The shell is your most powerful window into the world of a Unix system. Whether you're running it in an X Window System window or through a text-based dumb terminal, the shell gives you full access to a Unix system's many powerful command-line tools, configuration files, and devices. Code listing 1.1. Using the pwd command and PWD environment variable to find your current directory. bender:~ chrish$ pwd /Users/chrish bender:~ chrish$ echo $PWD /Users/chrish Moving around and seeing what's there When you first log in to a Unix system, whether it's via GUI, Telnet, or SSH connection, your current directory will be your account's home directory. To find your current directory Do either of the following: pwd When you first log in, type the pwd (presentworking directory ) command. Unless you've got a strange shell startup script that changes your directory, your home directory will be displayed (Code Listing 1.1): bender:~ chrish$ pwd /Users/chrish
echo $PWD The $PWD environment variable displays the current working directory: bender:~ chrish$ echo $PWD /Users/chrish
Environment variables are easier to use in complex command sequences, as well as in shell scripts, because you can use $PWD anywhere in a command without any extra work. From your current directory, you can get back to your home directory. To get back home Code listing 1.2. Getting back to your home directory using the HOME environment variable or the ~ shortcut. bender:/tmp chrish$ pwd /tmp bender:/tmp chrish$ cd $HOME bender:~ chrish$ pwd /Users/chrish bender:~ chrish$ cd /etc bender:/etc chrish$ pwd /etc bender:/etc chrish$ cd ~ bender:~ chrish$ pwd /Users/chrish Do either of the following: cd $HOME Just as the PWD environment variable always contains your current directory, the HOME environment variable always has your home directory inside. cd ~ Want to get home with the least amount of typing? The ~ (tilde) shortcut also refers to your home directory. Unix developers love shortcuts (Code Listing 1.2)! Tips In some shells, typing cd without an argument will also return you to your home directory. You can use the HOME environment variable or the ~ shortcut in any argument that uses a path or filename: cat ~/my-file.txt cp $HOME/my-file.txt $HOME/my-file-backup.txt
The ~ shortcut also lets you refer to other people's home directories by including their login name immediately after the tilde. For example, this command copies my-file.txt to the user david's home directory: cp ~/my-file.txt ~david/your-file.txt
So now that we know where we are, what files are there? The ls command holds the answer. You have several options. To list the files in a directory Do any of the following: ls The ls command will show you which files are in your current directory (Code Listing 1.3): bender:~ chrish$ ls Default Documents Movies Pictures Sites emacs-lisp Desktop Library Music Public daily-urls.html
ls -F The -F option adds / to the end of directory names, * to executables, and @ to symbolic links. This makes it easier to find specific things in the file system. bender:~ chrish$ ls -F Default Documents/ Movies/ Pictures/ Sites/ emacs-lisp/ Desktop/ Library/ Music/ Public/ daily-urls.html
ls -a Unix hides configuration files and directories by starting them with a . (period) character. These dotfiles are hidden by convention; Unix applications ignore them and usually only show them if you specifically ask them to. The ls command's -a option tells it to show all files, including the "hidden" dotfiles. Code listing 1.3. Listing files with the ls command and its -F (add file-type decorations) and -a (show all files) options. bender:~ chrish$ ls Default Documents Movies Pictures Sites emacs-lisp Desktop Library Music Public daily-urls.html bender:~ chrish$ ls -F Default Documents/ Movies/ Pictures/ Sites/ emacs-lisp/ Desktop/ Library/ Music/ Public/ daily-urls.html bender:~ chrish$ ls -a . .bash_history .lpoptions Desktop Public .. .cvspass .ncftp Documents Sites .CFUserTextEncoding .emacs .profile Library daily-urls.html .DS_Store .emacs.d .ssh Movies emacs-lisp .MacOSX .irssi .viminfo Music .Trash .lftp Default Pictures bender:~ chrish$ ls -aF ./ .bash_history .lpoptions Desktop/ Public/ ../ .cvspass .ncftp/ Documents/ Sites/ .CFUserTextEncoding .emacs .profile Library/ daily-urls.html .DS_Store .emacs.d/ .ssh/ Movies/ emacs-lisp/ .MacOSX/ .irssi/ .viminfo Music/ .Trash/ .lftp/ Default Pictures/ Now that we've found some files, we probably want to see what's in them. Again, there are several options. To look at a file's contents Do any of the following: cat filename Now that you've used your cd and ls expertise to find a file you're interested in, cat will print the contents of a file to the terminal (Code Listing 1.4). head filename Only want to see the beginning of the file? Use the head command instead (see Code Listing 1.4). tail filename You can also use the tail command (see Code Listing 1.4) to see just the end of a file. less filename Usually you'll want to use the less command (Figure 1.1) so that you can interactively page through or search the file. Figure 1.1. The less command lets you view a file one screen at a time. 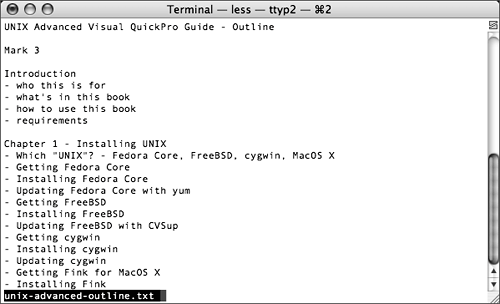
more filename If less isn't available for some reason, its older, utilitarian brother more can help you out. Tip Sometimes if you cat a binary file (such as a program, an image, or a sound file), it'll screw up your terminal's settings. This could result in strange keyboard behavior, invisible text, and so on. To fix it, carefully type this command and press Enter: stty sane
Code listing 1.4. Looking at a file's contents with cat, head, and tail. chrish@taffer [508]: cat unix-advanced-outline.txt UNIX Advanced Visual QuickPro Guide - Outline Introduction - who this is for - what's in this book - how to use this book - requirements . . . - Apache forums, etc. - MySQL forums, etc. - PHP forums, etc. chrish@taffer [509]: head unix-advanced-outline.txt UNIX Advanced Visual QuickPro Guide - Outline Introduction - who this is for - what's in this book - how to use this book - requirements Chapter 1 - Installing UNIX - Which "UNIX"? - Fedora Core, FreeBSD, cygwin, MacOS X chrish@taffer [510]: tail unix-advanced-outline.txt Appendix A - Finding out more - how to find out more - man, info (and pinfo), Google, mailing lists, RFCs - Fedora Core forums, mailing lists, etc. - FreeBSD forums, mailing lists, etc. - cygwin forums, mailing lists, etc. - fink forums, mailing lists, etc. - system administration mailing lists (risks-l, CERT, etc.) - Apache forums, etc. - MySQL forums, etc. - PHP forums, etc. Redirecting input and output Because so many Unix utilities operate on text files, it can be very useful to redirect a program's output to a file for later processing by another application. You can even use a file as input to a program. Code listing 1.5. Redirecting output to a file with > and input from a file with <. chrish@taffer [506]: ls chrish@taffer [507]: ps > ps.txt chrish@taffer [508]: ls ps.txt chrish@taffer [509]: sort < ps.txt > ps-sorted.txt chrish@taffer [510]: ls ps-sorted.txt ps.txt To send a command's output to a file command > filename You can redirect a program's output to the specified file using the > character (Code Listing 1.5): chrish@taffer [508]: ps > ps.txt chrish@taffer [509]: ls ps.txt
The ps.txt file now contains the output of the ps command. Code listing 1.6. Using >> to append to an existing file. chrish@taffer [513]: echo "hello" > hello-there.txt chrish@taffer [514]: ls hello-there.txt chrish@taffer [515]: echo "there" > hello-there.txt chrish@taffer [516]: cat hello-there.txt there chrish@taffer [517]: echo "hello" > hello-there.txt chrish@taffer [518]: echo "there" >> hello-there.txt chrish@taffer [519]: cat hello-there.txt hello there To append a command's output to a file command >> filename Use the >> redirection (Code Listing 1.6) to append a program's output to an existing file instead of just overwriting it: chrish@taffer [520]: echo hello > hello-there.txt chrish@taffer [521]: echo there >> hello-there.txt chrish@taffer [522]: cat hello-there.txt hello there
To get a command's input from a file command < filename Use the < character to redirect a program's input from the specified file (Code Listing 1.5): chrish@taffer [510]: sort < ps.txt > ps-sorted.txt
As you can see, you can also combine the two redirections on one command line. In the example above, the output of the sort command, which is operating on the data in ps.txt, is stored in ps-sorted.txt. Streams Unix has three standard text streams automatically associated with every program: stdin (standard input), stdout (standard output), and stderr (standard error). While programmers can refer to these streams by name, shell users have to refer to them by number: 0 stdin 1 stdout 2 stderr As we've seen, < redirects from stdin and > redirects the stdout stream, but what about stderr? That's an output stream for errors, but we can't redirect it with > because it's already taken by stdout. By specifying the stream with the redirection, we can send stderr to a file as well: chrish@taffer [511]: sort < ps.txt > ps-sorted.txt 2> sort-errors.txt This sorts the contents of ps.txt and saves the output to ps-sorted.txt. Any error messages will go to sort-errors.txt. What if you wanted stdout and stderr in the same file? There's another special redirection for just such an occasion: chrish@taffer [523]: sort < ps.txt > ps-sorted.txt 2>&1 You can think of the & as tying the error stream to the output stream for this command. |
Plumbing Code listing 1.7. Piping the output of one command into another lets you create your own powerful chain of tools. bender:~ chrish$ ps PID TT STAT TIME COMMAND 1853 p1 S 0:00.42 -bash 2168 p1 S+ 0:00.65 ssh taffer 1875 std S 0:00.51 -bash bender:~ chrish$ ps | sort PID TT STAT TIME COMMAND 1853 p1 S 0:00.42 -bash 1875 std S 0:00.51 -bash 2168 p1 S+ 0:00.65 ssh taffer 2175 std R+ 0:00.00 sort Being able to chain together a series of commands without having to use intermediate files is a very powerful feature. It goes hand-in-hand with another Unix design philosophy: providing a set of small, well-defined tools that work together. To use a program's output as input command | command Instead of redirecting the output from ps to a file and then sorting it, we could combine the two commands (Code Listing 1.7) using |, the pipe: chrish@taffer [524]: ps | sort > ps-sorted.txt
That's exactly the same as this sequence, but without the temporary file: chrish@taffer [525]: ps > ps.txt chrish@taffer [526]: sort < ps.txt > ps-sorted.txt
This gets more powerful as you chain together more and more commands. tee for Two If you're nosy like me, you'd probably prefer that commands show progress while redirecting their output, especially when they take a long time to finish. Luckily, the tee command takes its input, saves it to a file, and then prints it to the terminal. For example, the following command, chrish@taffer [530]: ps | tee ps.txt will write the output of ps to ps.txt and also display it onscreen. This gets really handy when you're using tee to watch something that runs forever (like a Web server) or that might take a long time (like a program compile). |
|