Now take a look at how the registration form is validated with JavaScript. Whenever you click the Submit button on a form, two events are triggered in the browser: onclick and onsubmit. You're interested in the onsubmit event. When the onsubmit event occurs, a JavaScript function will be called to validate the form data. To call a JavaScript function when the onsubmit event occurs, you include onsubmit as an attribute of the <form> tag, like this: <form method="post" action="http://www.example.com/cgi-bin/post-query" onsubmit="return checkform(this)"> In this example, the value assigned to onsubmit is a call to a function named checkform()which will be defined in a bit. But first, the return statement at the beginning of the onsubmit field and the this argument inside the parentheses in the checkform() function need some further explanation. First, let's tackle this. Whenever you call a function, you can send it a list of parameters, such as numbers, strings, or other objects, by including them in the parentheses that follow the function name. In the preceding example, the this statement passes a reference to the form object associated with the current form. Second, the return statement transmits a value back to the internal routine that called the onsubmit event handler. For example, if the checkform() function returns a value of false after evaluating the form, the submission process will be halted as the return command transmits this false value back to the submit event. If the return statement was not included in the event handler, the false value returned by the function would not be received by the event handler, and the submission process would continue even if problems were detected by the checkform() function. The Validation Script As you've done before, define a <script> tag inside the <head> block and declare checkform() as a function. But this time, you also need to define a variable to hold a reference to the form object sent by the calling function, as mentioned previously. The code for the function declaration looks like this: <script language="JavaScript"> <!-- start script here function checkform(thisform) The reference to the current form is given the name thisform by the function declaration. By accessing the thisform object, you can address all the fields, radio buttons, check boxes, and buttons on the current form by treating each as a property of thisform. Having said this, you first want to test whether a name has been entered in the Name text box. In the HTML code for this form, the <input> tag for this field is assigned a name attribute of theName, like this: <input type="text" name="theName" /> You use this name to reference the field as a child of thisform. As a result, the field theName can be referenced as thisform.theName, and its contents can be referenced as thisform.theName.value. Using this information and an if test, you can test the contents of theName to see whether a name has been entered: if (thisform.theName.value == null || thisform.theName.value == "") { alert ("Please enter your name"); thisform.theName.focus(); thisform.theName.select(); return false; } Note The || operator (the or operator) shown in the if expression tells JavaScript to execute the statements if either of the two tests is true. In the first line, thisform.theName.value is tested to see whether it contains a null value or is empty (""). When a field is created and contains no information at all, it is said to contain a null; this is different from being empty or containing just spaces. If either of these situations is true, an alert() message is displayed (a pop-up dialog box with a warning message), the cursor is repositioned in the field by thisform.theName.focus(), the field is highlighted by thisform.theName.select(), and the function is terminated by a return statement that is assigned a value of false. If a name has been entered, the next step is to test whether a gender has been selected by checking the value of gender. However, because all the elements in a radio button group have the same name, you need to treat them as an array. As a result, you can test the status value of the first radio button by using testform.gender[0].status, the second radio button by using testform.gender[1].status, and so on. If a radio button element is selected, the status returns a value of true; otherwise, it returns a value of false. To test whether one of the gender radio buttons has been selected, declare a new variable called selected and give it a value of false. Now loop through all the elements using a for loop; if the status of any radio button is true, set selected = true. Finally, if selected still equals false after you finish the loop, display an alert() message and exit the function by calling return false. The code required to perform these tests is shown here: var selected = false ; for (var i = 0; i <= 2 ; ++i) { if (thisform.gender[i].status == true) { selected = true; } } if (selected == false) { alert ("Please choose your gender"); return false; } If both of the tests pass successfully, return a value of true to indicate that the form submission should go ahead: return true; } The Completed Registration Form with JavaScript Validation When the JavaScript script that you just created is integrated with the original registration form document from Lesson 10, the result is a web form that tests its contents before they're transmitted to the CGI script for further processing. This way, no data is sent to the CGI script until everything is correct. If a problem occurs, the browser informs the user (see Figure 13.3). Figure 13.3. An alert message. 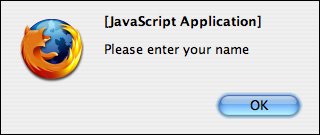 So that you don't need to skip back to the exercise in Lesson 10 to obtain the HTML source used when creating the form, here's the completed form with the full JavaScript code. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Registration Form</title> <script language="JavaScript"> <!-- start script here function checkform(thisform) { if (thisform.name.value == null || thisform.name.value == "") { alert ("Please enter your name"); thisform.name.focus(); thisform.name.select(); return false; } var selected = false ; for (var i = 0; i <= 2 ; ++i) { if (thisform.gender[i].status == true) { selected = true; } } if (selected == false) { alert ("Please choose your sex"); return false; } return true; } // End of script --> </script> <style type="text/css"> body { background-color: #9c9; } input.required { width: 300px; font: bold 12px Verdana; background-color: #6a6; border: solid 2px #000; } select.required { width: 300px; font: bold 12px Verdana; background-color: #6a6; border: solid 2px #000; } td.required { font: bold 12px Verdana; } input.optional { width: 300px; font: 12px Verdana; background-color: #6a6; border: solid 2px #999; } textarea.optional { width: 300px; font: 12px Verdana; background-color: #6a6; border: solid 2px #666; } td.optional { font: 12px Verdana; } input.submit { background-color: #6a6; border: solid 2px #000; font: bold 12px Verdana; } </style> </head> <body> <h1>Registration Form</h1> <p>Please fill out the form below to register for our site. Fields with bold labels are required.</p> <form action="/cgi-bin/register.cgi" method="post" enctype="multipart/form-data" onsubmit="return checkform(this)"> <table> <tr> <td align="right" ><b>Name:</b></td> <td><input name="name" /></td> </tr> <tr> <td align="right" ><b>Gender:</b></td> <td > <input type="radio" name="gender" value="male" /> male <input type="radio" name="gender" value="female" /> female</td> </tr> <tr> <td align="right" > <b>Operating System:</b></td> <td> <select name="os" > <option value="windows">Windows</option> <option value="macos">Mac OS</option> <option value="linux">Linux</option> <option value="other">Other ...</option> </select> </td> </tr> <tr> <td valign="top" align="right" >Toys:</td> <td > <input type="checkbox" name="toy" value="digicam" /> Digital Camera<br /> <input type="checkbox" name="toy" value="mp3" /> MP3 Player<br /> <input type="checkbox" name="toy" value="wlan" /> Wireless LAN</td> </tr> <tr> <td align="right" >Portrait:</td> <td><input type="file" name="portrait" /></td> </tr> <tr> <td valign="top" align="right" > Mini Biography:</td> <td> <textarea name="bio" rows="6" cols="40" ></textarea> </td> </tr> <tr> <td colspan="2" align="right"> <input type="submit" value="register" /> </td> </tr> </table> </form> </body> </html> |