17.6. Drawing Lines, Rectangles and Ovals This section presents Graphics methods for drawing lines, rectangles and ovals. Each of the drawing methods has several overloaded versions. Methods that draw hollow shapes typically require as arguments a Pen and four ints. Methods that draw solid shapes typically require as arguments a Brush and four ints. The first two Integer arguments represent the coordinates of the upper-left corner of the shape (or its enclosing area), and the last two ints indicate the shape's (or enclosing area's) width and height. Figure 17.13 summarizes several Graphics methods and their parameters. [Note: Many of these methods are overloadedconsult the online Graphics class documentation for a complete listing (msdn2.microsoft.com/en-us/library/system.drawing.graphics).] Figure 17.13. Graphics methods that draw lines, rectangles and ovals. Graphics Drawing Methods and Descriptions DrawLine(ByVal p As Pen, ByVal x1 As Integer, ByVal y1 As Integer, _ ByVal x2 As Integer, ByVal y2 As Integer) Draws a line from (x1, y1) to (x2, y2). The Pen determines the line's color, style and width. DrawRectangle(ByVal p As Pen, ByVal x As Integer, ByVal y As Integer, _ ByVal width As Integer, ByVal height As Integer) Draws a rectangle of the specified width and height. The top-left corner of the rectangle is at point (x, y). The Pen determines the rectangle's color, style and border width. FillRectangle(ByVal b As Brush, ByVal x As Integer, ByVal y As Integer, _ ByVal width As Integer, ByVal height As Integer) Draws a solid rectangle of the specified width and height. The top-left corner of the rectangle is at point (x, y). The Brush determines the fill pattern inside the rectangle. DrawEllipse(ByVal p As Pen, ByVal x As Integer, ByVal y As Integer, _ ByVal width As Integer, ByVal height As Integer) Draws an ellipse inside a bounding rectangle of the specified width and height. The top-left corner of the bounding rectangle is located at (x, y). The Pen determines the color, style and border width of the ellipse. FillEllipse(ByVal b As Brush, ByVal x As Integer, ByVal y As Integer, _ ByVal width As Integer, ByVal height As Integer) Draws a filled ellipse inside a bounding rectangle of the specified width and height. The top-left corner of the bounding rectangle is located at (x, y). The Brush determines the pattern inside the ellipse. | The application in Fig. 17.14 draws lines, rectangles and ellipses. In this application, we also demonstrate methods that draw filled and unfilled shapes. Figure 17.14. Demonstration of methods that draw lines, rectangles and ellipses. 1 ' Fig. 17.14: FrmLinesRectanglesOvals.vb 2 ' Demonstrating lines, rectangles and ovals. 3 Public Class FrmLinesRectanglesOvals 4 ' override Form OnPaint method 5 Protected Overrides Sub OnPaint(ByVal paintEvent As PaintEventArgs) 6 ' get graphics object 7 Dim g As Graphics = paintEvent.Graphics 8 Dim brush As New SolidBrush(Color.Blue) 9 Dim pen As New Pen(Color.Black) 10 11 ' create filled rectangle 12 g.FillRectangle(brush, 90, 30, 150, 90) 13 14 ' draw lines to connect rectangles 15 g.DrawLine(pen, 90, 30, 110, 40) 16 g.DrawLine(pen, 90, 120, 110, 130) 17 g.DrawLine(pen, 240, 30, 260, 40) 18 g.DrawLine(pen, 240, 120, 260, 130) 19 20 ' draw top rectangle 21 g.DrawRectangle(pen, 110, 40, 150, 90) 22 23 ' set brush to red 24 brush.Color = Color.Red 25 26 ' draw base Ellipse 27 g.FillEllipse(brush, 280, 75, 100, 50) 28 29 ' draw connecting lines 30 g.DrawLine(pen, 380, 55, 380, 100) 31 g.DrawLine(pen, 280, 55, 280, 100) 32 33 ' draw Ellipse outline 34 g.DrawEllipse(pen, 280, 30, 100, 50) 35 End Sub ' OnPaint 36 End Class ' FrmLinesRectanglesOvals 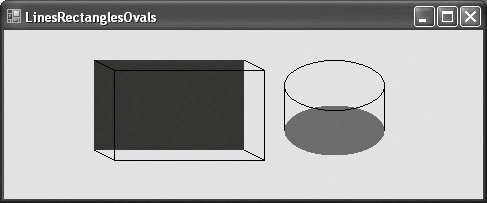 | Methods FillRectangle and DrawRectangle (lines 12 and 21) draw rectangles on the screen. For each method, the first argument specifies the drawing object to use. The FillRectangle method uses a Brush object (in this case, an instance of SolidBrusha class that derives from Brush), whereas the DrawRectangle method uses a Pen object. The next two arguments specify the coordinates of the upper-left corner of the bounding rectangle, which represents the area in which the rectangle will be drawn. The fourth and fifth arguments specify the rectangle's width and height. Method DrawLine (lines 1518) takes a Pen and two pairs of ints, specifying the start and end of a line. The method then draws a line, using the Pen object. Methods FillEllipse and DrawEllipse (lines 27 and 34) each provide overloaded versions that take five arguments. In both methods, the first argument specifies the drawing object to use. The next two arguments specify the upper-left coordinates of the bounding rectangle representing the area in which the ellipse will be drawn. The last two arguments specify the bounding rectangle's width and height, respectively. Figure 17.15 depicts an ellipse bounded by a rectangle. The ellipse touches the midpoint of each of the four sides of the bounding rectangle. The bounding rectangle is not displayed on the screen. Figure 17.15. Ellipse bounded by a rectangle. 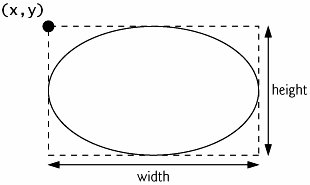 |