You want to build and deploy a JSP that uses AspectJ into Apache Tomcat.
Create a Java Servlet from your JSP using the tools supplied with Tomcat. Compile your Java Servlet from the command line using the ajc command or inside an Eclipse AspectJ project.
Set up a directory structure from which to deploy your complete JSP application, including the aspectjrt.jar support library and the .class files that were compiled by the ajc command-line tool.
Java Server Pages are trickier than Java Servlets when it comes to using a custom compiler such as AspectJ because a JSP is traditionally compiled into a Java Servlet transparently by the Servlet containers like Apache Tomcat.
The following steps describe how to build and deploy a simple JSP that uses AspectJ into Apache Tomcat:
Create a directory in your project area called jsp.
Create a file in the jsp directory called simple.jsp that has the following contents:
<html> <body bgcolor="white"> <h1> Request Information </h1> <font size="4"> JSP Request Method: <%= request.getMethod( ) %> <br> Request URI: <%= request.getRequestURI( ) %> <br> Request Protocol: <%= request.getProtocol( ) %> <br> Servlet path: <%= request.getServletPath( ) %> <br> </font> </body> </html>
Create a directory in your project area called source to hold your aspect and preprocessed JSP source code.
Create a directory in your project area called myjspapplication and a subdirectory called WEB-INF to hold your finished web application when it is ready for deployment to Tomcat. Also, add classes and lib subdirectories to the WEB-INF directory. Once you've completed these changes your directory structure should look something like what is shown in Figure 3-8.
Figure 3-8. The directory structure after you have created the myjspapplication deployment directory, the source directory, and one .jsp file in the jsp directory
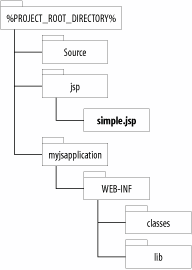
Run the jspc command-line tool that comes with Tomcat from your project area. This will generate the source code Java Servlet that is the first step when precompiling JSPs:
jspc -d source/ -webxml myjspapplication/WEB-INF/web.xml -webapp jsp/
After you have run the jspc command-line tool, you will find a web.xml file in the myjspapplication/WEB-INF directory and a simple_jsp.java file in the source directory.
Create a file called AddCopyrightTomcatJSP.java in the source directory with the code shown in Example 3-4.
Example 3-4. Using an aspect to affect the output of a JSP
import javax.servlet.jsp.JspWriter; public aspect AddCopyrightTomcatJSP { public pointcut captureOutput(String message, JspWriter writer) : call(public void JspWriter.write(String) ) && within(simple_jsp) && args(message) && target(writer) && if (message.equals("</body>\r\n")); before(String message, JspWriter writer) : captureOutput(message, writer) { try { writer.write("<p>Copyleft Russ Miles 2004</p>\n"); } catch (Exception e) { e.printStackTrace( ); } } }
Compile the two files, simple_jsp.java and AddCopyrightTomcatJSP.java, in your source directory using the ajc command-line tool from the project root directory:
ajc -classpath %TOMCAT_INSTALL_DIRECTORY%/common/lib/servlet.jar:%TOMCAT_INSTALL_ DIRECTORY%/common/lib/jasper-runtime.jar -d myjspapplication/WEB-INF/classes source/simple_jsp.java source/AddCopyrightTomcatJSP.java
The result of running this ajc command will be that two .class files will be generated in the myjspapplication/META-INF/classes directory.
Copy the aspectjrt.jar from %ASPECTJ_INSTALL_DIRECTORY%/lib to myweb-application/WEB-INF/lib.
For convenience, create a new file inside mywebapplication called index.html that has the following contents:
<html> <title>Homepage for MyJSPApplication</title> <body> <h1> Testing hompage for aspect-oriented Java Server Pages</h1> <p> The purpose of this page is to simply provide a set of links with which to conveniently access your aspect-oriented Java Server Pages. </p> <p> <ul> <li> <a href="simple.jsp"> The simple aspect-oriented Java Server Page example </a> </li> </ul> </p> </body> </html>
This is an optional step that provides an HTML page that can be used to access your JSP conveniently from the browser without having to enter its specific URL manually.
The final contents of your deployable myjspapplication directory are shown in Figure 3-9.
Figure 3-9. The contents of the myjspapplication directory when it is all set for deployment into Tomcat
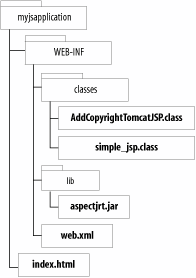
Copy the entire myjspapplication directory and all its contents to %TOMCAT_INSTALL_DIRECTORY%/webapps.
Edit the %TOMCAT_INSTALL_DIRECTORY%/conf/server.xml and add the following lines to enable your web application inside Tomcat:
<Context path="/myjspapplication" docBase="myjspapplication" debug="0" reloadable="true" crossContext="true"> </Context>
Finally, restart Tomcat and you should be able to access your web application and its Java Servlets using your browser by entering a URL similar to :/myjspapplication/index.html">http://<HOSTNAME>:<TOMCAT_PORT>/myjspapplication/index.html and entering the hostname and Tomcat port number for your installation of Tomcat. Figure 3-10 shows the homepage of your new JSP web application.
Figure 3-10. Your JSP web applications homepage
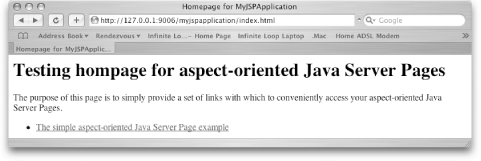
By clicking on the "The simple aspect-oriented Java Server Page example" link on your web applications homepage, your browser should show the output of your JSP, as shown in Figure 3-11.
Figure 3-11. The output from your aspect-oriented Java Server Page
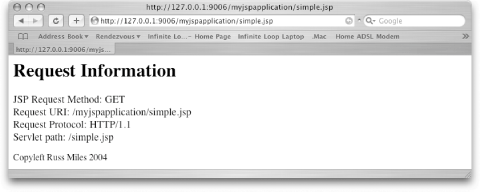
The "Copyleft Russ Miles 2004" message was woven into the output of the JSP using the AddCopyrightJSPTomcat aspect.