In this task, you will build a custom ActionScript class that defines a grocery product. This class enables you to specify each property of the object as a typed property such as String or Number, which will result in better performance and error handling. All of your data for a single grocery item will be encapsulated into one ActionScript object. Throughout the FlexGrocer application, you will need to manage large amounts of typed data and send this data to other applications. When you have a recurring problem in application design, developing a high-quality solution to this problem is known as developing a design pattern. In this task, you will use a well-known design pattern, the value object pattern, to solve this problem. 1. | Create a new ActionScript class file by choosing File > New > ActionScript class. Set the Package to valueObjects, which will cause Flex Builder to automatically create a folder with the same name. Name the class Product and leave all other fields with the defaults. Click Finish to create the file.
This process creates a file for you with the basic structure of an ActionScript class. The word package and class are both keywords used in defining this class. Remember this class will be a blueprint for many objects that you will use later to describe each grocery product.
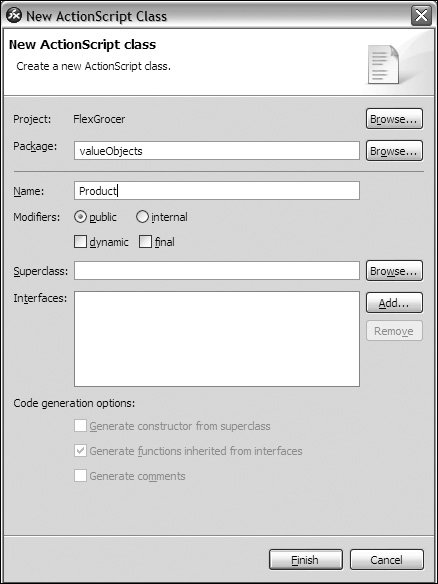 | | | 2. | Before the class keyword, but after the package keyword, add a [Bindable] metadata tag. Inside of the Product class definition, add a public property with the name of catID and a data type of Number.
package valueObjects { [Bindable] public class Product { public var catID:Number; } } The [Bindable] metadata tag, when specified in front of the class keyword, means that every property in the class can be bound to controls or other data structures. Metadata is a term used to refer to data that describes other data. In this case, you are using metadata to describe an entire class. When a property is specified as [Bindable], Flex framework will automatically copy the value of the source property to any destination property when the source property changes. Instead of specifying the whole class as [Bindable], you can specify individual properties. In the case of the FlexGrocer application, we want every property to be [Bindable].
| 3. | Create public properties with the names of prodName (String), unitID (Number), cost (Number), listPrice (Number), description (String), isOrganic (Boolean), isLowFat (Boolean), and imageName (String). Your class should look as follows:
package valueObjects { [Bindable] public class Product { public var catID:Number; public var prodName:String; public var unitID:Number; public var cost:Number; public var listPrice:Number; public var description:String; public var isOrganic:Boolean; public var isLowFat:Boolean; public var imageName:String; } } You are creating a data structure to store inventory information for the grocery store. In the top portion of the class, the structure includes all the properties that will be used in the class. This provides a nice separation between the data in the class and the visual UI components, which will display the data.
| | | 4. | Within the braces for the Product class, after the imageName property, define the constructor function of the class and specify the parameters that will be passed to this function. The parameters should match the data type of the properties you already defined, and the names should match but begin with an underscore in order to avoid name collision (when the same name could refer to two separate variables) between parameters and properties. Be sure that the name of the function matches the class name and that the constructor is public.
[View full width] public function Product (_catID:Number, _prodName:String, _unitID:Number, _cost:Number, _listPrice:Number, _description:String,_isOrganic:Boolean, _isLowFat:Boolean, _imageName :String){ }
The constructor function is automatically called every time an object is created from a class. You can create an object from a class by using the new keyword and passing the class parameters. You will eventually pass the new object elements from your <mx:Model> tag or database, which will result in more-organized data.
| 5. | Inside the constructor function, set each property to the value passed to the constructor function.
[View full width] public function Product (_catID:Number, _prodName:String, _unitID:Number, _cost:Number, _listPrice:Number, _description:String, _isOrganic:Boolean, _isLowFat:Boolean, _imageName :String){ catID = _catID; prodName = _prodName; unitID = _unitID; cost = _cost; listPrice = _listPrice; description = _description; isOrganic = _isOrganic; isLowFat = _isLowFat; imageName = _imageName; }
This code will set each property to the value passed when the object is first instantiated using the new keyword.
| | | 6. | Create a new method directly below the constructor function with the name of toString(), which will return the string [Product] and the name of the product.
public function toString():String{ return "[Product]"+this.prodName; } This method will return the name of the current product and will be handy for retrieving the name when you need it. Building methods to access properties is good practice because if the property name ever changes, you still call the same function, potentially saving much legacy code. The toString() method is automatically invoked by Flex framework any time an object is traced. This can be very useful for debugging and displaying data structures.
| 7. | Return to the EComm.mxml file and locate the script block at the top of the page. Import the Product class from the valueObjects folder.
import valueObjects.Product; To use a custom class, Flex Builder needs an import statement that references the location or package in which the class is located. Flex framework comes with many default classes. However, when you write a custom class, you need to explicitly import a class so it is available to Flex framework. You do this by using the import keyword.
| 8. | Before the prodHandler() function, in the script block, declare the theProduct object as a private property. Add a [Bindable] metadata tag.
[Bindable] private var theProduct:Product; All MXML files ultimately compile to an ActionScript class. You must follow the same conventions when creating an MXML file as when creating an ActionScript class. For example, you must import any classes that are not native to the ActionScript language, such as the Product class you have built, and you must declare any properties that you will use in your MXML file. This is done in a script block. The [Bindable] metadata tag will ensure that the property can be connected to visual controls.
| 9. | Within the prodHandler() function, create a new instance of the Product class with the name of theProduct, and populate and pass the parameter to the constructor function with the information from the <mx:Model> tag, as follows:
[View full width] theProduct = new Product(theItems.catID, theItems.prodName, theItems.unitID, theItems.cost , theItems.listPrice, theItems.description, theItems.isOrganic, theItems.isLowFat, theItems .imageName);
This code will place the data from the <mx:Model> tag into a Product value object, allowing for information to be better organized and more accessible. In addition, all of the property values will have datatypes which will result in a faster performing application and more detailed errors.
| | | 10. | Delete the two TRace statements from the prodHandler() function and add a new trace statement that will automatically retrieve the information you specified in the toString() method.
trace(theProduct); | 11. | Save and debug the MXML file.
You should see [Product]Milk in the console window, which indicates that you are using a Product value object to store the data.
| |