User controls are wonderful features of Web forms. They allow you to package and reuse any UI functionality that you want. Do you need a combination Calendar/DropDownList control to use in your Web application? Simply combine those two controls and wrap them up into a user control. User controls work exactly like server controls, so you can place them wherever you want and you don't have to figure out how they work. Let's examine a typical user control scenario. Imagine you've created a Web site that requires users to log in to access certain sections. Login forms are fairly standard; they contain two text boxes (for username and password information) and a Submit button, as shown in Figure 6.1. You could use two TextBox controls and a Button control, and then wire up the methods to handle those controls. But what if you need this form on several pages? Rather than creating these three controls and their event handlers over and over again, you can simply create a user control that does the exact same thing but only takes up one line of code. Figure 6.1. A typical login form with two text box controls and a Submit button.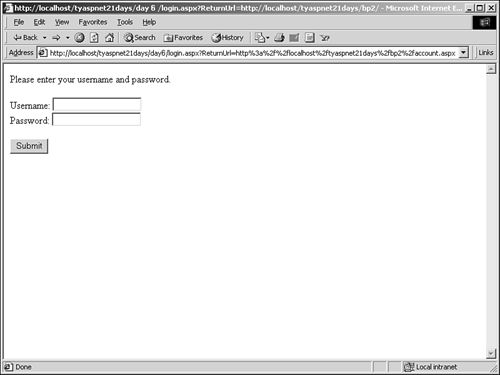 A more complex example is a news portal Web site that displays news from around the Web in subelements on the main page. Since all of these subelements follow the same format but pull data from different sources, they could be implemented as user controls. Each user control wraps up the display functionality, and you can set up each one to retrieve different information. In fact, you can use a single user control and create multiple instances of it, much as you can create multiple instances of text box controls. Of course, user controls don't necessarily have to encapsulate any real functionality. You can build a user control that simply contains a navigation bar with links, or a standard header or footer for your pages. The possibilities are endless. User controls fully support the Web forms framework, so your controls can have properties and events just as built-in controls do. Also, with the .NET Famework, you can author each individual user control in any programming language that supports MSIL (although you can only use one language per control). And best of all, user controls are easy to make. By changing a few simple things, you can convert just about any ASP.NET page you've already built into a user control. Let's take a look at how to accomplish this. Creating User Controls Essentially, a user control is an ASP.NET file with a different extension: .ascx. It contains UI elements and code to control those elements. The difference is that user controls are embedded in other pages, rather than standing alone. Think of a server control the TextBox control, for example. This control displays an HTML text box and provides built-in properties and methods, which it handles itself. It cannot stand alone and must be used within an ASP.NET page. User controls are exactly the same. Let's take a look at a simple user control, shown in Listing 6.1. This control encapsulates the user login form described in the previous section. Listing 6.1 A Typical Login Form Wrapped in a User Control 1: <table style="background-color:<%=BackColor%>; 2: font: 10pt verdana; border-width:1;border-style:solid; 3: border-color:black;" cellspacing=15> 4: <tr> 5: <td><b>Login: </b></td> 6: <td><ASP:TextBox runat="server"/></td> 7: </tr> 8: <tr> 9: <td><b>Password: </b></td> 10: <td><ASP:TextBox TextMode="Password" 11: runat="server"/></td> 12: </tr> 13: <tr> 14: <td></td> 15: <td><ASP:Button Text="Submit" runat="server" 16: OnClick="Submit_Click" /></td> 17: </tr> 18: </table> 19: <p> 20: <ASP:Label runat="server"/>  | Save this listing as LoginForm.ascx. Notice that it looks very similar to the HTML portion of an ASP.NET page. It contains two text box controls, a button control, a label control, and a table to provide formatting. However, it doesn't include any <html>, <form>, or <body> tags. You can't see what this control will look like yet because first it needs to be placed in an ASP.NET page. | Also notice the <%@=BackColor%> on line 1. This looks like a code render block because that's what it is. It sets the background color of the table to the value contained in the variable named BackColor. More on this later. For now, let's examine how to create this user control from an ASP.NET page, shown in Listing 6.2. Listing 6.2 The ASP.NET Page That Your User Control Is Based On 1: <%@ Page Language="VB" %> 2: 3: <script runat="server"> 4: dim Username as string 5: dim Password as string 6: dim BackColor as string 7: 8: sub Submit(Sender as Object, e as EventArgs) 9: lblMessage.Text = "Username: <b>" & User.Text & _ 10: "</b><br>" & "Password: <b>" & Pass.Text & _ 11: "</b><p>" 12: end sub 13: </script> 14: 15: <html><body> 16: <form runat="server"> 17: <table style="background-color:<%=BackColor%>; 18: font: 10pt verdana;border-width:1; 19: border-style:solid;border-color:black;" 20: cellspacing=15> 21: <tr> 22: <td><b>Login: </b></td> 23: <td><ASP:TextBox runat="server"/></td> 24: </tr> 25: <tr> 26: <td><b>Password: </b></td> 27: <td><ASP:TextBox TextMode="Password" 28: runat="server"/></td> 29: </tr> 30: <tr> 31: <td></td> 32: <td><ASP:Button Text="Submit" runat="server" 33: OnClick="Submit" /></td> 34: </tr> 35: </table> 36: <p> 37: <ASP:Label runat="server"/> 38: </form> 39: </body></html>  | You declare the variables Username, Password, and BackColor on lines 4 6. These can be used to store the corresponding information. On line 8, you create the Submit method, which simply displays the supplied information in a label. The HTML portion of the page, beginning on line 15, should look familiar because it's similar to Listing 6.1. Figure 6.2 shows the results when viewed in a browser. | Figure 6.2. The ASP.NET page your user control is based on.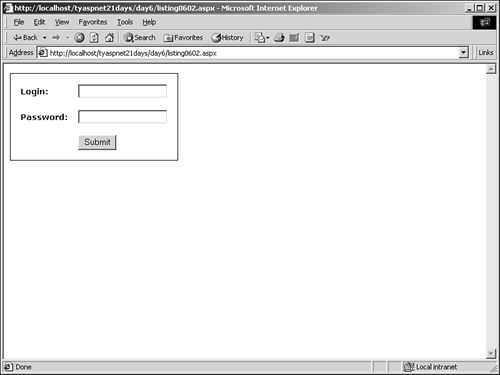 There are three steps to converting an ASP.NET page to a user control. The first step is to remove all <html>, <form>, and <body> tags. Keep all other HTML formatting tags, however. The ASP.NET page that contains the user control will already have these tags, so there's no reason to use them again in your user control. Adding these tags may cause formatting errors or may even cause your application to crash. Secondly, you must rename your file to include an .ascx extension. For example, listing0602.aspx would become listing0602.ascx. The new extension is required in order for the file to be recognized as a user control and not a malformed ASP.NET page. Finally, if the page you're converting includes an @ Page directive, you must change it to an @ Control directive. This directive supports all the same attributes as the @ Page directive, except for tracing. Take the following directive, for example: <%@ Page Language="VB" %> This would become the following: <%@ Control Language="VB" %> After applying these changes to Listing 6.2, you end up with the user control in Listing 6.1. You're nearly ready to use it in any ASP.NET page. Note You don't have to create a user control from an existing ASP.NET page. It was done that way here because it highlights the changes effectively. You can easily create user controls from scratch if you follow these guidelines. So far, this user control encapsulates the UI elements of the login form. This already saves you time and energy if you want to implement this module in an ASP.NET page. User controls can encompass much more functionality, however. They can contain the code to control the UI elements as well. Let's build a code declaration block that exposes all the properties, events, and methods you want this control to have. Listing 6.3 exposes the Username, Password, and BackColor properties from Listing 6.2 and defines a Submit method that handles the OnClick event of the button. Listing 6.3 The User Control Code Declaration 1: <script language="VB" runat="server"> 2: public BackColor as String = "White" 3: public UserName as string 4: public Password as string 5: 6: public sub Submit(Sender as Object, e as EventArgs) 7: lblMessage.Text = "Username: <b>" & User.Text & _ 8: "</b><br>" & "Password: <b>" & Pass.Text & "</b><p>" 9: end sub 10: </script> 11: 12: <table style="background-color:<%=BackColor%>; 13: font: 10pt verdana;border-width:1; 14: border-style:solid;border-color:black;" 15: cellspacing=15> 16: <tr> 17: <td><b>Login: </b></td> 18: <td><ASP:TextBox runat="server"/></td> 19: </tr> 20: <tr> 21: <td><b>Password: </b></td> 22: <td><ASP:TextBox TextMode="Password" 23: runat="server"/></td> 24: </tr> 25: <tr> 26: <td></td> 27: <td><ASP:Button Text="Submit" runat="server" 28: OnClick="Submit" /></td> 29: </tr> 30: </table> 31: <p> 32: <ASP:Label runat="server"/>  | Replace the previous LoginForm.ascx file with the code in this listing. The only addition is the code declaration block on lines 1 10. It's largely similar to the one from Listing 6.2, with a few modifications. On line 2, the BackColor property is initially set to "White", and you define the UserName and Password properties for the user to manipulate. Notice the public keyword; another ASP.NET page can now use these properties programmatically to set or get the values in the UI. | The Submit method is exactly the same as it is in Listing 6.2. Since user controls are very similar to ASP.NET pages, you can simply copy and paste this code. Once this file is saved with an .ascx extension, you're ready to use it in your ASP.NET pages. Using User Controls User controls are used in ASP.NET pages just like other server controls. You create an instance in the UI portion of the page, and you control it programmatically in your code. Because these controls are custom built, however, you need to use some additional syntax. To place user controls onto other ASP.NET pages, you must first register the control on the page with the @ Register page directive. Registering a control effectively tells ASP.NET that you want to extend your Web form with a new server control. This is done so that the page has a valid path and defined name to use for the control. Let's look at the syntax for this directive: <%@ Register TagPrefix="Prefix" TagName="ControlName" src="filepath" Namespace="name" %> The TagPrefix attribute defines the group that your control belongs to. All of the Web server controls you examined yesterday had a TagPrefix of asp. For example: <asp:Button runat="server" /> User controls can have any TagPrefix you desire. Simply specify it in the @ Register directive. The TagName property then gives the user control a name so the page can reference it. For example, your login form user control could use the name LoginForm. The src property specifies the location of the source for the user control. The page must know this path so that it knows what the user control can do. Finally, the Namespace attribute is an optional element that specifies a namespace to associate with the TagPrefix. This helps to further group and classify your user controls. Let's create an ASP.NET page to take advantage of your new login form user control. Listing 6.4 shows an ASP.NET page that includes an @ Register directive and creates an instance of the user control. Listing 6.4 Embedding a User Control in ASP.NET Is Easy 1: <%@ Page Language="VB" %> 2: <%@ Register TagPrefix="TYASPNET" TagName="LoginForm" src="/books/4/226/1/html/2/LoginForm.ascx" %> 3: 4: <script runat="server"> 5: sub Page_Load(Sender as Object, e as EventArgs) 6: lblMessage.Text = "Properties of the user " & _ 7: "control:<br> " & _ 8: "id: " & LoginForm1.id & "<br>" & _ 9: "BackColor: " & LoginForm1.BackColor & "<br>" & _ 10: "Username: " & LoginForm1.Username & "<br>" & _ 11: "Password: " & LoginForm1.Password 12: end sub 13: </script> 14: 15: <html><body> 16: <form runat="server"> 17: <TYASPNET:LoginForm runat="server" 18: Password="MyPassword" 19: Username="Chris" 20: BackColor="Beige" /> 21: </form> 22: <p> 23: <asp:Label runat="server" /> 24: </body></html>  | This page has quite a few interesting features. First, notice the @ Register directive on line 2. You set your prefix to TYASPNET and your control name to LoginForm. You can now reference this control elsewhere with the code, as shown on line 17: | <TYASPNET:LoginForm> The Page_Load event simply displays the user control's properties in the label on line 23. Finally, you implement the control on lines 17 20. The syntax is exactly the same as it is for any other server control. The runat="server" is still required as well. The properties you set on lines 18 20 are the public variables you created on lines 2 4 of Listing 6.3. Also notice the ID of the label on line 23: lblMessage. This is the same ID that you used for the label in the user control. Normally you'd get an error if you placed two controls with the same ID on one page, but this listing seems to work fine. The reason is that ASP.NET doesn't care which controls are used inside the user control or what their IDs are. It only cares that the user control presents a piece of UI functionality that will handle itself; it doesn't need to bother with the details. The public properties of the user control are also exposed to the page, but again, ASP.NET doesn't care what you do with them. It only knows that these properties are available, and the user control will take care of the rest. Because ASP.NET doesn't care about the details, it's impossible to set properties of the controls inside the user control from the ASP.NET page. For example, placing the following code inside Listing 6.4 would result in an error: User.Text = "clpayne" Pass.Text = "helloworld!" Recall that User and Pass refer to controls inside the user control, and ASP.NET doesn't know or care about them. Thus, you need to provide the code to handle your UI elements inside the user control. Figure 6.3 shows this page when viewed in a browser. Figure 6.3. The login form user control presents the appropriate UI display.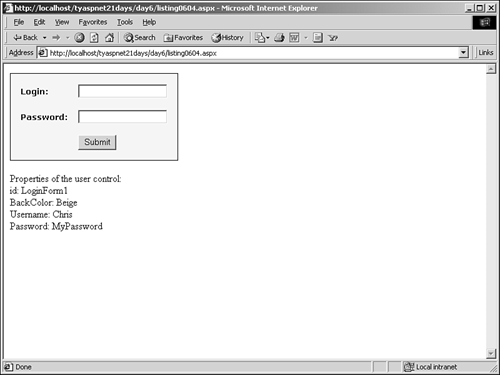 Enter some text into the login form and click the Submit button. The form post is handled, even though you didn't add any code to do so in Listing 6.4. This is because you put the functionality in the user control itself. After you click the Submit button, the user will see what's shown in Figure 6.4. Figure 6.4. The user control handles its own form submissions.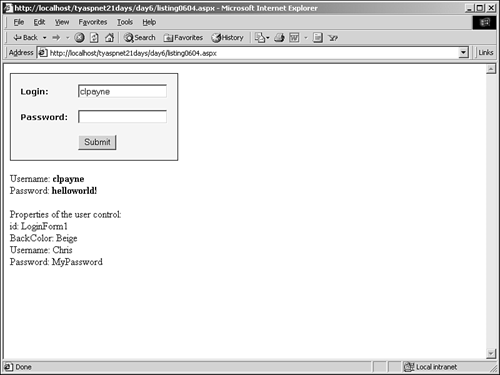 Enhancing the User Control So far, the Username and Password properties of your user control remain unused. When these properties are set, the login form should be prefilled with these values. You cannot use code render blocks as you did for the BackColor property. They are not allowed within server control tags, and an error will be produced. Instead, you'll have to convert the Username and Password public variables into actual VB.NET property elements. Replace lines 3 and 4 of LoginForm.ascx with the code in Listing 6.5. Listing 6.5 Using VB.NET Properties Instead of Public Variables 3: public property UserName as string 4: Get 5: UserName = User.Text 6: End Get 7: Set 8: User.Text = value 9: End Set 10: end property 11: 12: public property Password as string 13: Get 14: Password = Pass.Text 15: End Get 16: Set 17: Pass.Text = value 18: End Set 19: end property  | This is new VB.NET syntax. Essentially, you've transformed public variables into properties of the user control. The difference is that public variables provide very limited functionality; you can only assign and retrieve values. The properties of the user control allow you to perform actions each time the value is set or accessed. | Line 3 looks a lot like what you saw previously: public UserName as string, with the addition of the keyword property. When you use this keyword, you must have a corresponding end property, as shown on line 10. Inside the property tags, you have two additional elements: Get and Set. These elements contain the code which will be used to retrieve and assign values to the property. For example, when a developer writes the following line, the Get statement is actually being called: dim strName as string = LoginForm1.UserName And inversely, the Set statement is called with the following: LoginForm1.UserName = "Chris" Inside these elements, you can add code to perform any functionality that you want to happen when the property is accessed. On line 5, which occurs when the user retrieves the value, you return the value contained in the User text box control by assigning the value to the property itself. On line 8, which occurs when the property value is set, you assign the incoming value to the User text box control. The value keyword refers to the value that the user has assigned to this property. For example, in the following line, value would refer to "Chris": LoginForm1.UserName = "Chris" Tip If you want to create a read or write-only property, simply leave out the Set or Get element and add the keyword ReadOnly or WriteOnly. For example, the following code snippet creates a Username property that can only be read from public ReadOnly property UserName as string Get UserName = User.Text End Get end property Lines 12 19 provide the exact same functionality for the Password property and the Pass text box control. The following code snippet shows the use of the property syntax in C#: 3: public string UserName { 4: get { 5: return User.Text; 6: } 7: set { 8: User.Text = value; 9: } 10: } 11: 12: public string Password { 13: get { 14: return Pass.Text; 15: } 16: set { 17: Pass.Text = value; 18: } 19: } Now request Listing 6.4 from the browser again. You should see the page shown in Figure 6.4. Figure 6.4. Using properties to prefill user control elements.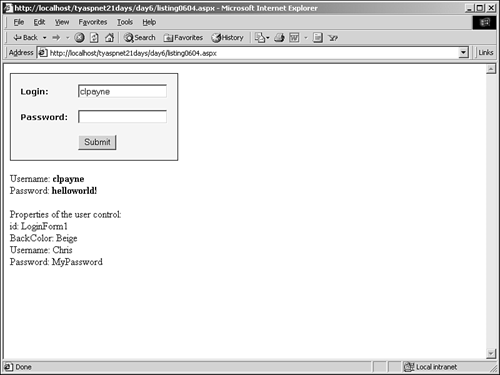 The username field is now prefilled because you set the corresponding property on line 19 of Listing 6.4. Notice that the password field is still empty. This is because that particular text box control has its TextMode property set to Password, and ASP.NET won't fill the text box for security reasons. However, note that the Password property is still assigned the value, as shown in the label below the user control. If you remove the TextMode="Password" code from this text box control, the value will appear. Your user control is now fully functional, with properties that can be manipulated from an ASP.NET page and methods to handle its own events. This control can be placed in any ASP.NET page and will work the same as it does here. |