You'll find that it's sometimes useful to have actions continually executed while a button is being pressed. A button set up to enable this is known as a continuous-feedback button and scroll buttons (the focus of this exercise) provide a perfect example of such. NOTE The Scrollbar component makes implementing scrolling functionality easy, in many cases. This exercise is intended to show some of logic behind the way scrollbars work, so you can implement your own custom scrolling solutions. If you wanted to scroll through a list of information in a window, you would quickly become frustrated if you had to click a button every time you wanted to make the information scroll up (or down). Far less frustrating is a button that needs to be pressed just once to scroll continuously until it's released. In Flash, you can create this type of functionality by using the enterFrame or mouseMove clip events with a button (as we'll soon demonstrate). In this exercise, you'll add scrolling buttons to the list you built in the previous exercise. -
Open scrollingList2.fla in the Lesson14/Assets folder. You'll pick up where you left off with this file in the previous exercise; thus, you should be familiar with its layout. In this exercise, you'll add ActionScript to Frame 1 of the Actions layer in the main timeline, to the scroll buttons themselves, and to the clips that contain the scroll buttons. First, you'll build the function that performs the actual scrolling; then you'll add the commands to the required areas so that the function is called. You'll remember that there's a movie clip instance called list inside the display clip. All of the attached movie clip instances that we create (of infoBar) will exist inside of the list instance. Thus, we'll set up our scrolling function to vertically move the list movie clip instance up and down to achieve the effect of scrolling through the list of items. NOTE When we use the term scrolling in this exercise, we're talking about increasing or decreasing the _y property value of the list movie clip instance to move it up or down on the screen. -
With the Actions panel open, select Frame 1 of the Actions layer and add the following line of script just below the line that creates the list array: startingY = display.list._y; With scrolling, you must establish maximum and minimum vertical locations (y) to which the list can scroll: These represent the boundaries for scrolling (or continued vertical movement). In any application where scrolling is allowed, you're prevented from scrolling beyond the top or bottom borders of the document. The above line of script is used to establish the edge of one of these vertical boundaries: The movie clip instance list (which will contain the list of attached instances) should not continue scrolling if its y position exceeds its starting y position. In a moment, we'll use the value of the startingY variable to accomplish this. 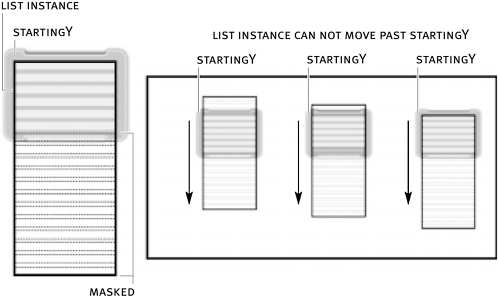 -
To set part of the other scrolling boundary, add bottom=120; to Frame 1, just below the line of script you added in the previous step. As mentioned in the previous step, the highest possible y value that the list movie clip instance can scroll to is its starting position. The bottom variable, as defined in this step, will be used as an offset to the upward extreme of scrolling so that the bottom of the list does not go all the way to the top of the window. (Our reasons for using this variable will become more obvious in coming steps.) -
Start defining the function that will be used to scroll the movie clip instance. To do this, add the following ActionScript to Frame 1, just below the buildList() function definition: function scroll (direction) { speed = 10; } Soon, we'll set up our project to call this function via an enterFrame clip event attached to the movie clip instance that contains the scroll buttons. One of the scroll buttons will be used to scroll up; the other will be used to scroll down. The direction parameter will be used to send a string value of "up" or "down" to this function. A conditional statement in the function (which we'll soon add) will evaluate this value so that the function knows which direction to scroll the list instance. The variable speed is just that the scrolling speed. When the scroll button is held down, the list instance will scroll up or down. Its rate of movement will be determined by the value of this variable. (Keep in mind that there's nothing magic about the number 10; you can adjust it to anything that looks good.) -
Add this if/else if statement inside the function definition, just below speed = 10 : if (direction == "up") { } else if (direction == "down") { } As mentioned in the previous step, the direction parameter is used to send a string value of "up" or "down" to the function. The above conditional statement determines how this function works, based on that value. Over the next few steps, you'll be adding actions to this conditional statement so that if the intended scrolling direction is "up," a certain list of actions will be performed, and if the scrolling direction is "down," another set of actions will be performed. -
Nest this if/else statement in the "up" leg of the if statement just entered: if (display.list._y speed + display.list._height > (startingY + bottom)) { display.list._y -= speed; } else { display.list._y = (startingY + bottom) display.list._height; } Since this statement is nested within the "up" leg of the previous statement, it is evaluated if a string value of "up" is sent to the function (as will occur when the Up scroll button is pressed, as we'll soon set up) to determine if upward scrolling of the list movie clip instance will occur. The first part of the expression in the statement is used to determine what the bottom position of the list instance would be if it were to move 10 pixels upward. The expression does this by looking at the list instance's current y position, then subtracting the value of speed and adding the height of the instance. That bottom position of the instance is then compared against one of the scrolling boundaries (as established by adding the value of bottom to the value of startingY) . If moving the list instance up doesn't cause the bottom of the instance to exceed the boundary, the first action in the statement is executed and the list is moved up. However, if moving the instance up would make it exceed the boundary, the else part of the statement would be executed, simply snapping its vertical position to the maximum allowable. 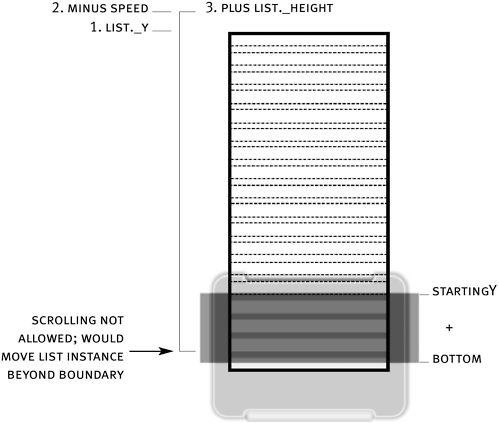 If you're confused, don't worry: Let's take a look at an example to see how this works. For this demonstration, we'll assume the following values for various elements: speed = 10 startingX = 100 bottom = 120 display.list._height = 400 //vertical height of the list instance display.list._y = -165 //current vertical position of list instance Using these values, the expression in the if statement is evaluated as follows: if ( 165 10 + 400 > (100 + 120)) Which further evaluates to: if (225 > 220) In this case, 225 is greater than 220, so the following action is executed: display.list._y -= speed; This moves the list instance up 10 pixels, and its y position now equals -175. With this in mind, if the statement were evaluated again, it would look like the following: if ( 175 10 + 400 > (100 + 120)) Which further evaluates to: if (215 > 220) In this case, 215 is less than 220, so the following action (the else part of the statement) is executed: display.list._y = (startingY + bottom) display.list._height; Here, the list instance's y position is set based on what the expression to the right of the equals sign evaluates to. Using the values established for this example, this expression would be evaluated as follows: (startingY + bottom) display.list._height Which further evaluates to: (100 + 120) 400 Which further evaluates to: 220 400 Which further evaluates to: 180 This means that the list instance would be snapped into a vertical position of -180, placing the bottom of the instance at the edge of the upper scrolling boundary. This statement is set up so that the bottom of the list instance never scrolls beyond this point. NOTE This can be a tricky concept: You may want to review this information several times. Next, let's set up the function to handle downward scrolling. -
Nest this if/else statement in the "down" portion of the outer if/else if statement: if (display.list._y + speed < startingY) { display.list._y += speed; } else { display.list._y = startingY; } 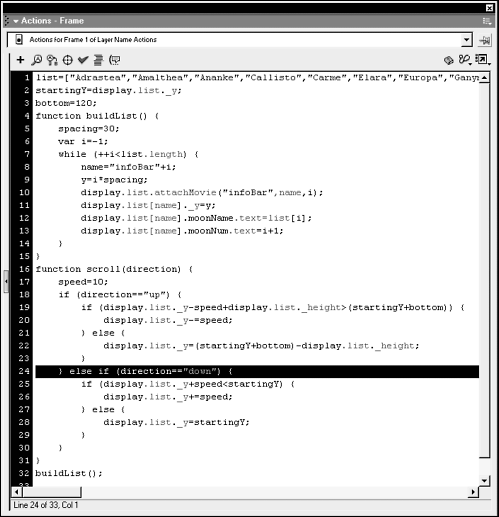 This part of the statement serves to scroll the movie clip instance downward. Similar to the statement in the previous step, this statement looks to see if moving the list instance down by the value of speed (display.list._y + speed ) will keep the instance within the lower scrolling boundary (startingY ). If it does, the first action in the statement is executed, moving the instance down. Otherwise (else ), if moving the instance by the value of speed causes it to exceed the lower boundary, the action within the else part of the statement is executed, snapping the instance into vertical alignment with the lower boundary. Now that you've defined this function, it's time to begin working with the scroll buttons. -
With the library open, double-click the movie clip instance named list arrow in the library window. Included on this movie clip's timeline is the Arrow button that appears in the middle of the screen. As you will soon see, this button (as well as the list arrow movie clip it's part of) will be used for both up and down scrolling. -
With the Actions panel open, select the button and add this script: on (press) { buttonPressed = "yes"; } on (release, releaseOutside, dragOut) { buttonPressed = ""; } This button simply sets the buttonPressed variable to either "yes" or "" based on user interaction. If the user presses the button, buttonPressed is set to "yes" . If the user releases the button, drags out, or releases outside of the button, the buttonPressed variable is set to "" . We'll show you how this variable is used in the next step. -
With the library open, double-click the movie clip named scroll in the library window. This movie clip's timeline includes two instances of the list arrow movie clip that contains the Arrow button to which we added ActionScript in the previous step. One of the instances has been rotated 180 degrees. The top clip has an instance name of down and the bottom one has an instance name of up. You'll remember that the button in this movie clip instance sets a variable named buttonPressed to "yes" when pressed. Note that the two instances of the movie clip in use here are considered separate timelines. Pressing the button in one of the instances will set the variable to "yes" in that timeline only. Pressing the button in the other instance will set the variable to "yes" in that timeline. It will be important that you understand this concept as you go through the following steps. -
With the Actions panel open, select the down movie clip instance and add this script: onClipEvent (enterFrame) { if (buttonPressed == "yes") { _root.scroll("down"); } } Using an enterFrame event, this ActionScript will continuously call (24 times a second) the scroll() function on the root, or main, timeline when the variable buttonPressed (in this timeline) has a value of "yes" . This is the heart of a continuous-feedback button. A Boolean value is set by the button itself here, we've chosen "yes" or "" as our Boolean. As long as buttonPressed has a value of "yes" (which it will as long as the button inside this instance is pressed), scrolling will occur. The parameter of "down" is passed into the scroll() function so that it knows which direction to scroll (as described earlier). -
With the Actions panel still open, select the up movie clip instance and enter the following: onClipEvent (enterFrame) { if (buttonPressed == "yes") { _root.scroll("up"); } } Other than the parameter passed to the scroll() function, "up" , this is identical to the ActionScript created in the previous step. -
Choose Control > Test Movie to test this movie. Give your buttons a try to test your ActionScript. You can press and hold a button down to make scrolling occur. When the list movie clip instance reaches its upper or lower maximum, it will stop scrolling. -
Close the test movie and save your work as scrollingList3.fla. You've now created the ActionScript required to scroll a list of dynamic items between upper and lower boundaries using continuous-feedback buttons. |