Most of the time, the MessageBox.Show() method should be a sufficient means to display messages to a user. At times, however, the MessageBox.Show() method is too limited for a given purpose. Suppose that you want to display a lot of text to a user, such as a log file of some sort, for example, and therefore want a message box that is sizable by the user. Custom dialog boxes are nothing more than standard modal forms with one notable exception: One or more buttons are designated to return a dialog result, just as the buttons on a message box shown with the MessageBox.Show() method return a dialog result. You're now going to create a custom dialog box. Begin by creating a new Windows Application titled Custom Dialog Example and then follow these steps to build the project: 1. | Right-click Form1.cs in the Solution Explorer, choose Rename, and change the name of the default form to frmMain.cs. Next, set the form's Text property to Custom Dialog Box Example.
| 2. | Add a new button to the form and set its properties as follows:
Property | Value |
---|
Name | btnShowCustomDialogBox | Location | 72,180 | Size | 152,23 | Text | Show Custom Dialog Box |
| 3. | Next, you're going to create the custom dialog box. Add a new form to the project by choosing Project, Add Windows Form from the menu. Save the new form with the name fclsCustomDialogBox.cs.
| 4. | Change the Text property of the new form to This is a custom dialog box, and set its FormBorderStyle to FixedDialog.
| | | 5. | Add a new text box to the form and set its properties as follows:
Property | Value |
---|
Name | txtCustomMessage | Location | 8,8 | Multiline | True | ReadOnly | True | Size | 275,220 | Text | Custom message goes here |
For a custom dialog box to return a result like a standard message box does, it must have buttons that are designated to return a dialog result. This is accomplished by setting the DialogResult property of a button (see Figure 17.6).
Figure 17.6. The DialogResult property determines the return value of the button. 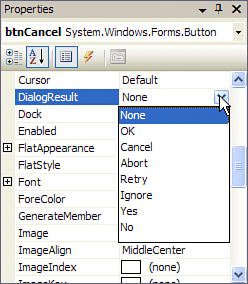 | 6. | Add a new button to the form and set its properties as shown in the following table. This button will act as the custom dialog box's Cancel button.
Property | Value |
---|
Name | btnCancel | DialogResult | Cancel | Location | 216,240 | Size | 75,23 | Text | Cancel |
| | | 7. | You need to create an OK button for the custom dialog box. Create another button and set its properties as follows:
Property | Value |
---|
Name | btnOK | DialogResult | OK | Location | 135,240 | Size | 75,23 | Text | OK |
Specifying a dialog result for one or more buttons is the first step in making a form a custom dialog box. The second part of the process is in how the form is shown. As you learned in Hour 5, "Building FormsThe Basics," forms are displayed by calling the Show() method of a form variable. However, to show a form as a custom dialog box, you call the ShowDialog() method instead. When a form is displayed using ShowDialog(), the following occurs:
The form is shown modally. If the user clicks a button that has its DialogResult property set to return a value, the form is immediately closed, and that value is returned as the result of the ShowDialog() method call. Notice how you don't have to write code to close the form; clicking a button with a dialog result closes the form automatically. This simplifies the process of creating custom dialog boxes.
| 8. | Return to the first form in the Form Designer by double-clicking frmMain.cs in the Solution Explorer.
| 9. | Double-click the button you created and add the following code:
fclsCustomDialogBox frmCustomDialogBox = new fclsCustomDialogBox(); if (frmCustomDialogBox.ShowDialog() == DialogResult.OK) MessageBox.Show("You clicked OK."); else MessageBox.Show("You clicked Cancel."); frmCustomDialogBox = null; | When you typed the equal sign and then a space after the word ShowDialog(), did you notice that Visual C# gave you an IntelliSense drop-down list with the possible dialog results? These results correspond directly with the values you can assign a button using the DialogResult property. Press F5 to run the project, click the button to display your custom dialog box (see Figure 17.7), and then click one of the available dialog box buttons. When you're satisfied that the project is working correctly, stop the project and save your work. Figure 17.7. The ShowDialog() method enables you to create custom message boxes. 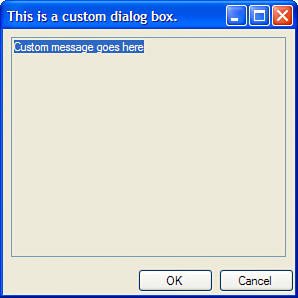 By the Way If you click the Close (X) button in the upper-right corner of the form, the form is closed, and the code behaves as if you've clicked Cancel because the else code occurs. The ability to create custom dialog boxes is a powerful feature. A call to MessageBox.Show() is usually sufficient, but when you need more control over the appearance and contents of a message box, creating a custom dialog box is the way to go. |