The next procedure that you need to learn is how to create JSPs and servlets using the IDE. These types of programs are different from Java applications in that they normally run as part of a set of components instead of as a single class file. The set of all files that make up one application is called a Web Module in Sun ONE Studio. A Web Module is a specialized file structure that contains a tree of directories that conform to the Java Servlet Specification Version 2.3. According to this specification, the structured hierarchy consists of a root directory, which contains HTML and JSP pages that are accessed directly by the browser. A special nonpublic directory called WEB-INF is created underneath the root. This includes the deployment descriptor file, the servlets, and the utility classes used by the application. Before we create the Web Module, let's first create a new project called WebProject1 using the procedure that you learned earlier in this chapter. Click the Project Tab in the Explorer Pane. Next, right-click the name of this project in the Explorer Pane and choose Add New. This will start the New Wizard. Expand the item in the New Wizard that is called JSP and Servlet. Figure B.7 shows this wizard. Figure B.7. The New Wizard can be used to create JSP and Servlet projects also. 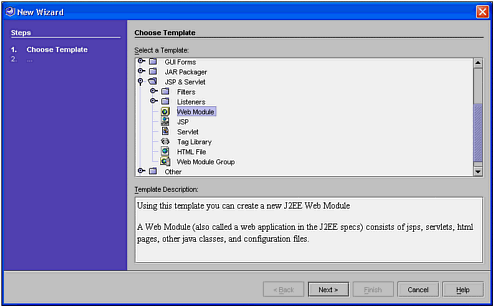 Click the Web Module option, and then click the Next button. When the Choose Target dialog appears, enter a location in the file system such as c:\jpp. This directory will be created automatically and the Web Module's file structure will be built under this location. Click the Finish button. Click the Filesystem Tab in the Explorer Pane and expand the WEB-INF directory. Figure B.8 shows the file structure after the Web Module is created. Figure B.8. Several new files appear under the target directory. 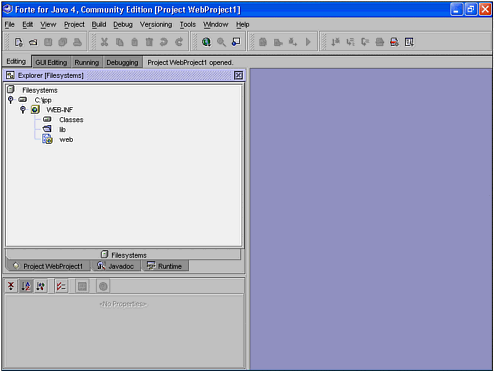 Each of these entities has a role that it plays in the project: The WEB-INF directory references all the items in the module. The Classes directory contains the servlets, Beans, and other classes that are instantiated by classes in this module. The lib folder contains tag libraries and imported JAR files. The web.xml file is a deployment descriptor for this application. It contains instructions about how this application is to be built. Adding a JSP Page Adding a JSP page to this Web Module is easy to do using the IDE. The first step is to create the JSP page using the New Wizard. To do this, you can right-click the project root node in the Project tab of the Explorer Pane. Choose Add New and the New Wizard will appear. Expand the JSP & Servlet item, and select the JSP item and click Next. A dialog will appear that will give you a chance to name the JSP page. Choose the name (ours is named FirstJSPage), but leave the package blank. Choose the root directory, c:\jpp as the directory for this file as shown here in Figure B.9. Figure B.9. The Add Wizard can also be used for JSP files. 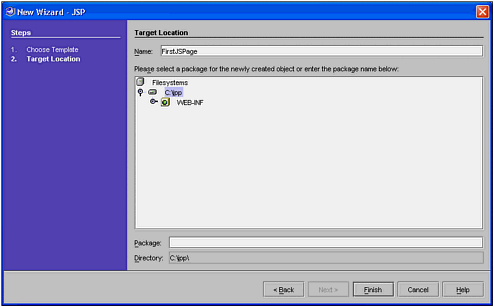 Caution  | Because different versions of Forte vary slightly in appearance, there might be small differences in the way your screens look. |
Click the Finish button, and you will see the JSP filename appear in the Filesystem Tab of the Explorer Panel. Double-click the filename in the Explorer to see the source code for the JSP page appear in the Editing Panel. Edit the JSP page by adding the following line to it in the body below the tags: This is the first JSP Page that we created. Listing B.2 shows the source code for this simple JSP page. Listing B.2 The FirstJSPage.jsp File <%@page contentType="text/html"%> <html> <head><title>JSP Page</title></head> <body> <%-- <jsp:useBean scope="session" /> --%> <%-- <jsp:getProperty name="beanInstanceName" property="propertyName" /> --%> This is the first JSP Page that we created. </body> </html> Running this JSP page from within the IDE is simple. All that you have to do is choose Execute from the Build menu. This will start a browser and display the JSP page, which is shown here in Figure B.10. Figure B.10. The JSP page execution causes a browser to open and display the result. 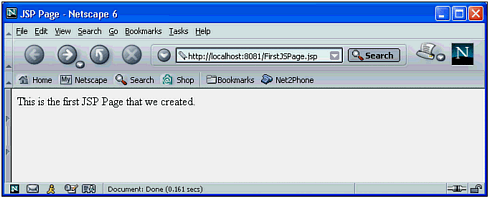 Notice that this JSP page is being run on a machine called localhost and on port 8081. This is the URL of your machine's copy of the Tomcat Web Server, which was installed along with the IDE. This server is integrated with the IDE in such a way that it is not necessary to even be aware that it is running behind the scenes. The IDE contains several panels that all deal with this one JSP, as shown in Figure B.11. Figure B.11. The IDE provides a lot of information about the JSP page being created. 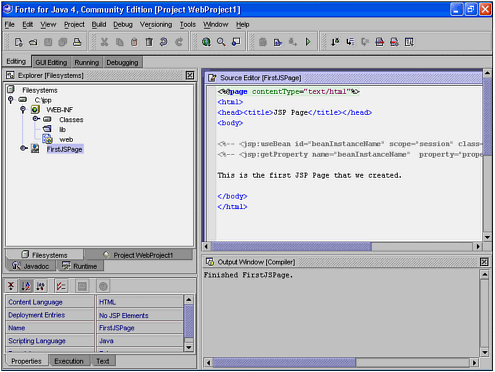 On the left is the Explorer with the JSP page highlighted. On the right is the Editing Panel with the source code displayed in editing mode. Below that, the output panel contains the compiler messages. If your page contains errors, they will appear here. During the execution of the page, the layout of the IDE changes. The Explorer is hidden and the Execution View takes over that part of the screen. Figure B.12 shows the IDE while the JSP is running. Figure B.12. The IDE changes during execution to provide runtime information. 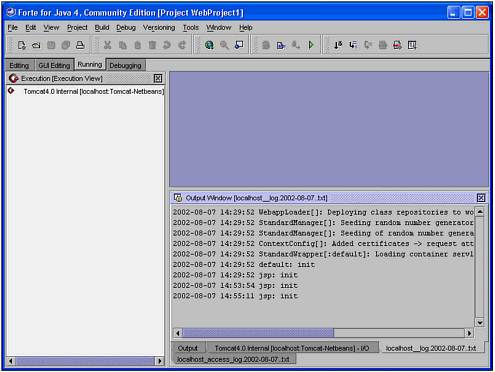 The default display in the output window changes to the localhost log. Each time you run the JSP page, a new entry is placed in this log. Adding a Servlet Creating a servlet with Sun ONE Studio 4 is similar to creating a JSP. You start out by right-clicking the project name and choosing Add New. When the New Wizard comes up, you choose to add a servlet and click the Next button. When the Target Location dialog appears, enter FirstServlet in the name field. The location of the file must be in the WEB-INF\Classes directory, according to the Java Servlet Specification Version 2.3. Figure B.13 shows this dialog. Figure B.13. The location of servlet files is different from the location of JSP files. 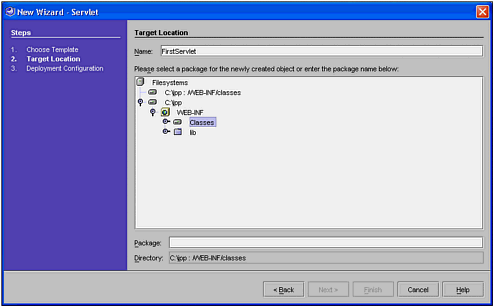 Click the Finish button to generate the servlet. We need to change the servlet contents slightly so that it will generate some visible output. We do this by removing the comments surrounding the out.println() method calls in the processRequest() method. In addition, we need to add the following line under the out.println() statement that adds the <body> tag to the output: out.println("This is our first Servlet"); Listing B.3 shows the full listing of this servlet. Listing B.3 The FirstServlet.java File /* * FirstServlet.java * * Created on August 7, 2002, 5:30 PM */ import javax.servlet.*; import javax.servlet.http.*; /** * * @author Stephen Potts * @version */ public class FirstServlet extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Processes requests for both HTTP <code>GET</code> * and <code>POST</code> methods. * @param request servlet request * @param response servlet response */ protected void processRequest(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { response.setContentType("text/html"); java.io.PrintWriter out = response.getWriter(); // output your page here out.println("<html>"); out.println("<head>"); out.println("<title>Servlet</title>"); out.println("</head>"); out.println("<body>"); out.println("This is our first Servlet"); out.println("</body>"); out.println("</html>"); out.close(); } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { processRequest(request, response); } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { processRequest(request, response); } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } See Chapter 21, "Servlets," for an explanation of what each of the elements in the servlet adds to the total. We can run this servlet by choosing Execute from the Build menu. Running this application starts a browser and displays the result, as shown in Figure B.14. Figure B.14. The servlet results are displayed in a browser also. 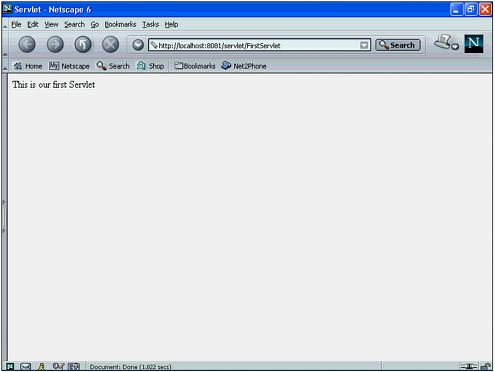 You can set breakpoints and watchpoints in servlets just like you can in Java applications. You must choose Start from the Debug menu if you want them to actually pause your program. |