 Becoming a better LabVIEW programmer means you know how to make applications that are mean and lean. Sure, if you have oodles of RAM and a dual terahertz processor, you may not need to worry about these things very much. But on average computers, critical or real-time applications are going to work better if you follow some simple guidelines for increasing performance and reducing memory consumption. Even if you don't foresee your application needing to conserve memory and processor speed, programmers who never take these issues into account arewell, just plain sloppy. Nobody likes debugging sloppy programs. Curing Amnesia and Slothfulness Let's face itLabVIEW does tend to make applications gobble memory, but you can make the best of it by knowing some tips. Memory management is generally an advanced topic, but quickly becomes a concern when you have large arrays and/or critical timing problems. Read anything you can find about improving LabVIEW performance and saving memory. Here is a summary of the tips: Are you using the proper data types? Extended precision (EXT) floats are fine where the highest accuracy is needed, but they waste memory if a smaller type will do the job. This is especially important where large arrays are involved. Globals use a significant amount of memory and time. Minimize not only the creation of globals, but the amount of times and number of places you read or write to them. Don't use complicated, hierarchical data types (such as an array of clusters of arrays) if you need more memory efficiency and speed. Avoid unnecessary coercion (the gray dots on terminals). Coercion indicates the expected data type is different from the data type wired to the terminalLabVIEW does an astounding job of accepting the data anyway in most cases (polymorphism), but the result is a loss of speed and increased memory usage because copies of the data must be made. This is especially true of large arrays. Figure 17.31. Numeric coercion 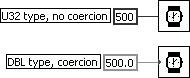 How are you handling arrays and strings? Are you using loops where you don't have to? Sometimes there is a built-in function that can do the job, or several loops can be combined into one. LabVIEW is pretty smart about compiling efficient code, but it's still good practice to watch out for putting unnecessary elements into loops, as shown in Figure 17.32. Figure 17.32. Putting computational work outside of loops, when possible, reduces redundant execution of code. Where possible, avoid using Build Array inside loops, thus avoiding repetitive calls to the Memory Manager. Every time you call the Build Array function, a new space is allocated in memory for the whole "new" array (see Figure 17.33). Use auto-indexing or Replace Array Element with a pre-sized array instead. When auto-indexing in a While Loop, LabVIEW allocates an increasingly bigger chunk of memory than it needs each time it runs out, reducing the number of memory manager calls (see Figure 17.34). In a For Loop, it can calculate and allocate enough memory for all auto-indexed tunnels before it starts iterating (because a For Loop knows how many times it will iterate before it starts) (see Figure 17.35). Or you can do the calculation when you're writing the code, initialize an array to the maximum size or larger than the maximum expected size, and use the Replace Array Subset or Replace Array Element function instead of the Build Array (not shown). Figure 17.33. Constructing an array inside of a While Loop using Build Array is very slow. 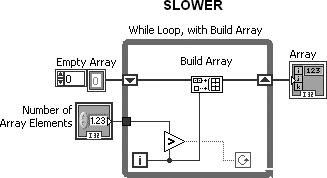 Figure 17.34. Using an auto-indexing tunnel is faster than using Build Array inside a While Loop. 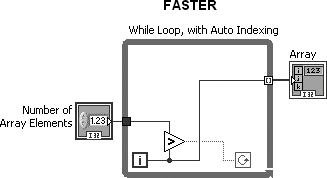 Figure 17.35. Using a For Loop with auto-indexing is faster than a While Loop with auto-indexing. 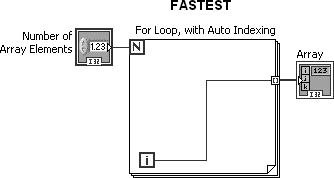 Similar problems occur with Concatenate Strings. Unfortunately, LabVIEW usually can't make very good guesses about the lengths of strings you might choose to concatenate. If you have a decent idea at programming time, initialize a string larger than the maximum size ever expected (allocate an array of U8s and convert it to a string) and use the Replace Substring function (and a final String Subset, to trim off the excess) instead of the Concatenate Strings. Consider indicator overhead. Minimize the use of graphics (especially graphs and charts) when speed is extremely important. Update controls and indicators outside of loops whenever possible; that is, whenever it is not critical to view the object's value until the loop is finished executing. In the Full and Professional versions of LabVIEW, you can use the Show Buffer Allocations window (Tools>>Advanced>>Show Buffer Allocations menu) to see black squares on the block diagram that indicate where LabVIEW creates buffers to hold the data. Because you can see where memory is being allocated, you can try to reduce the memory required by minimizing the buffer allocations. Refer to the Fundamentals>>Managing Performance and Memory>>VI Memory Usage section of the LabVIEW Help documentation for information about how LabVIEW allocates memory and for tips for using memory efficiently. If you really want to peer into the memory consumption of your VIs, you can use the Profile Performance and Memory tool (from the Tools>>Profile>>Performance and Memory menu). You can also use the VI Metrics tool (from the Tools>>Profile>>VI Metrics menu) to see how many nodes, structures, etc. are in your VIs. The Declaration of (Platform) Independence LabVIEW does just an amazing job of porting VIs between different platforms. For the most part, you can take a VI created on any platform (Linux, Mac OS X, or Windows) and run it on another platform (as long they use the same LabVIEW version or a later one). However, not all parts of a block diagram are portable. If you want to design your VIs to be platform-independent, there are a few things you should know about portability: Be aware that the LabVIEW application and everything included with it (such as all the VIs in the vi.lib directory) are not portable. What the LabVIEW for each OS does is to convert VIs from other platforms, using its internal code, to VIs for its own platform. Some of the VIs in the Connectivity palette (like AppleEvents, ActiveX, and .NET) are system-specific and thus are not portable. VIs that contain CINs or Call Library Functions are not immediately portable. But if you write your source code to be platform-independent and recompile it on the new operating system, the CIN should work fine. When calling shared libraries, you can create a shared library for each platform that has the same file name, but a different file extension for each platform (.dll for Windows, .framework for Mac OS X, and .so for Linux). In the "browse" file dialog of the Call Library Function, replace the file extension with an asterisk "*," which will cause LabVIEW to look for a shared library that matches the current system's naming convention. This allows you to use your VIs on all platforms without editing the code. Just ship your VIs along with the platform-specific shared library. Keep in mind such things as filenames that have their own special rules for each OS, and don't use characters such as [:/\], which are path delimiters in different operating systems. Store paths constants as the path data type, rather than the string data type, and LabVIEW will automatically convert the path delimiters. For example, a path constant stored as "..\setup.ini" in Windows will be automatically converted to "../setup.ini" when the VI is run on Linux. If you store your paths as string constants, this conversion will not occur and your code will probably not run correctly. The end of line (EOL) character is different on each platform (\r for Mac OS X, \r\n for Windows, and \n for Linux). The easiest solution is to use LabVIEW's End of Line constant (  ), from the String palette.Fonts can be a real mess when porting between systems. If you can, stick to the three standard LabVIEW font schemes (Application, Dialog, and System) and don't change their sizes, because custom fonts that look fine on one platform may look huge, tiny, or distorted on another. Also, if the Boolean text of a button grows in size, it can cause the button size to grow, as well. You can use system controls, indicators, decorations, and colors (as described in Chapter 13) to create user interfaces that adapt to the style of the operating system and theme of the end user. Screen resolution can also be a nuisance. Some people recommend sticking to using a screen resolution of 1024 x 768, which will make VI windows fit fine on most monitors. Remember, keeping diagrams small means making your block diagrams organized and modularthis is always a good idea! |