Struts was donated to the Apache Foundation in 2000 and provides a framework incorporating Java EE best practices. Struts is widely adopted due to its support for internationalization, powerful custom tag library (the first releases were prior to JSTL and contained similar tag capabilities), declarative configuration, support for a variety of presentation technologies, form validation, and its maturity. Struts provides a front controller servlet and relies on other technology to provide the model (typically data access technologies such as Enterprise JavaBeans) and view (typically JSP) of the MVC design pattern. The Struts controller uses a configuration file (struts-config.xml) to map incoming requests to an action. An action receives form data as an ActionForm instance, and uses this data to execute the appropriate business logic. The configuration file allows logical names to be used to represent a view; thus, an action can forward control to the appropriate view using a logical name. Many web applications use JSP for the view, so Struts provides custom tag libraries that facilitate interaction with HTML forms. The following are key elements of the Struts framework: Action. Struts incorporates the service to worker design pattern, where an action commonly invokes methods on a business delegate using the data supplied by an ActionForm bean and uses the Struts controller to forward control to the appropriate view. A business delegate provides a reusable web independent facade for interacting with business services such as relational databases, messaging systems, and web services. ActionForm Bean. An ActionForm bean represents data shared between the view and the action. An ActionForm bean is available both for populating the view and providing input to an action. An ActionForm instance also has a validate method to allow input mapped from the view to be verified. Configuration File (struts-config.xml). The struts-config.xml file supports declaratively specifying the behavior of the Struts controller. Navigation (forwards) and behavior (actions) are specified in the Struts configuration file. Tag Libraries. The Struts tag libraries support internationalized applications and provide additional support for input forms interacting with the Struts framework. Struts incorporates the internationalization support in Java, where user-visible strings are specified in a language-specific resource bundle. Struts tag libraries such as form elements can specify a resource bundle key to allow the language-specific text to be displayed. This section is intended to serve as an overview of the Struts capabilities. Additional details can be found on the Apache Struts project page (http://struts.apache.org). Creating a Web Project with Struts Support Struts support can be added during project creation when using the New Web Application wizard. Struts support is enabled by selecting the Struts 1.2.7 checkbox in the wizard (see Figure 9-6). This allows additional Struts specific information to be specified: The Action Servlet Name text field specifies the name of the servlet entry for the Struts action servlet to be specified. The web.xml deployment descriptor contains a servlet entry for the action servlet, specifying the appropriate Struts specific parameters, such as the name of the servlet class and the path to the struts-config.xml configuration file. The Action URI Pattern combo box allows the appropriate patterns that should be mapped to the Struts action controller to be specified. This generates a corresponding web.xml servlet mapping entry to map the specified URI pattern to the action servlet. The Application Resource text field provides the capability to specify the resource bundle that will be used in the struts-config.xml file for localizing messages. The Add Struts TLD checkbox provides the capability to generate tag library descriptors for the Struts provided tag libraries. A tag library descriptor is an XML document that contains additional information about the entire tag library and each individual tag. Figure 9-6. Struts support in the New Web Application wizard After you complete the wizard, the standard web application project is augmented to support Struts development as follows (see Figure 9-7 for the Struts enabled web application project): struts-config.xml, tiles-defs.xml, validation.xml, and validatorrules.xml files are generated in the configuration files area. The strutsconfig.xml file is populated with the resource bundle specified in the wizard, a single global forward that references a welcomeStruts.jsp page, a controller entry for the tiles configuration file (tiles is a templating library), and a single action mapping (which provides the mapping to the generated struts welcome JSP). The other configuration files provide template files that can be used to start using additional Struts capabilities. The default index.jsp is augmented to refer to the welcomeStruts.jsp page using the appropriate action. The Struts libraries are added to the project and can be viewed in the Projects window from the project's Libraries node. The libraries are included by default when packaging the WAR file inthe WEB-INF/lib directory. If the libraries have already been deployed to the server or will instead be deployed as part of a Java EE application, the Project Properties dialog box can be used to control which libraries will be packaged with the WAR file. The web.xml file references the Struts action servlet. The resource bundle specified in the wizard is generated and populated with some default messages. If tag library descriptor generation was specified as part of the wizard, the tag library descriptors are generated. Figure 9-7. Projects window with a web application project using the JSF framework 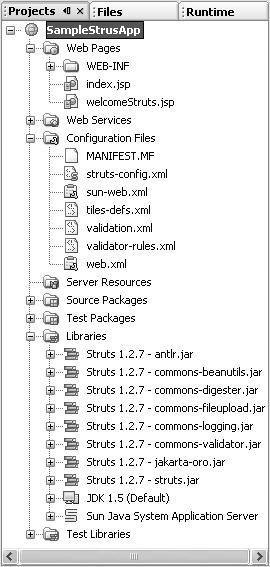 After you complete the wizard, code completion is enabled for Struts tag libraries, Struts Java classes, and Struts-related configuration files. Adding Struts support can also be done after a project has been created, through the Project Properties dialog box (see Figure 9-8), which you can open by rightclicking the project's main node and choosing Properties. Figure 9-8. Project Properties dialog box, Frameworks tab 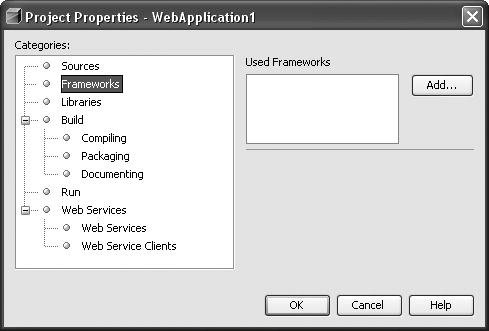 The Add button is used to select the desired web application support. The behavior after the initial launch is similar to what is done for a new project. Action Support An action class receives a request (as an ActionFormBean class), typically invokes business tier logic, and finally forwards control to the appropriate view object. The IDE provides a wizard to automate creation of action classes. To create an action class: Open the New Struts Action wizard by right-clicking the node of a Strutsenabled web application project and choosing New | File/Folder, selecting the Web category, and selecting the Struts Action template. In the Name and Location section of the wizard (shown in Figure 9-9), specify the name and package of the generated action class. The Struts configuration file and the action path must also be specified. The superclass for the generated action class can be selected from the combo box. The choices are: org.apache.struts.action.Action. An action provides the capability to map incoming requests to the appropriate business logic. The Struts controller will select an appropriate action for each request. This class serves as the superclass for the other classes and the controller logic is implemented in the execute method. org.apache.struts.actions.DispatchAction. A dispatch action uses the method specified in the request parameter named in the parameter property of the ActionMapping class. This allows a single action class to contain multiple methods for dispatching. The parameter property (shown in Figure 9-10) specifies the request parameter, which must match a method in the class and have the same signature as the execute method. Figure 9-9. Name and Location panel of the New Struts Action wizard Figure 9-10. New Struts Action Wizard, ActionForm Bean and Parameter page org.apache.struts.actions.MappingDispatchAction. A mapping dispatch action is similar to the dispatch action in that a different method can be used to dispatch the request. The difference is that the mapping dispatch action specifies the name of the method in the parameter property instead of the request parameter. This approach does not expose this mapping technique through application visible request parameters. The parameter property (shown in Figure 9-10) specifies the method name for dispatch. org.apache.struts.actions.LookupDispatchAction. A lookup dispatch action supports multiple submit buttons with the same name. The parameter mapping property specifies the name of the button. The value of the request parameter with the same button name is used to locate the key in the application resource bundle. The action class must provide a map between the button key and the method name in the class to dispatch. The last and optional step in the wizard is the ActionForm Bean and parameter step (see Figure 9-10), which allows you to specify the action form bean corresponding to the action. This step collects the following additional information used during the generation of the action mapping sections of the Struts configuration file. The ActionForm Bean name specifies the ActionBean that should be used asinput to the action. This list is populated from the action bean section of the Struts configuration file. The Input Resource and Input Action radio buttons determine where control is returned if a validation error occurs. The Scope radio buttons determine whether the ActionForm bean should be added using session or the request scope. The Attribute text field specifies the name of an attribute (in the scope defined above) where the ActionForm bean should be stored. The Validate checkbox specifies whether the validate method should be called prior to calling the action object. The Parameter text field allows an action specific context to be specified. The appropriate context for each action type is discussed in step 2. ActionForm Support An ActionForm bean provides a Java representation of an HTML form. The Struts framework provides the binding from request parameters to property methods. The ActionForm binding is performed using JavaBeans conventions to determine the appropriate method for each request parameter. The IDE provides a wizard to automate creation of an ActionForm bean. To create an ActionForm bean: Open the New Struts ActionForm wizard by right-clicking the node of a Struts-enabled web application project and choosing New | File/Folder, selecting the Web category, and selecting Struts Action Struts ActionForm Bean. In the Name and Location section of the wizard (shown in Figure 9-11), specify the name and package of the generated ActionForm class. The Struts configuration file must also be specified. The superclass for the generated ActionForm Form class can be selected from the combo box. The choices are: org.apache.struts.action.ActionForm. An ActionForm class may be associated with ActionMapping classes and represents the form data. An ActionForm class has several integration points into the Struts framework, notably the capability to reset the form data back to the default values and provide a way to validate user input. The ActionForm class is subclassed and must adhere to the JavaBeans convention for the mapping from the form data. org.apache.struts.validator.ValidatorForm. The validator form provides the capability to validate properties based on the validate.xml file. The validate.xml file uses the name of the action to match the form name in the validate.xml file. Adding Struts support using the wizards generates the appropriate entries into the struts-config.xml file to enable validation form support, which includes the resource bundle keys required by the validate.xml file. org.apache.struts.validator.ValidatorActionForm. The validator action form provides the capability to validate properties based on the validate.xml file. Similar to ValidatorForm, ValidatorActionForm uses the action path as the key into the validate.xml file form element's name. Using the specified Struts wizard adds the appropriate entries to enable the validator capabilities. This includes the resource bundle keys required by the validate.xml file. Figure 9-11. New Struts ActionForm wizard Click Finish. The wizard will generate the ActionForm classes along with an entry in the form beans section of the Struts configuration file. The generated file contains several TODO comments, which can be displayed using the To Do window.  | The Java node for the ActionForm class provides a wizard to help generate properties. A quick way to navigate to this node is to press Ctrl-Shift-1 from the Source Editor. The Bean Patterns node is a subnode that provides the capability to add various JavaBeans properties. |
Struts Configuration File Support In addition to code completion, the Struts configuration file provides several wizards launched from the context menu of the Source Editor. The wizards provided include: Add Action. The Add Action wizard collects the information necessary to generate an action element in the action mapping section. This wizard is used in conjunction with an existing action class and supports binding of an ActionForm bean. Add Forward/Include Action. These actions provide the capability to redirect to another action, possibly using a resource bundle. This feature can be used to change application behavior without modifying source code. Add Forward. A forward provides a way to associate a logical name with an existing action. The Forward wizard supports both global and local forwards and the capability to determine the forward through a resource bundle. Add Exception. An exception allows a mapping between a specific exception and an action to be specified. The exception can be specified either globally or locally and can be directed through a resource bundle. Add ActionForm Bean. Form Beans provide a mapping between user view forms and Java code and serve as input to actions. This wizard supports both strongly typed beans as well as dynamic beans (which support java.util.Map style access to view parameters). Add ActionForm Bean Property. The DynaActionForm class support allows property-based configuration of these classes using properties. This wizard supports both indexed and scalar values.  | When you open the Struts configuration file in the Source Editor, you can navigate to externally referenced artifacts (such as Java and JSP files) and internal references (such as form beans). Jumping to the source code is achieved by holding down the Ctrl key, moving the mouse over the identifier until it is underlined in blue, and then clicking it. For example, holding down the Ctrl key and moving the mouse over an action's input JSP name causes the JSP name to be underlined in blue (see Figure 9-12). Clicking the underlined identifier opens the JSP file in the Source Editor. |
Figure 9-12. Hyperlinking in struts-config.xml file  |