During the JSF life cycle, any object can create a message and add it to a queue of messages maintained by the faces context. At the end of the life cycle in the render response phase you can display those messages in a view. Typically, messages are associated with a particular component and indicate either conversion or validation errors. Although error messages are usually the most prevalent message type in a JSF application, messages come in four varieties: Information Warning Error Fatal All messages can contain a summary and a detail. For example, a summary might be Invalid Entry and a detail might be The number entered was greater than the maximum. JSF applications use two tags to display messages in JSF pages: h:messages and h:message. The h:messages tag displays all messages that were stored in the faces context during the course of the JSF life cycle. You can restrict those messages to global messages meaning messages not associated with a component by setting h:message's globalOnly attribute to true. By default, that attribute is false. The h:message tag displays a single message for a particular component. That component is designated with h:message's mandatory for attribute. If more than one message has been stored in the Faces context for a component, h:message shows only the last message that was created. h:message and h:messages share many attributes. Table 4-23 lists all attributes for both tags. Table 4-23. Attributes for h:message and h:messagesAttributes | Description |
---|
errorClass | CSS class applied to error messages | errorStyle | CSS style applied to error messages | fatalClass | CSS class applied to fatal messages | fatalStyle | CSS style applied to fatal messages | globalOnly | Instruction to display only global messages applicable only to h:messages. Default: false | infoClass | CSS class applied to information messages | infoStyle | CSS style applied to information messages | layout | Specification for message layout: table or list applicable only to h:messages | showDetail | A boolean that determines whether message details are shown. Defaults are false for h:messages, true for h:message. | showSummary | A boolean that determines whether message summaries are shown. Defaults are true for h:messages, false for h:message. | tooltip | A boolean that determines whether message details are rendered in a tooltip; the tooltip is only rendered if showDetail and showSummary are true | warnClass | CSS class for warning messages | warnStyle | CSS style for warning messages | binding, id, rendered, styleClass | Basic attributes[a] | style, title | HTML 4.0[b] |
[a] See Table 4-3 on page 90 for information about basic attributes.
[b] See Table 4-4 on page 93 for information about HTML 4.0 attributes. The majority of the attributes in Table 4-23 represent CSS classes or styles that h:message and h:messages apply to particular types of messages. You can also specify whether you want to display a message's summary or detail, or both, with the showSummary and showDetail attributes, respectively. The h:messages layout attribute can be used to specify how messages are laid out, either as a list or a table. If you specify true for the tooltip attribute and you've also set showDetail and showSummary to true, the message's detail will be wrapped in a tooltip that's shown when the mouse hovers over the error message. Now that we have a grasp of message fundamentals, let's take a look at an application that uses the h:message and h:messages tags. The application shown in Figure 4-9 contains a simple form with two text fields. Both text fields have required attributes. Moreover, the Age text field is wired to an integer property, so it's value is converted automatically by the JSF framework. Figure 4-9 shows the error messages generated by the JSF framework when we neglect to specify a value for the Name field and provide the wrong type of value for the Age field. Figure 4-9. Displaying Messages 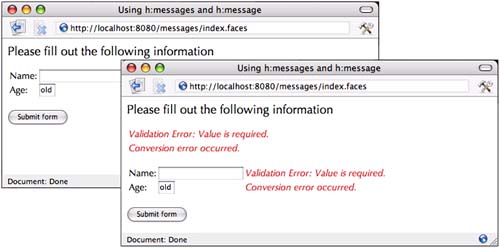 At the top of the JSF page we use h:messages to display all messages. We use h:message to display messages for each input field.
<h:form> <h:messages layout="table" error/> ... <h:inputText required="true"/> <h:message for="name" error/> ... <h:inputText value="#{form.date}" required="true"/> <h:message for="date" error/> ... </form> Both tags in our example specify a CSS class named errors, which is defined in styles.css. That class definition looks like this:
.errors { font-style: italic; } We've also specified layout="table" for the h:messages tag. If we had omitted that attribute (or alternatively specified layout="list"), the output would look like this: The list layout encodes the error messages, one after the other, as text, whereas the table layout puts the messages in an HTML table element, one row per message. Most of the time you will probably prefer the table layout (even though it's not the default) because it's easier to read. Figure 4-10 shows the directory structure for the application shown in Figure 4-9. Listing 4-16 through Listing 4-18 list the JSP page, resource bundle, and stylesheet for the application. Listing 4-16. messages/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 6. <head> 7. <link href="styles.css" rel="stylesheet" type="text/css"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. <body> 13. <h:form> 14. <h:outputText value="#{msgs.greeting}" style/> 15. <br/> 16. <h:messages error/> 17. <br/> 18. <table> 19. <tr> 20. <td> 21. <h:outputText value="#{msgs.namePrompt}"/> 22. </td> 23. <td> 24. <h:inputText 25. value="#{user.name}" required="true"/> 26. </td> 27. <td> 28. <h:message for="name" error/> 29. </td> 30. </tr> 31. <tr> 32. <td> 33. <h:outputText value="#{msgs.agePrompt}"/> 34. </td> 35. <td> 36. <h:inputText 37. value="#{user.age}" required="true" size="3"/> 38. </td> 39. <td> 40. <h:message for="age" error/> 41. </td> 42. </tr> 43. </table> 44. <br/> 45. <h:commandButton value="#{msgs.submitPrompt}"/> 46. </h:form> 47. </body> 48. </f:view> 49. </html> Listing 4-17. messages/WEB-INF/classes/com/corejsf/messages.properties 1. windowTitle=Using h:messages and h:message 2. greeting=Please fill out the following information 3. namePrompt=Name: 4. agePrompt=Age: 5. submitPrompt=Submit form Listing 4-18. messages/styles.css 1. .errors { 2. font-style: italic; 3. } 4. .emphasis { 5. font-size: 1.3em; 6. } Figure 4-10. Directory Structure for the Messages Example 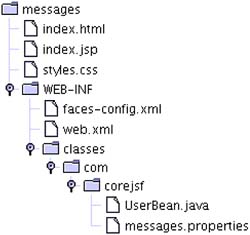
NOTE  | By default, h:messages shows message summaries but not details. h:message, on the other hand, shows details but not summaries. If you use h:messages and h:message together, as we did in the preceding example, summaries will appear at the top of the page, with details next to the appropriate input field. |
|