Now that you've created a data-storage architecture, you need to develop a way to retrieve the information from storage and that involves some new syntax that provides a means of writing a dot syntax path dynamically. Square brackets are used to evaluate an expression. Here's how it works: Assume we have three variables that contain animal sounds: dog = "bark"; cat = "meow"; duck = "quack"; We'll create a variable and assign it a text value of "dog," "cat," or "duck" (note that these text values have the same names as our variables). We'll start with "dog": currentAnimal = "dog"; Using the following syntax, we can access the value of the variable named dog: animalSound = this[currentAnimal]; Here, animalSound is assigned a value of "bark." The expression to the right of the equals sign looks at currentAnimal and sees that it currently contains a text value of "dog." The brackets surrounding currentAnimal tell ActionScript to treat its value as a variable name. Thus, this line of script is seen by Flash as the following: animalSound = this.dog; Since dog has a value of "bark," that's the value assigned to animalSound . If we were to change the value of currentAnimal to "cat," animalSound would be assigned a value of "meow." Note that you must use this in the expression to include the target path of the variable used to set the dynamic variable name (in this case currentAnimal ) as it relates to the variable to the left of the equals sign (in our case animalSound ). Using this denotes that these two variables are on the same timeline. If animalSound existed in a movie clip instance's timeline while currentAnimal was on the root, or main timeline, the syntax would look like this: animalSound = _root[currentAnimal]; A couple of other examples include the following: animalSound = _parent[currentAnimal]; animalSound = myMovieClip[currentAnimal]; This syntax is critical to the way you'll be retrieving information from the objects in this exercise. You'll remember that all of the objects are built with the same array names and structure; they just have different parent object names (monday and tuesday ). We can use the aforementioned syntax to access the data in these objects dynamically based on the current value of a variable. -
With newsFlash4.fla still open, select Frame 1 of the Actions layer. Open the Actions panel and enter the following two variables: day = "monday"; section = "entertainment"; 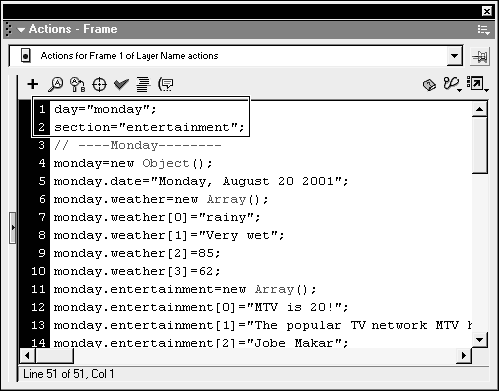 When our application plays for the first time, we want Monday to appear initially and Entertainment news to be displayed. These two variables allow us to accomplish this you'll see how in the next few steps! NOTE The above two variables are known as initializing variables. Since the information our application displays will depend on which buttons the user clicks, these variables provide some starting settings (prior to user input). -
Select the frame labeled refresh in the Actions layer. Enter this ActionScript onto that frame: date.text = this[day].date; This uses the syntax we introduced at the beginning of this exercise. The value of the variable date.text is set by dynamically referencing another variable. Since day initially has a value of "monday" (as set in the last step), ActionScript sees the above code as the following: date.text = monday.date; You'll remember that monday.date contains the text value of "Monday, August 20 2001" and date is the name of the text field in the bottom-left portion of the screen. Thus, as a result of this action, "Monday, August 20 2001" will be displayed in the date text field. You can begin to see how dynamically named variables can be useful. If the variable day had a value of "Tuesday," this line of code would reference the date variable in the object called tuesday . In a moment, we'll explain why we placed this action on the frame labeled refresh. -
With the current frame still selected, add the following ActionScript to the bottom of the current script: days.gotoAndStop(day); icon.gotoAndStop(this[day].weather[0]); weatherBlurb.text = this[day].weather[1]; 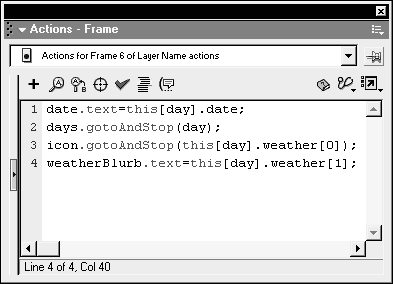 These three actions depend on the current value of day (which we set to an initial value of "monday" in Step 1) when they are executed. Here's how. The movie clip instance named days in the bottom right portion of the stage contains five buttons (M, T, W, T, F) that will enable the user to select the day's news that he or she wishes to see. This movie clip also contains five frame labels ("Monday," "Tuesday," and so on), one for each day of the business week. Each of the frame labels displays a different day in yellow. The first line of ActionScript above tells the days movie clip instance to go to the appropriate frame, based on the current value of day . This cues the user to what day's news he or she is viewing. Since day has an initial value of "monday," that day will appear in yellow.  The weather icon (instance name icon) contains three frame labels, one for each weather type ("Sunny," "Stormy," "Rainy"). You'll remember that the zero element of the weather array in the monday and tuesday objects contains one of these three values. The second line of ActionScript above dynamically pulls that value from the weather array using the correct object and sends the icon movie clip instance to the correct frame. Flash sees the following: icon.gotoAndStop(this[day].weather[0]); as icon.gotoAndStop(this.monday.weather[0]); Or, taking it one step further, since monday.weather[0] has a value of "rainy": icon.gotoAndStop("rainy"); In the same way, the last action will populate the weatherBlurb text field with the value of monday.weather[1] , which is "Very Wet." Keep in mind that if day had a value of "Tuesday," these actions would be executed based on the respective values in the tuesday object. -
Add the following ActionScript to the selected frame: high.text = this[day].weather[2]; low.text = this[day].weather[3]; Using the same syntax as in the previous step, the high and low text fields are populated by referencing the second and third elements of the weather array dynamically. -
Add these final actions to the currently selected frame: headline.text = this[day][section][0]; article.text = this[day][section][1]; author.text = this[day][section][2]; 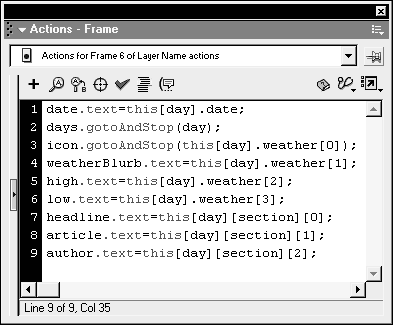 The dynamic referencing performed in this step is one level deeper into the storage objects than referenced in the previous step. We're trying to dynamically pull information about a news article from an object and an array because the day can be either Monday or Tuesday and the section can be Entertainment, Politics, Sports, or Technology. The initialization variables you set in Step 2 of this exercise set section = "entertainment ." Using the values of our initialization variables, Flash will read the above three lines of ActionScript as follows when the movie first plays: headline.text = this.monday.entertainment[0]; article.text = this.monday.entertainment[1]; author.text = this.monday.entertainment[2]; The only text field instances affected by the current section variable are headline, article, and author. The ActionScript added in Steps 2 through 5 exists at the refresh label on the timeline. It's important to note that our application will be sent back to this frame label whenever the value of day or section is changed so that the information on the screen can be updated. -
Move to the frame labeled sit and select it in the Actions layer. With the Actions panel open, enter a stop(); action. When you test the movie, you don't want the entire movie to loop repeatedly. Instead, the movie will initialize the storage variables on Frame 1 and then move to the refresh label and populate the text fields on the screen. Once this has been done, the movie will halt on the sit frame. -
Choose Control > Test Movie. Your on-screen text fields should be populated with information pertaining to Monday entertainment. The weather icon movie clip should display the correct icon, and the days movie clip instance (on the bottom right) should have the M (for Monday) highlighted. -
Close the test movie. Select the Entertainment button and add this ActionScript: on (release) { section = "entertainment"; this.gotoAndPlay("refresh"); } 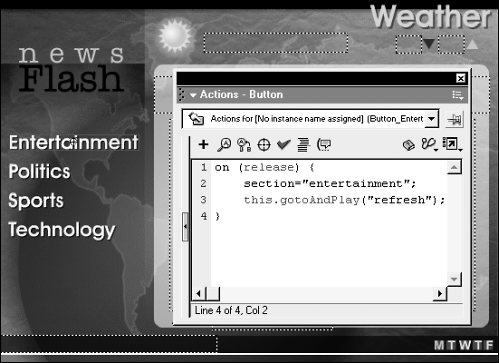 When that button is released, the section variable is set to "entertainment" and the screen is refreshed. This changes the display to an entertainment-related article (if one isn't already showing). -
Select the Politics button and add this ActionScript: on (release) { section = "politics"; this.gotoAndPlay("refresh"); } This ActionScript does the same thing as the ActionScript attached to the Entertainment button the only difference is that section is set to "politics ." -
Select the Sports button and add this ActionScript: on (release) { section = "sports"; this.gotoAndPlay("refresh"); } The ActionScript added to this button is identical to that on the other two buttons; however, this time section is set to "sports ." -
Select the Technology button and add this ActionScript: on (release) { section = "technology"; this.gotoAndPlay("refresh"); } The section variable is set to "technology," and the screen is then refreshed to show the updated information the same as in the previous three buttons. -
Choose Control > Test Movie. Click the four category buttons to see the headlines, articles, and authors change. The information should change very easily now. If you wanted to add more sections of news stories, it would be easy to do so using this object-oriented coding technique. -
Close the test movie and double-click the days movie clip instance on the bottom right of the screen. On the Buttons layer, select each button individually and add the following using the appropriate day: on (release) { _parent.day = "monday"; _parent.gotoAndPlay("refresh"); } 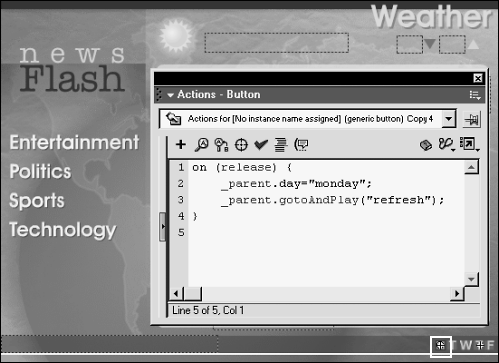 For the button that overlaps T (for Tuesday), change the ActionScript to _parent.day = "tuesday ." This ActionScript is similar to that used to update sections: It changes the day variable and then refreshes the screen. In this project, all of the buttons are used to set the values of either the day or section variables. The playhead is then sent back to the frame labeled refresh. Since the refresh frame contains all of the ActionScript used to populate our text fields, each time the timeline is sent back to this frame, it will refresh the content on the screen based on the current values of day and section . -
Choose Control > Test Movie. Click the M and T on the bottom right of the screen to change the display. Notice that the weather and date change. The way we've coded this makes it easy to add day objects without changing the information retrieval code. -
Close the test movie and save your work as newsFlash5.fla You have now completed a project in which displayed information is retrieved dynamically from a logical storage structure. The next logical step in a project like this would be to put all of our project's data into a database or text file. The information would then be grabbed and routed into the storage structure that you created. We'll cover loading information in and out of Flash in Lesson 11, Getting Data In and Out of Flash, and Lesson 12, Using XML with Flash. |