Encapsulate Composite with Builder Building a Composite is repetitive, complicated, or error-prone. Simplify the build by letting a Builder handle the details. 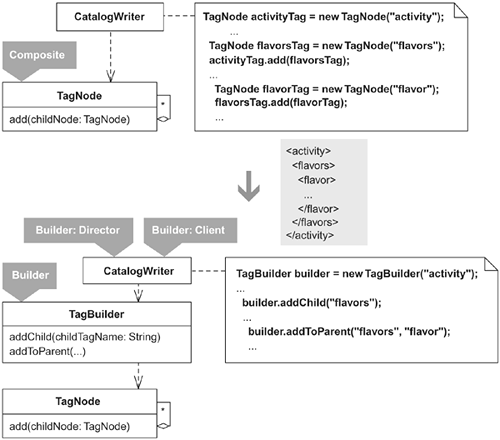 A Builder [DP] performs burdensome or complicated construction steps on behalf of a client. A common motivation for refactoring to a Builder is to simplify client code that creates complex objects. When difficult or tedious parts of creation are implemented by a Builder, a client can direct the Builder's creation work without having to know how that work is accomplished. Builders often encapsulate Composites [DP] because the construction of Composites can frequently be repetitive, complicated, or error-prone. For example, to add a child node to a parent node, a client must do the following: This process is error-prone because you can either forget to add a new node to a parent or you can add the new node to the wrong parent. The process is repetitive because it requires performing the same batch of construction steps over and over again. It's well worth refactoring to any Builder that can reduce errors or minimize and simplify creation steps. Another motivation for encapsulating a Composite with a Builder is to decouple client code from Composite code. For example, in the client code shown in the following diagram, notice how the creation of the DOM Composite, orderTag, is tightly coupled to the DOM's Document, DocumentImpl, Element, and Text interfaces and classes. 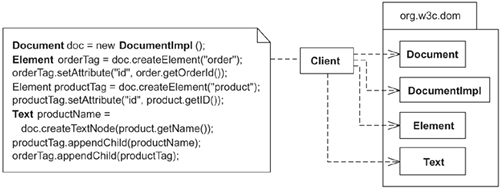 Such tight coupling makes it difficult to change Composite implementations. On one project, we needed to upgrade our system to use a newer version of the DOM, which happened to have several differences from the DOM 1.0 version we had been using. This painful upgrade involved changing many lines of Composite-construction code that were spread across the system. As part of the upgrade, we encapsulated the new DOM code within a DOMBuilder, as shown in the diagram on the following page. 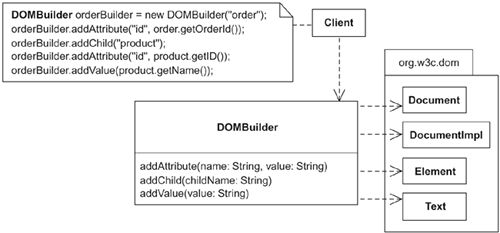 All of the methods on DOMBuilder accept strings as arguments and return void. There is no mention of DOM interfaces or classes in DOMBuilder's interface, yet DOMBuilder internally assembles DOM objects at runtime. Client code that uses a DOMBuilder is loosely coupled to DOM code. That's good, for when future versions of the DOM are released or when we decide to use JDOM or our own TagNode objects, we can easily produce new Builders that feature the identical interface as DOMBuilder. Having one generic Builder interface also enables us to configure our system to use whatever Builder implementation is needed in a given context. The authors of Design Patterns describe the intent of the Builder pattern as follows: "Separate the construction of a complex object from its internal representation so that the same construction process can create different representations" [DP, 97]. While "creat[ing] different representations" of a complex object is a useful service, it isn't the only service a Builder provides. As mentioned earlier, simplifying construction or decoupling client code from a complex object are also equally good reasons for using a Builder. A Builder's interface ought to reveal its intentions so clearly that anyone who looks at it will instantly know what it does. In practice, a Builder's interface, or a portion of it, may not be so clear because Builders do a lot of work behind the scenes to make construction simple. This means that you may need to look at a Builder's implementation or read its test code or documentation to fully understand the features it provides. Benefits and Liabilities + | Simplifies a client's code for constructing a Composite. | + | Reduces the repetitive and error-prone nature of Composite creation. | + | Creates a loose coupling between client and Composite. | + | Allows for different representations of the encapsulated Composite or complex object. | | May not have the most intention-revealing interface. | | Mechanics Because there are numerous ways to write a Builder that builds a Composite, there cannot be one set of mechanics for this refactoring. Instead, I'll offer general steps that you can implement as you see fit. Whatever design you choose for your Builder, I suggest that you use test-driven development [Beck, TDD] to produce it. The following mechanics assume you already have Composite-construction code and you'd like to encapsulate this code with a Builder. - 1. Create a builder, a new class that will become a Builder [DP] by the end of this refactoring. Make it possible for your builder to produce a one-node Composite [DP]. Add a method to the builder to obtain the result of its build.
- 2. Make the builder capable of building children. This often involves creating multiple methods for allowing clients to easily direct the creation and positioning of children.
- 3. If the Composite-construction code you're replacing sets attributes or values on nodes, make the builder capable of setting those attributes and values.
- 4. Reflect on how simple your builder is for clients to use, and then make it simpler.
- 5. Refactor your Composite-construction code to use the new builder. This involves making your client code what is known in Design Patterns as a Builder: Client and Builder: Director.
Example The Composite I'd like to encapsulate with a Builder is called TagNode. This class is featured in the refactoring Replace Implicit Tree with Composite (178). TagNode facilitates the creation of XML. It plays all three Composite roles because the TagNode class is a Component, which can be either a Leaf or a Composite at runtime, as shown in the following diagram. 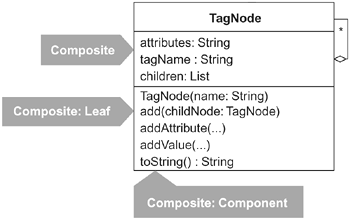 TagNode's toString() method outputs an XML representation of all TagNode objects it contains. A TagBuilder will encapsulate TagNode, providing clients with a less repetitive and error-prone way to create a Composite of TagNode objects. - 1. My first step is to create a builder that can successfully build one node. In this case, I want to create a TagBuilder that produces the correct XML for a tree containing a single TagNode. I begin by writing a failing test that uses assertXmlEquals, a method I wrote for comparing two pieces of XML:
public class TagBuilderTest... public void testBuildOneNode() { String expectedXml = "<flavors/>"; String actualXml = new TagBuilder("flavors").toXml(); assertXmlEquals(expectedXml, actualXml); } Passing that test is easy. Here's the code I write: public class TagBuilder { private TagNode rootNode; public TagBuilder(String rootTagName) { rootNode = new TagNode(rootTagName); } public String toXml() { return rootNode.toString(); } } The compiler and test code are happy with this new code. - 2. Now I'll make TagBuilder capable of handling children. I want to deal with numerous scenarios, each of which causes me to write a different TagBuilder method.
I start with the scenario of adding a child to a root node. Because I want TagBuilder to both create a child node and position it correctly within the encapsulated Composite, I decide to produce one method for doing just that, called addChild(). The following test uses this method: public class TagBuilderTest... public void testBuildOneChild() { String expectedXml = "<flavors>"+ "<flavor/>" + "</flavors>"; TagBuilder builder = new TagBuilder("flavors"); builder.addChild("flavor"); String actualXml = builder.toXml(); assertXmlEquals(expectedXml, actualXml); } Here are the changes I make to pass this test: public class TagBuilder { private TagNode rootNode; private TagNode currentNode; public TagBuilder(String rootTagName) { rootNode = new TagNode(rootTagName); currentNode = rootNode; } public void addChild(String childTagName) { TagNode parentNode = currentNode; currentNode = new TagNode(childTagName); parentNode.add(currentNode); } public String toXml() { return rootNode.toString(); } } That was easy. To fully test that the new code works, I make an even harder test and see if it runs successfully: public class TagBuilderTest... public void testBuildChildrenOfChildren() { String expectedXml = "<flavors>"+ "<flavor>" + "<requirements>" + "<requirement/>" + "</requirements>" + "</flavor>" + "</flavors>"; TagBuilder builder = new TagBuilder("flavors"); builder.addChild("flavor"); builder.addChild("requirements"); builder.addChild("requirement"); String actualXml = builder.toXml(); assertXmlEquals(expectedXml, actualXml); } The code passes this test as well. It's now time to handle another scenarioadding a sibling. Again, I write a failing test: public class TagBuilderTest... public void testBuildSibling() { String expectedXml = "<flavors>"+ "<flavor1/>" + "<flavor2/>" + "</flavors>"; TagBuilder builder = new TagBuilder("flavors"); builder.addChild("flavor1"); builder.addSibling("flavor2"); String actualXml = builder.toXml(); assertXmlEquals(expectedXml, actualXml); } Adding a sibling for an existing child implies that there is a way for TagBuilder to know who the common parent is for the child and its new sibling. There is currently no way to know this, as each TagNode instance doesn't store a reference to its parent. So I write the following failing test to drive the creation of this needed behavior: public class TagNodeTest... public void testParents() { TagNode root = new TagNode("root"); assertNull(root.getParent()); TagNode childNode = new TagNode("child"); root.add(childNode); assertEquals(root, childNode.getParent()); assertEquals("root", childNode.getParent().getName()); } To pass this test, I add the following code to TagNode: public class TagNode... private TagNode parent; public void add(TagNode childNode) { childNode.setParent(this); children().add(childNode); } private void setParent(TagNode parent) { this.parent = parent; } public TagNode getParent() { return parent; } With the new functionality in place, I can refocus on writing code to pass the testBuildSibling() test, listed earlier. Here's the new code I write: public class TagBuilder... public void addChild(String childTagName) { addTo(currentNode, childTagName); } public void addSibling(String siblingTagName) { addTo(currentNode.getParent(), siblingTagName); } private void addTo(TagNode parentNode, String tagName) { currentNode = new TagNode(tagName); parentNode.add(currentNode); } Once again, the compiler and tests are happy with the new code. I write additional tests to confirm that sibling and child behavior works under various conditions. Now I need to handle a final child-building scenario: the case when addChild() or addSibling() won't work because a child must be added to a specific parent. The test below indicates the problem: public class TagBuilderTest... public void testRepeatingChildrenAndGrandchildren() { String expectedXml = "<flavors>"+ "<flavor>" + "<requirements>" + "<requirement/>" + "</requirements>" + "</flavor>" + "<flavor>" + "<requirements>" + "<requirement/>" + "</requirements>" + "</flavor>" + "</flavors>"; TagBuilder builder = new TagBuilder("flavors"); for (int i=0; i<2; i++) { builder.addChild("flavor"); builder.addChild("requirements"); builder.addChild("requirement"); } assertXmlEquals(expectedXml, builder.toString()); } The preceding test won't pass because it doesn't build what is expected. When the loop runs for the second time, the call to the builder's addChild() method picks up where it left off, meaning that it adds a child to the last added node, which produces the following incorrect result: <flavors> <flavor> <requirements> <requirement/> <flavor> Error: misplaced tags <requirements> <requirement/> </requirements> </flavor> </requirements> </flavor> <flavors> To fix this problem, I change the test to refer to a method I call addToParent(), which enables a client to add a new node to a specific parent: public class TagBuilderTest... public void testRepeatingChildrenAndGrandchildren()... ... TagBuilder builder = new TagBuilder("flavors"); for (int i=0; i<2; i++) { builder. addToParent("flavors", "flavor"); builder.addChild("requirements"); builder.addChild("requirement"); } assertXmlEquals(expectedXml, builder.toXml()); This test won't compile or run until I implement addToParent(). The idea behind addToParent() is that it will ask the TagBuilder's currentNode if its name matches the supplied parent name (passed via a parameter). If the name matches, the method will add a new child node to currentNode, or if the name doesn't match, the method will ask for currentNode's parent and continue the process until either a match is found or a null parent is encountered. The pattern name for this behavior is Chain of Responsibility [DP]. To implement the Chain of Responsibility, I write the following new code in TagBuilder: public class TagBuilder... public void addToParent(String parentTagName, String childTagName) { addTo(findParentBy(parentTagName), childTagName); } private void addTo(TagNode parentNode, String tagName) { currentNode = new TagNode(tagName); parentNode.add(currentNode); } private TagNode findParentBy(String parentName) { TagNode parentNode = currentNode; while (parentNode != null) { if (parentName.equals(parentNode.getName())) return parentNode; parentNode = parentNode.getParent(); } return null; } The test passes. Before I move on, I want addToParent() to deal with the case where the name supplied for a parent node does not exist. So I write the following test: public class TagBuilderTest... public void testParentNameNotFound() { TagBuilder builder = new TagBuilder("flavors"); try { for (int i=0; i<2; i++) { builder.addToParent("favors", "flavor"); should be "flavors" not "favors" builder.addChild("requirements"); builder.addChild("requirement"); } fail("should not allow adding to parent that doesn't exist."); } catch (RuntimeException runtimeException) { String expectedErrorMessage = "missing parent tag: favors"; assertEquals(expectedErrorMessage, runtimeException.getMessage()); } } I make this test pass by making the following modifications to TagBuilder: public class TagBuilder... public void addToParent(String parentTagName, String childTagName) { TagNode parentNode = findParentBy(parentTagName); if (parentNode == null) throw new RuntimeException("missing parent tag: " + parentTagName); addTo( parentNode, childTagName); } - 3. Now I make TagBuilder capable of adding attributes and values to nodes. This is an easy step because the encapsulated TagNode already handles attributes and values. Here's a test that checks to see whether both attributes and values are handled correctly:
public class TagBuilderTest... public void testAttributesAndValues() { String expectedXml = "<flavor name='Test-Driven Development'>" + tag with attribute "<requirements>" + "<requirement type='hardware'>" + "1 computer for every 2 participants" + tag with value "</requirement>" + "<requirement type='software'>" + "IDE" + "</requirement>" + "</requirements>" + "</flavor>"; TagBuilder builder = new TagBuilder("flavor"); builder.addAttribute("name", "Test-Driven Development"); builder.addChild("requirements"); builder.addToParent("requirements", "requirement"); builder.addAttribute("type", "hardware"); builder.addValue("1 computer for every 2 participants"); builder.addToParent("requirements", "requirement"); builder.addAttribute("type", "software"); builder.addValue("IDE"); assertXmlEquals(expectedXml, builder.toXml()); } The following new methods make the test pass: public class TagBuilder... public void addAttribute(String name, String value) { currentNode.addAttribute(name, value); } public void addValue(String value) { currentNode.addValue(value); } - 4. Now it's time to reflect on how simple TagBuilder is and how easy it is for clients to use. Is there a simpler way to produce XML? This is not the kind of question you can normally answer right away. Experiments and hours, days, or weeks of reflection can sometimes yield a simpler idea. I'll discuss a simpler implementation in the Variations section below. For now, I move on to the last step.
- 5. I conclude the refactoring by replacing Composite-construction code with code that uses the TagBuilder. I'm not aware of any easy way to do this; Composite-construction code can span large parts of a system. Hopefully you have test code to catch you if you make any mistakes during the transformation. Here's a method on a class called CatalogWriter that must be changed from using TagNode to using TagBuilder:
public class CatalogWriter... public String catalogXmlFor(Activity activity) { TagNode activityTag = new TagNode("activity"); ... TagNode flavorsTag = new TagNode("flavors"); activityTag.add(flavorsTag); for (int i=0; i < activity.getFlavorCount(); i++) { TagNode flavorTag = new TagNode("flavor"); flavorsTag.add(flavorTag); Flavor flavor = activity.getFlavor(i); ... int requirementsCount = flavor.getRequirements().length; if (requirementsCount > 0) { TagNode requirementsTag = new TagNode("requirements"); flavorTag.add(requirementsTag); for (int r=0; r < requirementsCount; r++) { Requirement requirement = flavor.getRequirements()[r]; TagNode requirementTag = new TagNode("requirement"); ... requirementsTag.add(requirementTag); } } } return activityTag.toString(); } This code works with the domain objects Activity, Flavor, and Requirement, as shown in the following diagram.  You may wonder why this code creates a Composite of TagNode objects just to render Activity data into XML, rather than simply asking Activity, Flavor, and Requirement instances to render themselves into XML via their own toXml() method. That's a fine question to ask, for if domain objects already form a Composite structure, it may not make any sense to form another Composite structure, like activityTag, just to render the domain objects into XML. In this case, however, producing the XML externally from the domain objects makes sense because the system that uses these domain objects must produce several XML representations of them, all of which are quite different. A single toXml() method for every domain object wouldn't work well hereeach implementation of the method would need to produce too many different XML representations of the domain object. After transforming the catalogXmlFor(…) method to use a TagBuilder, it looks like this: public class CatalogWriter... private String catalogXmlFor(Activity activity) { TagBuilder builder = new TagBuilder("activity"); ... builder.addChild("flavors"); for (int i=0; i < activity.getFlavorCount(); i++) { builder.addToParent("flavors", "flavor"); Flavor flavor = activity.getFlavor(i); ... int requirementsCount = flavor.getRequirements().length; if (requirementsCount > 0) { builder.addChild("requirements"); for (int r=0; r < requirementsCount; r++) { Requirement requirement = flavor.getRequirements()[r]; builder.addToParent("requirements", "requirement"); ... } } } return builder.toXml(); } And that does it for this refactoring! TagNode is now fully encapsulated by TagBuilder. Improving a Builder I could not resist telling you about a performance improvement made to the TagBuilder because it reveals the elegance and simplicity of the Builder pattern. Some of my colleagues at a company called Evant had done some profiling of our system, and they'd found that a StringBuffer used by the TagBuilder's encapsulated TagNode was causing performance problems. This StringBuffer is used as a Collecting Parameterit is created and then passed to every node in a composite of TagNode objects in order to produce the results returned from calling TagNode's toXml() method. To see how this works, see the example in Move Accumulation to Collecting Parameter (313). The StringBuffer that was being used in this operation was not instantiated with any particular size, which meant that as more and more XML was added to the StringBuffer, it needed to automatically grow when it could no longer hold all its data. That's fine; the StringBuffer class is designed to automatically grow when needed. But there's a performance penalty because StringBuffer must work to transparently increase its size and shift data around. That performance penalty was not acceptable on the Evant system. The solution lay in knowing the exact size the StringBuffer needs to be before instantiating it. How could we compute the appropriate size? Easy. As each node, attribute, or value is added to the TagBuilder, it can increment a buffer size based on the size of what is being added. The final computed buffer size can then be used to instantiate a StringBuffer that never needs to grow in size. To implement this performance improvement, we started as usual by writing a failing test. The following test builds an XML tree by making calls to a TagBuilder, then obtains the size of the resulting XML string returned by the builder, and finally compares the size of that string with the computed buffer size: public class TagBuilderTest... public void testToStringBufferSize() { String expected = "<requirements>" + "<requirement type='software'>" + "IDE" + "</requirement>" + "</requirements>"; TagBuilder builder = new TagBuilder("requirements"); builder.addChild("requirement"); builder.addAttribute("type", "software"); builder.addValue("IDE"); int stringSize = builder.toXml().length(); int computedSize = builder.bufferSize(); assertEquals("buffer size", stringSize, computedSize); } To pass this test and others like it, we altered TagBuilder as follows: public class TagBuilder... private int outputBufferSize; private static int TAG_CHARS_SIZE = 5; private static int ATTRIBUTE_CHARS_SIZE = 4; public TagBuilder(String rootTagName) { ... incrementBufferSizeByTagLength(rootTagName); } private void addTo(TagNode parentNode, String tagName) { ... incrementBufferSizeByTagLength(tagName); } public void addAttribute(String name, String value) { ... incrementBufferSizeByAttributeLength(name, value); } public void addValue(String value) { ... incrementBufferSizeByValueLength(value); } public int bufferSize() { return outputBufferSize; } private void incrementBufferSizeByAttributeLength(String name, String value) { outputBufferSize += (name.length() + value.length() + ATTRIBUTE_CHARS_SIZE); } private void incrementBufferSizeByTagLength(String tag) { int sizeOfOpenAndCloseTags = tag.length() * 2; outputBufferSize += (sizeOfOpenAndCloseTags + TAG_CHARS_SIZE); } private void incrementBufferSizeByValueLength(String value) { outputBufferSize += value.length(); } These changes to TagBuilder are transparent to users of TagBuilder because it encapsulates the new performance logic. The only additional change needed to be made to TagBuilder's toXml() method, so that it could instantiate a StringBuffer of the correct size and pass it to the root TagNode, which accumulates the XML contents. To make that happen, we changed the toXml() method from public class TagBuilder... public String toXml() { return rootNode.toString(); } to public class TagBuilder... public String toXml() { StringBuffer xmlResult = new StringBuffer(outputBufferSize); rootNode.appendContentsTo(xmlResult); return xmlResult.toString(); } That was it. The test passed, and TagBuilder ran significantly faster. Variations A Schema-Based Builder TagBuilder contains three methods for adding nodes to a Composite: -
addChild(String childTagName) -
addSibling(String siblingTagName) -
addToParent(String parentTagName, String childTagName) Each of these methods is involved in creating and positioning new tag nodes within an encapsulated Composite. I wondered if I could write a Builder that would use only a single method, called add(String tagName). To do that, the Builder would need to know where to position tags added by clients. I decided to experiment with this idea. I called the result a SchemaBasedTreeBuilder. Here's a test that shows how it works: public class SchemaBasedTagBuilderTest... public void testTwoSetsOfGreatGrandchildren() { TreeSchema schema = new TreeSchema( "orders" + " order" + " item" + " apple" + " orange" ); String expected = "<orders>" + "<order>" + "<item>" + "<apple/>" + "<orange/>" + "</item>" + "<item>" + "<apple/>" + "<orange/>" + "</item>" + "</order>" + "</orders>"; SchemaBasedTagBuilder builder = new SchemaBasedTagBuilder(schema); builder.add("orders"); builder.add("order"); for (int i=0; i<2; i++) { builder.add("item"); builder.add("apple"); builder.add("orange"); } assertXmlEquals(expected, builder.toString()); } The SchemaBasedTreeBuilder learns where it needs to position tags by means of a TReeSchema instance. treeSchema is a class that accepts a tab-delimited string, which defines a tree of tag names: "orders" + " order" + " item" + " apple" + " orange" treeSchema takes this string and converts it into the following Map: Child | Parent | orders | null | order | orders | item | order | apple | item | orange | item | At runtime, the SchemaBasedTagBuilder uses a treeSchema's map to figure out where to position a new tag. For example, if I write builder.add("orange"), the builder learns from its treeSchema instance that the parent of an "orange" tag is an "item" tag and then proceeds to add a new "orange" tag to the closest "item" tag. This approach works well until you have two tags with the same name: "organization" + " name" + " departments" + " department" + " name" In this case, a treeSchema's map must list two parents for the "name" tag: Child | Parent | … | … | name | organization, department | … | … | At runtime, the SchemaBasedTagBuilder will look up a new tag's parent names, find the closest parent tag, and add the new tag to the closest parent tag. If a client wants to specify exactly which parent to add a new tag to, rather than relying on the closest tag behavior, the client can call an add() method that makes the connection explicit: builder.add("department","name"); // tell builder exactly where to add "name" So that's the SchemaBasedTagBuilder. It accomplishes the same work as TagBuilder but in a different way. I normally use a TagBuilder for building XML; however, given a large XML document to create, I would consider using a SchemaBasedTagBuilder because it would remove the need to worry about where to place tags. In addition, if the large XML document had an XML schema associated with it, I'd likely write code to transform that XML schema into a TReeSchema for use by the SchemaBasedTagBuilder. |