So far we have shown that the process of developing a Web application is very similar to that that of a Windows application with some subtle but important differences. Similarly, there are some special considerations that you may have to deal with when managing your Web project and associated files. For the most part, Web projects work as you would expect, but there are a few tricks you need to know. Working with an Offline Project When you create a new Web Application project, all the project files are stored on the Web server. As we discussed in Chapter 3, the Web server may or may not be running on the same physical computer on which Visual Studio is installed. Note Although the project code and compiled files are on the Web server, the solution file (.sln file) is stored locally. Suppose however, that you need to work on your Web application when the Web server is not available, such as when you are working on a disconnected laptop computer. In this case, you can work with your Web project in offline mode. The option to take a Web Project offline appears under the Project, Web Project menu, as pictured in Figure 18.6. Figure 18.6. When you take a Visual Studio Web Application Offline, you can work on a local copy, provided you have IIS installed. 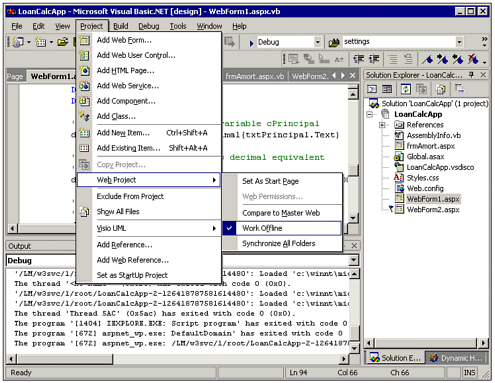 When you choose to take a project offline, Visual Studio works from the local VSWebCache directory, rather than the Web server's virtual directory for the project. As you work with the offline copy, you can add new pages. Until the pages are synchronized with the master copy on the Web server, they will appear with a flag icon next to them in the Solution Explorer, also shown in Figure 18.6. When you are finished working offline, deselect the Work Offline menu option from the Project menu. Your local files will be synchronized with the Web server and you can work online again. If the online project was modified while you were working offline, you will be prompted whether to load the new version. Note If you want to synchronize your files or check for changes while remaining offline, you can use the Synchronize and Compare options, also located in the Web Project menu. Setting the Start Page and Default Document Windows Applications with forms have a Startup form, which is displayed when the application starts. Similarly, Web applications have a Start page. You can set the Start page by using the Web Project menu described in the last section, or by right-clicking your form in the Solution Explorer and choosing Set as Start Page. When debugging in the Visual Studio environment, the Start Page is the page that opens the browser. From that point, links or controls on the Start Page can open other pages. However, the start page for a Web application only works in Visual Studio. To demonstrate, suppose you have created an accounting application located in the /accounting virtual directory of your Web server. It has two forms, login.aspx and invoiceentry.aspx. The URLs for these forms would be the following: http://servername/accounting/login.aspx http://servername/accounting/invoiceentry.aspx When you start your application in Visual Studio, the browser is automatically directed to the start page (presumably, login.aspx). However, users who need to run the application in production, that is, outside of Visual Studio, will have to type in the entire URL, including the aspx extension. A much easier address to remember is just the address of the virtual directory: http://servername/accounting Ideally, users should just be able to remember the address of the accounting application, not an individual page. To achieve this effect, your application needs a default document. A default document is a Web page that IIS automatically returns when just the virtual directory name is requested. The standard name for a default document is default, so in our example all you need to do is change the name of the startup Web form from login1.aspx to default.aspx. Alternatively, you could use the IIS management software to change the default for the directory, but it is much easier to change the name of the Web form without leaving Visual Studio. When you rename a Web form, Visual Studio automatically updates the name of the associated code behind file as well. Controlling Page Navigation Another concern with Web form addresses is that the end user can access them directly by simply remembering the name of the URL and typing it in the browser. In our example, you wouldn't want the end user to access the invoiceentry.aspx page without first visiting the login page. Not only would this be a security violation, but also the login page might perform database lookups or other information needed for the invoice entry page. To prevent this, you can use session variables. For example, the login page could set a session variable called LoggedIn to True, and then subsequent pages could check for this variable in their Page_Load event: If Not Session.Item("LoggedIn") Then Response.Redirect("default.aspx") End If The previous If statement checks to see whether the user has logged in before visiting this page. Depending on your level of security, you may need to perform additional checks to see whether they are authorized to visit the requested page. Laying Out Your Page Another difference between designing forms for windows and the Web is the pageLayout property of a Web form. As you may recall from Chapter 3, whenever you create a new Web form, a curious message regarding this property appears in the middle of the designer until you draw your first control. The message indicates which layout mode you are in. There are two values for this property: GridLayout Uses absolute positioning with x, y coordinates on a grid. FlowLayout You place elements on the Web page one after the other, giving up some control over their position. "Why," you might ask, "would I ever want to give up the additional control of the GridLayout mode?" The answer is that in some instances you may want the elements of your Web page to be responsive to the end user's browser resizing. Figure 18.7 shows an example of two rows of text boxes, which were drawn using different layout modes. Figure 18.7. The top row of text boxes was positioned using grid layout mode, the bottom row of text boxes uses flow layout and adjusted automatically to fit the browser size. 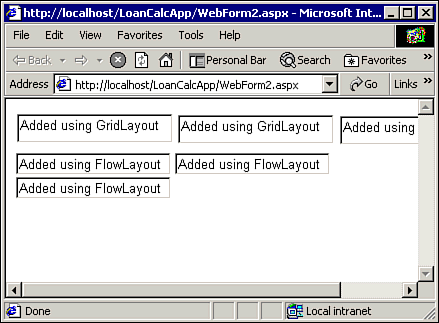 In the designer, the developer positioned the text boxes next to each other. However, only the text boxes positioned using FlowLayout mode responded to the resize of the browser. Making the objects on a Web form flow with the page may also become more advantageous in the future, as smaller mobile devices gain popularity. Note When you use FlowLayout mode, you cannot draw a control with the crosshairs. You must instead drag it from the toolbox to the designer. |