The variables you've created and used thus far can be accessed at any time by any script in the Flash movie. In contrast, local variables are special variables that you can only create and use within the scope of a function definition. In other words, a local variable is created within the function definition, used by the function when it's called, then deleted when that function has finished executing. Local variables exist only within the function definitions where they were created. Although local variables are not absolutely required in ActionScripting, it's good programming practice to use them. Applications that require many and frequent calculations end up creating a lot of variables which can slow applications. By using local variables, however, you minimize memory usage. And by employing them in function definitions, you also help prevent naming collisions which occur when your project gets so big that you unknowingly create and use variable names that are already in use. However, local variables in one function definition can have the same names as local variables within another function definition even if both definitions exist on the same timeline. This is because Flash understands that a local variable only has meaning within the function definition where it was created. There is just one way to manually create a local variable. Here's the syntax: var myName = "Jobe"; Note the use of var in the above syntax: It tells Flash to consider this particular variable as a local variable. If this line of code existed within a function definition, myName would only have meaning to (and could only be used by) that function. 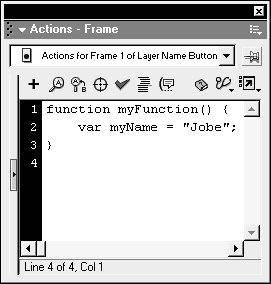 Multiple local variables can be declared on a single line using this syntax: var firstName = "Jobe", lastName = "Makar", email = "jobe@electrotank.com"; In addition to using local variables, you can build functions to return end results that is, returned values. Think of this as what happens when you enter numbers into a calculator: The calculator represents a function that tabulates numbers; the numbers entered represent parameter values sent to the function when it's called; and the total represents the result returned by the function once it's finished executing. Using a function return makes your code easy to read and update. The following represents the syntax for a return line: return myVariable; With a return, a function can perform all of its actions to determine a final result. This final result is then returned to the action line that called the function. When a return action is encountered in a function, execution of the actions in the function is immediately halted a handy feature if you want to stop a function before it has finished executing. Here's an example of a function definition that uses a local variable and a return: function convertToMoonWeight (myWeight) { var newWeight = myWeight/6.04; return newWeight; } This function is called in the following way: myWeightOnMoon = convertToMoonWeight(165); The above line of ActionScript uses a function call to determine the value of myWeightOnMoon . Since the function that's called is set up to return the value of newWeight , this will set the value of myWeightOnMoon to that value (27.32). The parameter myWeight is automatically created as a local variable and deleted when the function has executed. The local variable newWeight is also deleted when the function has finished its job. (This will make more sense in a moment.) In this exercise, you'll add a cable-box display to our project, which displays the name of the current channel. You will create a function that builds the text to be displayed on the cable box. TIP You can use the return action to return any data types, including variable values, arrays, or any other objects. -
Open television3.fla in the Lesson05/Assets folder. This file is set up in similar fashion to the one we just worked on, with the exception that it now contains a cable box below the television. This movie clip has an instance name of cableBox, and it contains a simple graphic and a text field with an instance name of cableDisplay. This text field will be filled with different channel names, depending on the channel selected. 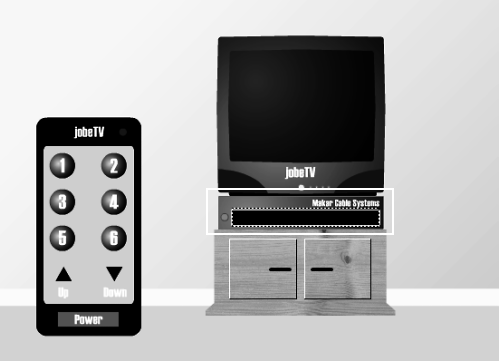 -
With the Actions panel open, select Frame 1 and enter the following ActionScript just below where it says numberOfChannels = 6; : channelNames = ["","News","Classics","Family","Cartoons","Horror","Westerns"]; You have just created an array named channelNames . This array contains names that will be dynamically inserted into a string of text that will be displayed inside the cable box. There are seven string elements in this array separated by commas. Each one of these elements has what is known as an associated index value, beginning with 0. For example, channelNames[0] = "" (or nothing), channelNames[1] = "News," channelNames[2] = "Classics," and so on. This is important to understand as we progress. NOTE For more information on arrays, see Lesson 7, Using Dynamic Data. Next, let's create a function that uses the text elements in this array to display a message in the cable box. -
With the frame still selected, enter the following ActionScript at the end of all scripts on Frame 1: function displayCableText () { if (currentChannel != 0) { var displayText = "You are viewing " + channelNames[currentChannel] + "."; } else { var displayText = ""; } return displayText; } This defines the displayCableText() function, which accepts no parameters. It is used to dynamically build a string of text that will eventually appear in the cableDisplay text field. This function then takes this string and returns it using the return action. The function contains a conditional statement that checks to make sure that the television channel is not the channel associated with the TV being in the off state (0). If the condition is satisfied, a local variable named displayText is created, and a line of text is dynamically built from the channelNames array as well as the current value of currentChannel . For example, if the value of currentChannel is 4 at the time this function is called and executed, this would essentially be the same as the following: var displayText = "You are viewing " + channelNames[4] + "."; Since "Cartoons" exists at index position 4 of the channelNames array, it can be further broken down: var displayText = "You are viewing Cartoons"; If the first part of the conditional statement is not satisfied (else ), the local variable displayText is created with no value (or simply ""). The function ends by returning the value of displayText . But where does this value actually get returned to? We'll explain that in the next step. Since displayText has been specified as a local variable (using var ), it's removed from memory as soon as its value is returned,. -
With the Actions panel still open, modify the changeTheChannel() function by inserting the following line of code after the fifth line of the function definition: cableBox.cableDisplay.text = displayCableText(); 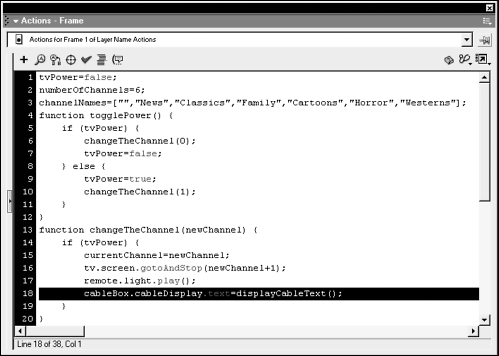 You have modified changeTheChannel() so that each time a channel is changed and the changeChannel() function is called, the cableDisplay text field (inside the cableBox movie clip instance) will be updated with the correct text. This line of ActionScript sets the value of the text field instance cableDisplay (which is actually the dynamic text field in our cable box) using the returned value of the displayCableText() function. Thus, the displayCable() function is called, goes to work, and comes up with a value. That value is then inserted after the equals sign. This is what's meant by a function returning a value. The value the function comes up with is returned to the line of script that called the function (as shown above). This is also a great example of how using functions can be a real time-saver. We've enhanced changeTheChannel() in a single location, but any script that calls the function will automatically execute this enhancement as well very efficient! -
Choose Control > Test Movie. Select the Power button to turn the television on. Change the channel a few times. Every time you select a button that changes the channel, the cable box is updated with the name of the channel you're watching. You have created a simple application that uses six functions to perform several tasks. 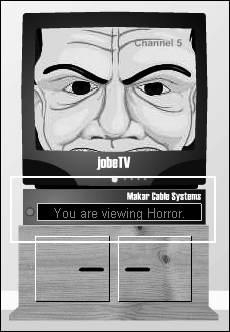 -
Close the test movie and save your work as television4.fla. You're now finished with this file. You'll apply what you've learned here in lessons to come. |