Finding information within your database can be a simple or a complex process. Depending on the search criteria, you can give your users the capability to narrow down their searches as fine as a specific date range. Suppose you wanted to find all customers within your database that had a last name of Smith; you could create a simple query within Access that selected all the records within the customers table whose last name was equivalent to the name Smith. Your SQL statement could look similar to SELECT LastName FROM Customers WHERE LastName = 'Smith' The result would return all the matching records. Indeed, the database is filled with information that users may want access to including the following: Customers As an admin, you could perform a search within your customers table to extract customer-specific information including last name, first name, city, state, and so on. CreditCards Again, as an admin, you could perform a search within the credit cards table to extract all Visa credit cards. This could eventually enable you to create a statistical analysis of the credit card of choice for your customers. Products Probably the most important container of information, the products table could be searched by a user to determine whether a certain product is being sold through your company. Inventory A user could search the inventory table, which would return results along with the product search to guarantee that a specific item is in stock. Orders As an admin, you could perform a search to determine how many items you sold for a specific day, week, or month. You could also return a statistical analysis of those results so that you could better understand your customers' ordering habits and possibly suggest products in the future. As you can see, just within the Web Store database, there is plenty available to search on. Whether you are approaching the problem as an admin or a user, the database ultimately is a warehouse of information. How you choose to access that information is up to you. A Basic SQL-Based Search In Chapter 26, "Database Primer," you learned how to display dynamic data to the user. You created a query and ultimately integrated that query into your application using a server behavior that presented dynamic data. The problem with that approach is that it's hard-coded, meaning that the data the user would end up seeing would always remain the same. What if your users didn't want to see what you were presenting to them? Fortunately for you, you could allow your users to perform a search within your site based on criteria that they specify through the use of form objects, recordsets, and variables. Before we jump ahead, let's dissect a common approach to creating search functionality. Figure 30.1 shows the Amazon Web site and the search form you would use to request a book. Figure 30.1. Most Web sites employ some means of allowing their users to search for information.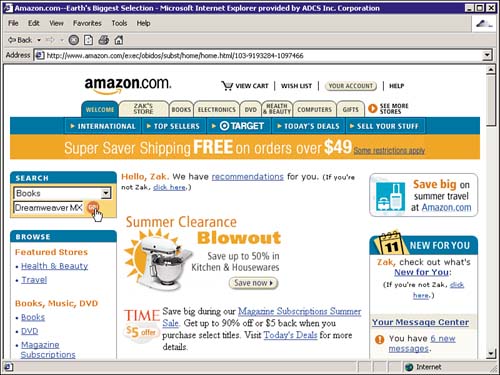 Consider now that you had a database with a list of book titles. The user of the Web site types in a book name; the value of that text box is dynamically appended to a WHERE or a LIKE clause within a SQL statement. The database is then queried and the results are presented to the user in a well-structured manner. You can create your own basic SQL-based search page by following the steps outlined next: NOTE If you have not done so already, create a new project folder within the wwwroot directory of the Inetpub folder. Within your project folder, create a database file to use with this section. Also, make sure that the necessary steps have been taken to define that site within Dreamweaver MX. -
Create a new page either within Notepad or Dreamweaver and enter the following HTML code: <html> <head> <title>Search Page</title> </head> <body> <form action="search_results.asp" method="post"> <input type="text" name="txtSearch"> <input type="submit" value="Submit"> </form> </body> </html> -
Save the page as search.asp inside a folder within the Inetpub\wwwroot directory. -
Create a new page for the search results named search_results.asp and enter the following code: <% Dim txtSearch, objConn, objRS, sqltext txtSearch = Request.Form("txtSearch") set objConn = Server.CreateObject("ADODB.Connection") set objRS = Server.CreateObject("ADODB.RecordSet") objConn.Open "Provider=Microsoft.Jet.OLEDB.4.0;" & _ "Data Source=C:\Inetpub\wwwroot\Search\WebStore.mdb;" & _ "Persist Security Info=False" sqltext = "SELECT * FROM Products WHERE ProductName=" &txtSearch objRS.Open sqltext, objConn, 3, 3 %> <html> <head> <title>Search Page</title> </head> <body> <%= objRS("ID") %><br> <%= objRS("ProductName") %> <%= objRS("ProductDescription") %> </body> </html> <% objRS.Close objConn.Close Set objConn = Nothing Set objRS = Nothing %> -
Save your work and test it in the browser. This simple application performs a search on the products table based on the ProductName that you specify within the search.asp page. WARNING Don't forget to place a copy of the Web Store database within the new folder that you created. This code assumes that you called that folder Search. Stepping over the code reveals that the only real change to the code lies in the SQL statement. Notice the SQL statement contains a WHERE clause with the value of the previous form object appended to it. Request.Form("txtSearch") grabs that value and places it into the txtSearch variable. NOTE The search pages form method was created using POST. You could change the method to GET and produce the same result. Rather than using Request.Form, you would use Request.QueryString. The downside is that the value is exposed within the querystring. Creating the Web Store Search Page Now that you have a basic understanding as to how tables are queried based on user input, let's take a look at how the search functionality can be integrated into the Web Store application. You can begin creating a search page following the steps outlined next: -
Create a new page by selecting New from the File menu. Select the Advanced tab and choose the WebStore template. -
Save the page as search.asp. -
Change the Header editable region to read Search and place a new form within the Content editable region. -
Insert a text box form object into the form named txtSearch. Point the action to the search_results.asp page, which will be created next. Select POST as the method. -
Insert a submit form object into the form named btnSearch. The result is shown in Figure 30.2. Figure 30.2. Create a new search page by adding the necessary form objects.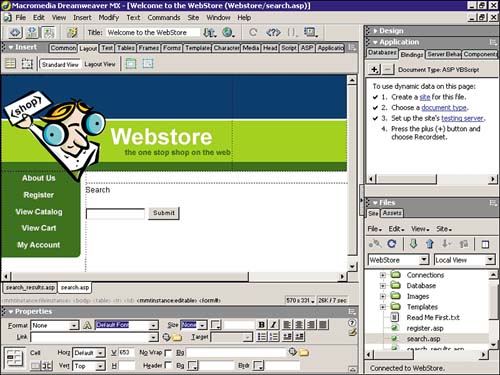 -
Save your work and test it in the browser. The result is shown in Figure 30.3. Figure 30.3. Place a value into the form and submit to send the value to the search results page.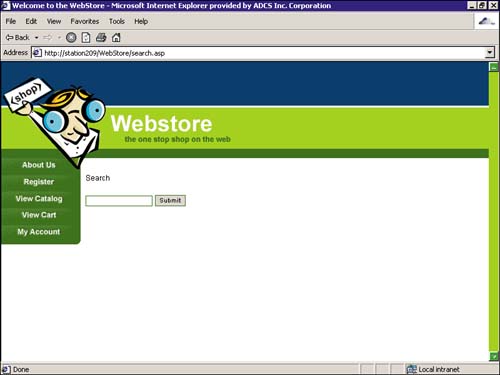 Creating the Web Store Search Results Page Now that the search page has been created, you'll want some way of collecting the value of the user input and actually processing the search. This page is where all the work is done. The search results page must contain the following components for the search to be processed correctly: Recordset of the table being searched on Proper variables to capture the users' input Dynamic text to display to the user the result of the search Remember, a recordset will always be used to capture the results of the table information. To create a new recordset for the search results page, follow the steps outlined next: -
Create a new page by selecting New from the File menu. Select the Advanced tab and choose the WebStore template. -
Save the page as search_results.asp. -
Select Recordset from the Bindings panel and switch to the advanced view. -
Name the recordset rsSearch and select the Web Store connection. -
You can build your SQL query either by typing the code into the box directly or by selecting the appropriate fields within the Database Items selection box, as shown in Figure 30.4. Figure 30.4. Create the query to extract a single record from the database.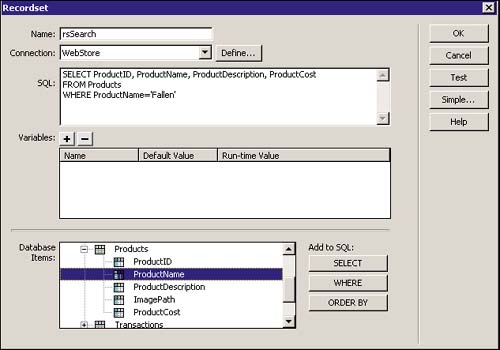 -
Notice that the value appended to the WHERE clause specifically looks for the DVD Fallen. -
Select Test. The results, as shown in Figure 30.5, show the data for the movie Fallen. Figure 30.5. The results for the movie Fallen are shown in the test dialog box.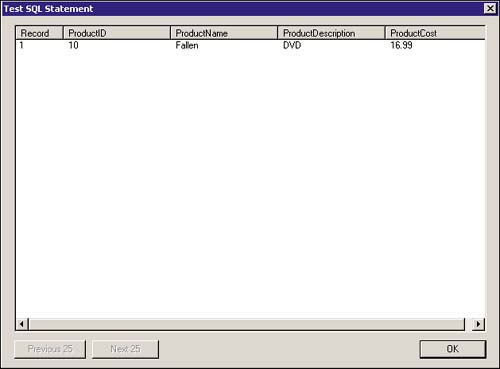 Although the query seems to work perfectly, it's still not dynamic to the user. The movie Fallen will always appear as the searched item. The next section will introduce you to working with variables, allowing you to capture the users' input, making the search much more dynamic. Working with Variables Up to this point, we have not discussed variables. Variables within Dreamweaver MX's Recordset dialog box allow you to capture user information from sessions, form requests, cookies, and application variables. They enable you to work dynamically, by passing values from one page to another. If you take the search page as an example, a user will submit the input via a form object to the search_results.asp page. Within the search_results.asp page, a piece of functionality needs to be added to allow for the capture of that input so that it can be automatically concatenated to the SQL statements' WHERE clause. That functionality is a variable. Variables within Dreamweaver enable you to capture the data being sent from a previous page and store it for later use. Variables contain three properties: Name The physical name of the variable Default Value A value to be assigned to the variable so that its value is never empty Runtime Value The value to assign the variable You can set up a variable to capture the user's input from the search page by following the steps outlined below: -
Open the rsSearch recordset. -
Add a variable to the variables list by selecting the plus (+) icon. The variable should contain the following properties: Name | Default Value | Runtime Value | Search | abc | Request.Form("txtSearch") | -
Remember, to capture requests made from a form object, you use request.form followed by the name of the form object within quotes. -
Modify the SQL statement so that you are using the keyword LIKE followed by the name of the variable, as shown in Figure 30.6. Figure 30.6. Add a variable and modify the SQL statement to accept the newly created variable name.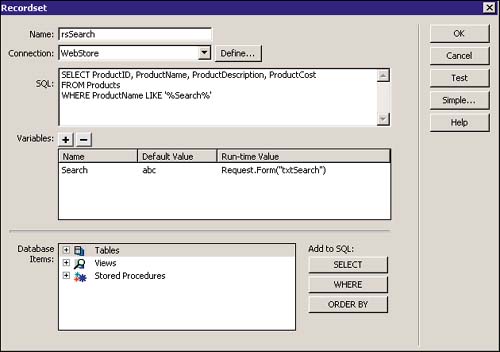 -
Select OK. Figure 30.7 shows that the recordset looks the same as it normally would without the addition of a variable. Figure 30.7. The recordset looks the same as it would without the variable addition.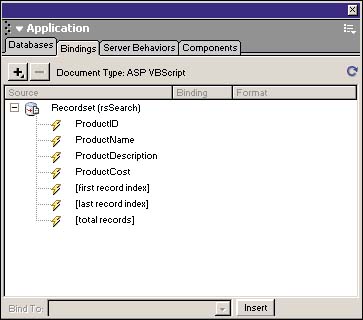 -
Continue to add all the dynamic text to the page, dragging the fields from the recordset onto their respective positions within the page. You may want to add captions for the field names. -
Drag the Total Records field into the header so users are aware of how many records the search produced. Figure 30.8 shows the result of the dynamic text. Figure 30.8. Add the fields from the recordset as dynamic text.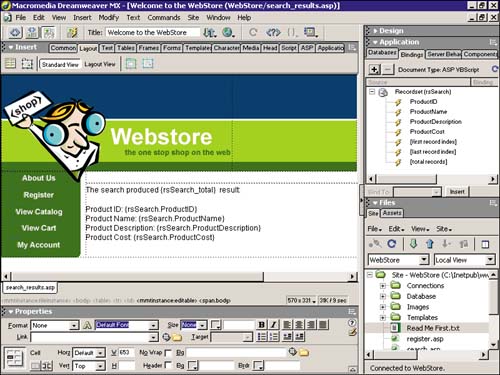 -
Save your work and test it in the browser. Figure 30.9 shows the result of the input Fallen. Figure 30.9. Fallen was searched for and the results came back with a match.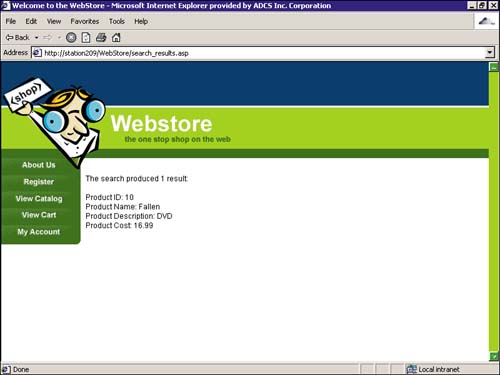 The results are made possible because of the variable. The variable captured the results of the request sent by the form's "post" submission. The variable was then appended to the SQL statement, which caused the dynamic search for the object's value. Repeating Regions Now that your application has search capabilities, you may want to allow the user to search for more than one item. For now, a user can search based on a DVD name. Fortunately for us, there's only one DVD that matches the name searched for. But what if the user decided to search based on the description of the DVD? If you look over the data that is contained within that field, you'll notice that the search can contain DVD, DVD Widescreen, and DVD Special Edition. You could easily create a repeating region that would effectively handle the situation by listing all the records that match that search criteria rather than one. To create a repeating region for your data, follow the steps outlined next: -
Add a line break after product cost and highlight the section of text including the dynamic data. -
Select Repeat Region from the Server Behavior panel. -
Figure 30.10 shows the dialog box that enables you to display all records within the recordset. Figure 30.10. Add a repeat region server behavior to the page.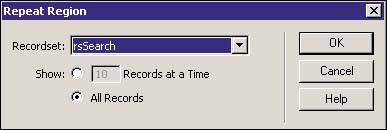 -
Next, open the recordset and change the WHERE clause to filter by ProductDescription, as shown in Figure 30.11. Figure 30.11. Change the recordset to filter by ProductDescription.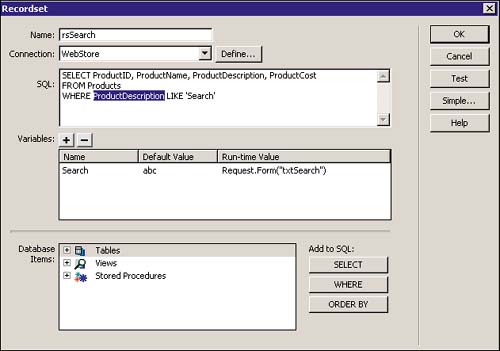 -
Select OK. -
Save your work and test it in the browser. Figure 30.12 shows the result of including DVD Special Edition as the search value in the search.asp page. Figure 30.12. The repeat region server behavior opens the door for your users by allowing them to search for data within the database that may have more than one result.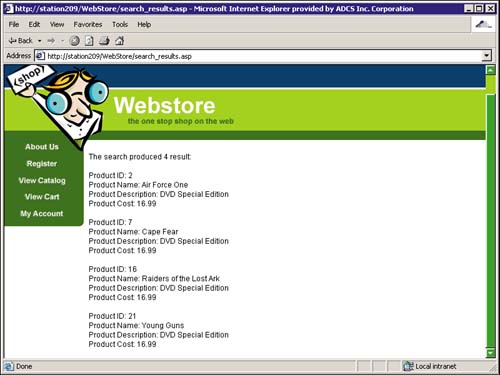 Alternative Text Currently, as users search for data within the database, they are presented with results in a structured format. But suppose users enter a value to search by and the recordset cannot return a match? Currently, the message returns The search produced 0 results. You can see how counterintuitive this message is to the user, not to mention that it isn't very user friendly. Using the Show Region server behavior set, you could present users with an alternative text message alerting them of the failed search result and allow them to link back to the search.asp page to try their search again. To insert the alternative text into your search result page, follow the steps outlined next: -
Insert a new line break just after the Show Region. -
Insert the text Sorry, no results match that search and create a link for Try Again. Make Try Again link back to the search.asp page. -
Highlight all the text and select the Show Region If Recordset Is Empty server behavior from the Show Region submenu, as shown in Figure 30.13. Figure 30.13. Insert the Show Region if Recordset Is Empty server behavior.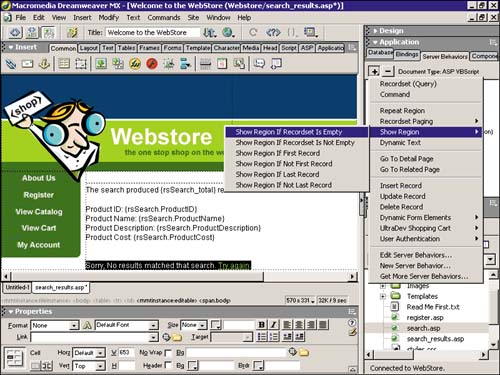 -
The Show Region dialog box will appear. Select the appropriate recordset (rsSearch) and click OK. -
Save your work and test it within the browser. -
In the search.asp page, type in something that you know it will not find, like VHS. Click Search. Your message will appear along with the link that allows you to link back to the search page, as shown in Figure 30.14. Figure 30.14. If the recordset comes up empty, you are presented with the custom message.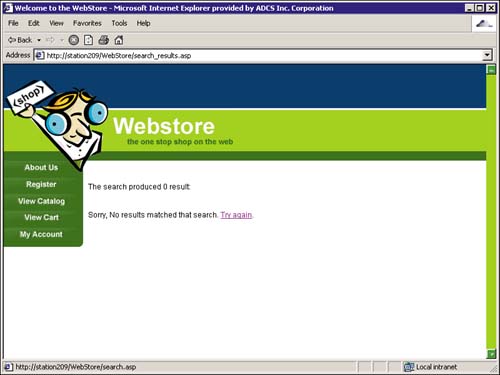 Linking to a Detail Page by Passing a Parameter Now that users know that the information they want exists within the database, you may want to add functionality that gives a little more detail about the product and allows them to purchase it. In the previous chapters you learned that a detail page is created to give more detail about a specific piece of data within your Web site. It doesn't have to be that simple; in fact, a detail page can be any page that you simply link to from a master page by passing a parameter. The detail page, in accordance with the parameter being passed either by the URL or by form post will change dynamically. In our search results example, you'll want to list the items that the user requested and provide the user with a way to link to that item within the viewcatalog.asp page, allowing them to purchase it. To create the detail page functionality, follow these steps: -
Select the dynamic text for ProductName and select the Go To Detail Page server behavior, as shown in Figure 30.15. Figure 30.15. Insert the Go To Detail Page server behavior.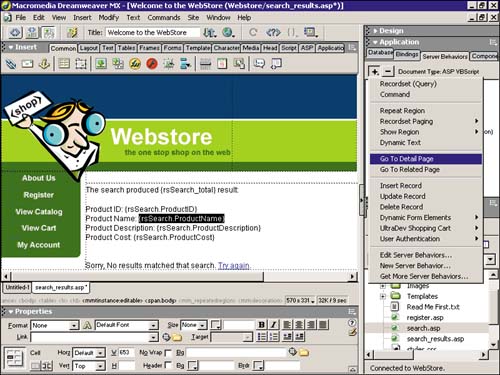 -
The Go To Detail Page dialog box appears. The dialog box allows you to select the item that you are creating the link for. In this case, because you already had the item selected, the field is populated for you. The dialog box also enables you to select the page that will serve as the detail page, the name of the parameter to pass, the recordset name, the field to use as the value to the parameter, and an option for how to send the parameter across. Figure 30.16 shows the configuration that you should be using. Figure 30.16. Apply all the appropriate settings to the Go To Detail Page dialog box.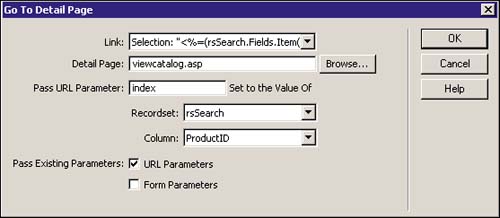 -
Select OK. -
Save your work and test it in the browser. Perform the same search that you did before. This time notice that the dynamic product names are linked. Figure 30.17 shows how you are able to select the product name. Figure 30.17. Select the product name to link to a detail page.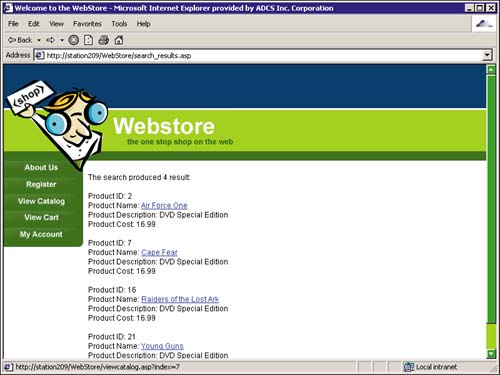 -
Select the link. Figure 30.18 shows how you are redirected to the appropriate page. Figure 30.18. The user is linked to the appropriate detail page.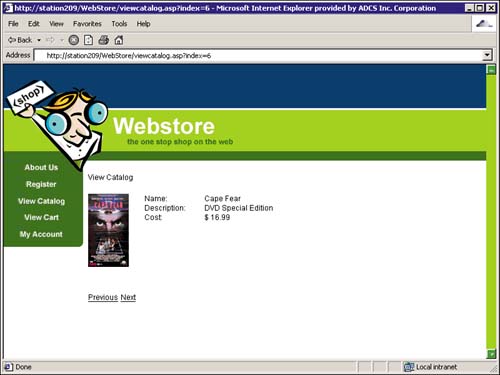 Globalizing the Search Functionality Now that most of the search functionality has been added to the Web store, you're ready to globalize it. What exactly does globalizing mean? Globalization is the term given to items or functionality that can be accessed from anywhere at any time. Currently, the search functionality can be accessed only from the search.asp page. What you want is for that functionality to be accessible from every page; that way, when a user has an immediate need to search for a particular item, it can be done no matter what page the user is on. Accomplishing this task is as simple as copying the information that currently resides within the search.asp page and integrating it into the template, allowing for every page within the Web store to use the search functionality. To globalize the search functionality, follow these steps: -
Open the template file that currently resides within the Templates folder. -
You can either copy the code that exists within the search.asp page or insert a new form, text field, and button, as shown in Figure 30.19. Align the objects to the right of the page. Figure 30.19. Insert the form objects into the top table row of the WebStore template.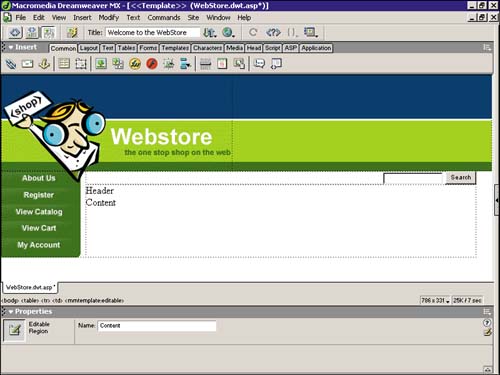 -
Select code/design view just to make sure all the code is formatted correctly. The result is shown in Figure 30.20. Figure 30.20. Format the code so that all the form objects are named appropriately and the form points to the search_results.asp page.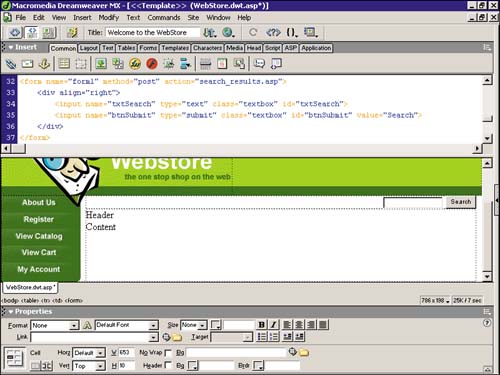 -
Save the page. You should be asked to update all the pages that use the template, as shown in Figure 30.21. Figure 30.21. The Update Template Files dialog box allows you to update all pages that use the master template.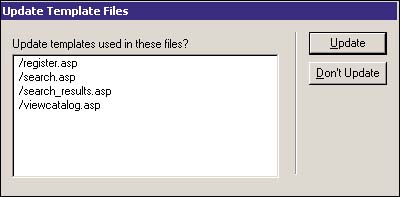 -
The Update Pages dialog box will appear, alerting you of the files that Dreamweaver MX has updated, as shown in Figure 30.22. Figure 30.22. The Update Pages dialog box alerts you of the files that have been updated.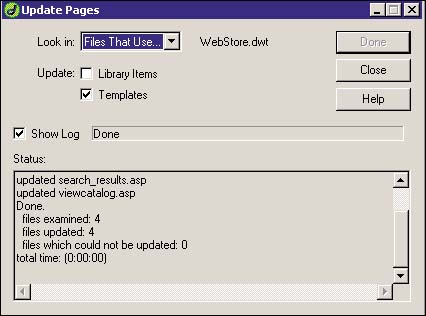 -
Select Close. -
Save your work and test it in the browser. Figure 30.23 shows that no matter what page you visit, the search will appear. Try typing in the value DVD Special Edition. Figure 30.23. The search form appears on every page.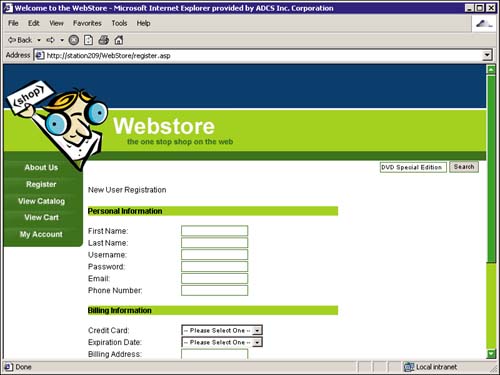 -
Select Search. The results should appear the same as they did from the search.asp page. Creating the Advanced Search Page The last step in creating a full-featured search engine within the Web store is to give the users as many options to select items within your Web application as possible. Currently a user must search using a keyword that currently exists within the database. Unfortunately, the user is able to select based on only one table within the database. Suppose the user wanted to select either DVD, DVD Widescreen, or DVD Special Edition from the ProductDescription field and choose a DVD name from the ProductName field. The current configuration would make it impossible. Just a few changes to the template and some modifications to the recordset will round out the search functionality for the Web Store application. Begin adding the advanced search functionality by following the steps outlined next: -
Open the template file from the Templates directory. -
Add a new link for Advanced Search and point it to search.asp, as shown in Figure 30.24. Figure 30.24. Add a new link for an advanced search.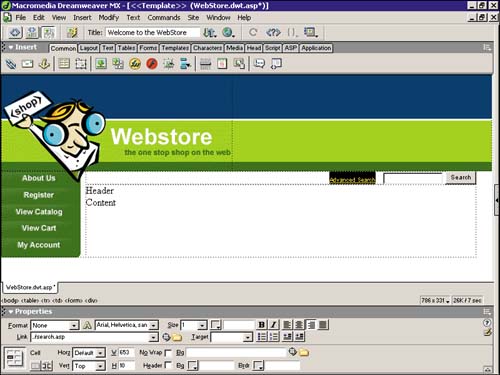 -
Save the template and update all files that use the template. -
Open search.asp. Change the header to Advanced Search and add a drop-down menu that will allow your users to search based on DVD, DVD Widescreen, and DVD Special Edition. The values are shown in Figure 30.25. Name the form object selType. Figure 30.25. Add list values for three separate items within the product description table.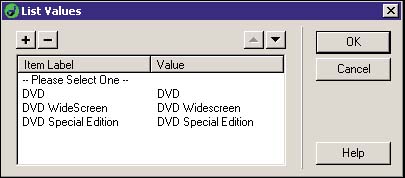 -
Figure 30.26 shows the result of adding the form values to the search.asp page. Figure 30.26. Add new form objects to handle the advanced search.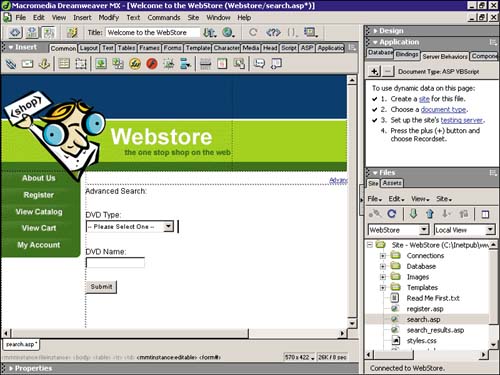 -
Save your work. Open search_results.asp. -
Open the recordset and make the changes as shown in Figure 30.27. Notice that a new variable was added to handle the request sent from the drop-down menu. Figure 30.27. Make the appropriate changes to the recordset.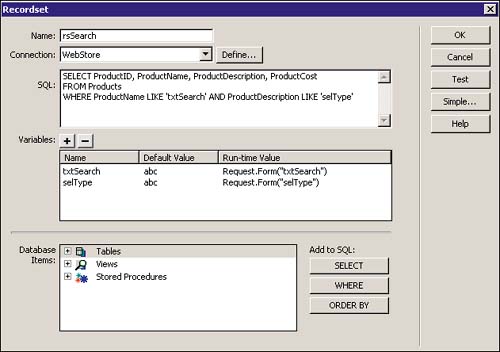 NOTE The keyword AND filters the search by two criteria: txtSearch will handle the product name and selType will handle which kind of DVD the item will be. Figure 30.28 shows an item being searched on from the search.asp page. Remember that this page can be accessed by linking from the Advanced Search link at the top of any page in the application. Figure 30.28. Enter values for the new search.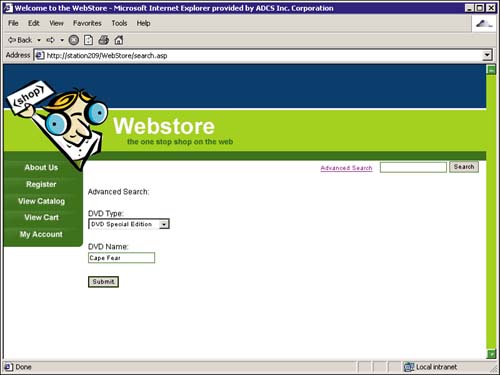 Figure 30.29 shows the results of the search. Figure 30.29. Cape Fear is the result of the search.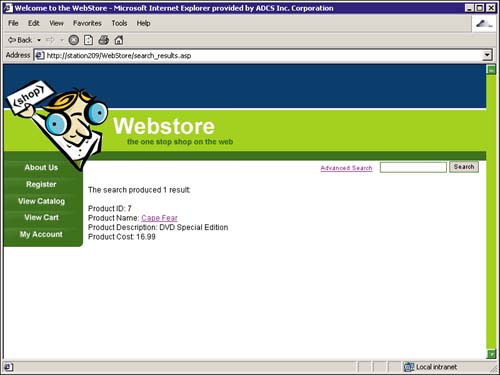 TIP You could narrow down the search results even further by using wildcards. A wildcard is signified by the "%" sign and allows for a keyword to be searched on that contains the letters that you specify. For instance, changing the SQL statement to read WHERE ProductName LIKE '%ll%' would return the results for Mallrats and Fallen because they both contain two l's. You can narrow your searches to be as complex as you want them; using wildcards is one way of accomplishing the task. Creating the Web Store Search Functionality Using ASP.NET Similar to the ASP server model, the search functionality can be created using ASP.NET. Unfortunately, with the ASP.NET server model comes a certain lack of functionality that will have to be hard coded in. For instance, using the ASP server model, you simply set the form action to point to the file that would do all the form processing. Because of PostBack, ASP.NET must be set up a bit differently. TIP PostBack refers to the process of submitting a form to itself. In a traditional ASP environment, a page is typically submitted to a second page that handles the processing for the first page. ASP.NET posts back to itself, handles the processing, and then uses a response.redirect to switch the user to a new page. Begin creating the ASP.NET search page by following the steps outlined next: -
Create a new page by selecting New from the File menu, switch to the Advanced tab, and select the WebStore template. WARNING For the page to be treated as an ASP.NET page by Dreamweaver MX, the document type must be set to ASP.NET VB/C#, and the testing server must be set up under an ASP.NET environment. -
Immediately save the page as search.aspx. Insert the text Search where the Header editable region is. -
Insert a new text box control as well as a button control where the Content editable region is. Because the Dreamweaver MX Properties Inspector will not recognize the objects, you will have to switch to code view and make the necessary changes, as shown in Figure 30.30. Be sure to name the controls appropriately. Figure 30.30. Create the new form and Web controls within the new page.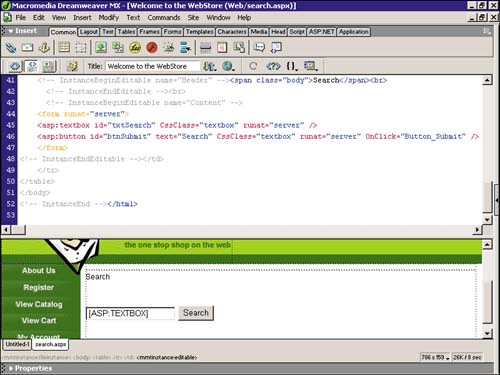 -
After you've created the new click event for the new button control, navigate to the top of the page and insert a new subroutine to handle the processing for the text box controls' input value. Notice how the text value of the text box control is concatenated to the URL within the response.redirect method, as shown in Figure 30.31. Figure 30.31. Create a new subroutine that redirects the users to a new page in the process, appending the value to the querystring as a new parameter.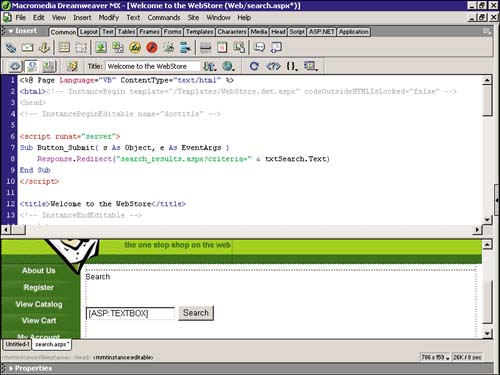 -
Save your work and close the page. -
Create a new page by selecting New from the File menu, switch to the Advanced tab, and select the WebStore template. -
Immediately save the page as search_results.aspx. Enter the text Results in the Header editable region. Create four new captions within the Content editable region, as shown in Figure 30.32. Eventually, these captions will display dynamic text. Figure 30.32. Insert captions to display what will eventually be dynamic text.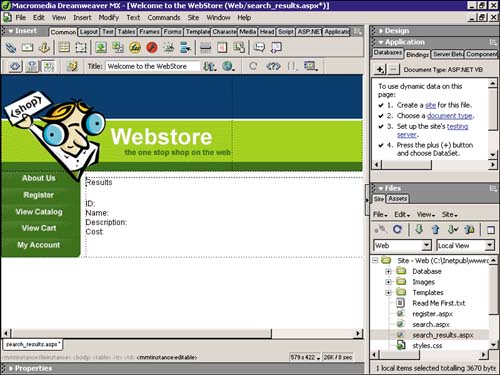 -
Insert a new DataSet by selecting it from the Bindings panel. Switch to advanced view and build the SQL statement either by using the database items or by manually typing it in. Figure 30.33 shows the result of building the SQL statement. Figure 30.33. Build a new SQL statement to handle the keyword search.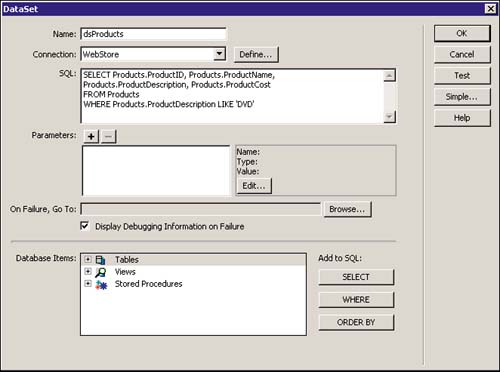 -
Notice the use of the LIKE keyword. In this case, assume that a user is performing a search based on the keyword "DVD." The SQL statement will look within the ProductDescription table for a word that is "like" DVD. Figure 30.34 shows the result of testing the SQL statement. Try testing the statement by changing the value to DVD Widescreen and then DVD Special Edition. The result should change every time. Figure 30.34. Test the results of the new query.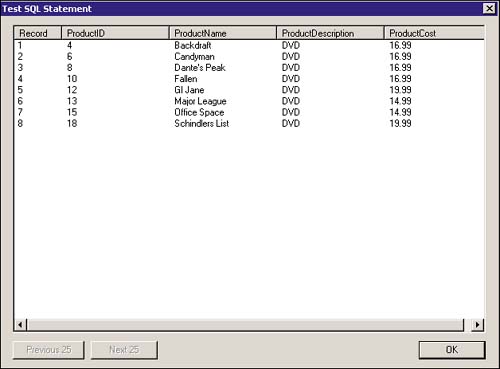 -
Select OK, close the DataSet, and save your work. Unfortunately, if you test the search page in the browser, your result will show little more than one record filtered by DVD. In the next section, you learn how to capture the value that is being sent across the querystring and dynamically concatenate it to the SQL statement as a parameter. Working with Parameters Now that the DataSet has been created and the SQL statement has been generated, you'll want to dynamically change the value that appears just after the LIKE keyword. Essentially what will happen is that value will become a parameter, meaning that rather than using a variable to store the value being sent across, a parameter will be passed into the SQL statement dynamically. To create a new parameter for the SQL statement, follow these steps: -
Open the DataSet from within the search_results.aspx page. -
Change the value just after the LIKE keyword to a question mark (?). This signifies that it will accept a new parameter. -
Select the plus (+) icon from the Parameters dialog box. The Add Parameter dialog box will appear. Give the parameter a name such as "criteria," select the Char data type, and select the Build button to create the code that will capture the value being sent across the querystring. -
Figure 30.35 shows the Build Value dialog box indicating the type of parameter being sent, the name of the parameter, and a default value in case the value being sent across is empty. Figure 30.35. Enter values for the parameter within the Build Value dialog box.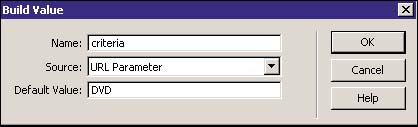 -
Select OK. Figure 30.36 shows the results of the code that is generated and inserted into the Value dialog box for you. Figure 30.36. The code that will be used to capture the value is automatically generated for you.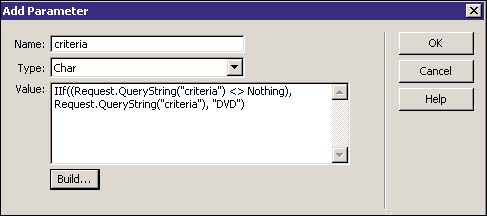 -
Select OK. Figure 30.37 shows the result of the new parameter that was created. You can edit the parameter at any time by selecting the Edit button. Figure 30.37. Create a new subroutine that redirects users to a new page, in the process, appending the value to the querystring as a new parameter.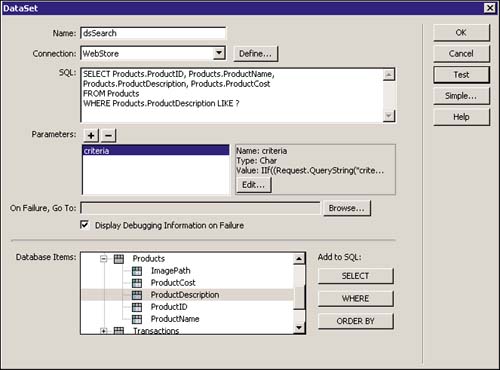 -
You can test the parameter by selecting the Test button. The Test Value dialog box will appear as shown in Figure 30.38, allowing you to enter a value for the new parameter. Enter DVD. Figure 30.38. Enter a new value to test the new parameter.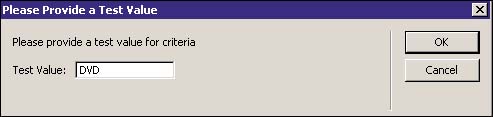 -
Figure 30.39 shows the result of the query based on the "DVD" filter criteria. Test the parameter's functionality by entering DVD Widescreen and DVD Special Edition within the Test Value dialog box. Figure 30.39. The results of the query display are shown.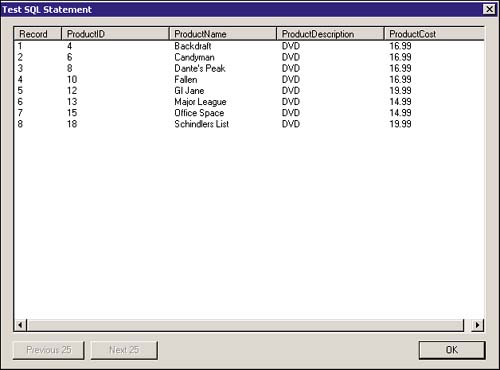 -
Now that the DataSet is complete, you can create dynamic text within the page by dragging the fields into their respective positions, as shown in Figure 30.40. Figure 30.40. Drag the fields of the DataSet into the page, next to the captions that you created earlier.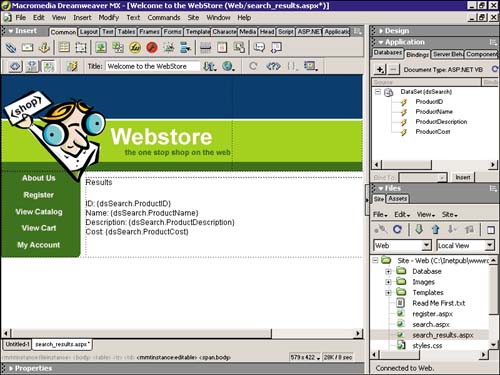 -
Save your work and test it in the browser. Enter a value into the text box, such as DVD, DVD Widescreen, or DVD Special Edition. The result will be shown after you click Submit. Figure 30.41 shows how the value was passed across from page to page within the querystring under the name "criteria." Figure 30.41. The parameter and value are passed along the querystring.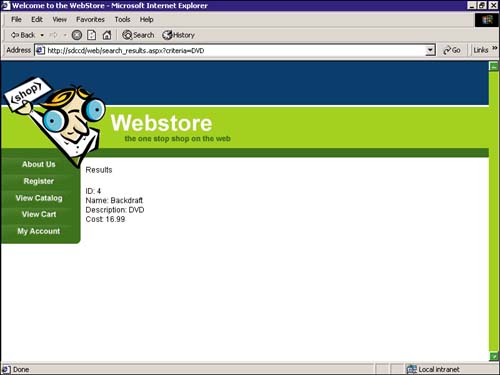 Repeating Regions If you test the search functionality, you will realize that although roughly 10 records matched the criteria for DVD, only one showed up. The problem lies in the fact that the DataSet that is returned with the data is not exposing at once everything that it contains. Using the Repeat Region server behavior, you could effectively force the DataSet to show all its values in an elegant list. To create a repeating region for your search results, simply follow the steps outlined next: -
Insert a new line break just after the last item (Product Cost). -
Highlight all the text and select the Repeat Region server behavior. -
Select OK from the Repeat Region dialog box. -
A new template of invisible elements will appear around the text. -
Save your work and test it in the browser. Figure 30.42 shows how all the records are exposed, rather than just one. Figure 30.42. The repeating region exposes all records within the DataSet.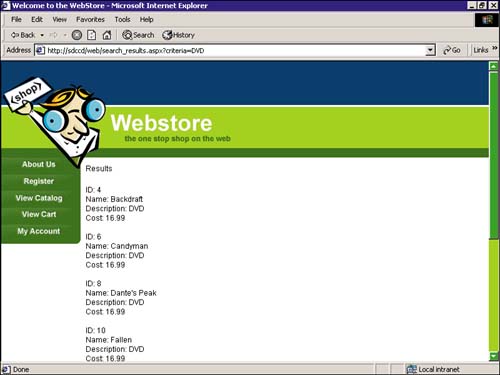 Alternative Text Currently, as users search for data within the database, they are presented with results in a structured format. But suppose a user enters a value to search by and the DataSet cannot return a match? Using the Show Region server behavior set, you could present an alternative text message, alerting the user of the failed search result and enabling the user to link back to the search.aspx page to try the search again. To insert the alternative text into your search results page, follow these steps: -
Insert a new line break just after the Show Region. -
Insert the text Your search produced no results and create a link for Try Again. Make Try Again link back to the search.aspx page. -
Highlight all the text and select the Show if DataSet Is Empty server behavior from the Show Region submenu, as shown in Figure 30.43. Figure 30.43. Insert the Show if DataSet Is Empty server behavior.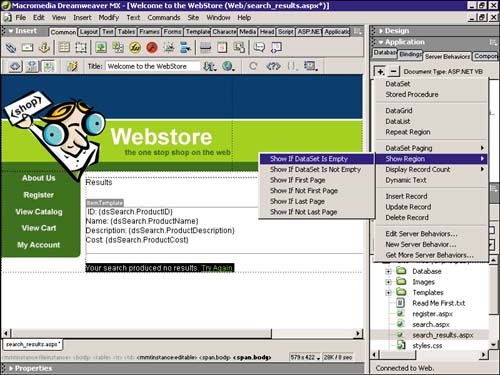 -
The Show Region dialog box will appear. Select the appropriate recordset (rsSearch) and click OK. -
Save your work and test it within the browser. -
In the search.aspx page, type in something that you know it will not find, like VHS. Click Search. Your message will appear along with the link that allows you to link back to the search page, as shown in Figure 30.44. Figure 30.44. If the DataSet comes up empty, you are presented with the custom message.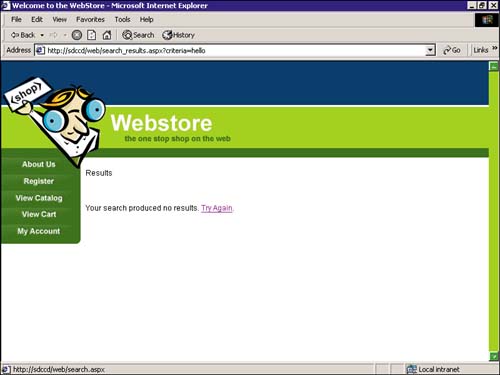 |