You can't rapidly understand a method's logic. Transform the logic into a small number of intention-revealing steps at the same level of detail. 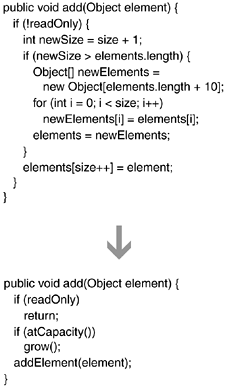 Motivation Kent Beck once said that some of his best patterns are those that he thought someone would laugh at him for writing. Composed Method [Beck, SBPP] may be such a pattern. A Composed Method is a small, simple method that you can understand in seconds. Do you write a lot of Composed Methods? I like to think I do, but I often find that I don't, at first. So I have to go back and refactor to this pattern. When my code has many Composed Methods, it tends to be easy to use, read, and extend. A Composed Method is composed of calls to other methods. Good Composed Methods have code at the same level of detail. For example, the code set in bold in the following listing is not at the same level of detail as the nonbold code: private void paintCard(Graphics g) { Image image = null; if (card.getType().equals("Problem")) { image = explanations.getGameUI().problem; } else if (card.getType().equals("Solution")) { image = explanations.getGameUI().solution; } else if (card.getType().equals("Value")) { image = explanations.getGameUI().value; } g.drawImage(image,0,0,explanations.getGameUI()); if (shouldHighlight()) paintCardHighlight(g); paintCardText(g); } By refactoring to a Composed Method, all of the methods called within the paintCard() method are now at the same level of detail: private void paintCard(Graphics g) { paintCardImage(g); if (shouldHighlight()) paintCardHighlight(g); paintCardText(g); } Most refactorings to Composed Method involve applying Extract Method [F] several times until the Composed Method does most (if not all) of its work via calls to other methods. The most difficult part is deciding what code to include or not include in an extracted method. If you extract too much code into a method, you'll have a hard time naming the method to adequately describe what it does. In that case, just apply Inline Method [F] to get the code back into the original method, and then explore other ways to break it up. Once you finish this refactoring, you will likely have numerous small, private methods that are called by your Composed Method. Some may consider such small methods to be a performance problem. They are only a performance problem when a profiler says they are. I rarely find that my worst performance problems relate to Composed Methods; they almost always relate to other coding problems. If you apply this refactoring on numerous methods within the same class, you may find that the class has an overabundance of small, private methods. In that case, you may see an opportunity to apply Extract Class [F]. Another possible downside of this refactoring involves debugging. If you debug a Composed Method, it can become difficult to find where the actual work gets done because the logic is spread out across many small methods. A Composed Method's name communicates what it does, while its body communicates how it does what it does. This allows you to rapidly comprehend the code in a Composed Method. When you add up all the time you and your team spend trying to understand a system's code, you can just imagine how much more efficient and effective you'll be if the system is composed of many Composed Methods. Benefits and Liabilities + | Efficiently communicates what a method does and how it does what it does. | + | Simplifies a method by breaking it up into well-named chunks of behavior at the same level of detail. | | Can lead to an overabundance of small methods. | | Can make debugging difficult because logic is spread out across many small methods. | | Mechanics This is one of the most important refactorings I know. Conceptually, it is also one of the simplestso you'd think that this refactoring would lead to a simple set of mechanics. In fact, it's just the opposite. While the steps themselves aren't complex, there is no simple, repeatable set of steps. Instead, there are guidelines for refactoring to Composed Method, some of which include the following. - Think small: Composed Methods are rarely more than ten lines of code and are usually about five lines.
- Remove duplication and dead code: Reduce the amount of code in the method by getting rid of blatant and/or subtle code duplication or code that isn't being used.
- Communicate intention: Name your variables, methods, and parameters clearly so they communicate their purposes (e.g., public void addChildTo(Node parent)).
- Simplify: Transform your code so it's as simple as possible. Do this by questioning how you've coded something and by experimenting with alternatives.
- Use the same level of detail: When you break up one method into chunks of behavior, make the chunks operate at similar levels of detail. For example, if you have a piece of detailed conditional logic mixed in with some high-level method calls, you have code at different levels of detail. Push the detail down into a well-named method, at the same level of detail as the other methods in the Composed Method.
Example This example comes from a custom-written collections library. A List class contains an add(…) method by which a user can add an object to a List instance: public class List... public void add(Object element) { if (!readOnly) { int newSize = size + 1; if (newSize > elements.length) { Object[] newElements = new Object[elements.length + 10]; for (int i = 0; i < size; i++) newElements[i] = elements[i]; elements = newElements; } elements[size++] = element; } } The first thing I want to change about this 11-line method is its first conditional statement. Instead of using a conditional to wrap all of the method's code, I'd rather see the condition used as a guard clause, by which we can make an early exit from the method: public class List... public void add(Object element) { if (readOnly) return; int newSize = size + 1; if (newSize > elements.length) { Object[] newElements = new Object[elements.length + 10]; for (int i = 0; i < size; i++) newElements[i] = elements[i]; elements = newElements; } elements[size++] = element; } Next, I study the code in the middle of the method. This code checks to see whether the size of the elements array will exceed capacity if a new object is added. If capacity will be exceeded, the elements array is expanded by a factor of 10. The magic number 10 doesn't communicate very well at all. I change it to be a constant: public class List... private final static int GROWTH_INCREMENT = 10; public void add(Object element)... ... Object[] newElements = new Object[elements.length + GROWTH_INCREMENT]; ... Next, I apply Extract Method [F] on the code that checks whether the elements array is at capacity and needs to grow. This leads to the following code: public class List... public void add(Object element) { if (readOnly) return; if (atCapacity()) { Object[] newElements = new Object[elements.length + GROWTH_INCREMENT]; for (int i = 0; i < size; i++) newElements[i] = elements[i]; elements = newElements; } elements[size++] = element; } private boolean atCapacity() { return (size + 1) > elements.length; } Next, I apply Extract Method [F] on the code that grows the size of the elements array: public class List... public void add(Object element) { if (readOnly) return; if (atCapacity()) grow(); elements[size++] = element; } private void grow() { Object[] newElements = new Object[elements.length + GROWTH_INCREMENT]; for (int i = 0; i < size; i++) newElements[i] = elements[i]; elements = newElements; } Finally, I focus on the last line of the method: elements[size++] = element; Although this is one line of code, it is not at the same level of detail as the rest of the method. I fix this by extracting this code into its own method: public class List... public void add(Object element) { if (readOnly) return; if (atCapacity()) grow(); addElement(element); } private void addElement(Object element) { elements[size++] = element; } The add(…) method now contains only five lines of code. Before this refactoring, it would take a little time to understand what the method was doing. After this refactoring, I can rapidly understand what the method does in one second. This is a typical result of applying Compose Method. |