Figure 5-1 shows a table of names. Figure 5-1. A Simple Table 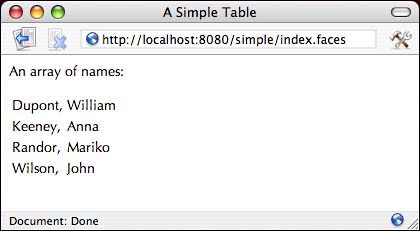
The directory structure for the application shown in Figure 5-1 is shown in Figure 5-2. The application's JSF page is given in Listing 5-1. Listing 5-1. simple/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 7. <title> 8. <h:outputText value="#{msgs.windowTitle}"/> 9. </title> 10. </head> 11. <body> 12. <h:outputText value="#{msgs.pageTitle}"/> 13. <p> 14. <h:form> 15. <h:dataTable value="#{tableData.names}" 16. var="name"> 17. <h:column> 18. <h:outputText value="#{name.last}"/> 19. <f:verbatim>,</f:verbatim> 20. </h:column> 21. 22. <h:column> 23. <h:outputText value="#{name.first}"/> 24. </h:column> 25. </h:dataTable> 26. </h:form> 27. </body> 28. </f:view> 29. </html> Figure 5-2. The Directory Structure for the Simple Example 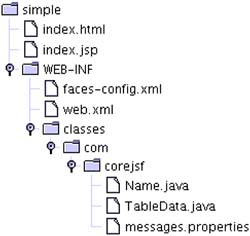
In Listing 5-1 we use h:dataTable to iterate over an array of names. The last name followed by a comma is placed in the left column and the first name is placed in the right column. Notice that we enclose the comma in an f:verbatim tag. Anytime you specify template text meaning anything other than a JSF tag inside of a component that renders its children, you must wrap that template text in the body of an f:verbatim tag. The following JSF HTML tags represent components that render their children. h:dataTable h:panelGrid h:panelGroup h:commandLink h:outputLink If you place template text in the body of any of the preceding tags, you must enclose that template text in an f:verbatim tag (or alternatively, produce that template text with an h:outputText tag). The array of names in this example is instantiated by a bean, which is managed by JSF. That bean is an instance of com.corejsf.TableData, which is listed in Listing 5-3. Listing 5-2 shows the Name class, and the faces configuration file and message resource bundle are listed in Listing 5-4 and Listing 5-5, respectively. Listing 5-2. simple/WEB-INF/classes/com/corejsf/Name.java 1. package com.corejsf; 2. 3. public class Name { 4. private String first; 5. private String last; 6. 7. public Name(String first, String last) { 8. this.first = first; 9. this.last = last; 10. } 11. 12. public void setFirst(String newValue) { first = newValue; } 13. public String getFirst() { return first; } 14. 15. public void setLast(String newValue) { last = newValue; } 16. public String getLast() { return last; } 17. } Listing 5-3. simple/WEB-INF/classes/com/corejsf/TableData.java 1. package com.corejsf; 2. 3. public class TableData { 4. private static final Name[] names = new Name[] { 5. new Name("William", "Dupont"), 6. new Name("Anna", "Keeney"), 7. new Name("Mariko", "Randor"), 8. new Name("John", "Wilson") 9. }; 10. 11. public Name[] getNames() { return names;} 12.} Listing 5-4. simple/WEB-INF/faces_config.xml 1. <?xml version="1.0"?> 2. 3. <!DOCTYPE faces-config PUBLIC 4. "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" 5. "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> 6. 7. <faces-config> 8. <managed-bean> 9. <managed-bean-name>tableData</managed-bean-name> 10. <managed-bean-class>com.corejsf.TableData</managed-bean-class> 11. <managed-bean-scope>session</managed-bean-scope> 12. </managed-bean> 13. </faces-config> Listing 5-5. simple/WEB-INF/classes/com/corejsf/messages.properties 1. windowTitle=A Simple Table 2. pageTitle=An array of names: The table in Figure 5-1 is intentionally vanilla. Throughout this chapter we'll see how to add bells and whistles, such as CSS styles and column headers, to tables, but first let's take a short tour of h:dataTable attributes. CAUTION  | h:dataTable data is row oriented; for example, the list of names in Listing 5-3 correspond to table rows, but the names say nothing about what's stored in each column it's up to the page author to specify column content. Row-oriented data might be different from what you're used to; Swing table models, for example, keep track of what's in each row and column. |
|