Creating components with Flash 8 can be difficult, but very rewarding. Back in Flash MX, to build a component, you placed information in a movie clip, and then converted it to a component. Version 2.0 of components goes a lot further. In this example, we will create a small component that will have a property specifically designed for data binding. What it will do is allow developers to test information by sending it to this component, which will then automatically send it to the Output panel. Keep in mind that this component will only work in the test mode of the authoring environment and is not meant to be used outside of that. The first part will be done in Flash, so follow these steps: 1. | Create a new Flash document called TRacer.fla and save it to the desktop.
| 2. | Using the Text tool, create a letter "T" on the stage and set its size to 16x16.
| 3. | Select the "T" and choose Modify, Convert to Symbol.
| 4. | Give it a symbol name of TRacerG and make sure the behavior is set for Movie clip, and set its registration point to centered.
| 5. | Right-click (MacCtrl-click) the tracerG movie clip in the library and choose Linkage.
| 6. | Select the Export for ActionScript check box, and uncheck the Export in First Frame check box to save file size. Leave the AS 2.0 Class blank and click OK.
| 7. | Back on the main stage, highlight the movie clip "T" and choose Modify, Convert to Symbol again.
| 8. | Give it a Symbol name of "Tracer," make sure the behavior is set to Movie clip, and set its registration point to centered.
| 9. | Right click (MacCtrl-click) the Tracer in the library and choose linkage.
| 10. | Select the Export for ActionScript check box, and uncheck the Export in First Frame check box to save file size. Set the AS 2.0 Class to "Tracer" and click OK.
| 11. | Now double-click the new movie clip symbol to enter edit mode.
| 12. | Name the layer the "T" is residing on Bound.
| 13. | Align the left edge and the top edge to the stage using the Align Panel (Window> Align).
| 14. | Create a second layer under the Bound layer called assets_mc and give it an extra keyframe, so there should be two blank key frames on the assets_mc layer.
| 15. | Make a copy of the first frame in the Bound layer, and paste it exactly the same in the second frame on the assets_mc layer.
This is so that when the component gets dragged onto the stage, the user will be able to see it.
| 16. | In the first frame of the Bound layer, open the Actions panel and place in a stop action like this:
stop(); This will stop the movie clip from looping back and forth, and should always be done in every component you make.
Your stage should now look like Figure 16.11.
Figure 16.11. The stage after step 16 after we have created all the content on the stage needed. 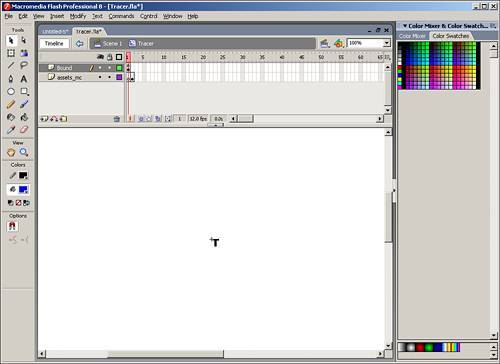
| 17. | Return to the main timeline. We are done with Flash for the time being.
The next section requires us to make a class file. Class files have not been discussed much so far, but you learn more about them in Chapter 18, "External ActionScript." All functionality of components are kept in external class files.
The next step requires the use of a text editor such as Notepad or SciTe. You can also use the AS file builder built into Flash by choosing File, New and choosing ActionScript File.
| 18. | When your new file is open, save it to the same directory as our Flash file as TRacer.as.
| 19. | Drop in this code and save the file again:
import mx.events.EventDispatcher; [Event("traced")] [TagName("Tracer")] [IconFile("Tracer.png")] [RequiresDataBinding(true)] class Tracer extends MovieClip{ private var tracerInfo:String; public var addEventListener:Function; public var removeEventListener:Function; private var dispatchEvent:Function; function Tracer(){ this._visible = false; EventDispatcher.initialize(this); super.init(this); } [Bindable(param1="writeonly",type="String"))] [ChangeEvent("traced")] function set tracerI(t:String):Void{ this.tracerInfo = t; if(tracerInfo.length > 0){ trace(tracerInfo); } dispatchEvent({type:"traced"}); } }
| Let's break this code into sections so you can see what is happening. The first section imports the EventDispatcher class, so our component will have events. The next section uses what are called MetaData tags. These tags are used in processing the component and set certain options. In this case, they are setting the Event we are going to create, the TagName, IconFile, and the fact that this component will need data binding. [Event("traced")] [TagName("Tracer")] [IconFile("Tracer.png")] [RequiresDataBinding(true)] The next section begins our class, which will extend the MovieClip class. Then it creates a private property, tracerInfo, that is the data we are going to trace and then a few methods that we need for the event. class Tracer extends MovieClip{ private var tracerInfo:String; public var addEventListener:Function; public var removeEventListener:Function; private var dispatchEvent:Function; The section after that creates the constructor function that will be called when the component initializes at runtime. In it, we set the visibility of our component to false. Then we initialize the EventDispatcher class so the component will have an event. After that, we initialize the super class, just as a precaution. function Tracer(){ this._visible = false; EventDispatcher.initialize(this); super.init(this); } After that, we use two more MetaData tags. The first declares the parameter of our component as being data bound. The second declares the event we are going to use. [Bindable(param1="writeonly",type="String"))] [ChangeEvent("traced")] Finally, we create two getter/setter methods to control the variable. And we also send the variable to the Output panel here. And finally, we close off the class with a closing curly bracket. function set tracerI(t:String):Void{ this.tracerInfo = t; if(tracerInfo.length > 0){ trace(tracerInfo); } } } Once that is saved as TRacer.as, you can return to Flash. NOTE The PNG file mentioned in the class file is a 16x16 picture of the letter "T," which can be downloaded from the accompanying site, or you can create your own. Just make sure it is residing in the same directory as the FLA and the class file. 1. | Back in the Flash file, open the Library (Window>Library).
| 2. | Select the Tracer movie clip, right-click, (MacCtrl-click) and choose Component Definition.
If you have built components before in Flash MX, the dialog screen will be familiar to you (see Figure 16.12).
Figure 16.12. The Component Definition dialog box where certain parameters of the component are set. 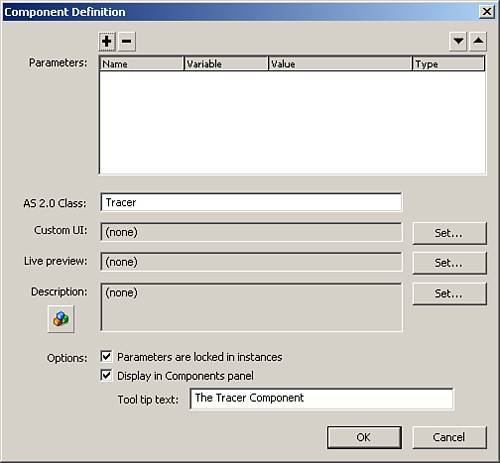
| 3. | Set the AS 2.0 Class to "Tracer" and check the Display in Components Panel check box.
| 4. | Type The Tracer Component in the Tool Tip Text Field and click OK.
| 5. | Now select the component you just made, and right-click again (MacCtrl-click). This time choose Convert to Compiled Clip.
Converting to a compiled clip will actually return a compiled SWF file to the library, which will be the actual component and will be included in your SWC file. This saves a great deal on file size.
| 6. | Select the component you created again, and right-click again (MacCtrl-click). This time choose Export SWC File, and save it to the desktop as TRacer.swc.
| That was it! Now go to the components directory and create a new folder called Unleashed Components, copy the SWC file into it, and restart Flash. NOTE If you get a warning telling you that you cannot have two symbols with the same linkage name, ignore it, it just means the component will not work in this file because when we created the compiled clip, it was given the same linkage name as the movie clip. To test the component, follow these steps after you have restarted Flash: 1. | Create a new Flash document.
| 2. | Drag an instance of the tracer component onto the stage and give it an instance name of mytracer.
| 3. | Now drag an instance of the Button component, give it an instance name of myButton, and set the toggle parameter to true.
| 4. | Select the tracer component, open the Component Inspector, and select the Bindings tab.
| 5. | Click the Add Binding button, choose tracerI:String, and click OK.
| 6. | Select the Bound To field and click the magnifying glass that appears.
| 7. | Choose the Button component in the left window, choose selected : Boolean in the right window, and click OK.
| 8. | Test the movie and every time you toggle the button, it will display a Boolean value in the Output panel.
| That was awesome! You have created a component that has data bindings, which will help you test other applications in the future. |