A powerful and capable scripting language, PHP lets you do almost anything, including Code listing 11.6. The world-famous "Hello world" program written in PHP. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <title>PHP Testing</title> </head> <body> <h1>PHP Test</h1> <?php echo "<p>Hello world.</p>\n"; ?> </body> </html> Processing HTML forms Dynamically generating Web pages based on file or database entries Sending email notifications to visitors or the system's administrators Creating an e-commerce site Generating images, PDFs, or XML files on demand This isn't a PHP programming book, though, so for more information about actually doing these things with PHP, check out PHP for the World Wide Web: Visual QuickStart Guide, 2nd Edition (2004), and PHP Advanced for the World Wide Web: Visual QuickPro Guide (2001), both written by Larry Ullman and published by Peachpit Press. To test your PHP installation: We'll create a really trivial PHP program to make sure that PHP is functioning properly. 1. | Log in as root, or use su to become root. If your DocumentRoot directory can be accessed by other users, you might be able to skip this step.
| 2. | cd /var/www/html on Fedora Core, or
cd /usr/local/www/data on FreeBSD, or
cd /usr/local/Apache2/htdocs on Cygwin, or
cd /Library/WebServer/Documents on Mac OS X.
Change to the Apache document directory.
| 3. | Use your favorite text editor to create hello.php (Code Listing 11.6).
Most of Code Listing 11.6 is just the HTML document; only the text between <?php and ?> is actual PHP code.
| 4. | Use your favorite Web browser to access http://hostname/hello.php.
You should see something like what's shown in Figure 11.2.
Figure 11.2. The output of the "Hello world" script in a Web browser. 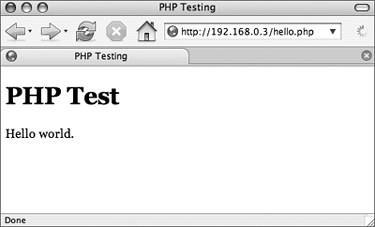
If you view the document's source in your Web browser, you'll see something like what's shown in Figure 11.3. Note how the PHP code is replaced in the document with the arguments of the echo statement (Code Listing 11.6).
Figure 11.3. The document source for the "Hello world" output. The browser doesn't see any PHP code. 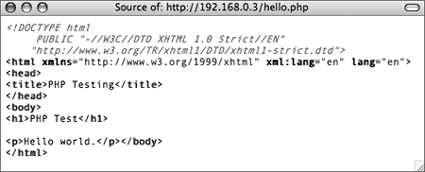
| To use PHP to upload files to the server: A common, simple application for PHP is to let Web-site visitors upload files to the Web server. 1. | Log in as root, or use su to become root. If your DocumentRoot directory can be accessed by other users, you might be able to skip this step.
| 2. | cd /var/www/html on Fedora Core, or
cd /usr/local/www/data on FreeBSD, or
cd /usr/local/Apache2/htdocs on Cygwin, or
cd /Library/WebServer/Documents on Mac OS X.
Change to the Apache document directory.
| 3. | Use your favorite text editor to create upload.html (Code Listing 11.7).
The HTML document in Code Listing 11.7 has no PHP code in it at all, although it does submit the form's contents to validate-upload.php.
Code listing 11.7. The HTML form used to upload a file from a Web browser to the server. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <title>PHP Upload</title> </head> <body> <h1>Upload a file</h1> <p> Enter a full path to the file, or click Browse… to select a file. Click the Send File button to upload the file. </p> <!-- The data encoding type, enctype, MUST be specified as below --> <form enctype="multipart/form-data" action="/validate-upload.php" method="POST"> <!-- MAX_FILE_SIZE must precede the file input field --> <input type="hidden" name="MAX_FILE_SIZE" value="30000" /> <!-- Name of input element determines name in $_FILES array --> Send this file: <input name="userfile" type="file" /> <input type="submit" value="Send File" /> </form> </body> </html> | 4. | Use your favorite text editor to create validate-upload.php (Code Listing 11.8).
This is the PHP code that handles the upload and moves the transferred file from PHP's temporary directory to a more permanent storage location.
If the file isn't moved, it will be deleted at the end of the PHP script.
Code listing 11.8. The PHP script that processes an uploaded file. <?php // Change $uploaddir to the directory for storing uploaded files. $uploaddir = '/tmp/'; $uploadfile = $uploaddir . basename($_FILES['userfile']['name']); // HTML document preamble. echo '<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"'; echo ' "http://w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">'; echo '<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">'; echo '<head>'; echo '<title>PHP Upload Complete!</title>'; echo '</head>'; echo '<body>'; echo '<h1>Upload a file</h1>'; echo '<pre>'; if (move_uploaded_file($_FILES['userfile']['tmp_name'], $uploadfile)) { echo "File is valid, and was successfully uploaded.\n"; } else { echo "Possible file upload attack!\n"; } echo 'Here is some more debugging info:'; print_r($_FILES); print "</pre>"; echo '</body>'; echo '</html>'; ?> | 5. | Use your favorite Web browser to access http://hostname/upload.html.
You should see something like what's shown in Figure 11.4.
Figure 11.4. The upload form in action. 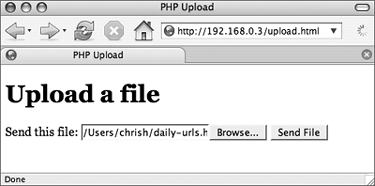
| 6. | Enter the full path to a file in the "Send this file" field, or click the Browse button on the PHP Upload page to select a file using your system's file selector. Click the Send File button to complete the transaction and send the file.
You should see something like what's shown in Figure 11.5.
Figure 11.5. The results of a successful upload. 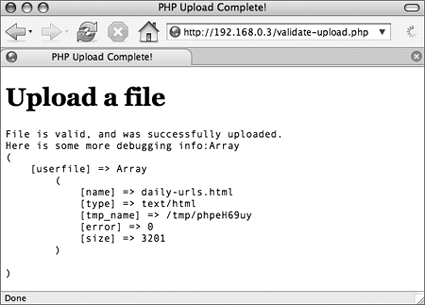
| |