In chapter 10, we show you how to connect to a database and perform queries. In this section, we show you how to display the results of a database query. Figure 5-9 shows a JSF application that displays a database table. Figure 5-9. Displaying a Database Table 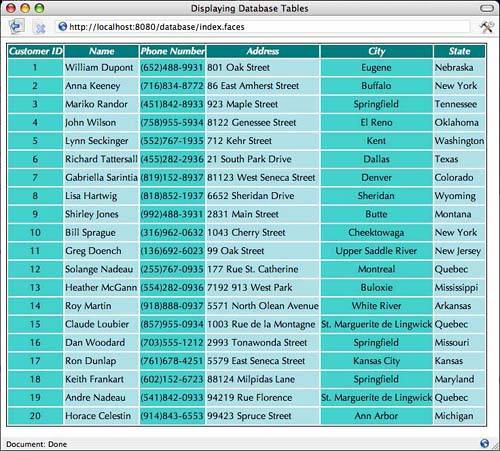
The JSF page shown in Figure 5-9 uses h:dataTable like this:
<h:dataTable value="#{customer.all}" var="customer" style header columnClasses="custid,name"> <h:column> <f:facet name="header"> <h:outputText value="#{msgs.customerIdHeader}"/> </f:facet> <h:outputText value="#{customer.Cust_ID}"/> </h:column> <h:column> <f:facet name="header"> <h:outputText value="#{msgs.nameHeader}"/> </f:facet> <h:outputText value="#{customer.Name}"/> </h:column> ... </h:dataTable> The customer bean is a managed bean that knows how to connect to a database and perform a query of all customers in the database. The CustomerBean.all method performs that query and returns a JSTL Result object. The preceding JSF page accesses column data by referencing column names; for example, #{customer.Cust_ID} references the Cust_ID column. In this example, we've strictly adhered to a column's capitalization, but we weren't required to; for example, #{customer.CuSt_Id} would work equally well in the preceding code. The directory structure for the database example is shown in Figure 5-10. Listings for the application are given in Listing 5-13 through Listing 5-16. Listing 5-13. database/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="styles.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.pageTitle}"/> 10. </title> 11. </head> 12. <body> 13. <h:form> 14. <h:dataTable value="#{customer.all}" var="customer" 15. style 16. header columnClasses="custid,name"> 17. <h:column> 18. <f:facet name="header"> 19. <h:outputText value="#{msgs.customerIdHeader}"/> 20. </f:facet> 21. <h:outputText value="#{customer.Cust_ID}"/> 22. </h:column> 23. <h:column> 24. <f:facet name="header"> 25. <h:outputText value="#{msgs.nameHeader}"/> 26. </f:facet> 27. <h:outputText value="#{customer.Name}"/> 28. </h:column> 29. <h:column> 30. <f:facet name="header"> 31. <h:outputText value="#{msgs.phoneHeader}"/> 32. </f:facet> 33. <h:outputText value="#{customer.Phone_Number}"/> 34. </h:column> 35. <h:column> 36. <f:facet name="header"> 37. <h:outputText value="#{msgs.addressHeader}"/> 38. </f:facet> 39. <h:outputText value="#{customer.Street_Address}"/> 40. </h:column> 41. <h:column> 42. <f:facet name="header"> 43. <h:outputText value="#{msgs.cityHeader}"/> 44. </f:facet> 45. <h:outputText value="#{customer.City}"/> 46. </h:column> 47. <h:column> 48. <f:facet name="header"> 49. <h:outputText value="#{msgs.stateHeader}"/> 50. </f:facet> 51. <h:outputText value="#{customer.State}"/> 52. </h:column> 53. </h:dataTable> 54. </h:form> 55. </body> 56. </f:view> 57. </html> Listing 5-14. database/WEB-INF/classes/com/corejsf/CustomerBean.java 1. package com.corejsf; 2. 3. import java.sql.Connection; 4. import java.sql.ResultSet; 5. import java.sql.SQLException; 6. import java.sql.Statement; 7. import javax.naming.Context; 8. import javax.naming.InitialContext; 9. import javax.naming.NamingException; 10. import javax.servlet.jsp.jstl.sql.Result; 11. import javax.servlet.jsp.jstl.sql.ResultSupport; 12. import javax.sql.DataSource; 13. 14. public class CustomerBean { 15. private Connection conn; 16. 17. public void open() throws SQLException, NamingException { 18. if (conn != null) return; 19. Context ctx = new InitialContext(); 20. DataSource ds = (DataSource) ctx.lookup("java:comp/env/jdbc/test"); 21. conn = ds.getConnection(); 22. } 23. 24. public Result getAll() throws SQLException, NamingException { 25. try { 26. open(); 27. Statement stmt = conn.createStatement(); 28. ResultSet result = stmt.executeQuery("SELECT * FROM Customers"); 29. return ResultSupport.toResult(result); 30. } finally { 31. close(); 32. } 33. } 34. 35. public void close() throws SQLException { 36. if (conn == null) return; 37. conn.close(); 38. conn = null; 39. } 40. } Listing 5-15. database/WEB-INF/faces-config.xml 1. <?xml version="1.0"?> 2. 3. <!DOCTYPE faces-config PUBLIC 4. "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" 5. "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> 6. 7. <faces-config> 8. <managed-bean> 9. <managed-bean-name>customer</managed-bean-name> 10. <managed-bean-class>com.corejsf.CustomerBean</managed-bean-class> 11. <managed-bean-scope>session</managed-bean-scope> 12. </managed-bean> 13. </faces-config> Listing 5-16. database/WEB-INF/classes/com/corejsf/messages.properties 1. pageTitle=Displaying Database Tables 2. customerIdHeader=Customer ID 3. nameHeader=Name 4. phoneHeader=Phone Number 5. addressHeader=Address 6. cityHeader=City 7. stateHeader=State 8. refreshFromDB=Read from database Figure 5-10. The Directory Structure for the Database Example 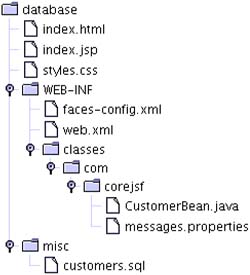
JSTL Result vs. Result Sets The value you specify for h:dataTable can be, among other things, an instance of javax.servlet.jsp.jstl.Result or an instance of java.sql.Result as was the case in "Database Tables" on page 179. h:dataTable wraps instances of those objects in instances of ResultDataModel and ResultSetDataModel, respectively. So how do the models differ? And which should you prefer? If you've worked with result sets, you know they are fragile objects that require a good deal of programmatic control. The JSTL Result class is a bean that wraps a result set and implements that programmatic control for you; results are thus easier to deal with than are result sets. On the other hand, wrapping a result set in a result involves some overhead involved in creating the Result object; your application may not be able to afford the performance penalty. In the application discussed in "Database Tables" on page 179, we follow our own advice and return a JSTL Result object from the CustomerBean.all method. |