Before you can display the report from within an ASP.NET application, you'll need to embed the CrystalReportViewer control on a page. In this section, you'll learn to use the control and then to view the page you've created. Creating a Report Viewer Page Follow these steps to set up the CrystalReportViewer control on a page to display your report: -
Use the Project, Add Web Form menu item to add a new page. Name the page ProductsReport.aspx. -
Set the PageLayout property for this new page to flowLayout. -
In the Toolbox window, double-click the CrystalReportViewer control to add an instance to your new page. -
Set the new control's ID property to cvwProducts and its DisplayGroupTree property to False. TIP The viewer control's DisplayGroupTree property enables the display of a navigation tool on the report for working with groupings. If your report doesn't contain groupings (as is the case with this sample report), you'll save space on the screen by setting this property to False. Displaying the Report To actually display the report, you'll need to write a little bit of code. You must first set the ReportSource property to be the full path and filename of the saved report template. Next, you must call the DataBind method so that the control will render the report on the page. Follow these steps to display the report: -
Double-click the page to load the page's code-behind file. Modify the Page_Load procedure so that it calls the ReportBind procedure (which you'll add in the next step): Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles MyBase.Load If Not Page.IsPostback Then ReportBind() End If End Sub -
Add the ReportBind procedure, which sets the path and binds the data for your report: Private Sub ReportBind() With cvwProducts .ReportSource = Server.MapPath("ProductsReport.rpt") .DataBind() End With End Sub  | To test out your new report, follow these steps: | -
Add a hyperlink to Main.aspx, which navigates to your new ProductsReport.aspx page. -
Run the project and select the new link, which loads the report-viewing page. -
If you've done everything right, your page should appear, displaying the finished report, as shown earlier in Figure 20.1. TIP Try out some of the features the control provides you can browse through the pages of data on the report, and you can search for data. You can also control the "zoom" of the report, zooming in and out visually. All these features are controllable programmatically, and you can turn any of them off using properties at design time. Selecting Specific Rows Although you can apply a filter to your data at design time, you may not know which rows a user will want to view and must therefore allow for some filtering at runtime. You might want to supply a drop-down list of available suppliers, for example, and allow users to view a report based on a particular supplier. Although there's not much new technology involved in filling a DropDownList control with a list of suppliers you've seen how to do that in a number of other chapters there is one issue that we've not mentioned previously. You may want to display an "empty"item in the list, allowing you to prompt the user to select an item, hiding the report until the user actually selects an item. Figure 20.7 shows how the DropDownList control will look with the "empty" item selected. Figure 20.7. Create a drop-down list with an "empty" item requesting user input. Once the user selects an item, you can set the CrystalReportViewer control's SelectionFormula property so that the control only displays rows that match the criteria you supply. If you only want rows where the SupplierID field contains the value 18, you can use code like this: cvwProducts.SelectionFormula = _ "{Products.SupplierID} = 18" TIP You must surround Table.Field names in your selection formula expression with braces. It's important that you include both the table and the field names. Also, in this example, you won't hard-code the supplier ID (18). Instead, you'll retrieve its value from the DropDownList control on the page. To modify ProductsReport.aspx so that you can select a supplier and filter the report as well as to see how to add a "Select an item" entry in a drop-down list, follow these steps: -
Open ProductsReport.aspx in the Visual Studio .NET page designer. -
Click to the left of cvwProducts, moving the insertion point to the left of the control. -
Press Enter to insert a "new line," making room for the DropDownList control. -
Drag a Label control and then a DropDownList control onto the page. Set the properties for these controls as shown in Table 20.1. When you're done, the page should look like Figure 20.8. Figure 20.8. Add a label and a drop-down list to select a supplier.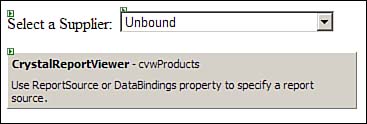 Table 20.1. Set the Properties of the New Controls Using These Guides. Control | Property | Value | Label | Text | Select a Supplier: | DropDownList | ID | ddlSuppliers | | AutoPostBack | True | -
Add the following procedure to your page's class. This procedure allows you to pass in a ListControl object (either a DropDownList or a ListBox control), and it adds a new item in the first position. In addition, the code sets the Value property of the new ListItem object to "-1": Private Sub BlankRowAdd(ByVal lc As ListControl) Dim oItem As New ListItem() oItem.Text = "<-- Select an Item -->" oItem.Value = "-1" lc.Items.Insert(0, oItem) End Sub -
Add the SupplierLoad procedure to your page's class. This procedure loads the ddlSuppliers DropDownList control with a list of all the available suppliers: Private Sub SupplierLoad() Dim strSQL As String Dim strConn As String Dim ds As DataSet strConn = Session("ConnectString").ToString() strSQL = "SELECT SupplierID, CompanyName " & _ "FROM Suppliers ORDER BY CompanyName" ds = DataHandler.GetDataSet(strSQL, strConn) With ddlSuppliers .DataTextField = "CompanyName" .DataValueField = "SupplierID" .DataSource = ds .DataBind() End With BlankRowAdd(ddlSuppliers) End Sub -
Modify the Page_Load procedure to hide the report when the page first loads (forcing the user to select a supplier before the report displays at all) and to fill the list of available suppliers: Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles MyBase.Load If Not Page.IsPostBack Then cvwProducts.Visible = False SupplierLoad() ReportBind() End If End Sub -
Add code to handle the SelectedIndexChanged event of the DropDownList control: Private Sub ddlSuppliers_SelectedIndexChanged( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles ddlSuppliers.SelectedIndexChanged Dim id As String Dim strFormula As String id = ddlSuppliers.SelectedItem.Value ' See the comments below. If id = "-1" Then cvwProducts.Visible = False Else strFormula = "{Products.SupplierID} = " & id cvwProducts.SelectionFormula = strFormula ReportBind() End If End Sub This procedure first retrieves the Value property of the selected item in the DropDownList control: id = ddlSuppliers.SelectedItem.Value Then, the code hides the report viewer: If id = "-1" Then cvwProducts.Visible = False Alternatively, it creates a filtering formula, applies it to the control, and rebinds the report to its data source: Else strFormula = "{Products.SupplierID} = " & id cvwProducts.SelectionFormula = strFormula ReportBind() End If Try out the page, and you should now be able to filter the report based on a supplier. If you select the "empty" item from the list, the report should disappear until you make a new selection. Figure 20.9 shows the page in use. Figure 20.9. Use the DropDownList control to select a supplier.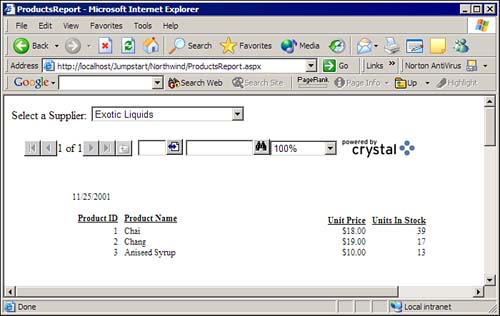 |