Using the EL Expression Language EL provides two methods for using expressions. We discuss the first method, the EL expression language, next, and cover the RT expression language in the section that follows. The designers of JSTL assumed that most developers will use the EL expression language. An expression using EL must always be delimited by ${ and }. Accessing Properties To access the properties of objects with EL, you should use either the . or [] operator. The . operator is used when you know ahead of time exactly what property you want to access. Listing 4.7 shows both techniques. Listing 4.7 Accessing Properties (prop.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Property Access</title> </head> <body> <c:if test="${pageContext.request.method=='POST'}"> <c:set var="idx" value="name" /> param.name = <c:out value="${param.name}" /> <br /> param[name] = <c:out value="${param[idx]}" /> <br /> </c:if> <br /> <form method="post">Please enter your name? <input type="text" name="name" /> <input type="Submit" /> <br /> </form> </body> </html> Listing 4.7 begins by displaying the name property of the param object. The following line of code does this: param.name = <c:out value="${param.name}" /> We have used this method of property access many times already. The only problem with this approach is that you cannot access a different property at runtime. To dynamically choose the property that you will access, you must use the [] operator. The following lines of code demonstrate this: <c:set var="idx" value="name" /> param[name] = <c:out value="${param[idx]}" /> These lines also access the name property of the params collection. The difference is the variable idx is used to specify what property to use. Figure 4.3 shows the output of this program. 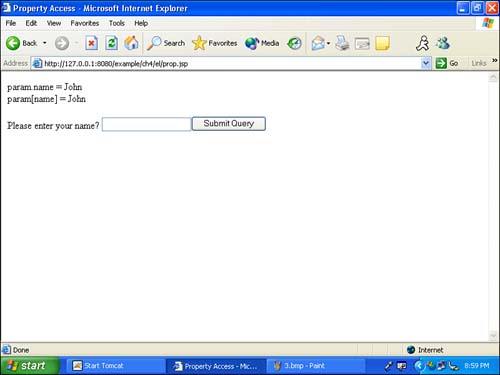 Literals Literals are constant values that you enter into EL code. EL supports four literal types: Boolean | true and false. | Whole | Whole numbers such as 100, just like in Java. | Floating Point | Numbers such as 3.14. Unlike Java, JSTL allows you to use the d and f suffixes to differentiate between Double and Float. | | String-delineated with single or double quotes. | Null | The value null. | It is important to note that an EL expression cannot use the numeric suffixes d and f because they are used in Java to differentiate between Float and Double constants. The following JSP, which uses a <c:set> tag, can use the d suffix since the <c:set> tag is not being given an EL expression: <%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Floats</title> </head> <body> <c:set var="idx" value="3.140d" /> idx = <c:out value="${idx}" /><br /> idx = <c:out value="${idx == 3.14}" /><br /> </form> </body> </html> If this same value were tried with an EL expression, an error would result: <%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Floats</title> </head> <body> <c:set var="idx" value="3.140d" /> idx = <c:out value="${idx}" /><br /> idx = <c:out value="${idx == 3.14d}" /><br /> </form> </body> </html> Strings allow certain characters to be escaped within them. The quote (") in a string is escaped as \". An apostrophe (') is escaped as \'. Finally, the backslash (\) is escaped as \\. Property Access Operators: [] and. EL expressions can access objects; therefore, it is necessary for EL expressions to be able to access the properties that are stored within the objects. EL provides the . and [] operators to do this. Unlike in Java, the . and [] operators have the same meaning in EL. While the meaning of the operators are the same, they are used somewhat differently. The . operator works essentially the same as in Java; it is used to access an individual property in the object. For example, the following line of code would display the p property in the object obj. (In EL, a property can mean several things: It can be a public class scope variable, just as in Java, or it can also mean a getter method. For example, specifying obj.firstName would access the getter getFirstName.) <c:out value="${obj.p}"/> This code allows you to access any property in an object or collection. The [] operator accesses the same property. However, you can put a variable inside the [] operator to allow you to specify which property you are seeking. For example, the following line of code would also display the p property in the object obj: <c:out value="${obj['p']}"/> This may not seem any more useful than the previous line of code. However, this method becomes very powerful when you substitute another variable in the [] operator. Consider the following lines of code, which also display the p property of the object obj: <c:set var="i" value="p"/> <c:out value="${obj[i]}"/> Here, the variable i is used to specify the property that the program should retrieve. This allows your program to determine, at runtime, which property to use. Arithmetic Operators Arithmetic is provided to act on Integer (Long) and Floating Point. These operators will be discussed in this section. Addition, Subtraction, and Multiplication Addition, subtraction, and multiplication are handled by the typical +,-, and * operators. Given the equation of A{+,-,*}B, the following list summarizes the results of this operator: -
If A and B are null, then the result is 0. -
If A or B is Float or Double, then A and B are coerced to Double and the operator is applied. -
If A or B is Byte, Short, Character, Integer, or Long, then A and B are coerced to Long and the operator is applied. -
If the operator results in an exception, then an error is raised. -
Otherwise, an error is raised. It is important to note that the EL plus operator cannot be used to concatenate two strings. If you did have two strings, str1 and str2, the following command would concatenate them into a third variable, named c: <c:set var="c" value="${a}${b}"/> Division Division is handled by the / operator. The textual operator div is also allowed for XPath compatibility. Like all EL operators, the rules for how the division is applied depends on the operands. Given the equation of A/B, the following list summarizes the results of this operator: -
If A and B are null, then the result is 0. -
Otherwise, coerce both A and B to Double and apply the operator. -
If division by zero, then return Infinity. -
If another exception, then raise an error. The Modulo Operator Modulo is handled by the % operator. The textual operator mod also allows for XPath compatibility. As you may recall, modulo is the remainder of a division. For example, 10%3 is 1, because 1 is the remainder of the division between 10 and 3. Like all EL operators, the rules for how the modulus is applied depends on the operands. Given the equation of A%B, the following list summarizes the results of this operator: -
If A and B are null, the result is 0. -
If A or B is Float or Double, coerce both to Double and apply the operator. -
Otherwise, coerce both A and B to Long and apply the operator. If the operator results in an exception, raise an error. The Unary Minus Operator The unary minus operator inverts the sign of a number. Placing the unary minus operator in front of a number let's say 5 results in -5. Given the equation of -A, the following list summarizes the results of this operator: -
If A is null, the result is 0. -
If A is a string and unable to coerce to a Double, raise an error. -
If A is a string, coerce to a Double and apply the operator. -
If A is Byte, Short, Integer, Long, Float, or Double, then retain the type and apply the operator. -
If the operator results in an exception, raise an error. -
Otherwise, raise an error. Relational Operators The relational operators compare two variables to each other. These operators allow such comparisons as equal, not equal, and greater or less than. The relational operators are listed here: -
> and gt, greater than -
< and lt, less than -
<= and le, less than or equal -
>= and ge, greater than or equal Given the equation of A==B, the following summarizes the results of these operators: -
If A==B, and the operator is <= or >=, the result is true. Otherwise, the result is false. -
If A or B is Float or Double, coerce both A and B to Double and apply the operator. -
If A or B is Byte, Short, Character, Integer, or Long, then coerce both A and B to Long and apply the operator. -
If A or B is a string, then coerce both to a string and compare A and B lexically. -
If A is comparable, then if A.compareTo (B) throws an exception, an error is raised; otherwise, the result is returned. -
If B is comparable, then if B.compareTo (A) throws an exception, an error is raised; otherwise, the result is returned. -
Otherwise, raise an error. Equality and Inequality Operators Equality and inequality are handled by the == and != operators. As with all EL operators, the rules for how this operator is applied depends on the operands. Given the equation A==B, the following list summarizes the results of this operator: -
If A==B, then the operator is applied. -
If A or B is null, then return false for == or true for !=. -
If A or B is Float or Double, then coerce both A and B to Double and apply the operator. -
If A or B is Byte, Short, Character, Integer, or Long, then coerce both A and B to Long and apply the operator. -
If A or B is a Boolean, then coerce both A and B to a Boolean and apply the operator. -
If A or B is a string, then coerce both A and B to a string and compare lexically. -
Otherwise, if an error occurs while calling A.equals(B), then raise an error. -
Otherwise, apply the operator to the result of A.equals(B). One unfortunate aspect of the == and != operators is that if a coercion fails, then an exception is thrown. This is somewhat counterintuitive. If the coercion fails, then you would assume that the values are in fact not equal. Logical Operators EL supports the usual complement of logical operators. These include and, or, and not. In this section, we examine these operators. The EL operators support the concept of "short-circuiting," just like the Java programming language. That means that an expression will stop being evaluated as soon as the result can be determined. For example, the and operator requires that both operands be true for the and operator itself to be true. If the first operand is evaluated to be false, then EL already knows that the expression can never be true. In this case, the and operator would return false without evaluating the expression any further. The and and or Operators The and and or operators are two basic logic operators. For an and expression to be true, both operands must be true. For an or to be true, either one or the other operator must be true. For an and to be true, both sides of the operation must be true. -
Attempt to coerce A and B to a Boolean; then return the result and apply the operator. -
If it is not possible to coerce A or B to a Boolean, then raise an error. The Unary Not Operator The unary not operator is handled by the ! and not operators. Given the equation of !A, the operation can be summarized as follows: -
Attempt to coerce A to a Boolean; then return the result and apply the operator. -
If it is not possible to coerce A to a Boolean, then raise an error. Operator Precedence The order of precedence is an important concept in any programming language. With only a few exceptions, the order of precedence in EL closely follows that of Java. The following list summarizes the order of precedence in EL: -
[]. -
() -
- (unary) not ! empty -
* / div % mod -
+ - (binary) -
< > <= >= lt gt le ge -
== != eq ne -
&& and -
|| or Reserved Words The following are the reserved words in EL, and may not be used as identifiers. We have already reviewed most of these. NOTE Many of the reserved words are not currently implemented in EL, but they may be used in the future. Sun has placed them in the reserved word list so they may serve as placeholders for future functionality. -
and -
div -
eq -
false -
ge -
gt -
instanceof -
le -
lt -
mod -
ne -
not -
null -
or -
true Implicit Objects EL provides several predefined objects, called implicit objects, which allow you to access application data that your JSP pages may need. Table 4.2 lists these objects and their purposes. Table 4.2. EL Implicit Objects pageContext | Specifies the PageContext object. | pageScope | Provides access to all page-scoped objects. | requestScope | Provides access to all request-scoped variables. | sessionScope | Provides access to all session-scoped variables. | applicationScope | Provides access to all application-scoped variables. | param | Provides access to all single-value components that were posted from a form. | paramValues | Provides access to all array value components that were posted from a form. | header | Provides access to all single-value HTTP headers. | headerValues | Provides access to all array components that were sent as HTTP headers. | cookie | Provides access to the cookies. | initParam | Used to access XPath initialization parameters. | Type Conversion When programming EL, you do not have direct control over types. When a variable is created in EL, the type is not specified. It is the context in which the variable is used that determines the type. For example, if you assign the value 10 to a variable and then print out that variable, the variable is coerced to be a string for display. If you then add 5 to that variable, the value of that variable will be coerced to a numeric type for the addition. This section summarizes the rules that EL uses in order to properly coerce one type into another. Coerce to a String Nearly any variable should be capable of being coerced to a string. The following summarizes this process when you attempt to coerce the variable A to a string and the situations when you might get an error: -
If A is a string, then the result is A. -
Otherwise, if A is null, then the result is "". -
Otherwise, if A.toString() throws an exception, then raise an error. -
Otherwise, the result is A.toString(). -
Otherwise, if A is a primitive type, return a string as in the Java expression ""+A. Coerce to a Primitive Numeric Type You may encounter numerous problems when you attempt to coerce a string to a primitive numeric variable. The following summarizes this process when you attempt to coerce the variable A to a primitive numeric type and the situations when you might get an error: -
If A is null or "", then the result is 0. -
If A is a character, then convert to Short and apply the following rules: -
If A is a Boolean, then raise an error. -
If A is a number type N, then the result is A. -
If A is a number with less precision than N, then coerce with no error. -
If A is a number with greater precision than N, then coerce with no error. -
If A is a string and N.valueOf(A) throws an exception, then raise an error. -
If A is a string and N.valueOf(A) does not throw an exception, then return it as the result. -
Otherwise, raise an error. Coerce to a Character You may encounter numerous problems when you attempt to coerce to a primitive character as well. The following summarizes the process when you attempt to coerce the variable A to a character and the situations when you might get an error: -
If A is null or "", then the result is 0. -
If A is a character, then the result is A. -
If A is a Boolean, then raise an error. -
If A is a number with less precision than Short, then coerce with no error. -
If A is a number with greater precision than Short, then coerce with no error. -
If A is a string, then the result is A.charAt(0). -
Otherwise, raise an error. Coerce to a Boolean There are a few times when you can coerce a type to a Boolean. The following summarizes this process when you attempt to coerce the variable A to a Boolean and the situations when you might get an error: -
If A is null or "", then the result is A. -
Otherwise, if A is a Boolean, then the result is A. -
Otherwise, if A is a string and Boolean.valueOf(A) throws an exception, then raise an error. -
Otherwise, if A is a string, then the result is Boolean.valueOf(A). -
Coerce to any other type. -
Otherwise, raise an error. |