 | TOOL KITGenerating a Dateline This JavaScript function comes in two parts. The main script goes between script tags in the head section of your HTML file or in a separate JS file. You need an additional script tag inside the body of your Web page wherever you want the dateline to display. You'll get to that momentarily. First, here is the main script: [View full width] function doDateline() { /* First, get the current date. JavaScript's Date object does just that. This line of code gets the current date and puts it in a variable called now. */ var now = new Date(); /* The following lines extract the day of the week, the month, the calendar date, and the year from the now variable. These values are all numerical. */ var day = now.getDay(); var month = now.getMonth(); var date = now.getDate(); var year = now.getYear(); /* Internet Explorer returns the correct year, while Netscape and Opera are off by exactly 1900 years. The following if/then block makes sure everyone's in the right century. */ if (year < 2000) { year += 1900; } /* Initialize the dateline variable. This variable will eventually contain the text of the dateline. */ var dateline = ""; /* Now build the text of the dateline. The day of the week currently exists in numerical form, from 0 to 6 representing Sunday through Saturday. The following if/then blocks add the correct name of the day to the dateline variable depending on the numerical value in the day variable. Notice the comma and the trailing space after the name of the day . You need the space so that the next item, the month, doesn't appear squished up against the comma. If you want a simple dateline, such as 12/22/2005, omit this block of code. */ if (day == 0) { dateline = "Sunday, "; } if (day == 1) { dateline = "Monday, "; } if (day == 2) { dateline = "Tuesday, "; } if (day == 3) { dateline = "Wednesday, "; } if (day == 4) { dateline = "Thursday, "; } if (day == 5) { dateline = "Friday, "; } if (day == 6) { dateline = "Saturday, "; } /* The month also exists in numerical form, with 0 for January, 1 for February, 2 for March, and so on, until you get to 11 for December. These if/then blocks append the correct month name to the dateline variable. Notice again the trailing space for proper formatting. If you want to use European style for your dateline, switch the order of this block and the next block. If you want a simple dateline, replace this block of code with the following line: dateline += (month + 1) + "/"; */ if (month == 0) { dateline += "January "; } if (month == 1) { dateline += "February "; } if (month == 2) { dateline += "March "; } if (month == 3) { dateline += "April "; } if (month == 4) { dateline += "May "; } if (month == 5) { dateline += "June "; } if (month == 6) { dateline += "July "; } if (month == 7) { dateline += "August "; } if (month == 8) { dateline += "September "; } if (month == 9) { dateline += "October "; } if (month == 10) { dateline += "November "; } if (month == 11) { dateline += "December "; } /* The next line appends the numerical date to the dateline and adds a comma character followed by a space. For European formatting, switch this block with the preceding one , delete the comma and space, and add the comma after the name of each month in the preceding block of code. If you want a simple dateline, replace this line with the following: dateline += date + "/"; */ dateline += date + ", "; /* The next line appends the numerical year to the dateline. */ dateline += year; /* Your dateline is ready for display. This line writes the dateline to the page. */ document.write(dateline); }
So much for the main script. Now, in the place where you want the dateline to appear on your page, add the following code: <script language="JavaScript">doDateline();</script>
If you prefer, you may mark up this script tag as a paragraph, header, or any other type of text element: <p><script language="JavaScript">doDateline();</script></p>
Put the main script together with the doDateline callout, and you get something that looks like Figure 55.1. The simple version looks like Figure 55.2. Figure 55.1. Add a dateline to any Web page. 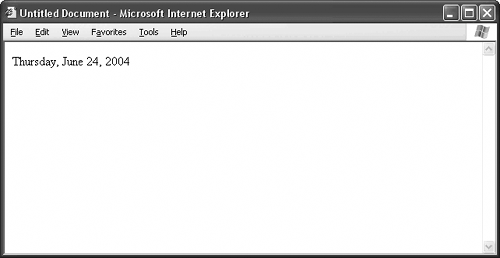
Figure 55.2. If you prefer, you can create a simple dateline. 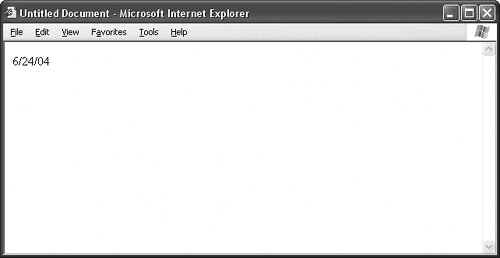
|