Visual Basic .NET includes two controls that allow your programs to accept date (and possibly time) information as input. These two controls are discussed in this section. The DateTimePicker Control When you want to allow your users to enter date and time information directly, the DateTimePicker control is a flexible and user-friendly entry tool. Its basic interface allows the user to enter a date in one of two predefined formats, a time in a predefined format, or a date and/or time in a customizable format. Depending on how you set its properties (see Table 12.1), the DateTimePicker offers the user either a dynamic drop-down calendar from which to select a date or a formatted text box in which he can manually enter a date or time, with spinners (small up and down arrows) to assist him. A typical DateTimePicker control is illustrated in Figure 12.7. Figure 12.7. The DateTimePicker control makes it easy for the user to enter date information. 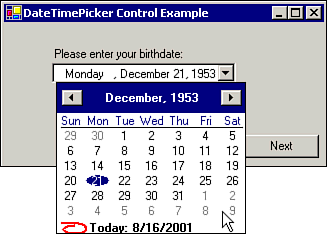 Tip When the user is selecting a date from the calendar-like interface, if he clicks the year at the top of the control, he will be given up and down buttons that he can use to quickly move the calendar a year at a time. In addition, clicking the month name causes a list of all 12 months to appear; he can click the name of a month to go quickly to that month. Once the user has selected a date or time in a DateTimePicker control, that information is available to your program via the control's Value property, which returns a DateTime value. You can then use one of several methods of the DateTime structure to retrieve the information in the control in a String format that you can use. For example, the ToShortDateString method would retrieve the date contained in the DateTimePicker control and return it as a short-formatted date, as follows: lblBirthDate = DateTimePicker1.Value.ToShortDateString Table 12.1 shows examples of some of the formats in which you can retrieve the date and time information contained in the Value property of a DateTimePicker control by using various methods of the DateTime structure. Table 12.1. Methods for Retrieving Data from the DateTimePicker Control Method | Type | Example |
---|
ToShortDate | Date | 02/18/2002 | ToLongDate | Date | Monday, February 18, 2002 | ToShortTime | Time | 23:55 | ToLongTime | Time | 23:55:37 | The DateTimePicker control is customized using the properties described in Table 12.2. Table 12.2. Common DateTimePicker Control Properties Property | Description |
---|
Format | Specifies the format of the information being requested from the user. The default value is Long, which accepts and displays dates in the format "day-of-week, month, day, year;" for example, Monday, November 12, 2001. Another possible value for this property is Short, which displays a shorter date format (11/12/2001). Setting the property to Time accepts time information (10:42:35 PM). The fourth value, Custom, is used in conjunction with the CustomFormat property to utilize a special format. | CustomFormat | This property is set to a string value to specify how a customized date or time format is to be displayed and accepted in the control. For example, a CustomFormat of dddd M/d/yyyy HH:mm would display the day of the week, the month/day/year, and the time in 24-hour format (Friday, April 27, 2001 23:11). | ShowUpDown | When set to False (the default), the control will display a drop-down arrow that the user can click to view a navigable pop-up calendar. When he clicks a date in the calendar, that date is used to populate the date in the control. Setting this property to True replaces the drop-down arrow with up and down spinners. He can then select any of the date or time fields (year, for example) and use the spinners to automatically move the selected portion of the date or time up or down. | MaxDate | The latest possible date that may be entered or selected in the control. The default value is 12/31/9998. | MinDate | The earliest possible date that may be entered or selected in the control. The default value is 1/1/1753. | CalendarFont | The font used to display the pop-up calendar. The CalendarFont property supports the subproperties Name, Size, Unit, Bold, Italic, Strikeout, and Underline. | Value | The Value property contains the date and time currently displayed in the control. You can set the Value property using code like DateTimePicker1.Value = #11/12/1962.htm# (note that literal dates are enclosed in pound signs instead of quotes). You can retrieve the Value property in a similar manner (lblBirthdate.Text = DateTimePicker1. Value.ToString). | Note See the Help system topic for the CustomFormat property for a complete list of acceptable characters and their meanings. The MonthCalendar Control When you need to let your application's users select a date, the MonthCalendar control (shown in Figure 12.8) provides an easily navigable, graphical calendar to make this task easy. Unlike the DateTimePicker control, the MonthCalendar control does not have an input area into which the user can type a date; its date entry and display is done entirely through the calendar-like interface. Also, while the DateTimePicker control allows your user to specify a single date, the MonthCalendar control supports the selection of a series of dates. Figure 12.8. The MonthCalendar control gives your program's users an easy way to select a range of dates. 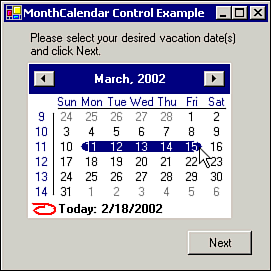 The MonthCalendar control's SelectionRange property contains a SelectionRange class value, representing the starting and ending dates that are currently selected in the control's interface. You can access the Start and End subproperties of the SelectionRange property to determine the date range that the user has specified in the control. The following sample code will populate two Label controls, lblStartDate and lblEndDate, with the beginning and ending dates specified in a MonthCalendar control (by using the ToString method of the Start and End subproperties of the SelectionRange property): lblStartDate.Text = MonthCalendar1.SelectionRange.Start.ToString lblEndDate.Text = MonthCalendar1.SelectionRange.End.ToString You can use the properties described in Table 12.3 to customize and utilize the MonthCalendar control. Table 12.3. Common MonthCalendar Control Properties Property | Description |
---|
AnnuallyBoldedDates | An array of DateTime values that are to be shown in boldface type on the calendar each year. | BoldedDates | An array of nonrecurring DateTime values that are to be shown in boldface type on the calendar. | CalendarDimensions | Sets the number of months to be displayed in a MonthCalendar control at one time. The CalendarDimensions property is set to a pair of values representing the width and height of the "grid" of calendar months. CalendarDimensions.Width and CalendarDimensions.Height are directly accessible as subproperties as well. The default CalendarDimensions setting is 1, 1, which displays a single month. | FirstDayOfWeek | Specifies the first day to be displayed in each week of a calendar. | MaxDate | Specifies the latest date that the calendar will accept. The default value is 12/31/9998. | MinDate | Specifies the earliest date that the calendar will accept. The default value is 1/1/1753. | MonthlyBoldedDates | An array of DateTime values that are to be shown in boldface type on the calendar each month. | ScrollChange | The number of months that the calendar will be moved when the user clicks one of the arrow controls that are used to navigate the calendar. The default value is the number of months displayed (as determined by the CalendarDimensions property); the maximum value is 20000. | SelectionStart | The beginning date of the selected range of dates. | SelectionEnd | The ending date of the selected range of dates. | SelectionRange | The entire range of selected dates (see the previous text). | ShowToday | When set toTrue (the default), the current date (as specified by the TodayDate property) is displayed at the bottom of the calendar. | ShowTodayCircle | When set to True (the default), the current date (as specified by the TodayDate property) is circled in the appropriate month of the calendar. | ShowWeekNumbers | When set toTrue, the week number of each week is displayed along its left side. The default value is False. | TitleBackColor | Specifies the color to be used as the background of the control's title area, which displays month and year information. | TitleForeColor | Specifies the color to be used for the text in the control's title area, which displays month and year information. | TodayDate | Specifies the date that the calendar displays as the current date. The default value is the current system date. | |