6.1. How do I do that? To verify that ASP.NET 2.0 will dynamically recompile your .aspx and code-behind files, you will create a web application that uses a class stored in a special directory called App_Code. When the class stored in the App_Code folder is modified, ASP.NET 2.0 will automatically recompile it when the page is requested. Launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap06-DynamicRecompilation. To use dynamic runtime compilation for your ASP.NET 2.0 application, you need to add a special folder called App_Code to your project. Right-click the project name in Solution Explorer and select Add New Folder Application Code Folder. You should now see the App_Code folder (see Figure 6-1). Figure 6-1. The App_Code folder in Solution Explorer 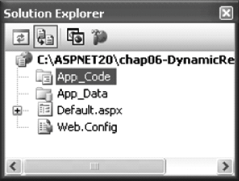
Special Folders in ASP.NET 2.0 In ASP.NET 2.0, there are several of directories under your project that have special significance. They are:
- App_Code
-
The App_Code folder is a container for class files and WSDL and XSD documents. All files contained within this folder are automatically compiled during runtime.
- App_Data
-
The App_Data folder is used to contain database files as well as XML and schema files.
- App_LocalResources
-
The App_LocalResources folder stores the resource files generated by Visual Studio to be used for implicit localization. See Chapter 8 for more information.
- App_GlobalResources
-
The App_GlobalResources folder is used to store resource files required for globalizations. Note that the resource files (.resx) placed in this folder are automatically exposed via the Resources class, providing IntelliSense statement completion for the resources contained within. See Chapter 8 for more information.
- App_Themes
-
The App_Themes folder stores themes and skin files.
- App_Browsers
-
The App_Browsers folder is used to store browser files for supporting different types of clients.
- App_WebReferences
-
The App_WebReferences folder stores .wsdl and .discomap files of web services. The /Bin directory is still supported for backward compatibility with ASP.NET 1.x applications. |
Right-click the App_Code folder and select Add New Item. Select Class and name it TimeClass.vb. If you right-click the project name and try to add a class file to your project, you will be prompted to add the class within the App_Code folder. If the folder does not exist, one will be created automatically. Code TimeClass.vb as follows: Imports Microsoft.VisualBasic Public Class TimeClass Public Function getCurrentTime( ) As String Return "The current time is " & Now End Function End Class Switch to the code-behind of the default Web Form and in the Form_Load event, code the following: Protected Sub Page_Load(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.Load Dim time As New TimeClass Response.Write(time.getCurrentTime( )) End Sub Press F5 to test the application. You should see something like Figure 6-2. Figure 6-2. Testing the application 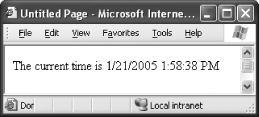
Without closing IE, make some modifications to the TimeClass.vb file. Use Notepad and load TimeClass.vb from C:\ASPNET20\chap06-DynamicRecompilation\App_Code\. Change the code shown in bold in the following snippet: Imports Microsoft.VisualBasic Public Class TimeClass Public Function getCurrentTime( ) As String Return "Server time is now" & Now End Function End Class Refresh the IE web browser. You will notice that it takes a while to reload. This is because ASP.NET has detected that the TimeClass.vb file has changed and hence is recompiling it. Once compilation is complete, you should see the updated output from the TimeClass class shown in Figure 6-3. Figure 6-3. Displaying the updated page 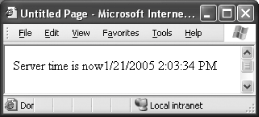
6.1.1. What about... ...using multiple languages in the same project? As you have seen, you can place your class files into the App_Code folder so that ASP.NET can dynamically compile them. However, because all these files will be compiled into a single assembly, you cannot have files of different languages (such as VB2005 and C#) all contained within this folder. Note: ASP.NET will automatically use the correct language compiler to compile the files contained in the App_Code folder. If you want to keep multi-language files within the App_Code folder, you need to separate them into different subfolders within the App_Code folder. Tip: As mentioned in Chapter 1, while support for multiple languages is a useful feature, developers should weigh the pros and cons of mixing languages in a project. The pro is that you can have different programmers (with different language preferences) working together on a project. However, this also may increase the effort required in maintaining the project in the future. To create subfolders within the App_Code folder, add the <codeSubDirectories> element to the Web.config file: <compilation debug="true"> <codeSubDirectories> <add directoryName="VB" /> <add directoryName="CS" /> </codeSubDirectories> </compilation> Tip: The names of the subfolders under App_Code are purely arbitrary and will not influence the type of language files you can store. ASP.NET will examine the file types within the subfolders and use the appropriate compiler to compile the source code in each folder. The important point is this: files of the same language must be in the same folder. Right-click the App_Code folder, and then select Add Folder Regular Folder. Name the folder VB. Repeat this step one more time and name the folder CS. Your App_Code folder tree should now look like the one shown in Figure 6-4. Figure 6-4. The subfolders within the App_Code folder 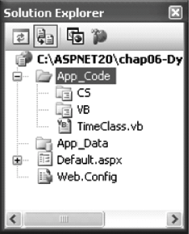 You can now drag and drop the TimeClass.vb file into the VB folder. You can also add a new class file (say, Employee.cs, written in C#) to the CS folder (see Figure 6-5). Figure 6-5. Adding source files to the respective folders 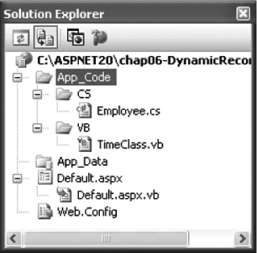 6.1.2. Where can I learn more? The choice of which language to use has always been a hotly debated topic. If you are also pondering whether to use VB.NET or C#, check out some of the discussions on the Web and decide for yourself: http://blogs.msdn.com/csharpfaq/archive/2004/03/11/87816.aspx http://dotnetjunkies.com/WebLog/sahilmalik/archive/2004/05/07/13071.aspx http://www.developerdotstar.com/community/node/61 |