The Java platform is an ideal platform for developing XML-based applications such as Web services. This is because of the following reasons: -
Java provides a rich set of capabilities to do object-oriented programming without the complexity found in languages such as C++. -
Java provides a rich set of standardized classes. -
Java is portable. -
Java provides an extension mechanism to extend the functionality of the core platform. -
Java provides extensions for component development and reuse. -
The J2EE platform provides the necessary support for security, scalability, and so on, that is required for deploying and supporting Web services. XML and Java APIs for XML: A Quick Look The Java platform has an extended framework that provides standardized APIs to handle XML data and build Web services. These APIs are available through two downloadable packs: the Java Web Services Developer Pack (JWSDP) and the Java XML Pack. The Java XML Pack contains the core APIs that enable you to build XML-based applications. The JWSDP is a superset of the two. It contains the Java XML Pack and other components such as the Tomcat Web server and the ant build tool that simplify the process of developing and deploying Web services using the Java platform. The core APIs that enable you to develop XML-based applications are as follows: -
Java API for XML Processing (JAXP) -
Java Architecture for XML Binding (JAXB) -
Java API for XML Messaging (JAXM) -
Java API for XML-based RPC (JAX-RPC) -
JavaAPI for XML Registries (JAXR) The first two APIs (JAXP and JAXB) are essentially document-oriented, in the sense that they work directly with XML documents. The remaining three APIs (JAXM, JAXR, and JAX-RPC) are procedure-oriented. This means that these APIs are useful in developing business procedures where XML is used as the primary information exchange medium. For example, JAXM enables you to do business messaging over the Internet using the SOAP protocol. The Java APIs for XML have two very important features, which make them the ideal choice for developing XML-based applications: -
Complete adherence and support to industry standards developed by standards bodies and industry consortia such as W3C, UDDI, and so on. This implies that applications based on these APIs will be interoperable with all other applications based on these standards. These applications could have been developed in any language, including but not limited to Java. -
Flexibility and ease of use to developers. For example, when using JAXP to process an XML document, you can choose between either SAX or DOM, and at runtime decide which implementation of parser to use. Also, using JAXB, you can very easily create Java objects from an XML DTD, manipulate them, and write back as an XML document without needing to write complex parsing and processing code. Let us now look at each of the APIs in a little more detail. JAXP One of the document-centric APIs, JAXP provides the means by which you can parse, manipulate, and transform XML documents from within a Java application. JAXP supports the Simple API for XML (SAX) 2.0 and Document Object Model (DOM) 2.0 parser standards for processing XML documents, along with the XSLT standards for transforming XML documents to other XML documents and display formats such as HTML. The JAXP support for SAX enables you to parse the XML document as events, thereby making the parsing of XML documents extremely fast. The support for DOM standard ensures that the XML data can be loaded in memory as data-objects that can be manipulated in the Java application. The XSLT support ensures that you can translate the XML document into another XML document with completely different tags, apply stylesheets, and write out XML documents in different formats such as HTML, WML, and so on. But then why use JAXP? After all, there are a number of SAX, DOM, and XSLT parsers, all of which have their own published APIs that can be used as well. To answer this question, let us first look at the issues involved in using a parser-specific API set. When using a parser-specific API set, you need to be aware of the issues that might arise when using vendor-dependent APIs. For example, in any application that uses a SAX parser, you need to get an instance of the SAX parser class. If you were using the Xerces parser, then you need to explicitly import the org.apache.xerces.parsers.SAXParser class to get access to the SAX parser. This affects the portability of the code, because the code cannot be run or compiled on a platform that does not have the Xerces parser. Another situation where the code could break is if there is a change in the packaging structure of the Xerces parser. Also, after the application's release, there might be a new and more optimized version of the parser available. To handle these changes, you need to edit the source code and recompile. Not a very rosy picture, to say the least. Now imagine a situation in which you do not have to do any of the previously mentioned tasks and can still use any parser of your choice, and have the capability to change the parser at runtime. This is exactly what JAXP provides. JAXP provides a platform-independent and consistent set of APIs for using the SAX, DOM, and XSLT parsers. Additionally, the JAXP architecture has a pluggability layer that enables JAXP to use any SAX, DOM, or XSLT parser of your choice at runtime. JAXP also provides support for XML namespaces, thereby ensuring that there is no conflict when processing XML documents that have elements from different DTDs. Additionally, if you're using the DOM parser, JAXP provides a method to bootstrap a DOM Document object from an XML document. At the time of this writing, this feature was not available in any of the DOM 2.0 parsers. JAXB Another of the document-centric APIs for XML is JAXB. Before beginning the discussion about the JAXB API, let us look at the need that it fills. An XML document that follows a DTD can be thought of as an instance of the DTD. This is analogous to a Java object being an instance of a class. Also, the Java programming language provides a standard and convenient way to access and manipulate objects. Therefore, it would greatly simplify things if there were a mechanism by which the XML data can be represented as objects in a Java application. In that case you could concentrate on developing applications that use the XML data, rather than spending time on writing code for parsing and processing XML documents. The JAXB API fills this need. By means of a schema compiler and a runtime framework, it provides a two-way mapping between XML documents and Java objects. By providing a DTD and a binding schema, the schema compiler generates the required Java classes from the schema. A binding schema is an XML document that contains instructions on how to bind a schema to classes, such as what data type to bind an attribute value to in the generated class. For example, you can bind the content of the element Date to the DATE data type in the generated class. As a developer, you can use these generated classes to create the object tree representing the data hierarchy of the XML document, and manipulate the objects as you would do normally for any Java objects. The JAXB API also provides the necessary mechanism to regenerate the XML from the object tree. There are other benefits of using JAXB as well. First, the generated classes contain all the necessary code to parse XML documents based on the schema. This saves you the time you would have spent writing XML-parsing code, which can be used to write an application that does something useful with the XML data. Secondly, the JAXB API provides structure and content validation in the generated code. This is possible because JAXB writes out Java code from the XML DTD. Therefore, by using an appropriate binding schema, you can decide on the data types for each of the elements in a schema. This type of validation is not possible in a DTD. For example, you might have an element called Date. In a DTD, there is no way to validate that the content between <Date> and </Date> is a date value, and not junk. But when using JAXB, you can ensure that the data type for the Date element is only of type Date. Thirdly, applications using JAXB are faster, and JAXB is more convenient to use than the SAX and DOM parsers. Being serial processors by design, the SAX parsers are fast. But they do not store the information obtained from the XML document in memory. The DOM parsers, on the other hand, store the entire XML document in memory. This allows the XML document to be manipulated. However, because it loads the contents into memory and also contains a lot of tree manipulation features, it makes your applications slower. The JAXB API provides the best of both worlds. It is as fast as the SAX parser, and also creates an in-memory data object representation of the XML document. It is faster than DOM because it does not contain the tree manipulation features of DOM. The manipulation of the objects is left to the application. Finally, applications based on JAXB are extensible. This is because JAXB generates Java classes, which can be subclassed for providing additional features. JAXM JAXM enables developers to write applications that generate XML messages based on industry standard SOAP 1.1 and SOAP with Attachment specifications. The messages are delivered using standard Internet transport mechanisms such as HTML, SMTP, FTP, and so on. Simple Object Access Protocol (SOAP) is an XML-based protocol that enables programs running on heterogeneous hardware and software in a distributed environment to communicate with each other and do remote procedure calls (RPCs). All communications in the Web service architecture are done via SOAP. SOAP is discussed in greater detail in Chapter 2, "Components of Web Services." JAXM is extensible by design. This is made possible through the use of profiles. A profile is a specific implementation of SOAP, such as ebXML, which is based on SOAP 1.1 and SOAP with Attachments specifications. The SOAP specification itself is a very basic packaging model and provides no specific addressing scheme or message identification information. A profile, being a specific implementation, provides the necessary information, such as the packaging model, addressing scheme, and so on. For example, the ebXML profile specifies how a SOAP header has to be created with the addressing and message identification information. An application that implements JAXM is called the JAXM client. Normally, a business also uses a messaging provider, which takes care of processes such as message routing, message delivery, and so on. A message provider that supports JAXM is called the JAXM provider. A JAXM client can either directly send a SOAP message, or use the JAXM provider. In case the JAXM provider is used, the JAXM client sends a SOAP message to its JAXM provider, which transmits the SOAP message to the recipient's provider. The recipient's message provider then forwards the message to the recipient client. When a messaging provider is used, a JAXM client can act both as a client and a server. This implies that a JAXM client can send and receive SOAP messages and process them. For example, consider three JAXM clients, B and C. A sends a SOAP message to B. In this case, A acts as the client and B as the server. B processes the message and forwards the message to C. In this scenario, C is the server, and B is the client. A JAXM client can also directly send messages to the recipient application. In this scenario, the JAXM client can only send SOAP messages and accept responses for the sent message. It cannot act as a server to process SOAP messages sent from other clients. Because it's based on W3C standards, a JAXM client can communicate with any application that is capable of producing and consuming SOAP messages. A JAXM message itself is made up of two parts: the required SOAP part and the optional attachment part. The SOAP part is the standard SOAP envelope that contains the Header and the Body elements. The content of the message is stored in the Body element. All non-XML content of the message is stored in the attachment part of the message. JAXR Registries are the yellow pages of the Internet. They are Web-based services that enable companies to register information about the products and services they offer. Using registries, businesses can query and discover other businesses with which they want to do business. A company (called the seller) that is providing certain services registers itself with a registry. A company (called the buyer) desirous of a particular product or service looks in the registry for the service and finds the seller. The buyer and seller then agree on a mutually agreeable collaborative process, which might include the DTD for their industry's standards, after which the buyer can access and use the services hosted by the seller. The purpose of registries is to facilitate the growth of e-business. At the time of this writing, there are several standards for registries, such as the Organization for the Advancement of Structured Information Standards (OASIS), the Electronic Business using eXtensible Markup Language (ebXML), the eCo Framework, Universal Description, Discovery, and Integration (UDDI), and so on. These registries are either based on open standards or are promoted by industry consortiums. These registries provide their own APIs, which you can use to manage and query information in the registries. NOTE The registry standards exist to facilitate and promote e-commerce through the use of XML. A detailed discussion about these standards is beyond the scope of this book. However, you can access the Web sites for these standards to find out more about them: -
OASIS http://www.oasis-open.org/ -
ebXML http://www.ebxml.org/ -
eCO Framework http://www.commerce.net/projects/currentprojects/eco/ -
UDDI http://www.uddi.org/ As with the SAX or DOM APIs, using a registry-specific API set results in code changes and recompilation if you need to change over from one registry to another. So, similar to JAXP, JAXR provides a convenient and standard API to access the registries over the Internet. Figure 1.1 shows how JAXR enables you to access diverse registries. 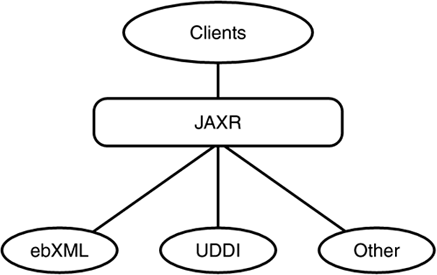 Therefore, JAXR can be used in a Java application to publish business information in public registries and query the registries for information in a standard manner. JAX-RPC RPC (Remote Procedure Calls) is the mechanism by which an application executes procedures on remote systems. RPC is a complex process, and RPC over the Internet brings its own set of complexities because of the heterogeneous systems on which applications are hosted. JAX-RPC provides a mechanism by which applications can make RPC using the XML-based protocol, SOAP. SOAP is a natural choice because the specification provides mechanisms for representing remote procedure calls. The API ensures that RPC and its responses are done using SOAP messages over the HTTP protocol. When using JAX-RPC, you do not need to worry about the complexities of RPC such as data marshaling and unmarshaling. As with other Java RPC APIs, such as RMI and CORBA/IDL, JAX-RPC hides the complexity from you, thereby enabling you to concentrate on the business logic rather than plumbing code. Also, because the JAX-RPC is based on W3C standards such as SOAP and HTTP, an application using JAX-RPC can also access Web services that are not running on the Java platform. |