Data types define the storage capacity and usage for a region in memory, but how is that memory accessible? Memory itself has a numerical address associated with it that, when accessed, references its value. If you think of memory linearly starting from 0 and ascending one value per byte you might represent your data as shown in Figure 2.3. Figure 2.3. Data in memory. 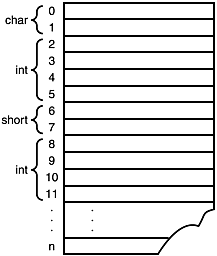 Figure 2.3 shows a simplistic view of how you might have a char, two ints, and a short stored in memory. Consider computing the sum of the two ints, how would you do it? Take the integer value stored in memory location 2 5 and add that to the integer value stored in memory location 8 11. Now let's give them real-world meaning: Call the first integer the students in classroom 1, and the second integer the students in classroom 2. How many students are there in the two classrooms? The answer is the sum of the integer value stored in memory location 2 5, and the integer value stored in memory at location 8 11. Not only is reading that unfriendly, I would venture to say that it is completely unusable! What are we to do? Wouldn't it be nice to associate a meaningful identifier with the portion of memory it refers to? For example we could call the memory location 2 5 classroomA, and the memory location 8 11 classroomB. Then, when asked for the total number of students we could answer with the following: classroomA + classroomB That is much easier to read and far more understandable. In Java when you assign a meaningful identifier to a region of memory, that identifier is called a variable. Furthermore, you do not have to concern yourself with the actual location in memory that variable represents, that task can be delegated to the virtual machine, you only need to specify the data type and the variable name. The act of creating a variable is called declaring a variable, and it has the following form: datatype variableName; The first thing you specify is the data type, which is one of the data types you learned about earlier in this chapter. Next is a meaningful name for your variable; you are free to use any name you want, with the following restrictions: It must start with an alphabetic character (a through z, A through Z, or any Unicode character that denotes a letter in a language), an underscore, or the dollar sign. It can have any alphabetic or numeric value as well as an underscore anywhere in its name. It can be of unlimited length. It cannot have any spaces or non-alphanumeric characters, such as + or -, in it. It cannot be a Java keyword. It is case sensitive (the case of a letter uppercase or lowercase has meaning, for example, myBook is not the same as myBooK. The convention adopted by the Java world in naming variable is to start with a lowercase letter, and if the name has multiple words, capitalize the first letter of every word. For example: thisIsMyVariableName classroomA totalNumberOfStudents Finally, all variable declarations are terminated by a semicolon. As an example consider the following variable declarations: char c; int numberOfStudentsInClassroom1; float bankAccountBalance; After a variable as been declared, a value can be assigned to it. Remember that its data type defines the valid values for a variable. Java defines an assignment operator for assigning values to variables: the equal sign (=). For example: int myAge; myAge = 30; A line of Java code is called a statement, and a semicolon terminates each statement in Java. Another more common method of initializing a variable is to do so during its declaration. For example: int myAge = 30; This statement reads: define a variable named myAge that represents a 4-byte integer in memory and assign it the value 30. |