Imagine going to school to register for classes. The registrar asks you for your name. If you tell him that your name is "56," he's going to wonder if you're telling the truth. Most likely, he'll ask you for your name again. He must either verify that your name is indeed "56" or get your real name. Any time that you ask people for information, you have to be prepared for the unexpected. You never know what they'll say when presented with a simple question. With computers, this kind of behavior can be disastrous. If an application is expecting one type of data, giving it another type of data may result in an error or even a crash (as you may have already experienced with your ASP.NET pages). Input validation is the process of making sure that the user provides the correct type of data. Input validation is a must when you allow users to enter information manually into your ASP.NET pages. Most users will do their best to comply and enter the information you ask for in the proper format, but sometimes they make mistakes. Other users may like to try breaking applications by entering invalid information. In either case, getting data that you weren't expecting is a very common situation. For example, imagine that you've created an e-commerce site that takes orders for office supplies. Eventually, you'll need to take personal information from the user, such as her name, e-mail, billing address, and so on. You must ensure that this information is accurate, or the order won't be processed correctly. For example, if the user enters a number for the shipping state, such as 45, and the application doesn't catch it immediately, the shipment may never be delivered and you'll end up with an irate customer. So what happens if the user enters a number in place of her name? Or she enters a random string of characters for her e-mail address? Eventually, what you end up with is incorrect data being entered into your system. Detecting invalid input is vital for presenting a solid application. Input validation also provides benefits for developers specifically, coherent data. When you're designing your application, you should always be able to count on the data being in a specific format. For example, if you need to add two numbers, you need to make sure they are indeed numbers. Otherwise, the add operation won't work and your application will fail. When the input has been validated beforehand, you know that your add operation will succeed. Let's examine a typical situation that requires user input validation. Listing 7.1 shows a simple user interface ASP.NET page that allows users to enter some personal information. Listing 7.1 A Typical Scenario for Validation 1: <html><body> 2: <form runat="server"> 3: <asp:Label runat="server" 4: Height="25px" Width="100%" BackColor="#ddaa66" 5: ForeColor="white" Font-Bold="true" 6: Text="A Validation Example" /> 7: <asp:Label runat="server" /><br> 8: <asp:Panel runat="server"> 9: <table> 10: <tr> 11: <td width="100" valign="top"> 12: First and last name: 13: </td> 14: <td width="300" valign="top"> 15: <asp:TextBox runat="server" /> 16: <asp:TextBox runat="server" /> 17: </td> 18: </tr> 19: <tr> 20: <td valign="top">Email:</td> 21: <td valign="top"> 22: <asp:TextBox 23: runat="server" /> 24: </td> 25: </tr> 26: <tr> 27: <td valign="top">Address:</td> 28: <td valign="top"> 29: <asp:TextBox 30: runat="server" /> 31: </td> 32: </tr> 33: <tr> 34: <td valign="top">City, State, ZIP:</td> 35: <td valign="top"> 36: <asp:TextBox 37: runat="server" />, 38: <asp:TextBox runat="server" 39: size=2 /> 40: <asp:TextBox runat="server" 41: size=5 /> 42: </td> 43: </tr> 44: <tr> 45: <td valign="top">Phone:</td> 46: <td valign="top"> 47: <asp:TextBox runat="server" 48: size=11 /><p> 49: </td> 50: </tr> 51: <tr> 52: <td colspan="2" valign="top" align="right"> 53: <asp:Button runat="server" 54: text="Add" /> 55: </td> 56: </tr> 57: </table> 58: </asp:Panel> 59: </form> 60: </body></html>  | This listing uses a few Web controls to display a user interface. The user interface prompts the user for first and last name, e-mail address, street address, city, state, ZIP code, and phone number (note, though, that the form won't actually do anything when submitted, yet). The result of Listing 7.1 is shown in Figure 7.1. | Figure 7.1. A typical user-entry page.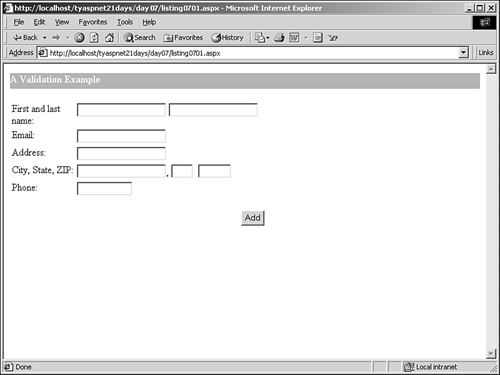 Once the form is submitted, you have to verify that each field contains a valid entry before entering the data in a database. A common way to do this is through a series of if statements, as shown in Listing 7.2. This series of if statements validates all of the server controls in Listing 7.1. Listing 7.2 Validating User Input from Listing 7.1 Through if Statements 1: <script runat="server"> 2: sub Submit(Sender as Object, e as EventArgs) 3: if tbFName.Text <> "" and not IsNumeric(tbFName.Text) then 4: if tbLName.Text <> "" and not IsNumeric (tbLName.Text) then 5: if tbAddress.Text <> "" then 6: if tbCity.Text <> "" and not IsNumeric(tbCity. Text) then 7: if tbState.Text <> "" and not IsNumeric( tbState.Text) then 8: if tbZIP.Text <> "" and IsNumeric( tbZIP.Text) then 9: if tbPhone.Text <> "" then 10: lblMessage.Text = "Success!" 11: else 12: lblMessage.Text = "Phone is incorrect!" 13: end if 14: else 15: lblMessage.Text = "ZIP is incorrect!" 16: end if 17: else 18: lblMessage.Text = "State is incorrect!" 19: end if 20: else 21: lblMessage.Text = "City is incorrect!" 22: end if 23: else 24: lblMessage.Text = "Address is incorrect!" 25: end if 26: else 27: lblMessage.Text = "Last name is incorrect!" 28: end if 29: else 30: lblMessage.Text = "First name is incorrect!" 31: end if 32: end sub 33: </script>  | As you can see, validating all of the controls in this manner is rather long and tedious. The if statement on line 3 verfies that the user has filled in the first name field, and also that the supplied data is not a number. The second if statement on line 4 does the same for the last name field. You proceed this way until you've checked every control that contains user input. Don't forget the else statements, which display a message telling the user what the error is. | After checking all of the user inputs in this fashion, you can take one of two courses. If all of the information entered by the user is in the correct format, you can insert the data in the data source. If any of the information is formatted improperly, you need to alert the user to the errors in the input (done with the else statements) and allow her to fix them before inserting the results into the data store (by simply redisplaying the page). However, this can get quite complex because you often have to check for multiple cases on each input. For example, for the first name, you have to check that the field isn't blank, doesn't contain any numbers, doesn't contain spaces, and so on. This makes for a lot of if statements. As tedious as this may be, it's a typical method of validating user input. The process boils down to the following steps: Display the form to the user and allow her to enter data. Upon form submission, use if statements to check every user input. This includes checking for blank entries, the proper format and data type, valid ranges of information (for dates), and so on. If all if statements pass, perform your functionality. If an if statement fails, redisplay the form, preferably with the fields prefilled with the previously entered user input. This allows the user to correct only the incorrect fields and leave the correct ones. Start over at step 2. |