One of the most important aspects in designing any program is providing the users with easy access to all the program's functions. Users are accustomed to accessing most functions with a mouse click or two. In addition, they want all the functions located conveniently in one place. To help facilitate this strategy, most commercial Windows programs provide a menu bar across the top of the screen. Although today's users may be more accustomed to using hyperlinks, a menu system is still a convenient way to provide access to your program's functions, especially if screen space is limited. In this section we will show you how to create a menu bar on a form. We will also describe how to create a context menu, which is a menu that pops up when the user clicks a form or control. Getting Started The first step in creating a menu is determining what program commands need to be on the menu and how these commands should be organized. For example, look at Visual Basic's own main menu. As you can see, the commands are organized into functional groups (File, Edit, View, and so forth). When you create your program's menu, you should group similar items. In fact, if possible, you should use groups with which your users are already familiar. This way, users have some idea where to find particular menu items, even if they've never used your program. The following list describes some standard menus you will find on many menu bars: File This menu contains any functions related to files used by your program. Some of the typical menu items are New, Open, Close, Save, Save As, and Print. If your program works extensively with different files, you also might want to include a quick access list of the most recently used files. If you include a File menu, the program's Exit command is usually located near the bottom of this menu. Edit The Edit menu contains the functions related to editing of text and using the Windows Clipboard. Some typical Edit menu items are Undo, Cut, Copy, Paste, Clear, Find, and Replace. View The View menu might be included if your program supports different looks for the same document. A word processor, for example, might include a normal view for editing text and a page-layout view for positioning document elements, as well as a variety of zoom options. Another use of the View menu is to allow the users to display special forms in your program, such as the Visual Basic View, Toolbox option. Tools This menu is a catchall for your program's utilities or helper functions. For example, a spelling checker, grammar checker, or other less-frequently used options. Window This menu typically is included if your program uses a Multiple Document Interface (MDI), which will be discussed in a later chapter. MDI programs, like Microsoft Word, support the simultaneous editing of different documents, databases, or files. The Window menu lets users arrange open documents or switch rapidly between them. Help The Help menu contains access to your program's Help system. Typically, it includes menu items for a Help Index (a table of contents for help), a Search option (to let the users quickly find a particular topic), and an About option (providing summary, authoring, and copyright information regarding your program). You can use these six standard menus as a basis for creating your own menu system. The items in the previous list are examples of top-level menu items, because they are at the top of the menu hierarchy, always visible on the screen, and very general in nature. Beneath the top-level are submenu items, which represent more specific menu choices. For example, the File menu item does not do anything by itself, but the items beneath it all perform specific file-related functions. Note Occasionally, the text of a menu item ends with an ellipsis ( . . . ). The ellipsis is an accepted menu standard that indicates more information is required to execute the command. One example is Visual Basic's own Print menu item, which displays a dialog box for the user to enter additional information. Note The top-level/submenu organization is just an accepted convention; from the programming point of view each item in the menu is treated the same. Although it would seem odd to a user, there is nothing to prevent you from initiating program actions from a top-level item. Using the Menu Editor The .NET framework provides menu functionality via classes, and like any other classes they can be created entirely in code by instantiating objects. However, it is much easier to add menus using the Menu Editor provided by Visual Studio, which generates the code for you. To work with the Menu Editor, you place one of the following controls on the form: MainMenu This control is typically used to create a menu that will appear at the top of your form. You can add more than one MainMenu control to a form and switch between them programmatically. ContextMenu This is used to provide pop-up functionality. It can be tied to a control or form via the ContextMenu property, as we will see in a moment. When you drag either type of menu control to your form, it will appear in the bottom area of the form for invisible controls, as shown in Figure 10.8. To edit a menu, you select it by single-clicking its icon, then the Menu Editor will appear beneath the form's title bar. Figure 10.8. Starting with Visual Studio .NET, the Menu Editor is integrated into the form and no longer appears as a separate dialog box. 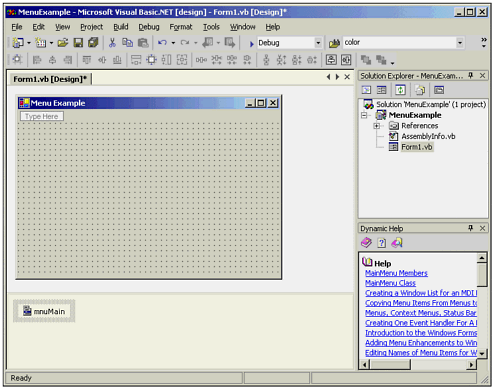 Creating the First Menu Item A menu is a collection of menu items. All the menu item objects are basically treated the same, although their appearance and organization can be altered. Each menu item contains text (usually only a word or two), which the user can click with the mouse to select an option. To see how the Menu Editor works, we will walk through creating a simple menu. Perform the following steps to create a sample menu. -
Start a new Windows Application project. -
Display the toolbox and drag a MainMenu control to the form. -
Single-click the ManuMenu1 control. Using the Properties window, change the name of the control to mnuMain. -
When you select the menu, notice the words Type Here appear at the top of the form, as shown in Figure 10.8. This is the location of the first menu item. -
Type File in the Type Here box and press Enter. You have just created a new menu item. As you type the text for each menu item, you will notice that additional Type Here boxes appear, as shown in Figure 10.9. As you might imagine, typing in one of those boxes will create an additional menu item. Figure 10.9. Type below the current menu item to continue adding items to the same menu list; to create a submenu, type in the box to the right of the current item. 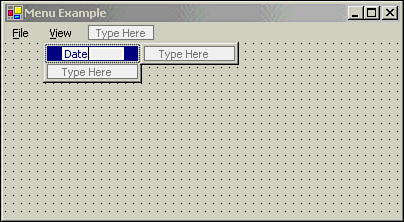 Adding Additional Menu Items By creating menu items in the appropriate Type Here box, you can create new top-level menus or submenus. Notice that a black arrow indicates a menu item that contains a submenu. Note You can use Tab, Enter, down arrow to quickly enter several menu items without lifting the mouse. You can add new menu items from left-to-right, top to bottom, very quickly. However, if you need to go back and insert a new menu item before an existing one, just right-click the menu item and choose Insert. If you accidentally add an empty menu item or need to delete an extra menu item, right-click the unwanted item and choose delete. After you have keyed in your menu items, you can immediately see how the menu will look in Design mode by using the mouse to navigate it. To continue creating our sample menu, perform the following steps. -
Beneath the File menu, create new menu items for Open and Exit. -
Create an additional top-level menu item, View. -
Beneath the View menu item create two submenu items, Date and Time. Adding Code to Respond to Menu Events As you can see, it is very easy to add items to a menu. If you run the sample program, you will see the menu bar displayed in your form. However, just designing the menu is not enough to make it useful. You also have to write VB code and associate it with the menu items, usually in the Click event. Setting Menu Item Names Each item in your menu is like a control, and as you know, controls have properties. When you created the sample menu, you were actually setting the values of the menu items' Text properties. The Text property determines what text is displayed in the menu. One of the most important of these properties is the Name property, which you use to identify the menu item in your code. You also can enter these using the Menu Editor. To learn how to do this, right-click any of the sample menu items you just created and choose Edit Names from the menu. You should see the menu names displayed in the Menu Editor, as shown in Figure 10.10. Figure 10.10. You can edit both the control name or display text of a menu item using the Menu Editor. 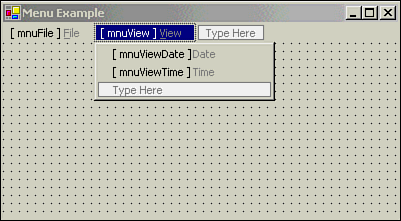 I typically use a hierarchical structure to assign the Name property of my menu items. Each menu item's name begins with the prefix mnu, followed by words that describe the portion of the menu in which the item is located. For example, mnuFileSendToFax is used to represent the menu item with the caption Fax Recipient; I can tell from the name that this item is in the Send To submenu of the File menu. As with any object you create in VB .NET, providing a readable variable name that follows a known naming convention will make it easier to understand your code. For more on naming conventions, p.139 Note You can display the Properties window of a menu item just like any other control, and use it to set both the Name and Text properties. Following along with our sample application, use the Menu Editor to rename the menu items as follows: Text | Name |
---|
File | mnuFile | Open | mnuFileOpen | Exit | mnuFileExit | View | mnuView | Date | mnuViewDate | Time | mnuViewTime | Coding the Click Event Menu items generally work like button controls in that you click them and something happens. To demonstrate, perform the following modifications to the sample program: -
From the form designer, double-click the Open menu item. The Code Editor will be opened to the Click event for mnuFileOpen. Enter the following lines of code: Dim dlgFile As New OpenFileDialog() dlgFile.ShowDialog() -
Enter the following line of code in the Click event for mnuFileExit: Application.Exit() Now press F5 to run the sample program. Click the Open menu item and a File Open dialog box will appear. Click the Exit option and the program will end. Changing Menu Behavior and Appearance You now know how to create a standard menu item that acts like a button control. However, there are times when you may need a menu item that is used to turn an option on or off rather than initiating an action. Also, you may want to separate menu items so they appear more organized to the user. To get an idea of the many different ways you can customize your menu, look at Figure 10.11. Figure 10.11. Menu items can be customized in several different ways. 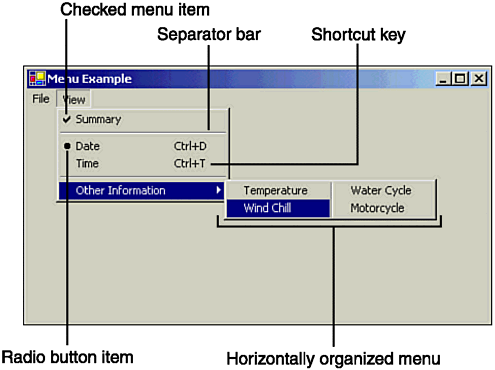 Adding Check Marks By default, menu items are created to initiate an action. However, some menu items can be used to indicate the status of an option or select from a group of options. Check marks can be used with menu items to indicate selected options and are controlled by two key properties: Checked If this property is set to True, a check mark is displayed to the left of the menu item. Generally the Checked property is controlled with VB code, although you also can use the Properties window or by clicking in the margin when using the Menu Editor. RadioCheck Determines whether the check marks appear as a check or a round radio button. Note Check marks are not available on items that contain submenus. Keep in mind that these properties only control visual aspects of a menu item; the events and function of the menu item is no different than any other. In our sample program, perform the following steps to make the View menu work: -
Add a text box to the form. Set its Name property to txtInfo. -
Place the following lines of code in the Click event of mnuViewDate: If mnuViewDate().Checked <> True Then mnuViewDate().Checked = True mnuViewTime().Checked = False txtInfo().Text = Format(Date.Now, "MM/dd/yyyy") End If -
Place the following lines of code in the Click event of mnuViewTime: If mnuViewTime().Checked <> True Then mnuViewTime().Checked = True mnuViewDate().Checked = False txtInfo().Text = Format(Date.Now, "hh:mm:ss") End If Run the program and try clicking on the menu items in the View menu. The code in the Click event not only updates the text box with the appropriate information, but also displays a check mark beside the appropriate item. Note If you want to use a check mark to indicate an on/off setting, you can use the Not operator, as in the following example: mnuShowDetail.Checked = Not(mnuShowDetail.Checked) The line of code will toggle the check mark each time the menu item is selected. You can then use an If statement to take appropriate action based upon the value of the Checked property. Using Keystrokes in a Menu Using keystroke combinations instead of a mouse is a quicker way to access program functions that is available in many Windows programs. You can let users access your menu items in the same way. Two types of key combinations can be used: access keys and shortcut keys. If a menu item has an access key defined, the access key is indicated by an underscore beneath the letter in the item's caption (for example, the x in Exit). You create an access key by placing an ampersand (&) in front of the appropriate letter in the Text property of a menu item. (To display an & character in your menu, simply type two ampersands.) For the File menu, the Text property would be &File. To use a top-level access key, press Alt and the access key. For example, in Visual Basic press Alt+F to open the File menu, then release the Alt key and press the letter x to exit the program. Note Visual Basic provides automatic access keys for top-level menu items such as File, View, and so on, using the first letter of the menu. You can override the default by defining another access key. The access key indicators for top-level menus are not displayed until you press the Alt key. (Pressing and releasing the Alt key by itself will activate the menu bar and allow you to use the arrow keys to move from item to item.) Another way to use the keyboard to access the menu is to add a shortcut key. A shortcut key can be used to instantly execute the Click event of a menu item without any menu navigation. To create a shortcut key, display the Properties window for a menu item and assign a value to the Shortcut property, shown in Figure 10.12. Figure 10.12. Shortcut keys allow you to quickly execute menu commands. 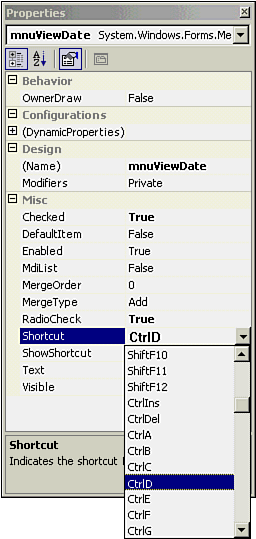 The ShowShortcut property determines whether the menu displays the shortcut key, so the user can learn it. If this is set to True, the shortcut appears to the right of the menu item text. Organizing Your Menu As you saw earlier in this section, you can create new menu items to the right of a submenu item. This multi-level approach to organizing your menu allows you to group related items. In addition to using menu levels to organize the items in your menu, you might want to further separate items in a particular level. Placing separator bars in the menu breaks up a long list of items and further groups the items, without your having to create a separate level. To place a separator bar in your menu, right-click a menu item in the Menu Editor and choose Insert Separator. A horizontal line will be displayed before the current menu item. As an example, the Exit option is usually separated from the other options in your File menu. Another way of organizing your menu is to use the BarBreak property to create a new column. If your submenu has a lot of items, setting the BarBreak property of a menu item to True will cause the item and subsequent items to be placed in separate columns, as shown in Figure 10.11. Enabling and Disabling Menus A menu item'sVisible and Enabled properties work just like they do with any other object. When the Visible property is set to True, the menu item is visible to the users. If the Visible property is set to False, the item (and any associated submenus) are hidden from the users. You have probably seen the Enabled and Visible properties used in a word processing program (though you might not have been aware of how it was accomplished), where only the File and Help menus are visible until a document is selected for editing. After a document is open, the other menu items are shown. Changing the setting of the Visible property allows you to control what menu items are available to the users at a given point in your program. Controlling the menu this way lets you restrict the users' access to menu items that might cause errors if certain conditions are not met. (You wouldn't want the users to access edit functions if no document was open to edit, right?) The Enabled property serves a function similar to that of the Visible property. The key difference is that when the Enabled property is set to False, the menu item is grayed out. This means that the menu item still can be seen by the users but cannot be accessed. For example, the standard Edit, Cut and Edit, Copy functions should not be available if no text or object is selected, but nothing is wrong with letting the users see that these functions exist. Using Context Menus So far, the discussion of menus has looked at the menu bar that appears along the top of the form. Visual Basic also supports pop-up menus in your programs. A pop-up menu, also known as a context menu, is a small menu that appears somewhere on your form in response to a program event. Pop-up menus often are used to handle operations or options related to a specific area of the form (see Figure 10.13) for example, a format pop-up menu for a text field that lets you change the font or font attributes of the field. You can find such context-sensitive in Visual Basic itself, for example, when you right-click an object in the Solution Explorer, a context menu allows you to view the code or designer associated with that object. Figure 10.13. Because a context menu is not displayed in the menu bar, it does not have a top-level menu item, only submenus. 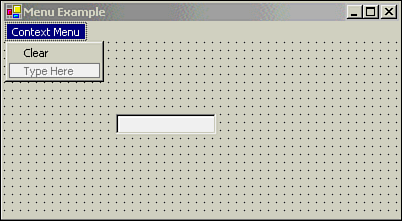 Designing the Menu You can create a pop-up menu in the same way that you created the main menu for your program with the Menu Editor. Just drag a ContextMenu control to the form and edit the menu items as you did with the MainMenu control. To add a context menu to our sample program, perform the following steps: -
Add a new ContextMenu control to the form. Set its Name property to mnuPopUp. -
Using the Menu Editor, add one menu item to the context menu. Set its Text property to Clear and its Name property to mnuClear. Your context menu in design mode should look similar to Figure 10.13. Associating a Context Menu with a Control After you have designed your menu, you need to set the ContextMenu property of a control or form to make the menu display. Go ahead and set the ContextMenu property of txtInfo to mnuPopUp and run the program. When you right-click the text box, you will see the Clear option appear. Note Text box controls have a default context menu that includes copy and paste commands. By associating your context menu with the text box control, you replace the default menu. Changing Menu Items with Code As you might imagine, writing VB code for items in a context menu is very similar to writing code for a main menu. In our sample context menu, you could add the following line of code to the Click event of mnuClear, which would clear the text box: txtInfo.Text = "" However, this code works for a specific text box. What if you want to associate the same context menu with every text box on your form? Fortunately, you can: Private Sub Form1_Load(ByVal sender As System.Object,_ ByVal e As System.EventArgs) Handles MyBase.Load 'During startup, we add a custom MouseDown event handler to all the text boxes Dim ctlTemp As Control For Each ctlTemp In Me.Controls If TypeOf ctlTemp Is TextBox Then AddHandler ctlTemp.MouseDown, AddressOf MyMouseDownEvent End If Next End Sub Private Sub MyMouseDownEvent(ByVal sender As Object, ByVal e As MouseEventArgs) 'The custom event handler creates a context menu dynamically 'for the text box that called it. Dim CurrentTextBox As TextBox = CType(sender, TextBox) If e.Button = MouseButtons().Right Then Dim PopUpMenu As New ContextMenu() Dim MyMenuItem As New MenuItem("Clear " & CurrentTextBox.Text) AddHandler MyMenuItem.Click, AddressOf ClearTextBox PopUpMenu.MenuItems.Add(MyMenuItem) CurrentTextBox.ContextMenu = PopUpMenu End If End Sub Private Sub ClearTextBox(ByVal sender As Object, ByVal e As System.EventArgs) 'A menu item fires this event, so we have to create several objects to 'work our way back to the source text box. Dim pmTemp As MenuItem = CType(sender, MenuItem) Dim cmTemp As ContextMenu = CType(pmTemp.Parent, ContextMenu) Dim CurrentTextBox As TextBox = CType(cmTemp.SourceControl, TextBox) CurrentTextBox.Text = "" End Sub As you can see, doing everything with code requires a lot more work than using the Menu Editor. (Keep in mind this can be simplified a bit by creating the menu controls in Design mode and manipulating them in code.) However, the advantage of the previous code is that it will work with any number of text boxes, and is able to use characteristics of the text box itself in the menu. For example, the menu item displays "Clear" followed by the text in the text box. Because this menu is created every time the user right-clicks the text box, the menu item will always be up to date. |