The Windows registry is a hierarchical database used by the operating system to maintain a variety of system settings. For example, Internet Explorer stores option settings and recent URLs you have typed in the registry. The information for ODBC data sources you create also is stored in the registry. Figure 24.6 shows the Windows Registry Editor, which can be used to view and update registry information. Figure 24.6. You can use the registry to store information related to your application, such as a recent file list or database connection string. 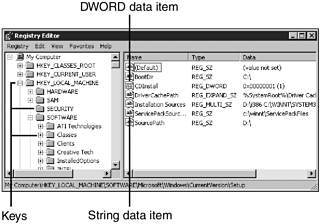 You can access the registry by running the regedit.exe program, as shown in Figure 24.6. Understanding the Registry's Structure As you can see from the figure, the registry is displayed visually as a tree of folders, much like subdirectories on your hard drive in Windows Explorer. Each folder in the registry tree is referred to as a registry key. If you run the regedit.exe program, you will notice there are several top-level keys that begin with the prefix HKEY such as HKEY_CURRENT_USER, which provides an information store for the currently logged-in user. A registry keys can contain additional keys (subkeys) as well as name-value pairs. The name-value pairs may be binary, hex, decimal, or strings, depending on the needs of your application. Updating and Reading Registry Values If you want to store or retrieve registry data, the Registry class in the Microsoft.Win32 namespace provides the necessary methods. You can associate a key in the registry with an instance of the Registry class and then call methods to set or read values. Note Use caution when editing unknown values in the registry. Messing around with Windows' internal settings could leave your system in an inoperable state! Registry keys have a path, which like file paths is delimited by the slash character. To identify a key, you have to provide both a top-level key and a subkey path. Each top-level key is associated with a static field in the Registry class, as described in Table 24.4: Table 24.4. Static Fields for Registry Keys Registry Class Field | Corresponding Registry Key |
---|
ClassesRoot | HKEY_CLASSES_ROOT | CurrentConfig | HKEY_CURRENT_CONFIG | CurrentUser | HKEY_CURRENT_USER | DynData | HKEY_DYN_DATA | LocalMachine | HKEY_LOCAL_MACHINE | PerformanceData | HKEY_PERFORMANCE_DATA | Users | HKEY_USERS | To access a key in the registry, use the OpenSubKey method of the appropriate top-level field. The remaining subkey path is provided as a parameter to the OpenSubKeymethod, as in the following example: Const WinLogonPath As String =_ "SOFTWARE\Microsoft\Windows NT\CurrentVersion\WinLogon" Dim LogonKey As RegistryKey LogonKey = Registry.LocalMachine.OpenSubKey(WinLogonPath, True) In the third line of code, the LocalMachine field is used to identify the KEY_LOCAL_MACHINE top-level key, which stores information about the local PC. The OpenSubKey function accepts the remaining subkey path, followed by a Boolean variable that determines whether the key is updateable. If you just want to read information from the registry, set this parameter to False. If you plan on updating the registry, set this parameter to True. With an instance of a RegistryKey object, you can manipulate values in the registry key by calling the GetValue and SetValue functions. The following example sets registry values in the Winlogon subkey that will cause a Windows 2000 or NT machine to automatically log on at startup: LogonKey.SetValue("AutoAdminLogon", "1") LogonKey.SetValue("DefaultUserName", "Administrator") LogonKey.SetValue("DefaultPassword", "topsecret") If a value does not already exist, then SetValue will create it. Reading a value from the registry is easy as well simply use the GetValue method: Dim strValue As String strValue = LogonKey.GetValue("AutoAdminLogon", "?").ToString The GetValue method returns an object that can be converted to a string or numeric value. A second optional parameter allows you to specify a default return value. In the previous example, if no AutoAdminLogon value exists, a question mark (?) will be returned. If the default value is not passed to the GetValue method and the value does not exist, Null will be returned. |