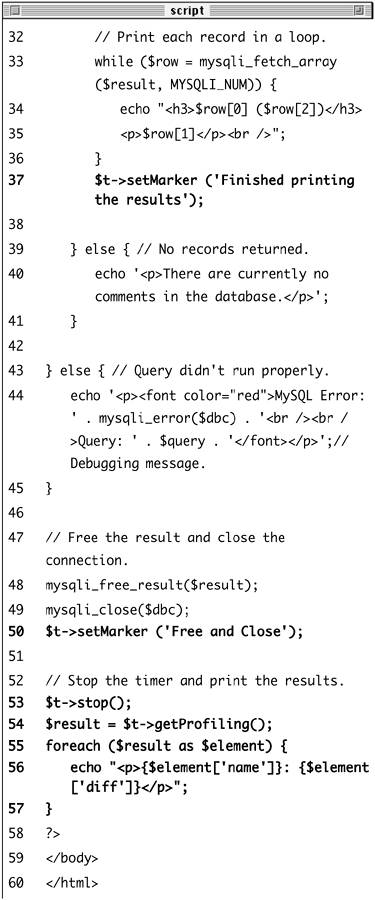
To use any PEAR class, you must first require the appropriate script. Then, following the example shown in the Timer.php script (which is part of the Benchmark package), I'll create a new timer object, called $t, and start the timer.
| 3. | After the initial HTML code, set a marker.
$t->setMarker ('Header printed'); First, I'll time how long it takes to print the header HTML. To do so, I set a marker using an appropriate marker name or caption with the setMarker() function (or method).
| 4. | After connecting to the database, set another marker.
$t->setMarker ('Connected to MySQL); Database connections are normally big resource hogs. To time how long this took, I create another marker.
| 5. | After querying the database, make another marker.
$t->setMarker ('Query has been run); This third marker will be used to determine how long it took to define and run a query.
| 6. | After printing all of the query results, set another marker.
$t->setMarker ('Finished printing the results);
| 7. | After closing the database connection, establish the final marker.
$t->setMarker ('Free and Close');
| 8. | Finally, stop the timer.
$t->stop(); The stop() method of the timer will end the benchmarking process.
| 9. | Print out the benchmark results.
$result = $t->getProfiling(); foreach ($result as $element) { echo "<p>{$element['name']}: {$element['diff]}</p>"; } Once you've stopped the timer, a call to the getProfiling() function will return a multidimensional array with the timing results. The easiest way to print out $result is to use a foreach loop. Within the loop, each element of the $result array is itself an array of four elements: name (the marker name), time (the time the marker was set), diff (the difference between this marker and the previous marker or start), and total (the total time elapsed since the timer was started). Using this information, I'll print out the name of each marker and the amount of time each marker took.
| 10. | Save the file as benchmark.php, upload to your Web server, and test in your Web browser (Figure 11.27).
Figure 11.27. At the bottom of the page, the result of using the Benchmark class helps reveal how long different steps of the script took to execute. 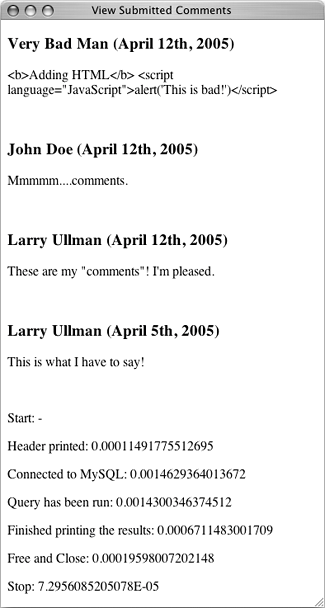
Looking at the resulting numbers in the figure, you can see that connecting to MySQL and executing the query are the most involving steps, taking ten times as long as any other step.
| Tips Some PEAR packages will not work on the Windows operating system because they involve Unix-specific functions. To see the currently available list of packages, type pear list-remote-packages in a command prompt, assuming the PEAR package manager has been installed. There is a subset of PEAR called PECL ("pickle," the PHP Extension Code Library) that consists of standard PEAR modules written directly in C and C++, rather than PHP, resulting in better performance. An important aspect of PEAR is the set of standards under which all PHP modules must be written (in terms of variable names, document structure, and so forth). Even if you will never attempt to add to the PEAR library, following these standards as a PHP programmer is well worth considering. The PEAR online documentation lists PEAR-specific Web sites, mailing lists, and other places to turn for support. If you are really interested in fine-tuning the performance of your Web applications, use the Benchmark class to time your scripts, and then focus on those parts that take the longest to execute (for example, the database query in this script). You can also use this class to see the speed difference between, say, using mysql_* versus mysqli_* functions, or to see the benefit of prepared statements. PEAR's database abstraction class is one of its most popular tools, allowing you to develop database-independent Web applications.
A Brief Introduction to Objects and Classes There is one last variable type that I have not discussed in this book: objects. Object-oriented programming (OOP) is a subject so complex that multiple chapters and even entire books are dedicated to the subject. PHP has supported objects for some time now, and version 5 of the language has taken that support to new heights. I have omitted discussion of objects for two reasons. First, a reasonably thorough coverage of them would have meant less room for what I consider to be far more important information. And second, you can go your entire PHP programming life without ever using objects. So that the subject isn't entirely foreign to you, here's a three-second introduction: One uses objects to encapsulate related blocks of code into one easy-to-use, easy-to-maintain object. You start by creating a class. A class is a definition of a thing, normally containing both functions (called methods) and variables (called properties). Once you've defined a class, you can create an instance of that class, which is a variable of an object type. $var = new ObjectName; To then call an object's method (a function), the syntax is $var->functionName(); If you ever see this syntax, as with the PEAR example in this chapter, you'll now understand its meaning. More advanced objects involve special functionscalled constructorsthat are called when a new instance is created and those that are invoked when an instance is deletedcalled destructors. There is also inheritance, where you create a new class by expanding upon an existing class definition. While OOP is quite powerful, it's not for faint of heart, nor should you start trying to write your own objects without a thorough understanding of the concept. And as I said, you can continue to program in PHP without ever using objects, without limiting your potential at all. Also, even the relatively novice programmer can use existing objectslike the PEAR body of codeby merely following the example syntax. |
|