Processing numerical data is one of the key activities of many computer programs. Mathematical operations determine customer bills, interest due on savings or credit card balances, average scores for class tests, and many other bits of information. Visual Basic supports a number of different math operators that you can use in program statements. These operations and the Visual Basic symbol for each operation are summarized in Table 6.5. The operations are then described in detail in the following sections. Table 6.5. Math Operations and the Corresponding Visual Basic Symbol Operation | Operator |
---|
Addition | + | Subtraction | - | Multiplication | * | Division | / | Integer division | \ | Modulus | Mod | Exponentiation | ^ | In Visual Basic, you use mathematical operations to create equations. These equations can include multiple operators, variables, and expressions, as in the following example: Result = (115 + Val(TextBox1.Text)) / 69 * 1.0825 The preceding line of code assigns the result of the mathematical expression to a variable named Result, which itself may be used in another calculation or displayed on the screen. Addition and Subtraction The two simplest math operations are addition and subtraction. If you have ever used a calculator to do addition and subtraction, you already have a good idea how these operations are performed in a line of computer code. A computer program, however, gives you greater flexibility in the operations you can perform than a calculator does. Your programs are not limited to working with literal numbers (for example, 1, 15, 37.63, -105.2). Your program can add or subtract two or more literal numbers, numeric variables, or any functions that return a numeric value. Also, as with a calculator, you can perform addition and subtraction operations in any combination. Now take a look at exactly how you perform these operations in your program. Using the Addition Operator The operator for addition in Visual Basic is the plus sign (+). The general use of this operator is shown in the following syntax line: result = number1 + number2 [+ number3] result is a variable that contains the sum of the numbers. The equal sign indicates the assignment of a value to the variable. number1 , number2 , and number3 are the literal numbers, numeric variables, or functions that are to be added together. You can add as many numbers together as you like, but each number pair must be separated by a plus sign. Note When assigning the results of an equation to a string, such as a text box, you can enclose the equation in parentheses and use the ToString method to avoid declaring another variable: TextBox2.Text = (1 + 2 + 3).ToString Using the Subtraction Operator The operator for subtraction is the minus sign (-). The syntax is basically the same as for addition: result = number1 - number2 [- number3] Although the order does not matter in addition, in subtraction, the number to the right of the minus sign is subtracted from the number to the left of the sign. If you have multiple numbers, the second number is subtracted from the first, then the third number is subtracted from that result, and so on, moving from left to right. For example, consider the following equation: result = 15 - 6 - 3 The computer first subtracts 6 from 15 to yield 9. It then subtracts 3 from 9 to yield 6, which is the final answer stored in the variable result. Tip You can control the order of operations by using parentheses. For example, the following line of code assigns 12 to the variable result: result = 15 - (6 - 3) You can create assignment statements that consist solely of addition operators or solely of subtraction operators. You can also use the operators in combination with one another or other math operators. The following code lines show a few valid math operations: val1 = 1.25 + 3.17 val2 = 3.21 - 1 val3 = val2 + val1 val4 = val3 + 3.75 - 2.1 + 12 - 3 Note in the previous lines of code we are adding fractional numbers together, so the variables are assumed to be type Double. (By placing a D after the numbers, you could store the result in a Decimal or Double type.) Addition and subtraction can also be combined with other operations, as we'll see in the next section. Multiplication and Division Two other major mathematical operations with which you should be familiar are multiplication and division. Like addition and subtraction, these operations are used frequently in everyday life. Using the Multiplication Operator Multiplication in Visual Basic is straightforward, just like addition and subtraction. You simply use the multiplication operator the asterisk (*) operator to multiply two or more numbers. The syntax of a multiplication statement, which follows, is almost identical to the ones for addition and subtraction: result = number1* number2 [* number3] As before, result is the name of a variable used to contain the product of the numbers being multiplied, and number1 , number2 , and number3 are the literal numbers, numeric variables, or functions. Using the Division Operators Division in VisualBasic is a little more complicated than multiplication. In Listing 6.1, you see how one type of division is used. This division is what you are most familiar with and what you will find on your calculator. This type of division returns a number with its decimal portion, if one is present. However, this type is only one of three different types of division supported by Visual Basic. They are known as floating-point division (the normal type of division, with which you are familiar); integer division; and modulus, or remainder, division. Floating-point division is the typical division that you learned in school. You divide one number by another, and the result is a decimal number. The floating-point division operator is the forward slash (/): result = number1 / number2 [/ number3] 'The following line returns 1.333333 Debug.WriteLine(4 / 3) Integer division divides one number into another and then returns only the integer portion of the result. The operator for integer division is the backward slash (\): result = number1 \ number2 [\ number3] 'The following line returns 1 Debug.WriteLine(4 \ 3) Modulus, or remainder, division divides one number into another and returns what is left over after you have obtained the largest integer quotient possible. The modulus operator is the word mod: result = number1 mod number2 [mod number3] 'The following line returns 2, the remainder when dividing 20 by 3 Debug.WriteLine(20 Mod 3) As with the case of addition, subtraction, and multiplication, if you divide more than two numbers, each number pair must be separated by a division operator. Also, like the other operations, multiple operators are handled by reading the equation from left to right. Figure 6.3 shows a simple form that is used to illustrate the differences between the various division operators. The code for the command button of the form is shown as follows: Figure 6.3. This program demonstrates the difference between Visual Basic's three types of division operators. 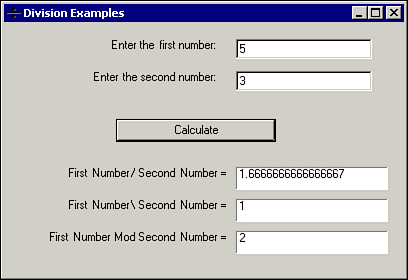 Dim FirstNumber, SecondNumber As Integer FirstNumber = TextBox1.Text.ToInt32 SecondNumber = TextBox2.Text.ToInt32 TextBox3.Text = (FirstNumber / SecondNumber).ToString TextBox4.Text = (FirstNumber \ SecondNumber).tostring TextBox5.Text = (FirstNumber Mod SecondNumber).ToString After you set up the form, run the program, enter 5 in the first text box and 3 in the second text box, and then click the command button. Notice that different numbers appear in each of the text boxes used to display the results. You can try this example with other number combinations as well. Using Multiplication and Division in a Program As a demonstration of how multiplication and division might be used in a program, consider the example of a program to determine the amount of paint needed to paint a room. Such a program can contain a form that allows the painter to enter the length and width of the room, the height of the ceiling, and the coverage and cost of a single can of paint. Your program can then calculate the number of gallons of paint required and the cost of the paint. An example of the form for such a program is shown in Figure 6.4. The actual code to perform the calculations is shown in Listing 6.1. Figure 6.4. Multiplication and division are used to determine the amount of paint needed for a room. 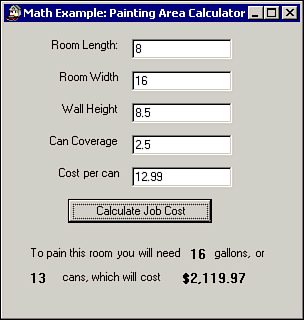 Listing 6.1 MATHEX.ZIP Painting Cost Estimate Using Multiplication and Division Operators Dim RoomLength As Double Dim RoomWidth As Double Dim RoomHeight As Double Dim CanCoverage As Double Dim CanCost As Decimal Dim GalsPerCan As Double Dim RoomPerimeter As Double Dim WallArea As Double Dim NumCans As Double Dim ProjCost As Decimal 'GET INFORMATION FROM TEXT BOXES RoomLength = Val(txtRoomLength.Text) RoomWidth = Val(txtRoomWidth.Text) RoomHeight = Val(txtWallHeight.Text) CanCoverage = Val(txtCoverage.Text) CanCost = Convert.ToDecimal(txtCanCost.Text) GalsPerCan = Val(txtGallons.Text) 'CALCULATE NUMBER OF CANS (ROUND UP) RoomPerimeter = 2 * RoomLength + 2 * RoomWidth WallArea = RoomPerimeter * RoomHeight NumCans = WallArea / CanCoverage lblCansNeeded.Text = Math.Ceiling(NumCans).ToString 'CALCULATE NUMBER OF GALLONS AND COST lblGallonsNeeded.Text = GalsPerCan * NumCans ProjCost = Convert.ToDecimal(Math.Ceiling(NumCans) * CanCost) lblCost.Text = Format(ProjCost, "C") Notice the code in Listing 6.1 introduces the Val function, which converts a string value to a Double. To test this code, start by creating a new EXE project in Visual Basic. Add six text boxes to the form; set their Name properties to txtRoomLength, txtRoomWidth, txtWallHeight, txtGallons, txtCoverage, and txtCanCost. These text boxes are to accept information from the user; use three labels called lblGallonsNeeded, lblCansNeeded, and lb lblCost to report information back to the user. You might want to add descriptive Label controls beside each text box, as in the figure. Next, add a command button to the form. Change its Name property to btnCalculate. Enter the lines of code in Listing 6.1 into the command button's Click event procedure. Save and run the program to see it in action. Exponentiation Exponents are also known as powers of a number. For example, 2 raised to the third power (23) is equivalent to 2x2x2, or 8. Exponents are used quite a lot in computer operations, where many things are represented as powers of two. Exponents are also used extensively in scientific and engineering work, where mathematical terms are often represented as powers of 10 or as natural logarithms. Simpler exponents are used in statistics, where many calculations depend on the squares and the square roots of numbers. To raise a number to a power, you use the exponential operator, which is a caret (^). Exponents greater than one indicate a number raised to a power. Fractional exponents indicate a root, and negative exponents indicate a fraction. The following is the syntax for using the exponential operator: answer = number1 ^ exponent The equations in the following table show several common uses of exponents. The operation performed by each equation is also indicated. Sample Exponent | Function Performed |
---|
3 ^ 2 = 9 | This is the square of the number. | 9 ^ 0.5 = 3 | This is the square root of the number. | 2 ^ 2 = 0.25 | A fraction is obtained by using a negative exponent. | Note You can also use the Math.Pow and Math.Sqrt functions to calculate powers and square roots. Incrementing the Value of a Variable One frequent use of variables is a counter, where you update the same variable with the result of an equation: RocketCountdown = RocketCountdown - 1 If you are not familiar with computer programming, seeing the same variable name appear both on the right and left of the equal sign might look a little strange to you. It tells the program to take the current value of a variable (RocketCoundown), add another number to it, and then store the resulting value back in the same variable. Starting with Visual Basic .NET, new operators have been added to make incrementing and decrementing a variable's value easier. The following lines of code demonstrate how you can save a little typing by using the decrement and assign (-=) operator: RocketCountdown -= 1 Other new operators are available for the other mathematical operations (*=, +=, and so on) which work the same way. Operator Precedence Many expressions contain some combination of the operators just discussed. In such cases, knowing in what order Visual Basic processes the various types of operators is important. For example, what's the value of the expression 4 * 3 + 6 / 2? You might think that the calculations would be performed from left to right. In this case, 4 * 3 is 12; 12 + 6 is 18; 18 / 2 is 9. However, Visual Basic doesn't necessarily process expressions straight through from left to right. It follows a distinct order of processing known as operator precedence. Simply put, Visual Basic performs subsets of a complex expression according to the operators involved, in this order: Exponentiation (^) Negation (-) Multiplication and division (*, /) Integer division (\) Modulus arithmetic (Mod) Addition and subtraction (+, -) Within a subset of an expression, the components are processed from left to right. When all subset groups have been calculated, the remainder of the expression is calculated from left to right. In the previous example (4 * 3 + 6 / 2), the multiplication and division portions (4 * 3, which is 12, and 6 / 2, which is 3) would be calculated first, leaving a simpler expression of 12 + 3, for a total of 15. An important note is that you can override normal operator precedence by using parentheses to group subexpressions that you want to be evaluated first. You can use multiple nested levels of parentheses. Visual Basic calculates subexpressions within parentheses first, innermost set to outermost set, and then applies the normal operator precedence. Built-In Math Functions In addition to the basic mathematical operations already discussed, Visual Basic .NET includes a class devoted entirely to Math, aptly named the Math class. This class is included in the System namespace, so your Visual Basic .NET programs automatically have access to a wide variety of functions, such as the following: Rounding functions such as Round, Ceiling, and Floor allow you to round double or decimal numbers up or down to the next whole number. The comparison methods Max and Min compare two numbers or numeric variables and return the greater or lesser value. The sign-related functions Abs and Sign allow you to determine the absolute value of a number, as well as whether it is positive or negative. Trigonometry functions such as Sin, Cos, and Tan allow you to work with geometric angles. Note that all the functions in the Math class are static, shared methods. This means you do not need to declare an object variable to use them: Dim x As Double = 6.5444 Debug.WriteLine(Math.Floor(x)) 'Returns 6 The math namespace also includes two shared fields, E and PI, which work like constant values. For example, all the trigonometry functions work in radians but you can use PI to convert the value to degrees: Dim AnswerRadians As Double = Math.Asin(0.5) Dim AnswerDegrees As Double = AnswerRadians * (180 / Math.PI) Debug.WriteLine(AnswerDegrees) 'Returns 30 degrees If you didn't fall asleep in math class, you should be able to apply many of the built-in functions to solving equations you already know. For more information on all of the math functions, see the Help file topic Math Members. |