Normally, a movie's timeline plays in a forward direction at a pace dictated by the fps (frames-per-second) setting in the Movie Properties dialog box. However, you can control the direction in which your timeline moves as well as its speed by using ActionScript. In fact, you'll find that by combining scripting elements (which control direction) with the enterFrame event, you can gain incredible control over your project's timeline. The first two scripting elements we'll discuss here are the nextFrame() and prevFrame() methods of the Movie Clip object. You use these methods to move a timeline to the next or previous frame by employing the following syntax: on(release) { myMovieClip.nextFrame(); } or on(release) { myMovieClip.prevFrame(); } By placing the above scripts on buttons, you can easily create navigation controls that will automatically advance or rewind a timeline a frame at a time with each click of the button. Even more powerful is a timeline's _currentframe property (a read-only property): Its value represents the frame number at which the playhead currently resides. For example, if the main movie were being played, the following script would place the numerical value of the playhead's current frame position into a variable named whereAreWe: whereAreWe = _root._currentframe; 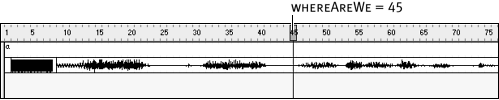 You can also use this property in conjunction with a conditional statement to determine when the playhead is within a specific range of frames and make it act accordingly: onClipEvent (enterFrame) { if(_root._currentframe >= 50 && _root.currentframe <= 100) // Perform these actions } } In the above script, the actions within the conditional statement are only executed when the playhead on the main timeline is between Frames 50 and 100. Using the _currentframe property in conjunction with a gotoAndPlay() action allows you to control the direction in which the timeline moves as well as the pace. Take a look at the following example: _root.gotoAndPlay (_currentframe +10); This will cause the playhead on the main timeline to advance 10 frames from its current position. In the same way, you can use the following script to make the timeline moved backward 10 frames: _root.gotoAndPlay (_currentframe -10); As you will see in this exercise, by using the enterFrame event to execute a line of script like the above, you can create fast-forward and rewind buttons to control a timeline's playback. -
Open makeMyDay3.fla in the Lesson15/Assets folder. We will continue to build on the project from the last exercise. Most of our work for this exercise will take place in the Messages scene. However, before we do anything, we need to give our project the ability to navigate to that scene when it is played which is where the button with the text "Get phone messages" (at the top-left corner of the Alarm scene) comes into play. To do this, we'll place a navigation script on this button. -
With the Actions panel open, select the "Get phone messages" button and add this script: on (release) { nextFrame(); } When the user presses and releases this button, the main timeline will advance to the next frame. Because there's only one frame in this scene, the next frame is actually considered to be the next scene. Thus, this action moves the timeline to the Messages scene, where we will be working next. -
With the Scene panel open, select the Messages scene in the scene list to make it visible in the authoring environment. This scene consists of four layers. The Background layer contains the main graphics. The Buttons layer contains the scene's four buttons: Play, Stop, FF, and Rew. The Flashing Indicator layer contains a movie clip instance of a flashing 4. The Sound Clip layer contains a graphically empty movie clip instance that appears just above the stage, on the left. This instance is named messages; let's take a closer look at it. 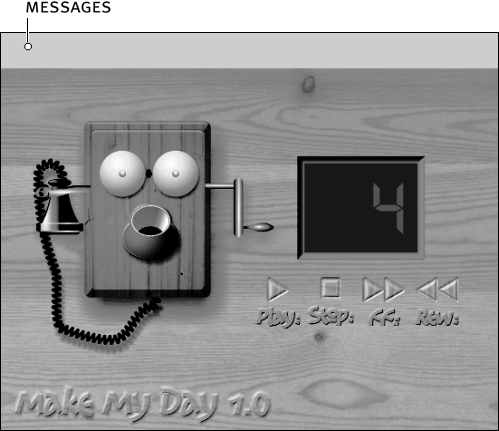 -
Double-click the messages movie clip instance to edit it in place. This movie clip's timeline contains two layers, Sound Clips and Actions. Frame 1 of the Actions layer contains a stop() action to prevent the timeline from moving forward until we instruct it to. The Sound Clips layer has a series of streaming sound clips that stretches across 700 frames. (Dragging the playhead will allow you to hear the various clips.) In this exercise, we'll set up the buttons on the main timeline that the user can employ to navigate this timeline forward and backward. -
Return to the main timeline. With the Actions panel open, select the messages movie clip instance and add the following script: onClipEvent (enterFrame) { if (action == "ff") { gotoAndStop (_currentframe + 3); } else if (action == "rew") { gotoAndStop (_currentframe - 10); } else if (action == "play") { play (); } else if (action == "stop") { stop(); } } This script is executed with an enterFrame event, meaning that the conditional statement is analyzed 24 times per second. This statement is set up to do various things based on the value of a variable named action . As you will soon see, pressing the Play, Stop, FF, and Rew buttons in the scene will change this variable's value accordingly. The first part of this statement says that if action has a value of "ff" (which it will when the FF button is pressed), the current movie (the messages movie clip) should advance three frames from its current frame and stop. Since this script is executed 24 times a second, as long as action equals "ff" (that is, as long as the FF button is pressed), the timeline will continue to advance, always skipping ahead three frames, and simulating the process of fast-forwarding. 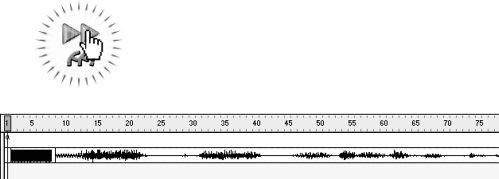 The next part of the statement has just the opposite effect. It says that if action has a value of "rew" (which it will when the Rew button is pressed), the current movie should start at its current frame then go to the frame that's 10 frames back and stop there. Once again, since this script is executed 24 times a second, as long as action equals "rew" (that is, as long as the Rew button is pressed), the playhead on the timeline will continue to move backward, always subtracting 10 more frames from the new current frame, which simulates the process of rewinding. The third part of the statement says that if action has a value of "play" (as it will when the Play button is clicked or the FF or Rew buttons are released), the current movie should play. The last part of the statement says that if action has a value of "stop" (which it will when the Stop button is pressed and released), the current movie should be halted. Next, let's set up our buttons. -
With the Actions panel open, select the Play button and add the following script: on (release) { messages.action = "play"; } When the user presses and releases this button, the value of action (in message's timeline) is set to a value of "play" . This will cause the script attached to that instance to take the appropriate action (as described in the previous step). -
With the Actions panel open, select the Stop button and add the following script: on (release) { messages.action = "stop"; } When the user presses and releases this button, the value of action (in message's timeline) is set to "stop" . This will cause the script attached to that instance to take the appropriate action, as described in the Step 5. -
With the Actions panel open, select the FF button and add this script: on (press) { messages.action = "ff"; fastSound = new Sound(); fastSound.attachSound("rewind"); fastSound.start(0, 50); } on (release) { messages.action = "play"; fastSound.stop(); } This button responds to two events: pressing and releasing. When the user presses this button, the value of action (in message's timeline) is set to "ff" , causing the script attached to that instance to take appropriate action (as described in Step 5). In addition, a Sound object is created to simulate the sound of fast-forwarding (and the "rewind" sound from the library is attached to it), and the sound itself starts, set to loop 50 times. NOTE To avoid confusion, its important to note that the "rewind" sound is used both to simulate the sound of fast-forwarding (here) and rewinding (next step), since they are very similar in nature. 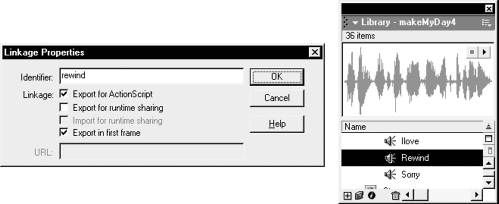 When the button is released, the value of action is set to "play" , thus halting the fast-forwarding process. In addition, the fastSound Sound object is instructed to stop. -
With the Actions panel open, select the Rew button and add this script: on (press) { messages.action = "rew"; fastSound = new Sound(); fastSound.attachSound("rewind"); fastSound.start(0, 50); } on (release) { messages.action = "play"; fastSound.stop(); } This button is set up in exactly the same way as the last one except that when pressed, it sets the value of action to "rew" . -
Choose Control > Test Movie to view the project to this point. When the movie appears, press the "Get phone messages:" button to advance the movie to the next scene. When the scene appears, use the various buttons to control the playback of the messages movie clip instance. Pressing the FF button moves that timeline quickly forward; pressing the Rew button moves it quickly backward (as is evident from the points where the various sound clips in the instance are heard). -
Close the test movie and save your project as makeMyDay4.fla. Playback control buttons like the ones we set up here have a wide range of uses not just for streaming sounds (as demonstrated) but for any kind of project whose timeline contains hundreds of frames of content. By making use of them, you enable users to control the way they view your projects a powerful capability. |