Exceptions are classes just like any other class, except that all exceptions must directly or indirectly extend the java.lang.Throwable class. Typically, your custom exceptions will extend one of the java.lang.Throwable class's subclasses: java.lang.Exception. Figure 9.2 shows the inheritance hierarchy of the three main types of exceptions: Exceptions, Errors, and Runtime Exceptions. Figure 9.2. Exception hierarchy class diagram. 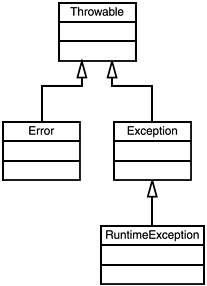 The java.lang.Exception class is used for most exceptions in Java and is the base class for most user-defined exceptions. This class of exception requires that methods throwing it explicitly publish that it can be thrown. It also encompasses all your database exceptions, input- and output-based exceptions, as well as a hoard of other Java exceptions. The java.lang.Error class is another extension of the Throwable class and indicates a serious problem in the system. Most errors are abnormal conditions such as the death of a thread or a problem with the Java Virtual Machine. Methods that throw errors do not have to explicitly publish them; therefore, callers of that method are not required to catch them. The java.lang.RuntimeException class represents a set of errors that can occur during the normal operation of a program, but they are so common that you are not required to explicitly handle them. The best example of a runtime exception is the java.lang.NullPointerException class, which states that you tried to call a method on an object that was not initialized (so the variable was not referencing anything). If you were required to explicitly handle this case, you would have to enclose all statements that ever used objects (all nonprimitive types) in a try block. Java realized that this request was too unreasonable for programmers and saw fit to provide a class of exceptions that can occur normally, but that you are not required to handle. Throwable Class The java.lang.Throwable class provides a familiar set of core methods that its subclasses use. These methods are shown in Table 9.2. Table 9.2. Throwable Class Methods Value | Description |
---|
Throwable fillInStackTrace() | Fills in the execution stack trace | String getLocalizedMessage() | Creates a localized description of this throwable object | String getMessage() | Returns the error message string of this throwable | void printStackTrace() | Prints this throwable, and its backtrace to the standard error stream | void printStackTrace(PrintStream s) | Prints this throwable, and its backtrace to the specified print stream | void printStackTrace(PrintWriter s) | Prints this throwable, and its backtrace to the specified print writer | String toString() | Returns a short description of this throwable object | The two methods that are the most interesting to you as a Java developer are the getMessage() method, because it tells you what circumstances caused the exception to occur, and the printStackTrace() variations. As methods are called, each method call is stored in what is referred to as a call stack. When an exception occurs, the throwable class provides you with a mechanism to review that call stack to help determine the cause of a problem. For example, consider the following code: 1: public class Test { 2: public void methodA() { 3: methodB(); 4: } 5: public void methodB() { 6: methodC(); 7: } 8: public void methodC() { 9: // Do something that causes an exception! 10: } 11: public static void main( String[] args ) { 12: Test test = new Test(); 13: test.methodA(); 14: } 15:} In this sample code, the main() method creates an instance of the Test class and calls its methodA() method. methodA() in turn calls methodB(), which in turn calls methodC(), which throws an exception. At the point of the exception, line 9 of the program, the call stack looks as follows: Line 9: Test.methodC() threw an exception: body of the message Line 6: Test.methodB() called Test.methodC() Line 3: Test.methodA() called Test.methodB() Line 13: main() called Test.methodA(); If you call one of the printStackTrace() methods, you will not only see the message describing the exception, but also the exact path through the code that caused the exception. This is extremely useful because sometimes your exception can occur inside one of the Java classes because of a parameter that you passed it. The context of the exception in and of itself is not useful without knowing how you got there. |