Putting It All Together We close out this chapter with an example of a poor man's implementation of a tabbed pane. That example demonstrates event handling and advanced aspects of using JSF HTML tags. Those advanced uses include the following: -
Nesting h:panelGrid tags -
Using facets -
Specifying tab indexing -
Adding tooltips to components with the title attribute -
Dynamically determining style classes -
Using action listeners -
Optional rendering -
Statically including JSF pages JSF 1.2 does not have a tabbed pane component, so if you want a tabbed pane in your application, you have two choices: implement a custom component or use existing tags with a backing bean to create an ad hoc tabbed pane. Figure 7-11 shows the latter. The former is discussed in "Using Child Components and Facets" on page 408 of Chapter 9. 4 The tabbed pane shown in Figure 7-11 is implemented entirely with existing JSF HTML tags and a backing bean; no custom renderers or components are used. The JSF page for the tabbed pane looks like this: ... <h:form> <%-- Tabs --%> <h:panelGrid style columnClasses="displayPanel"> <f:facet name="header"> <h:panelGrid columns="4" style> <h:commandLink tabindex="1" title="#{tp.jeffersonTooltip}" style actionListener="#{tp.jeffersonAction}"> <h:outputText value="#{msgs.jeffersonTab}"/> </h:commandLink> ... </h:panelGrid> </f:facet> <%-- Main panel --%> <%@ include file="jefferson.jsp" %> <%@ include file="roosevelt.jsp" %> <%@ include file="lincoln.jsp" %> <%@ include file="washington.jsp" %> </h:panelGrid> </h:form> ... The tabbed pane is implemented with h:panelGrid. Because we do not specify the columns attribute, the panel has one column. The panel's header defined with an f:facet tag contains the tabs, which are implemented with another h:panelGrid that contains h:commandLink tags for each tab. The only row in the panel contains the content associated with the selected tab. When a user selects a tab, the associated action listener for the command link is invoked and modifies the data stored in the backing bean. Because we use a different CSS style for the selected tab, the styleClass attribute of each h:commandLink tag is pulled from the backing bean with a value reference expression. As you can see from the top picture in Figure 7-11, we have used the title attribute to associate a tooltip with each tab. Another accessibility feature is the ability to move from one tab to another with the keyboard instead of the mouse. We implemented that feature by specifying the tabindex attribute for each h:commandLink. The content associated with each tab is statically included with the JSP include directive. For our application, that content is a picture and some text, but you could modify the included JSF pages to contain any set of appropriate components. Notice that even though all the JSF pages representing content are included, only the content associated with the current tab is rendered. That is achieved with the rendered attribute for example, jefferson.jsp looks like this: <h:panelGrid columns="2" columnClasses="presidentDiscussionColumn" rendered="#{tp.jeffersonCurrent}"> <h:graphicImage value="/images/jefferson.jpg"/> <h:outputText value="#{msgs.jeffersonDiscussion}" style/> </h:panelGrid> Figure 7-12 shows the directory structure for the tabbed pane application and Listing 7-20 through Listing 7-28 show those files. 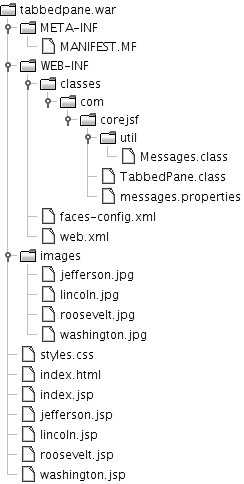 Note | The JSF reference implementation contains a samples directory that holds a handful of sample applications. One of those applications, contained in jsf-components.war, is a tabbed pane component. Although the sample components are provided largely as proof-of-concept, you may find them useful as a starting point for your own custom components. | Listing 7-20. tabbedpane/web/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. 5. <f:view> 6. <head> 7. <link href="styles.css" rel="stylesheet" type="text/css"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. 13. <body> 14. <h:form> 15. <h:panelGrid style columnClasses="displayPanel"> 16. <%-- Tabs --%> 17. 18. <f:facet name="header"> 19. <h:panelGrid columns="5" style> 20. 21. <h:commandLink tabindex="1" 22. title="#{tp.jeffersonTooltip}" 23. style 24. actionListener="#{tp.jeffersonAction}"> 25. 26. <h:outputText value="#{msgs.jeffersonTabText}"/> 27. </h:commandLink> 28. 29. <h:commandLink tabindex="2" 30. title="#{tp.rooseveltTooltip}" 31. style 32. actionListener="#{tp.rooseveltAction}"> 33. 34. <h:outputText value="#{msgs.rooseveltTabText}"/> 35. </h:commandLink> 36. 37. <h:commandLink tabindex="3" 38. title="#{tp.lincolnTooltip}" 39. style 40. actionListener="#{tp.lincolnAction}"> 41. 42. <h:outputText value="#{msgs.lincolnTabText}"/> 43. </h:commandLink> 44. 45. <h:commandLink tabindex="4" 46. title="#{tp.washingtonTooltip}" 47. style 48. actionListener="#{tp.washingtonAction}"> 49. 50. <h:outputText value="#{msgs.washingtonTabText}"/> 51. </h:commandLink> 52. </h:panelGrid> 53. </f:facet> 54. 55. <%-- Tabbed pane content --%> 56. 57. <%@ include file="washington.jsp" %> 58. <%@ include file="roosevelt.jsp" %> 59. <%@ include file="lincoln.jsp" %> 60. <%@ include file="jefferson.jsp" %> 61. </h:panelGrid> 62. </h:form> 63. </body> 64. </f:view> 65. </html>
| Listing 7-21. tabbedpane/web/jefferson.jsp 1. <h:panelGrid columns="2" columnClasses="presidentDiscussionColumn" 2. rendered="#{tp.jeffersonCurrent}"> 3. 4. <h:graphicImage value="/images/jefferson.jpg"/> 5. <h:outputText value="#{msgs.jeffersonDiscussion}" 6. style/> 7. 8. </h:panelGrid>
| Listing 7-22. tabbedpane/web/roosevelt.jsp 1. <h:panelGrid columns="2" columnClasses="presidentDiscussionColumn" 2. rendered="#{tp.rooseveltCurrent}"> 3. 4. <h:graphicImage value="/images/roosevelt.jpg"/> 5. <h:outputText value="#{msgs.rooseveltDiscussion}" 6. style/> 7. 8. </h:panelGrid>
| Listing 7-23. tabbedpane/web/lincoln.jsp 1. <h:panelGrid columns="2" columnClasses="presidentDiscussionColumn" 2. rendered="#{tp.lincolnCurrent}"> 3. 4. <h:graphicImage value="/images/lincoln.jpg"/> 5. <h:outputText value="#{msgs.lincolnDiscussion}" 6. style/> 7. 8. </h:panelGrid>
| Listing 7-24. tabbedpane/web/washington.jsp 1. <h:panelGrid columns="2" columnClasses="presidentDiscussionColumn" 2. rendered="#{tp.washingtonCurrent}"> 3. 4. <h:graphicImage value="/images/washington.jpg"/> 5. <h:outputText value="#{msgs.washingtonDiscussion}" 6. style/> 7. 8. </h:panelGrid>
| Listing 7-25. tabbedpane/src/java/com/corejsf/messages.properties 1. windowTitle=Mt. Rushmore Tabbed Pane 2. lincolnTooltip=Abraham Lincoln 3. lincolnTabText=Abraham Lincoln 4. lincolnDiscussion=President Lincoln was known as the Great Emancipator because \ 5. he was instrumental in abolishing slavery in the United States. He was born \ 6. into a poor family in Kentucky in 1809, elected president in 1860, and \ 7. assassinated by John Wilkes Booth in 1865. 8. 9. washingtonTooltip=George Washington 10. washingtonTabText=George Washington 11. washingtonDiscussion=George Washington was the first president of the United \ 12. States. He was born in 1732 in Virginia, was elected Commander in Chief of \ 13. the Continental Army in 1775, and forced the surrender of Cornwallis at Yorktown \ 14. in 1781. He was inaugurated on April 30, 1789. 15. 16. rooseveltTooltip=Theodore Roosevelt 17. rooseveltTabText=Theodore Roosevelt 18. rooseveltDiscussion=Theodore Roosevelt was the 26th president of the United \ 19. States. In 1901 he became president after the assassination of President \ 20. McKinley. At only 42, he was the youngest president in U.S. history. 21. 22. jeffersonTooltip=Thomas Jefferson 23. jeffersonTabText=Thomas Jefferson 24. jeffersonDiscussion=Thomas Jefferson, the 3rd U.S. president, was born in \ 25. 1743 in Virginia. Jefferson was tall and awkward, and was not known as a \ 26. great public speaker. Jefferson became minister to France in 1785, after \ 27. Benjamin Franklin held that post. In 1796, Jefferson was a reluctant \ 28. presidential candiate, and missed winning the election by a mere three votes. \ 29. He served as president from 1801 to 1809.
| Listing 7-26. tabbedpane/web/styles.css 1. body { 2. background: #eee; 3. } 4. .tabbedPaneHeader { 5. vertical-align: top; 6. text-align: left; 7. padding: 2px 2px 0px 2px; 8. } 9. .tabbedPaneText { 10. font-size: 1.0em; 11. font-style: regular; 12. padding: 3px; 13. border: thin solid CornflowerBlue; 14. } 15. .tabbedPaneTextSelected { 16. font-size: 1.0em; 17. font-style: regular; 18. padding: 3px; 19. background: PowderBlue; 20. border: thin solid CornflowerBlue; 21. } 22. .tabbedPane { 23. vertical-align: top; 24. text-align: left; 25. padding: 10px; 26. } 27. .displayPanel { 28. vertical-align: top; 29. text-align: left; 30. padding: 10px; 31. } 32. .tabbedPaneContent { 33. width: 100%; 34. height: 100%; 35. font-style: italic; 36. vertical-align: top; 37. text-align: left; 38. font-size: 1.2m; 39. } 40. .presidentDiscussionColumn { 41. vertical-align: top; 42. text-align: left; 43. }
| Listing 7-27. tabbedpane/web/WEB-INF/faces-config.xml 1. <?xml version="1.0"?> 2. <faces-config xmlns="http://java.sun.com/xml/ns/javaee" 3. xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 4. xsi:schemaLocation="http://java.sun.com/xml/ns/javaee 5. http://java.sun.com/xml/ns/javaee/web-facesconfig_1_2.xsd" 6. version="1.2"> 7. <application> 8. <resource-bundle> 9. <base-name>com.corejsf.messages</base-name> 10. <var>msgs</var> 11. </resource-bundle> 12. </application> 13. 14. <managed-bean> 15. <managed-bean-name>tp</managed-bean-name> 16. <managed-bean-class>com.corejsf.TabbedPane</managed-bean-class> 17. <managed-bean-scope>session</managed-bean-scope> 18. </managed-bean> 19. </faces-config>
| Listing 7-28. tabbedpane/src/java/com/corejsf/TabbedPane.java 1. package com.corejsf; 2. 3. import javax.faces.event.ActionEvent; 4. 5. public class TabbedPane { 6. private int index; 7. private static final int JEFFERSON_INDEX = 0; 8. private static final int ROOSEVELT_INDEX = 1; 9. private static final int LINCOLN_INDEX = 2; 10. private static final int WASHINGTON_INDEX = 3; 11. 12. private String[] tabs = { "jeffersonTabText", "rooseveltTabText", 13. "lincolnTabText", "washingtonTabText", }; 14. 15. private String[] tabTooltips = { "jeffersonTooltip", "rooseveltTooltip", 16. "lincolnTooltip", "washingtonTooltip" }; 17. 18. public TabbedPane() { 19. index = JEFFERSON_INDEX; 20. } 21. 22. // action listeners that set the current tab 23. 24. public void jeffersonAction(ActionEvent e) { index = JEFFERSON_INDEX; } 25. public void rooseveltAction(ActionEvent e) { index = ROOSEVELT_INDEX; } 26. public void lincolnAction(ActionEvent e) { index = LINCOLN_INDEX; } 27. public void washingtonAction(ActionEvent e) { index = WASHINGTON_INDEX; } 28. 29. // CSS styles 30. 31. public String getJeffersonStyle() { return getCSS(JEFFERSON_INDEX); } 32. public String getRooseveltStyle() { return getCSS(ROOSEVELT_INDEX); } 33. public String getLincolnStyle() { return getCSS(LINCOLN_INDEX); } 34. public String getWashingtonStyle() { return getCSS(WASHINGTON_INDEX); } 35. 36. private String getCSS(int forIndex) { 37. return forIndex == index ? "tabbedPaneTextSelected" : "tabbedPaneText"; 38. } 39. 40. // methods for determining the current tab 41. 42. public boolean isJeffersonCurrent() { return index == JEFFERSON_INDEX; } 43. public boolean isRooseveltCurrent() { return index == ROOSEVELT_INDEX; } 44. public boolean isLincolnCurrent() { return index == LINCOLN_INDEX; } 45. public boolean isWashingtonCurrent() { return index == WASHINGTON_INDEX; } 46. 47. // methods that get tooltips for titles 48. 49. public String getJeffersonTooltip() { 50. return com.corejsf.util.Messages.getString( 51. "com.corejsf.messages", tabTooltips[JEFFERSON_INDEX], null); 52. } 53. public String getRooseveltTooltip() { 54. return com.corejsf.util.Messages.getString( 55. "com.corejsf.messages", tabTooltips[ROOSEVELT_INDEX], null); 56. } 57. public String getLincolnTooltip() { 58. return com.corejsf.util.Messages.getString( 59. "com.corejsf.messages", tabTooltips[LINCOLN_INDEX], null); 60. } 61. public String getWashingtonTooltip() { 62. return com.corejsf.util.Messages.getString( 63. "com.corejsf.messages", tabTooltips[WASHINGTON_INDEX], null); 64. } 65. }
| |