Remoting enables the communication between the client and the server by creating proxy objects, as shown in Figure 4.1. Figure 4.1. Simplified view of .NET remoting. 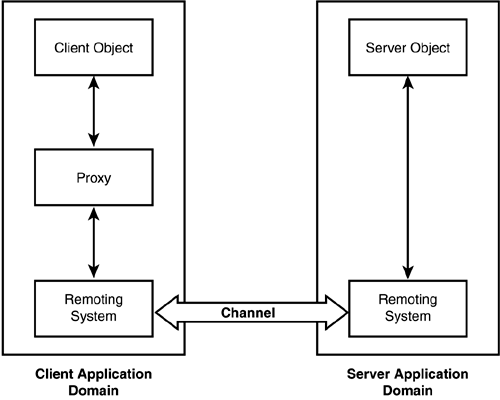 The client and server communicate by using the following steps: When a client object requests an instance of the server object, the remoting system at the client side creates a proxy of the server object. The proxy object behaves just like the remote object; this leaves the client with the impression that the server object is in the client's process. When the client object calls a method on the server object, the proxy passes the call information to the remoting system on the client. This remoting system, in turn, sends the call over the channel to the remoting system on the server. The remoting system on the server receives the call information and, on the basis of this information, invokes the method on the actual object on the server (creating the object, if necessary). The remoting system on the server collects the result of the method invocation and passes it through the channel to the remoting system on the client. The remoting system at the client receives the server's response and returns the results to the client object through the proxy. Object Marshaling Object marshaling specifies how a remote object is exposed to the client applications. The .NET remoting framework enables you to marshal the objects in the following two ways: Marshal-by-value (MBV) objects These objects are copied and passed out of the server application domain to the client application domain. Marshal-by-reference (MBR) objects A proxy is used to access these objects on the client side. The clients hold just a reference to these objects. Marshal-by-Value Objects MBV objects reside on the server. When a client invokes a method on the MBV object, the MBV object is serialized, transferred over the network, and restored on the client as an exact copy of the server-side object. Now, the MBV object is locally available; therefore, any method calls to the object do not require any proxy object or marshaling. The MBV objects can provide faster performance by reducing the network roundtrips, but in the case of large objects, the time taken to transfer the serialized object from the server to the client can be very significant. Furthermore, the MBV objects do not provide the privilege of running the remote object in the server environment. You can create an MBV object by declaring a class with the Serializable attribute. For example: [Serializable()] public class MyMBVObject { //... } If a class needs to control its own serialization, it can do so by implementing the ISerializable interface. Marshal-by-Reference Objects The MBR objects are remote objects. They always reside on the server, and all methods invoked on these objects are executed on the server side. The client communicates with the MBR object on the server by using a local proxy object that holds the reference to the MBR object. MBR objects are a likely choice when the objects are prohibitively large or when the functionality of the object is available only in the server environment on which it is created. You can create an MBR object by deriving the MBR class from the System.MarshalByRefObject class. For example: public class MyMBRObject : MarshalByRefObject { //... } Channels When a client calls a method on the remote object, the details of the method call are transported to and from the remote object through a channel. The channel object at the receiving end of a channel (the server channel) listens for messages by using a particular protocol on a specified port number. The channel object at the sending end of the channel (the client channel) sends information to the receiving end by using the same protocol and port number. The .NET Framework provides implementations for the HTTP channel through the classes of the System.Runtime.Remoting.Channels.Http namespace and provides implementation for the Transmission Control Protocol (TCP) channel through the classes of the System.Runtime.Remoting.Channels.Tcp namespace. If you want to use a different protocol, you can define your own channel by implementing the IChannelReceiver and IChannelSender interfaces. The following code example shows how to register a sender-receiver HTTP channel on port 1234: using System; using System.Runtime.Remoting.Channels; using System.Runtime.Remoting.Channels.Http; //... HttpChannel channel = new HttpChannel(1234); ChannelServices.RegisterChannel(channel); //... The following code example shows how to register a sender-receiver TCP channel on port 1234: using System; using System.Runtime.Remoting.Channels; using System.Runtime.Remoting.Channels.Tcp; //... TcpChannel channel = new TcpChannel(1234); ChannelServices.RegisterChannel(channel) ; //... Formatters Before the messages are sent over a channel, they need to be encoded and serialized; similarly messages need to be decoded and deserialized at the receiving end. Formatters are the objects that encode, decode, serialize, and deserialize messages. The .NET Framework packages two formatter classes for common scenarios. The SOAP formatter is implemented in the SoapFormatter class of the System.Runtime.Serialization.Formatters.Soap namespace. The binary formatter is implemented in the BinaryFormatter class of the System.Runtime.Serialization.Formatters.Binary namespace. If you want to use a different formatter, you can define your own formatter class by implementing the IFormatter interface. The HTTP channel uses the SOAP formatter as its default formatter. The TCP channel uses the binary formatter as its default formatter. However, channels are configurable. You can configure the HTTP channel to use the binary formatter or a custom formatter rather than the SOAP formatter. Similarly, the TCP channel can be configured to use the SOAP formatter or a custom formatter rather than the binary formatter. Figure 4.2 compares the various combinations of channels and formatters on the scale of efficiency and compatibility. Figure 4.2. Selecting a combination of channel and formatter. 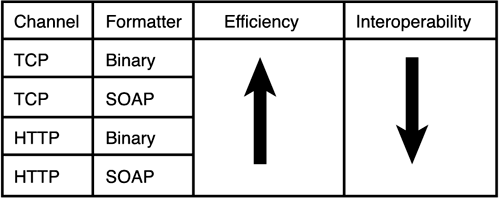 Remote Object Activation Only MBR objects can be activated remotely. No remote activation is needed in the case of MBV objects because the MBV object itself is transferred to the client side. Two types of MBR objects exist: server-activated objects (SAO) and client-activated objects (CAO). Server-activated objects (SAOs) are remote objects whose lifetime is directly controlled by the server. There are two possible activation modes for a server-activated object: SingleCall activation mode and Singleton activation mode. In the SingleCall activation mode, an object is instantiated for the sole purpose of responding to just one client request. After the request is fulfilled, the .NET remoting framework deletes the object and reclaims its memory. Objects created in SingleCall mode are inherently stateless. This behavior of the SingleCall mode accounts for greater server scalability because an object consumes server resources for only a small period, thereby allowing the server to allocate resources to other objects. 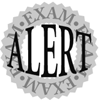 | Because the SingleCall objects are stateless, it does not matter which server processes their requests. For this reason, SingleCall activation is ideally suited for load-balanced environments. |
In Singleton activation mode, there is, at most, one instance of the remote object, regardless of the number of clients accessing it. A Singleton mode object can maintain state across the method calls, and this state is globally shared by all its clients. Singleton objects are a desired solution when the overhead of creating an object is substantial, when state is required, or when the clients need to share state. Client-activated objects (CAOs) are remote objects whose lifetime is directly controlled by the client. Client-activated objects are instantiated on the server as soon as the client requests the object to be created. Unlike SAOs, CAOs do not delay object creation until the first method is called on the object. An instance of the CAO serves only the client that was responsible for its creation, and the CAO doesn't get discarded with each request. For this reason, a CAO can maintain state with each client that it is serving. However, unlike Singleton SAOs, different CAOs cannot share a common state. CAOs are the desired solution when the clients want to maintain a private session or want to have more control over how the objects are created and how long they will live. Figure 4.3 compares the various object-activation techniques. Figure 4.3. SingleCall server activation offers maximum scalability, whereas client activation offers maximum flexibility. 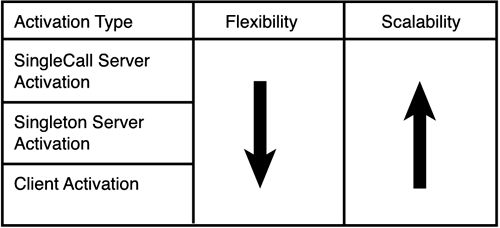 Lifetime Leases A lifetime lease is the period of time that a particular object shall be active in memory before the .NET Framework deletes it and reclaims its memory. Both Singleton SAOs and CAOs use lifetime leases to determine how long they should continue to exist. A lifetime lease is represented by an object that implements the ILease interface that is defined in the System.Runtime.Remoting.Lifetime namespace. 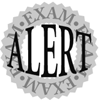 | A remote object can choose to have a custom-defined lifetime if the InitializeLifetimeService() method of the base class, MarshalByRefObject, is overridden. If the InitializeLifetimeService() method returns null, the type tells the .NET remoting system that its instances are intended to have an infinite lifetime. |
Simply speaking, the lease works as follows: When an object is created, its lifetime lease (CurrentLeaseTime) is set using the InitialLeaseTime property (which is 5 minutes, by default). Whenever the object receives a call, its CurrentLeaseTime is increased by the time specified by the value of RenewOnCallTime property (which is 2 minutes, by default). The client can also renew a lease for a remote object by directly calling the ILease.Renew() method: ILease lease = (ILease) RemotingServices. GetLifetimeService(RemoteObject); TimeSpan expireTime = lease.Renew(TimeSpan.FromSeconds(60)); When the value of CurrentLeaseTime reaches 0, the .NET Framework contacts any sponsors registered with the lease to check whether they are ready to sponsor a renewal of the lease of the object. If the sponsor does not renew the object or if the server cannot contact the sponsor within the duration specified by the SponsorshipTimeout property, the object is marked for garbage collection. Sponsors are the objects responsible for dynamically renewing an object's lease if its lease expires. For more information about sponsors, refer to the "Renewing Leases" section in the .NET Framework Developer's Guide. |