You may be the designer, but that doesn't mean that everybody is going to like your style. Why not provide your visitors with alternative looks and/or layouts for your Web page? Building on what you learned about disabling style sheets in "Disabling or Enabling a Style Sheet" in Chapter 18, we can do just that. In this example, four buttons are presented across the top of the page (Figure 24.6) The visitor can click one of these at any time, to choose between three alternate styles for the page design (Figure 24.7). Figure 24.6. The default style for the page. Figure 24.7. The three alternate styles (style1, style 2, and style). 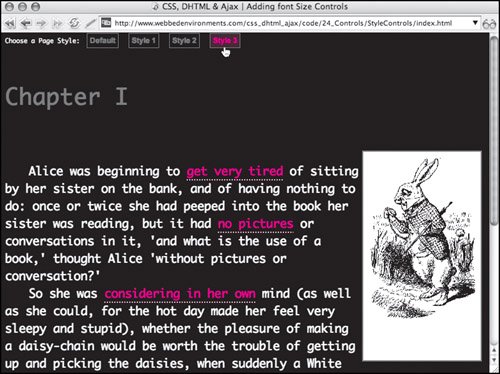 To set up style controls for a page: | | 1. | styleControls.js Save a new external JavaScript file called styleControls.js (Code 24.7). Steps 2 through 5 apply to this file.
Code 24.7. styleControl.js: The styles are initially set and changed by functions in this file. var styleNames = ['style1', 'style2', 'style3']; var oldStyle = null; function initStyles () { for (var i = 0; i < styleNames.length; i++) { styleObject = document.getElementById (styleNames[i]); styleObject.disabled = true; } } function setStyle (newStyle) { if (oldStyle) oldStyle.disabled = true; if (newStyle != 'default') { var styleObject = document.getElementById(newStyle); styleObject.disabled = false; oldStyle = styleObject; } else oldStyle =null; } window.onload = initStyles; | | 2. | var styleNames = ['style1', 'style2', 'style']; Initialize the following variables:
| 3. | function initStyles () {…} Add the function initStyles(), which is used to loop through the array of style IDs and disable all of the style sheets on the page except for the default style sheet.
| | | 4. | function setStyle (newStyle) {…} Add the function setStyle(), which disables the previous style (if there was one) and then enables the selected newStyle.
| 5. | window.onload = initStyles; Add a function call for initStyles(). This will cause the function to run as soon as the script loads, which works better than having an onload event handler in the <body> tag.
| 6. | default.css Create a new external CSS file and save it as default.css (Code 24.8). This style sheet will always be applied to the Web page, providing the general styles for the page.
Code 24.8. default.css: The default style sheet that will always be applied to the page. .dropBox { float: right; padding: 4px; margin: 4px; background-color: white; border: 2px solid #666; } .dropBox img { display: block; } h2 { padding-bottom: 0px; margin-bottom: 0px; color: #666; } h2 .chapterTitle { display: block; font-size: smaller; color: black; } p a { color: #f33; text-decoration: none; padding-bottom: 0px; } p a :link { color: #f33; border-bottom: 2px dotted #fcc; } #content .copy { margin-top: 24px; } #content .copy p { font-size: smaller; line-height: 1 .5; margin: 0px; text-indent: 2em; } .dropBox p { margin: 0px; padding: 0px; width: 175px; font: x -large "Party Let", "Comic Sans MS", Helvetica, Arial, sans-serif; } .styleControl { color: #666; font-family: Arial, Helvetica, sans-serif; font-weight: bold; text-decoration: none; margin: 0px 5px 0px 5px; border: 1px solid #666; padding: 4px; } .styleControl:hover{ color: #f00; border: 1px solid #666; background-color: #00; } | | | | 7. | style1.css, style2 .css, style.css Create as many alternate style sheets as you want (Codes 24.9, 24.10, and 24.11). These files can contain any CSS you want to completely restyle the page.
Code 24.9. style1.css: The first alternate style sheet. body { font-size: 1 .2em; font-family: Georgia, "Times New Roman", times, serif; color: #000000; background-color: #999; margin: 8px; } | Code 24.10. style2.css: The second alternate style sheet. body { font-size: .9em; font-family: sans -serif; color: #999; background-color: #fff; margin: 8px; } | Code 24.11. style3.css: The third alternate style sheet. body { font-size: 1 .5em; font-family: monospace; color: #fff; background-color: #000; margin: 8px; } h2 .chapterTitle { font-size: larger; color: black; } | In this example, I've kept it simple by changing some styles for the <body> element. However, you can completely change the look and layout of your Web site. Remember that any style you add to the default.css that you want changed in an alternate style will have to be included to be overridden.
| | | 8. | index.html Save a new HTML file as index.html (Code 24.12). Steps 9 through 14 apply to this file.
Code 24.12. index.html: All three alternate style sheets are imported into the page, but they are disabled until needed. | 9. | <link href ="css/default.css" rel="stylesheet" type ="text/css" media="all" /> Add a link to the default style sheet (default.css), which provides the core styles for the page. These styles can be overridden by the alternate style sheets from Step 7.
| 10. | <style id ="style1" type="text/css">…</style> For each of your alternate styles, set up a <style> block with an ID corresponding to one of the IDs you specified in the array from Step 2.
| 11. | @import url(css/style1.css); Within each of the <style> tags from Step 10, add @import with the url pointing to the relevant external CSS file from Step 7.
| 12. | <script type ="text/javascript" src="/books/3/292/1/html/2/styleControls.js"></script> Add a call to your external JavaScript file from Step 1.
| 13. | <div id ="controls" style="font: bold" 10px/10px monospace ;">…</div> Add a control layer to your page, setting the font declaration inline so that the changes in the page style do not make the controls shift around.
| 14. | [View full width] <div onclick ="setStyle('style1')" style ="font-size: 12px ">Style 1< /div>
Add controls used to enable any of the alternate styles. This uses the function from Step 4, passing it the ID for the style to be used (setStyle('style1')).
| |