Although DHTML incorporates a lot of different techniques, the simplest and one of the most common tasks you will be performing is to make changes to CSS properties on the fly. CSS already includes several dynamic pseudo-classes (:hover, :active, and :focus) that let you make changes without using JavaScript, and these pseudo-classes are preferred whenever they can be used. However, JavaScript provides you with a much broader toolset for changing styles on the fly. In this simple example (Figures 16.1 and 16.2), I've set up a function that takes as its input the ID of an element, the CSS property to be changed, and the new value to be assigned to the property. Figure 16.1. Before the object gets clicked, it is a perfectly innocent white box. 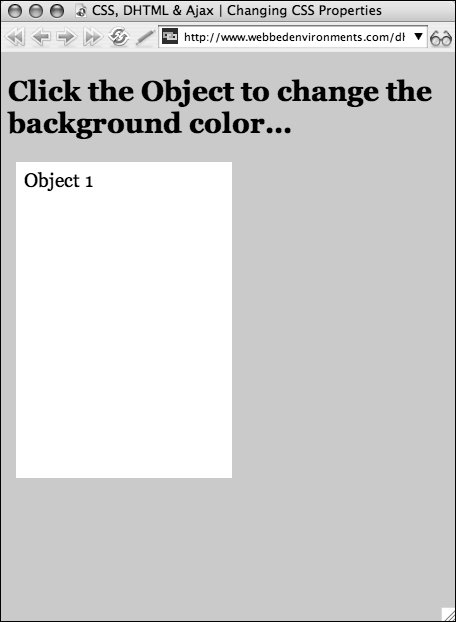 Figure 16.2. After clicking, though, the object's background-color CSS property changes to a vibrant red. 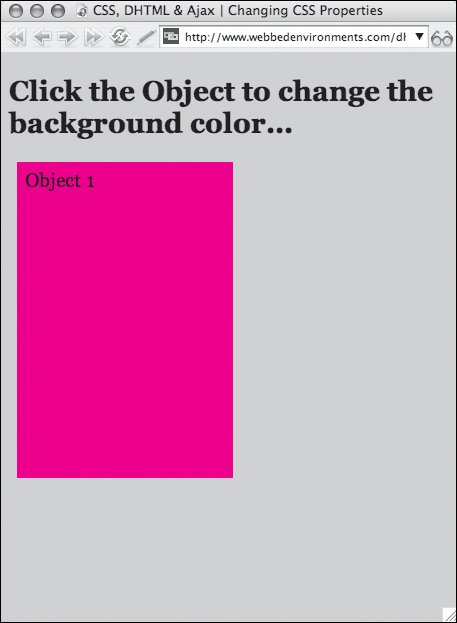 To change an element's style properties: | | 1. | function changeStyle(objectID, CSSProp, newVal) {...} Add the function changeStyle() to your JavaScript (Code 16.1).
Code 16.1. the function changeStyle() allows you to change the value of any CSS property. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>CSS, DHTML & Ajax | Changing CSS Properties</title> <script type="text/javascript"> function changeStyle(objectID,CSSProp,newVal) { var object = document.getElementById (objectID); object.style[CSSProp] = newVal; } </script> <style type="text/css" media="screen"> #object1 { background-color: #fff; width: 200px; height:300px; margin: 8px; padding: 8px; } </style> </head> <body> <h2>Click the Object to change the background color...</h2> <div onclick="changeStyle('object1', 'backgroundColor', 'red');">Object 1 </div> </body></html> | This function uses the ID of the object to be addressedpassed to it as the variable objectIDto find the element to be changed. It then uses that object address to change the style of the indicated property (CSSProp) to the new value (newVal).
Notice that since we are using a variable in place of the actual property name, the variable CSSProp is placed in square brackets.
| | | 2. | #object1{...} Add a CSS rule for the object. You can include the initial value of the CSS property you will be changing in the function from Step 1, but you don't have to.
| 3. | onclick="changeStyle('object1', 'backgroundColor', 'red');" Add an event handler to an element that triggers the function from Step 1, passing it the ID of the object being changed, the property name, and the new value.
| Tips Notice in this section's example that the CSS property name background-color is written as backgroundColor in JavaScript. JavaScript doesn't like hyphens, so hyphenated CSS property names are written without them, but the first letter of subsequent words are capitalized. Although CSS includes several dynamic pseudo-classes, they only work for the link tag in older versions of Microsoft Internet Explorer. Fortunately, Internet Explorer 7 corrects this. In order to be read by JavaScript, CSS properties have to be set by JavaScript first, or the value will appear as null. There is a way around this, but requires a good deal of additional code. See "Finding a Style Property's Value" in Chapter 18 for more details. |