8.4. Localize Your Application Note: ASP.NET 2.0 makes globalizing and localizing your web application easy with its new auto-culture handling feature. As global competition heats up, companies are increasingly developing their applications to serve a worldwide audience. One of the challenges in developing a truly global site is in understanding the language and culture of the local audience. An application written for the American market may not be usable in the Asian market. For your application to reach all its potential users, you need to localize it for each audience you plan to serve. Auto-Culture Handling in ASP.NET 2.0 ASP.NET 2.0 comes with the new auto-culture handling feature. Auto-culture handling can be enabled for each page by including the Culture="auto" and UICulture="auto" attributes in the Page directive of each page. Once enabled, ASP.NET will automatically map Accept-Language headers to CultureInfo objects and attach them to the current thread (unlike ASP.NET 1.x, where you need to do this manually). As a developer, you simply need to prepare the resources for the different cultures you want to support. ASP.NET will then do the work of loading the appropriate resources for each culture as they are needed. |
As a developer, you need to be concerned with the following:
- Globalization
-
When designing your application, you plan for all the necessary resources you'll need to enable your application to be modified with ease to suit different cultures.
- Localization
-
You perform the actual transformation to ensure that the user sees the application using the culture he has selected. Localizing an application for a particular audience involves tasks such as the following:
- Properly formatting dates
-
People in the United States represent dates in a different format than someone in, say, the United Kingdom. Does "2/7/2004" represent 2 July 2004, or does it represent February 7, 2004? The answer depends on where you are located.
- Translating content from one language to another
-
For example, the text in your application must change to Chinese if you are targeting the Chinese market.
- Setting text direction
-
Does text read from left to right or from right to left? In this lab, you will learn to use the localization feature in ASP.NET 2.0 and see how it simplifies the tasks you need to perform to create international applications. Localization Basics If you are not familiar with the concept of localization, let's go through some terminologies first: A culture is a way to identify a particular setting pertinent to a location or country. You use a culture code to represent a culture. A neutral culture is a culture that is associated with a language but is not specific to a particular location. For example, "en" is a neutral culture, because it represents the English language but does not provide a specific instance of where it is used. A specific culture is a culture that is specific to a region or country. For example, "en-GB" is a specific culture. Finally, the invariant culture is neither a neutral nor a specific culture. It is English, but is not associated with any location. The invariant culture is used to represent data that is not shown to the user. For example, you use the invariant culture to persist date information to a file. This ensures that the date information would not be misrepresented if is it going to be interpreted in another specific culture.
|
8.4.1. How do I do that? In this lab, you are going to create an application that supports two languages: English and Chinese. You will learn how to prepare the language resources needed so that when the user selects a language preference in IE, the application will display in the language selected by the user. ASP.NET 2.0 supports implicit localization, where the values of controls are checked at runtime against a particular resource file based on the specified culture. Note: In implicit localization, the values of controls are checked at runtime against a particular resource file based on the specified culture. To see how to use implicit localization in ASP.NET 2.0, launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap08-Localization. Populate the default Web Form with the controls shown in Figure 8-10. Apply the "Colorful 1" scheme to the Calendar control (through the Auto Format... link in the Calendar Tasks menu). Figure 8-10. Populating the Default.aspx page 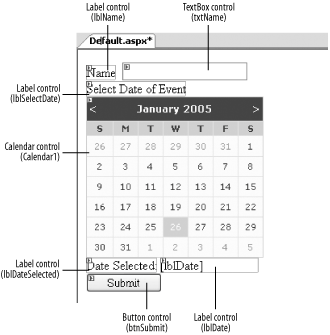
When a date is selected in the Calendar control, display the date in the Label control (lblDate). To do so, double-click the Calendar control and add the following code: Protected Sub Calendar1_SelectionChanged(ByVal sender As Object, _ ByVal e As System.EventArgs) lblDate.Text = Calendar1.SelectedDate.ToShortDateString End Sub Once the form is populated, go to Tools Generate Local Resource and Visual Studio 2005 will generate a resource file containing all the text resources used by your controls (see Figure 8-11). Figure 8-11. Generating the local resource in Visual Studio 2005 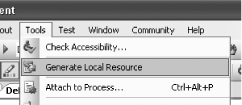
Step 4 causes a new folder named App_LocalResources to be automatically created, and within this folder you will find the resource file generated by Visual Studio 2005. The name of the resource file follows that of the Web Form and ends with a .resx extension (see Figure 8-12). Figure 8-12. The App_LocalResources folder in Solution Explorer 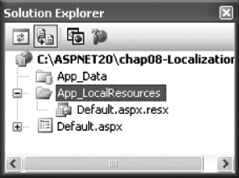
To view the resource file, double-click App_LocalResources to invoke the Resource Editor (see Figure 8-13). Figure 8-13. Invoking the Resource Editor 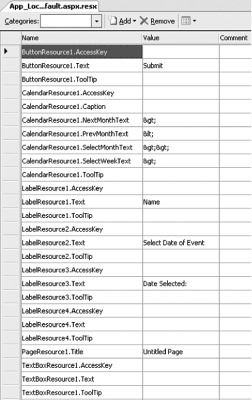
If you now switch the default Web Form to Source View, you will see that each control now has an additional attribute called meta:resourcekey. Its value corresponds to each field in the resource file: ... <asp:Label runat="server" Text="Name" meta:resourcekey="LabelResource1"> </asp:Label> ... <asp:TextBox runat="server" meta:resourcekey="TextBoxResource1"> </asp:TextBox> ... Suppose we want to be able to display this application in both the Chinese and the default English languages. To do so, you'll need to go to Solution Explorer and make a copy of the Default.aspx.resx file (right-click Default.aspx.resx and select Copy, then right-click App_Resources and select Paste). Rename the copy Default.aspx.zh-CN.resx (see Figure 8-14). The culture code must be used as part of the name of the resource file. In general, the resource files are named in the following format: <filename>.aspx.<culturecode>.resx. Figure 8-14. Duplicating the resource file 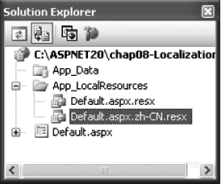
Open the new resource file and add in the values as shown in Figure 8-15 using the Resource Editor. Figure 8-15. The content of the resource file for Chinese culture 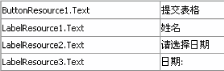
Tip: You can input Chinese characters using Windows, but first you need to install the language pack from the Windows CD. Refer to the section "Where can I learn more?" at the end of this lab to learn how to configure your computer for Chinese Input. Alternatively, you can use any third-party software that allows you to enter Chinese characters.
To use the auto-culture handling feature of ASP.NET 2.0, add the following two attributes into the Page directive (see Figure 8-16): Culture="auto" UICulture="auto" Figure 8-16. The Culture and UICulture attributes 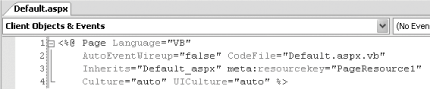
Tip: To apply auto-culture handling at the application level, add the <globalization> element to Web.config:
<system.web> <globalization culture="auto" uiCulture="auto" /> The Culture and UICulture attributes automatically map information received in the Accept-language headers to the CurrentCulture and CurrentUICulture properties of the current thread, thus allowing controls on the page that are culture-aware to localize. To test the application, press F5. You should see the Web Form displayed in English. To display the web application in Chinese, go to Tools Internet Options... (in Internet Explorer) and click the Languages... button. In the Language Preference window (see Figure 8-17), click the Add... button to add a new languagein this case, you should select "Chinese (China) [zh-cn]". Move the desired language to the top of the list and then click OK. Figure 8-17. Changing the language preference in Internet Explorer 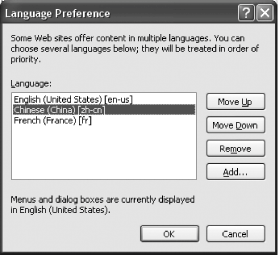
To see the page in Chinese, refresh Internet Explorer. Figure 8-18 shows the page displayed in both English and Chinese. Figure 8-18. Displaying the page in English and Chinese 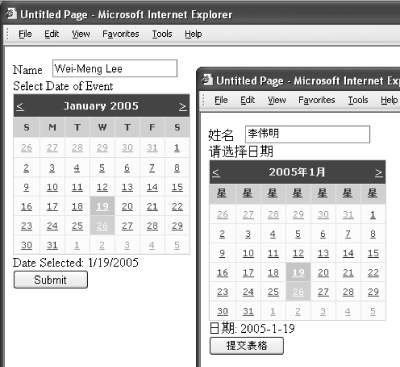 8.4.2. What just happened? At runtime, ASP.NET will automatically detect the culture settings of the requester. Using the culture setting, it then looks for the relevant resource file. If one is found, the values defined in the resource file are used. The Default.aspx.resx resource file is the resource for the default culture. It will also be used if the requester specifies a culture that is not defined in the App_LocalResources folder. It is worth noting that some controls, like the Calendar control, already support localization. In this case, you do not need to do any work on the Calendar control, since it is already able to display the date in Chinese. Also note that the format of the date displayed is different in the English culture and the Chinese culture. 8.4.3. What about... ...explicit Localization? In implicit localization, each Web Form has a separate set of resource files. This method is useful for cases where you need to localize the output of controls. However, it is not feasible when you need to localize large amounts of text or need to constantly reuse them (such as welcome or error messages). In this case, explicit localization is more useful. Explicit localization allows you to define a set of resources that can be used by all the pages in your project. Using the same project created earlier (C:\ASPNET20\chap08-Localization), you will now use explicit localization to store a message written in both English and Chinese. Using the Resources class, the application can then display the message in the appropriate language (based on the preference set in IE). Note: Explicit localization allows you to define a set of resources that can be used by all the pages in your project. To see how explicit localization works, use the same project (C:\ASPNET20\chap08-Localization) and add a new folder named App_GlobalResources (right-click the project name and select Add Folder App_GlobalResources) in Solution Explorer. Add a new resource file to the project by right-clicking on the App_GlobalResources folder and selecting Add New Item.... Select Assembly Resource File (see Figure 8-19). Name the resource file Resource.resx. Make a copy of the resource file and name it Resource.zh-CN.resx. Figure 8-19. Adding a resource file to the project 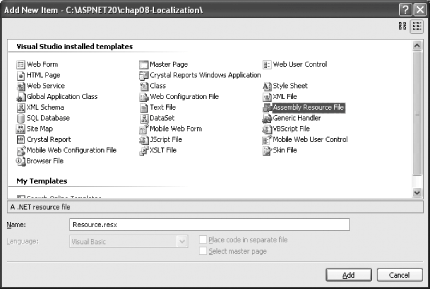
The Solution Explorer should now look like Figure 8-20. Figure 8-20. The new App_GlobalResources folder 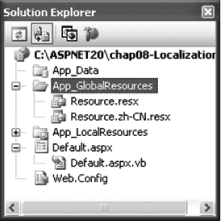
In the Resource.resx file, enter a new key/value pair as shown in Figure 8-21. Likewise, do the same for Resource.zh-CN.resx, but this time the string is in Chinese. Figure 8-21. Creating resource strings 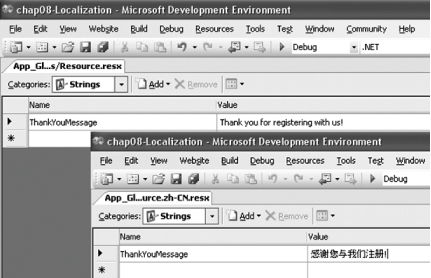
Add the following Click event handler to the Submit button in Default.aspx.vb: Protected Sub btnSubmit_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnSubmit.Click Dim message As String = _ Resources.Resource.ThankYouMessage.ToString( ) Dim script As String = "alert('" & _ message & "');" Page.ClientScript.RegisterClientScriptBlock(Me.GetType, _ "MyKey", script, True) End Sub The Resources class provides a programmatic way to dynamically access the resources located in the resource file. IntelliSense will automatically display the keys defined in the resource file. Press F5 to test the application. Set the language preference in IE to Chinese (see Figure 8-17). When you click on the Submit button (at the bottom of the form), the thank you message is retrieved and then displayed on the client side as a pop-up window (see Figure 8-22), using the egisterClientScriptBlock( ) method. Figure 8-22. Displaying a localized string 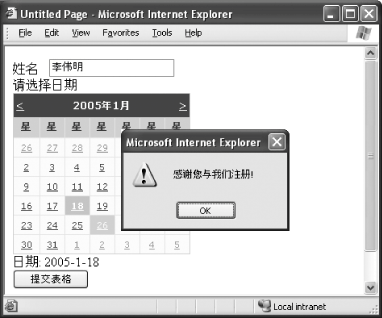 8.4.4. Where can I learn more? If you want to learn more about configuring Windows XP for foreign language input, check out my article on O'Reilly Network: - http://www.ondotnet.com/pub/a/dotnet/2003/09/29/globalization_pt2.html?page=3
Retrieving Values from Resource Files Besides programmatically retrieving values from resource files, you can also do it declaratively. For example, you can bind a Label control's Text property to the resource file by using the Expressions field in the Properties window (see Sidebar 8-6). 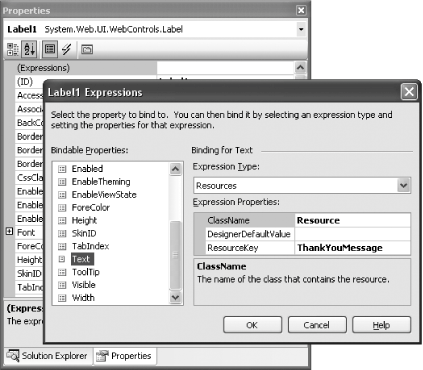 Binding a control to the Resource file Alternatively, you can do this declaratively via the Source View window: <asp:Label Runat="server" Text="<%$ Resources:Resource, ThankYouMessage %>"> </asp:Label> |
|