Another way to handle decisions in a program is to use the Select Case statement. It allows you to conditionally execute any of a series of statement groups based on the value of a test expression, which can be a single variable or a complex expression. The Select Case statement is divided into two parts: test expression to be evaluated and a series of Case statements listing the possible values. How Select Case Works The Select Case structure is similar to a series of If/Then/ElseIf statements. The following lines of code show the syntax of the Select Case block: Select Case testvalue Case value1 statement group 1 Case value2 statement group 2 End Select The first statement of the Select Case block is the Select Case statement itself. This statement identifies the value to be tested against possible results. This value, represented by the testvalue argument, can be any valid numeric or string expression, including literals, variables, or functions. Each conditional group of commands (those that are run if the condition is met) is started by a Case statement. The Case statement identifies the expression to which the testvalue is compared. If the testvalue is equal to the expression, the commands after the Case statement are run. The program runs the commands between the current Case statement and the next Case statement or the End Select statement. If the testvalue is not equal to the value expression, the program proceeds to compare the expression against the next Case statement. The End Select statement identifies the end of the Select Case block. Note Only one case in the Select Case block is executed for a given value of testvalue . Caution The testvalue and value expressions should represent the same data type. For example, if the testvalue is a number, the values tested in the Case statements also must be numbers. Case statements within a Select Case structure also can handle lists, ranges, and comparisons of values in addition to discrete values. Note the use of Case Is < 0, Case 1 to 9, and Case Is > 50 in this example: Select Case QuantityOrdered Case Is < 0 'note use of comparison MessageBox.Show("Order quantity cannot be negative!") Case 1, 2, 3 'note use of value list DiscountAmount = 0 Case 4 To 9 'note use of range DiscountAmount = 0.03 Case 10 To 49 DiscountAmount = 0.08 Case Is > 50 DiscountAmount = 0.1 End Select In the same Select Case statement, an integer quantity is compared against several values. Depending on the quantity value, a different discount amount is set. Handling Other Values The preceding example works fine if your test variable matches one of the conditions in a Case statement. But how do you handle other values that are outside the ones for which you tested? You can have your code do something for all other possible values of the test expression by adding a Case Else statement to your program. The Case Else statement follows the last command of the last Case statement in the block. You then place the commands that you want executed between the Case Else and the End Select statements. You can use the Case Else statement to perform calculations for values not specifically called out in the Case statements. Alternatively, you can use the Case Else statement to let users know that they entered an invalid value. Consider a simple form used to enter three test scores for a student into three text boxes. A Calculate button computes the average of the test scores and uses a Select Caseblock to determine the student's letter grade based on the average test score. Listing 7.1 shows the code behind the Calculate button's Click event. Listing 7.1 SELCASE.ZIP Using Select Case to Determine a Student's Letter Grade Private Sub btnCalc_Click(ByVal sender As System.Object,_ ByVal e As System.EventArgs) Handles btnCalc.Click Dim SumOfGrades As Integer Dim NumericAverage As Double SumOfGrades = Convert.ToInt32((Val(txtTest1.Text) +_ Val(txtTest2.Text) + Val(txtTest3.Text))) NumericAverage = (SumOfGrades / 3) txtAverage.Text = Format(NumericAverage, "n") Select Case Convert.ToInt32(NumericAverage) Case Is = 100 txtLetterGrade.Text = "A+" Case 93 To 99 txtLetterGrade.Text = "A" Case 83 To 92 txtLetterGrade.Text = "B" Case 73 To 82 txtLetterGrade.Text = "C" Case 63 To 72 txtLetterGrade.Text = "D" Case Is < 63 txtLetterGrade.Text = "F" End Select End Sub To test this code, follow these steps: -
Add five text boxes named txtTest1, txtTest2, txtTest3, txtAverage, and txtLetterGrade to a form. -
Add a command button named btnCalc. -
As with any form, you should add Label controls as appropriate to identify the text boxes. -
Enter the code from Listing 7.1 into the Click event procedure of btnCalc. -
Click the VB Start button. If you enter some grades into the input text boxes and click the button, the numeric and letter average will be displayed, as shown in Figure 7.1. Figure 7.1. To determine the letter grade, we convert the numeric average to an integer, which rounds it up, as shown here. 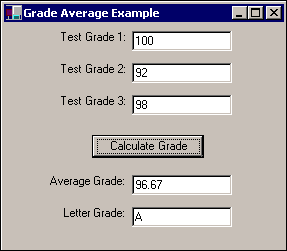 |